Last active
May 21, 2023 07:41
-
-
Save akasakakona/d5645d94858dfe6b74df7ff743b8cb0b to your computer and use it in GitHub Desktop.
A batch script written for those who are not comfortable with command lines when downloading YouTube videos.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
@echo off | |
echo Checking for update... | |
if exist youtube-dl.exe ( goto :computeSum ) else ( | |
echo It seems like you don't have youtube-dl on your computer. | |
goto :download | |
) | |
:computeSum | |
setlocal enableextensions enabledelayedexpansion | |
set /a counter = 0 | |
for /f "delims=" %%i in ('CertUtil -hashfile .\youtube-dl.exe SHA256') DO ( | |
set /a counter+=1 | |
if !counter!==2 ( | |
set hash1=%%i | |
) | |
) | |
endlocal && set hash1=%hash1% | |
echo Downloading checksum file... | |
echo. | |
powershell -Command "Invoke-WebRequest https://github.com/yt-dlp/yt-dlp/releases/latest/download/SHA2-256SUMS -OutFile tmp.txt" | |
echo Finished! | |
echo. | |
setlocal enableextensions enabledelayedexpansion | |
set /a counter = 0 | |
for /f "delims= " %%i in (tmp.txt) DO ( | |
set /a counter+=1 | |
if !counter!==2 ( | |
set hash2=%%i | |
) | |
) | |
endlocal && set hash2=%hash2% | |
del tmp.txt | |
if %hash1%==%hash2% ( goto :youtubeDL ) else ( goto :download ) | |
:download | |
echo Automatically downloading/upgrading youtube-dl.exe... | |
echo. | |
powershell -Command "Invoke-WebRequest https://github.com/yt-dlp/yt-dlp/releases/latest/download/yt-dlp.exe -OutFile youtube-dl.exe" | |
echo Finished! | |
echo. | |
:youtubeDL | |
set /p DIRECTORY=Where do you want to store your file (Enter a directory here, a directory should look something like C:\Users\Rick\xxx): | |
echo. | |
:while | |
set /p URL=What is the link to the video: | |
echo. | |
youtube-dl.exe -o "%DIRECTORY%\\%%(title)s.%%(ext)s" -f "bestvideo[ext=mp4]+bestaudio[ext=m4a]/mp4" "%URL%" | |
echo Download Complete! | |
set /p INPUT=Would you like to download more videos? (y/n): | |
if "%INPUT%"=="y" ( | |
echo. | |
goto :while | |
) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/bin/bash | |
computeSum() { | |
hash1=$(shasum -a 256 youtube-dl | cut -d " " -f 1) | |
echo Downloading checksum file... | |
echo | |
curl -sSL -o tmp.txt https://github.com/yt-dlp/yt-dlp/releases/latest/download/SHA2-256SUMS | |
echo Finished! | |
echo | |
hash2=$(grep 'yt-dlp$' tmp.txt | cut -d ' ' -f1) | |
rm tmp.txt | |
if [ "$hash1" = "$hash2" ]; then | |
youtubeDL | |
else | |
download | |
fi | |
} | |
download() { | |
echo Automatically downloading/upgrading youtube-dl... | |
echo | |
curl -sSL -o youtube-dl https://github.com/yt-dlp/yt-dlp/releases/latest/download/yt-dlp | |
echo Finished! | |
echo | |
chmod +x youtube-dl | |
youtubeDL | |
} | |
youtubeDL() { | |
read -p "Where do you want to store your file (Enter a directory here, a directory should look something like /Users/xxx): " DIRECTORY | |
if [ -z "$DIRECTORY" ]; then | |
DIRECTORY=$PWD | |
fi | |
while true; do | |
read -p "What is the link to the video: " URL | |
echo | |
./youtube-dl -o "$DIRECTORY/%(title)s.%(ext)s" -f "bestvideo[ext=mp4]+bestaudio[ext=m4a]/mp4" "$URL" | |
echo Download Complete! | |
read -p "Would you like to download more videos? (y/n): " INPUT | |
echo | |
if [ "$INPUT" != "y" ]; then | |
break | |
fi | |
done | |
} | |
echo Checking for update... | |
if [ -e youtube-dl ]; then | |
computeSum | |
else | |
echo "It seems like you don't have youtube-dl on your computer." | |
download | |
fi |
Updated to yt-dlp
instead of youtube-dl
since it's been years since they last updated their prebuilt binaries.
Added a shell script for Linux. Theoretically it also works on MacOS.
I'm honestly too lazy to rewrite this myself so I used ChatGPT, thus there could be bugs :p
Although... if you're using Linux, you probably don't need this lol.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
A quick demonstration of how this works. If you have more questions feel free to comment below and I'm happy to help.
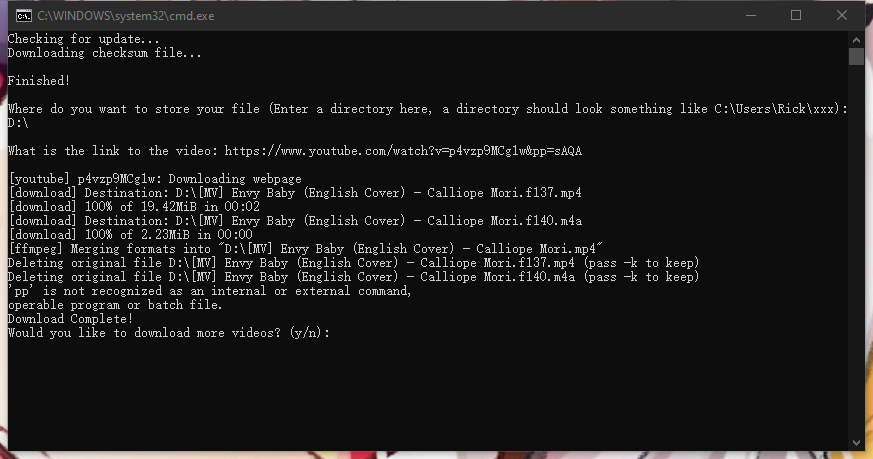