Last active
June 11, 2021 15:13
-
-
Save adrhill/6b3e78adaf3d8a681ac5e1bfeed2f153 to your computer and use it in GitHub Desktop.
Mandelbrot set in terminal
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# ]add https://github.com/IanButterworth/VideoInTerminal.jl | |
# ]add ImageCore | |
# ]add ColorSchemes | |
using VideoInTerminal | |
using ImageCore | |
using ColorSchemes | |
""" | |
Count number of Mandelbrot iterations until `threshold` is crossed, | |
up to a maximum of `maxits`. | |
""" | |
function iteration_count(c::Complex; threshold=4, maxits=100) | |
z = 0 | |
for i in 1:maxits | |
z = z^2 + c | |
if abs(z) > threshold | |
return i | |
end | |
end | |
return maxits | |
end | |
""" | |
Turn iteration count on Mandelbrot set into color image. | |
""" | |
function histogram_coloring(ics::AbstractArray{<:Integer}) | |
# Construct simple histogram with bin for each value | |
counts = zeros(Int, prod(size(ics))) | |
for val in unique(ics) | |
counts[val] = count(ic -> ic == val, ics) | |
end | |
# Get hues from normalized cumulative sum of iteration counts | |
cs = cumsum(counts) / sum(counts) | |
hues = map(ic -> cs[ic], ics) | |
return get(ColorSchemes.inferno, hues) | |
end | |
""" | |
Draw a frame of the Mandelbrot fractal around (cx, cy). | |
""" | |
function mb_fractal(width; cx=0, cy=0, h=100, w=100) | |
rowrange = range(-width / 2, width / 2; length=h) .+ cy | |
colrange = range(-width / 2, width / 2; length=w) .+ cx | |
cmat = [Complex(c, r) for r in rowrange, c in colrange] | |
ic = iteration_count.(cmat) | |
return histogram_coloring(ic) | |
end | |
""" | |
Zoom in on seahorse valley (https://mathworld.wolfram.com/SeaHorseValley.html). | |
""" | |
function mandelbrot_zoom(; frames=500) | |
zoomrange = 2 .^ range(3, -10; length=frames) | |
return play(map(w -> mb_fractal(w; cx=-0.75, cy=-0.1), zoomrange)) | |
end | |
mandelbrot_zoom() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
What it looks like:
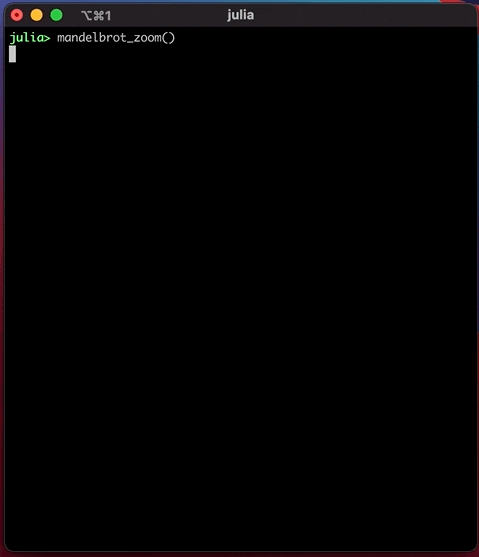