Created
October 23, 2016 07:18
-
-
Save Toxicable/1c736ed16c95bcdc7612e2c406b5ce0f to your computer and use it in GitHub Desktop.
How to upload files in angular 2
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/** | |
* Created by Fabian on 19/10/2016. | |
*/ | |
import { Component, ElementRef, Input } from '@angular/core'; | |
import { Http } from '@angular/http'; | |
@Component({ | |
selector: 'file-upload', | |
template: '<input type="file" [attr.multiple]="multiple ? true : null" (change)="upload()" >' | |
}) | |
export class FileUploadComponent { | |
constructor(private http: Http, | |
private el: ElementRef | |
) {} | |
@Input() multiple: boolean = false; | |
upload() { | |
let inputEl = this.el.nativeElement.firstElementChild; | |
if (inputEl.files.length == 0) return; | |
let files :FileList = inputEl.files; | |
const formData = new FormData(); | |
for(var i = 0; i < files.length; i++){ | |
formData.append(files[i].name, files[i]); | |
} | |
this.http | |
.post('/api/test/fileupload', formData) | |
.subscribe(); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
I have the same problem on primeng + ie9
fileupload.js line 89. files is undefined after choose one file
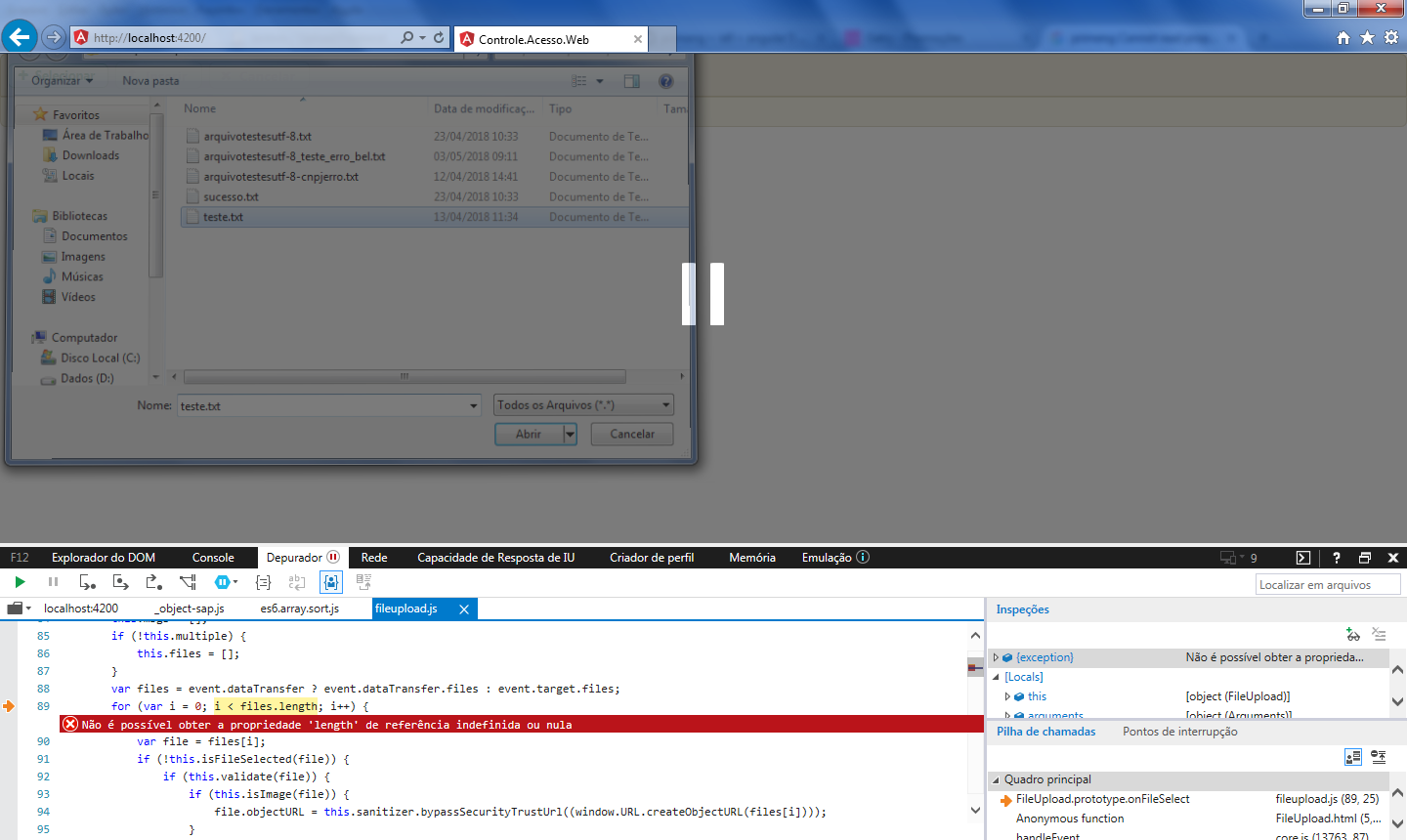