Last active
October 14, 2020 03:54
-
-
Save abhishtagatya/adc71e98dc51a45385798bf2198c96a1 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env python | |
import os | |
import numpy as np | |
import cv2 | |
import matplotlib.pyplot as plt | |
def canny_detector(image, weak=None, strong=None): | |
image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Grayscale | |
image = cv2.GaussianBlur(image, (5,5), 1.4) # Noise Reduction | |
gx, gy = ( | |
cv2.Sobel(np.float32(image), cv2.CV_64F, 1, 0, 3), | |
cv2.Sobel(np.float32(image), cv2.CV_64F, 0, 1, 3) | |
) # Calculating Gradients | |
mag, ang = cv2.cartToPolar(gx, gy, angleInDegrees=True) # Cartesian -> Polar | |
max_mag = np.max(mag) | |
if not weak:weak = max_mag * 0.1 # Minimum and Maximum | |
if not strong:strong = max_mag * 0.5 # Threshold | |
image_height, image_width = image.shape | |
for i in range(image_width): | |
for j in range(image_height): | |
gradient_ang = ang[j, i] | |
gradient_ang = abs(gradient_ang-180) if abs(gradient_ang) > 180 else abs(gradient_ang) | |
# Selecting neighbor of the target pixel | |
if gradient_ang <= 22.5: # X Axis | |
neighbor_i1, neighbor_j1 = i-1, j | |
neighbor_i2, neighbor_j2 = i+1, j | |
elif gradient_ang > 22.5 and gradient_ang <= (22.5+45): # Top Right | |
neighbor_i1, neighbor_j1 = i-1, j-1 | |
neighbor_i2, neighbor_j2 = i+1, j+1 | |
elif gradient_ang > (22.5+45) and gradient_ang <= (22.5+90): # Y Axis | |
neighbor_i1, neighbor_j1 = i, j-1 | |
neighbor_i2, neighbor_j2 = i, j+1 | |
elif gradient_ang > (22.5+90) and gradient_ang <= (22.5+135): # Top Left | |
neighbor_i1, neighbor_j1 = i-1, j+1 | |
neighbor_i2, neighbor_j2 = i+1, j-1 | |
elif gradient_ang > (22.5+135) and gradient_ang <= (22.5+180): # Cycle | |
neighbor_i1, neighbor_j1 = i-1, j | |
neighbor_i2, neighbor_j2 = i+1, j | |
# Non Maximum Suppresion Step | |
if image_width > neighbor_i1 >= 0 and image_height > neighbor_j1 >= 0: | |
if mag[j, i] < mag[neighbor_j1, neighbor_i1]: | |
mag[j, i] = 0 | |
continue | |
if image_width > neighbor_i2 >= 0 and image_height > neighbor_j2 >= 0: | |
if mag[j, i] < mag[neighbor_j2, neighbor_i2]: | |
mag[j, i] = 0 | |
weak_ids = np.zeros_like(image) | |
strong_ids = np.zeros_like(image) | |
ids = np.zeros_like(image) | |
# Double Threshold Step | |
for i in range(image_width): | |
for j in range(image_height): | |
gradient_mag = mag[j, i] | |
if gradient_mag < weak: | |
mag[j, i] = 0 | |
elif strong > gradient_mag >= weak: | |
ids[j, i] = 1 | |
else: | |
ids[j, i] = 2 | |
return mag | |
if __name__ == "__main__": | |
# Read and Pass Image | |
frame = cv2.imread('test.jpeg') | |
canny_image = canny_detector(frame) | |
# Image Plotting | |
plt.figure() | |
f, plts = plt.subplots(2, 1) | |
plts[0].imshow(frame) | |
plts[1].imshow(canny_image) | |
plt.show() | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
my result on a few tweaks of the code
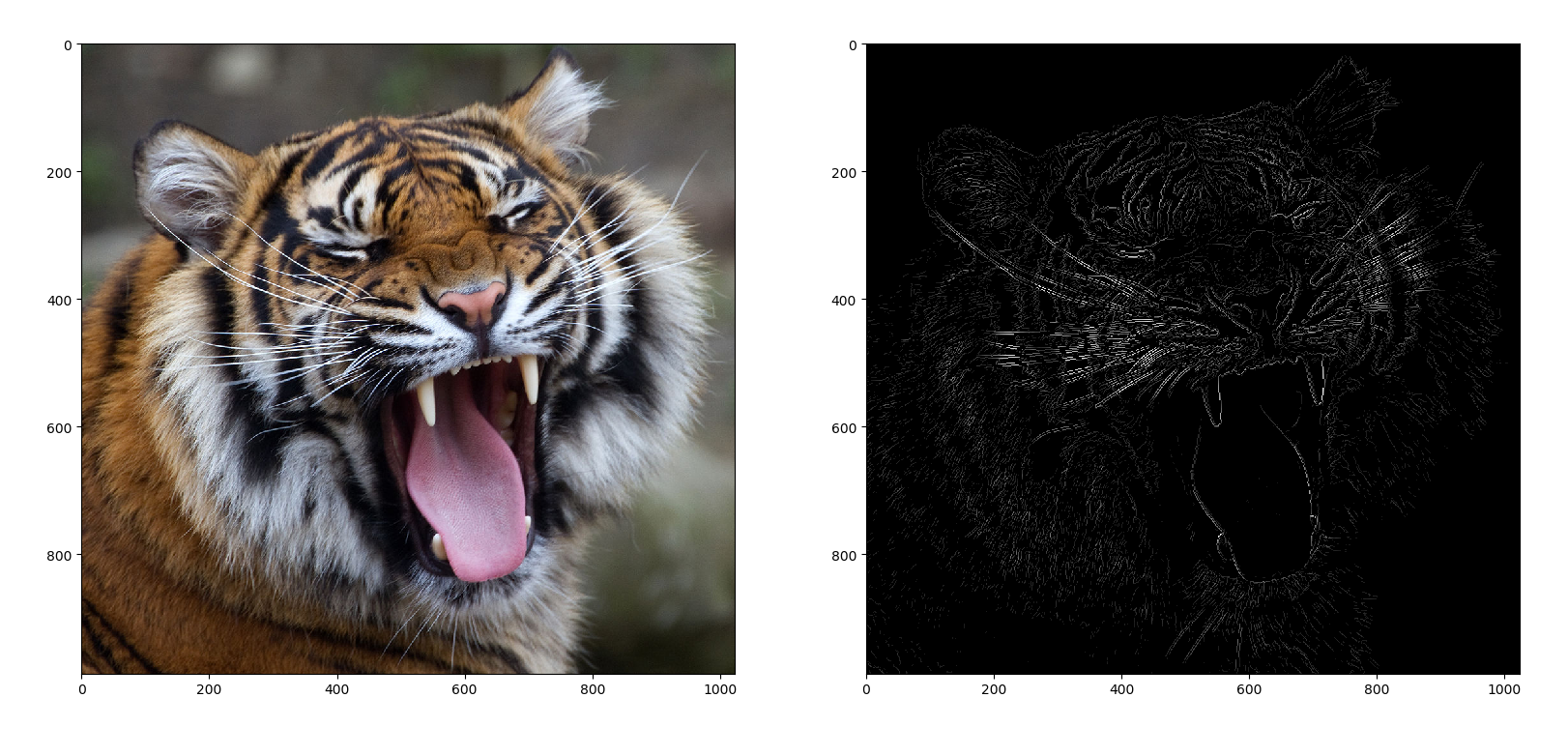