Last active
November 12, 2021 01:13
-
-
Save aclk/c12feff3e01f72bea59dd87e75b24fa1 to your computer and use it in GitHub Desktop.
Config proxy channel for IE and depandencies, work enjoy!
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Set-StrictMode -Version Latest | |
# $PSVersionTable.OS | |
# Write-Host | |
# User paths | |
if ($IsMacOS) { | |
$userPaths = @( | |
"$HOME/.bin", | |
"$HOME/.cargo/bin", | |
"$HOME/.gem/bin", | |
"/usr/local/opt/ruby/bin", | |
"/usr/local/sbin", | |
"/opt/homebrew/bin", | |
"/opt/homebrew/sbin" | |
) | |
# APP home | |
# cargo home | |
$env:CARGO_HOME = "$HOME/.cargo" | |
# rustup home | |
$env:RUSTUP_HOME = "$HOME/.rustup" | |
# nvm home | |
$env:NVM_DIR = "$HOME/.nvm" | |
} | |
# User alias | |
if ($IsWindows) { | |
Set-Alias -Name brew -Value scoop | |
} | |
if ($IsMacOS) { | |
if (Get-Command bat) { | |
Set-Alias -Name cat -Value bat | |
} | |
} | |
# Proxy ports | |
$windowsHttpProxyPort = 1080 | |
$macOSHttpProxyPort = 7890 | |
$macOSSocks5hProxyPort = 7891 | |
# Don't touch anything below if you're not orange enough. | |
# https://github.com/PowerShell/PowerShell-RFC/pull/186 | |
if ($IsMacOS) { | |
# if ($PSVersionTable.PSVersion -ge 7) { | |
# Write-Host "Please update PATH related script according to https://github.com/PowerShell/PowerShell-RFC/pull/186." | |
# return | |
# } | |
# macOS PATH | |
# $pathHelperResult = $(/usr/libexec/path_helper).Split('"')[1] | |
$originalPath = $env:PATH | |
try { | |
$env:PATH = $null | |
foreach ($userPath in $userPaths) { | |
$env:PATH += $userPath + ":" | |
} | |
$env:PATH += $originalPath | |
# $env:PATH += $pathHelperResult | |
} | |
catch { | |
$env:PATH = $originalPath | |
} | |
} | |
if ($IsMacOS -Or $IsLinux) { | |
# Set GPG tty to fix git code signing error: gpg failed to sign the data | |
$env:GPG_TTY = $(tty) | |
} | |
# Modules | |
Import-Module posh-git | |
Import-Module nvm | |
# Functions | |
function Enable-HttpProxy { | |
if ($IsMacOS) { | |
$env:http_proxy = $env:https_proxy = $env:all_proxy = "http://localhost:$macOSHttpProxyPort" | |
} | |
elseif ($IsWindows) { | |
$env:http_proxy = $env:https_proxy = $env:all_proxy = "http://localhost:$windowsHttpProxyPort" | |
} | |
else { | |
New-Object System.PlatformNotSupportedException | |
} | |
} | |
function Enable-Socks5hProxy { | |
if ($IsMacOS) { | |
$env:http_proxy = $env:https_proxy = $env:all_proxy = "socks5h://localhost:$macOSSocks5hProxyPort" | |
} | |
else { | |
New-Object System.PlatformNotSupportedException | |
} | |
} | |
function Enable-GitGlobalHttpProxy { | |
if ($IsMacOS) { | |
git config --global http.proxy "http://localhost:$macOSHttpProxyPort" | |
} | |
elseif ($IsWindows) { | |
git config --global http.proxy "http://localhost:$windowsHttpProxyPort" | |
} | |
else { | |
New-Object System.PlatformNotSupportedException | |
} | |
} | |
function Disable-Proxy { | |
if ($IsMacOS -or $IsWindows) { | |
$env:http_proxy = $env:https_proxy = $env:all_proxy = $null | |
git config --global --unset http.proxy | |
} | |
else { | |
New-Object System.PlatformNotSupportedException | |
} | |
} | |
# Enable proxy by default. | |
Enable-HttpProxy | |
Enable-GitGlobalHttpProxy |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# Culture | |
Set-Culture -CultureInfo en-us | |
# Chocolatey profile | |
$ChocolateyProfile = "$env:ChocolateyInstall\helpers\chocolateyProfile.psm1" | |
if (Test-Path($ChocolateyProfile)) { | |
Import-Module "$ChocolateyProfile" | |
} | |
# Check git | |
$DefaultErrorActionPreference = $ErrorActionPreference | |
$ErrorActionPreference= 'silentlycontinue' | |
(git -version) | Out-Null | |
if ($LASTEXITCODE -ne 0) { $gitInstalled = $true } | |
$ErrorActionPreference = $DefaultErrorActionPreference | |
# If behind a proxy, uncomment the lines below | |
# [System.Net.WebRequest]::DefaultWebProxy = New-Object System.Net.WebProxy('http://hostname:port') | |
# [System.Net.WebRequest]::DefaultWebProxy.Credentials = [System.Net.CredentialCache]::DefaultNetworkCredentials | |
# [System.Net.WebRequest]::DefaultWebProxy.BypassProxyOnLocal = $true | |
# [System.Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Tls12 # required for PS v5.1 and below | |
# Set proxy settings for git | |
# if ($gitInstalled) | |
# { | |
# git config --global https.proxy http://username:password@proxy:port | |
# git config --global http.sslVerify false | |
# } | |
# Modules | |
Import-Module posh-git # May throw an exception if git is not installed | |
Import-Module oh-my-posh | |
# Console modification | |
if($host.Name -eq 'ConsoleHost' -and $PSVersionTable.PSVersion.Major -ge 6) | |
{ | |
# Apply Theme (Font: DejaVuSansMono) | |
Set-Theme Operator | |
$ThemeSettings.GitSymbols.BranchSymbol = [char]::ConvertFromUtf32(0xE0A0) | |
$ThemeSettings.GitSymbols.BranchIdenticalStatusToSymbol = [char]::ConvertFromUtf32(0x25CF) | |
$ThemeSettings.GitSymbols.BranchUntrackedSymbol = [char]::ConvertFromUtf32(0x25CB) | |
$ThemeSettings.GitSymbols.FailedCommandSymbol = [char]::ConvertFromUtf32(0x2a2f) | |
$ThemeSettings.GitSymbols.PromptIndicator = [char]::ConvertFromUtf32(0x276f) | |
# Required for custom themes | |
$getStackContext = { Get-Location -Stack } | |
} | |
# Useful shortcuts for traversing directories | |
function cd.. { Set-Location .. } | |
function cd... { Set-Location ..\.. } | |
function cd.... { Set-Location ..\..\.. } | |
# Compute file hashes - useful for checking successful downloads | |
function md5 { Get-FileHash -Algorithm MD5 $args } | |
function sha1 { Get-FileHash -Algorithm SHA1 $args } | |
function sha256 { Get-FileHash -Algorithm SHA256 $args } | |
# Quick shortcut to start notepad | |
function n { notepad $args } | |
# Quick shortcut to start Explorer on current directory | |
function w { explorer . } | |
# Call Powershell ISE | |
function ise { Start-Process powershell_ise.exe } | |
# Get time | |
function Get-Time { return $(get-date | ForEach-Object { $_.ToLongTimeString() } ) } | |
# Use man for Get-Help | |
function man { Get-Help -Name $args -Full } | |
# Drive shortcuts | |
function HKLM: { Set-Location HKLM: } | |
function HKCU: { Set-Location HKCU: } | |
function Env: { Set-Location Env: } | |
# Simple function to start a new elevated process. If arguments are supplied then | |
# a single command is started with admin rights; if not then a new admin instance | |
# of PowerShell is started. | |
function admin | |
{ | |
$exe = "powershell.exe" | |
if($PSVersionTable.PSVersion.Major -gt 5) { $exe = "pwsh.exe" } | |
if ($args.Count -gt 0) | |
{ | |
$argList = "& '" + $args + "'" | |
Start-Process "$psHome\$exe" -Verb runAs -ArgumentList $argList | |
} | |
else | |
{ | |
Start-Process "$psHome\$exe" -Verb runAs | |
} | |
} | |
# Restart powershell scope | |
function Start-NewScope | |
{ | |
param($Prompt = $null) Write-Host “Starting New Scope” | |
if ($null -ne $Prompt) | |
{ if ($Prompt -is [ScriptBlock]) | |
{ | |
$null = New-Item function:Prompt -Value $Prompt -force | |
}else | |
{ function Prompt {“$Prompt”} | |
} | |
} | |
$host.EnterNestedPrompt() | |
} | |
Set-Alias ns Start-NewScope | |
Clear-Host |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
$defalut_proxy = 'http://127.0.0.1:1080'; | |
if ([System.Environment]::GetEnvironmentVariable('DEFAULT_PROXY') -ne $null) { | |
$defalut_proxy = [System.Environment]::GetEnvironmentVariable('DEFAULT_PROXY'); | |
} | |
function Env-Proxy($operate, $proxy = $defalut_proxy) { | |
if ($operate -eq "get") { | |
return $env:HTTP_PROXY; | |
} | |
elseif ($operate -eq "set") { | |
$env:HTTP_PROXY = $proxy; | |
$env:HTTPS_PROXY = $proxy; | |
return "Success"; | |
} | |
elseif ($operate -eq "del") { | |
if ($env:HTTP_PROXY -ne $null) { | |
Remove-Item env:HTTP_PROXY | |
Remove-Item env:HTTPS_PROXY | |
} | |
return "Success"; | |
} | |
} | |
function Git-Proxy($operate, $proxy = $defalut_proxy) { | |
if ($operate -eq "get") { | |
return (git config --global --get http.proxy); | |
} | |
elseif ($operate -eq "set") { | |
git config --global http.proxy $proxy; | |
git config --global https.proxy $proxy; | |
return "Success"; | |
} | |
elseif ($operate -eq "del") { | |
git config --global --unset http.proxy | |
git config --global --unset https.proxy | |
return "Success"; | |
} | |
} | |
function Node-Proxy($operate, $proxy = $defalut_proxy) { | |
if ($operate -eq "get") { | |
$yarn_proxy = (yarn config get proxy); | |
if ($yarn_proxy -eq "undefined") { | |
return $null; | |
} | |
else { | |
return $yarn_proxy; | |
} | |
} | |
elseif ($operate -eq "set") { | |
npm config set proxy $proxy | Out-Null; | |
npm config set https-proxy $proxy | Out-Null; | |
yarn config set proxy $proxy | Out-Null; | |
yarn config set https-proxy $proxy | Out-Null; | |
return "Success"; | |
} | |
elseif ($operate -eq "del") { | |
npm config delete proxy | Out-Null | |
npm config delete https-proxy | Out-Null | |
yarn config delete proxy | Out-Null | |
yarn config delete https-proxy | Out-Null | |
return "Success"; | |
} | |
} | |
function Composer-Proxy($operate, $proxy = $defalut_proxy) { | |
return (Env-Proxy $operate $proxy); | |
} | |
function Scoop-Proxy($operate, $proxy = $defalut_proxy) { | |
if ($operate -eq "get") { | |
$scoop_proxy = (scoop config proxy); | |
if ($scoop_proxy -eq "'proxy' is not set") { | |
return $null; | |
} | |
else { | |
return $scoop_proxy; | |
} | |
} | |
elseif ($operate -eq "set") { | |
scoop config proxy $proxy.Substring(7) | Out-Null | |
return "Success"; | |
} | |
elseif ($operate -eq "del") { | |
scoop config rm proxy | Out-Null | |
return "Success"; | |
} | |
} | |
# 脚本部分 | |
if ($args[0] -in "set", "get", "del") { | |
$used_proxy = $defalut_proxy; | |
if ($args[2] -ne $null) { | |
$used_proxy = $args[2]; | |
} | |
if ($args[1] -eq "env") { | |
Write-Output (Env-Proxy $args[0] $used_proxy); | |
} | |
if ($args[1] -eq "git") { | |
Write-Output (Git-Proxy $args[0] $used_proxy); | |
} | |
if ($args[1] -in "npm", "node", "yarn") { | |
Write-Output (Node-Proxy $args[0] $used_proxy); | |
} | |
if ($args[1] -eq "composer") { | |
Write-Output (Composer-Proxy $args[0] $used_proxy); | |
} | |
if ($args[1] -eq "all" -or $args[1] -eq $null) { | |
Write-Output ("Env: " + (Env-Proxy $args[0] $used_proxy)); | |
Write-Output ("Git: " + (Git-Proxy $args[0] $used_proxy)); | |
Write-Output ("Node: " + (Node-Proxy $args[0] $used_proxy)); | |
Write-Output ("Composer: " + (Composer-Proxy $args[0] $used_proxy)); | |
Write-Output ("Scoop: " + (Scoop-Proxy $args[0] $used_proxy)); | |
} | |
if ($args[1] -eq "proxy") { | |
if ($args[0] -eq "set") { | |
[System.Environment]::SetEnvironmentVariable('DEFAULT_PROXY', $args[2], [System.EnvironmentVariableTarget]::User); | |
} | |
elseif ($args[0] -eq "get") { | |
[System.Environment]::GetEnvironmentVariable('DEFAULT_PROXY', [System.EnvironmentVariableTarget]::User); | |
} | |
elseif ($args[0] -eq "del") { | |
[System.Environment]::SetEnvironmentVariable('DEFAULT_PROXY', '', [System.EnvironmentVariableTarget]::User); | |
} | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
try { $null = gcm pshazz -ea stop; pshazz init 'default' } catch { } | |
function set_proxy_variable { | |
$proxy = 'http://127.0.0.1:1080' | |
# temporary | |
$env:HTTP_PROXY = $proxy | |
$env:HTTPS_PROXY = $proxy | |
# forever | |
# [System.Environment]::SetEnvironmentVariable("HTTP_PROXY", $proxy, "User") | |
# [System.Environment]::SetEnvironmentVariable("HTTPS_PROXY", $proxy, "User") | |
Write-Host "`n OPEN powershell proxy channel!`n" | |
} | |
function unset_proxy_variable { | |
# temporary | |
Remove-Item env:HTTP_PROXY | |
Remove-Item env:HTTPS_PROXY | |
# forever | |
# [Environment]::SetEnvironmentVariable('http_proxy', $null, 'User') | |
# [Environment]::SetEnvironmentVariable('https_proxy', $null, 'User') | |
Write-Host "`n CLOSE powershell proxy channel!`n" | |
} | |
Set-Alias proxy set_proxy_variable | |
Set-Alias unproxy unset_proxy_variable | |
Invoke-Expression (&starship init powershell) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Plz follow as this setups
notepad $PROFILE
and press enter keyproxy
orunproxy
can active proxy featureLike this:
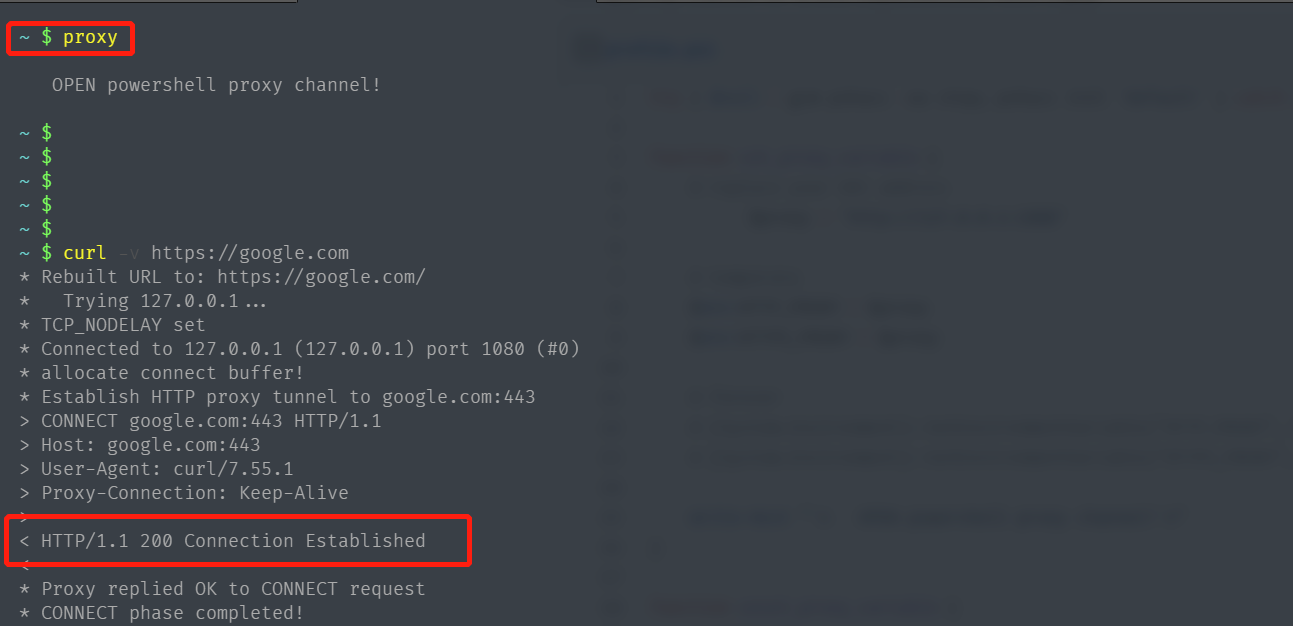