Last active
November 7, 2023 08:46
-
-
Save alfredplpl/a175aea0a97b7e90f6f3d8ccb4549cd7 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from diffusers import DiffusionPipeline | |
import torch | |
from consistencydecoder import ConsistencyDecoder | |
from PIL import Image | |
import numpy as np | |
pipe = DiffusionPipeline.from_pretrained("SimianLuo/LCM_Dreamshaper_v7", torch_dtype=torch.float32) | |
decoder_consistency = ConsistencyDecoder(device="cuda:0") # Model size: 2.49 GB | |
# To save GPU memory, torch.float16 can be used, but it may compromise image quality. | |
pipe.to(torch_device="cuda", torch_dtype=torch.float32) | |
prompt = "Self-portrait oil painting, a beautiful cyborg with golden hair, 8k" | |
# Can be set to 1~50 steps. LCM support fast inference even <= 4 steps. Recommend: 1~8 steps. | |
num_inference_steps = 10 | |
latent = pipe(prompt=prompt, num_inference_steps=num_inference_steps, guidance_scale=8.0, output_type="latent") | |
latent=latent.images[0]/0.18215 | |
latent=latent.unsqueeze(0) | |
with torch.no_grad(),torch.amp.autocast("cuda"): | |
consistent_latent = decoder_consistency(latent,schedule=[1.0]) | |
image = consistent_latent[0].cpu().numpy() | |
image = (image + 1.0) * 127.5 | |
image = image.clip(0, 255).astype(np.uint8) | |
image = Image.fromarray(image.transpose(1, 2, 0)) | |
image.save("con.png") |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
result:
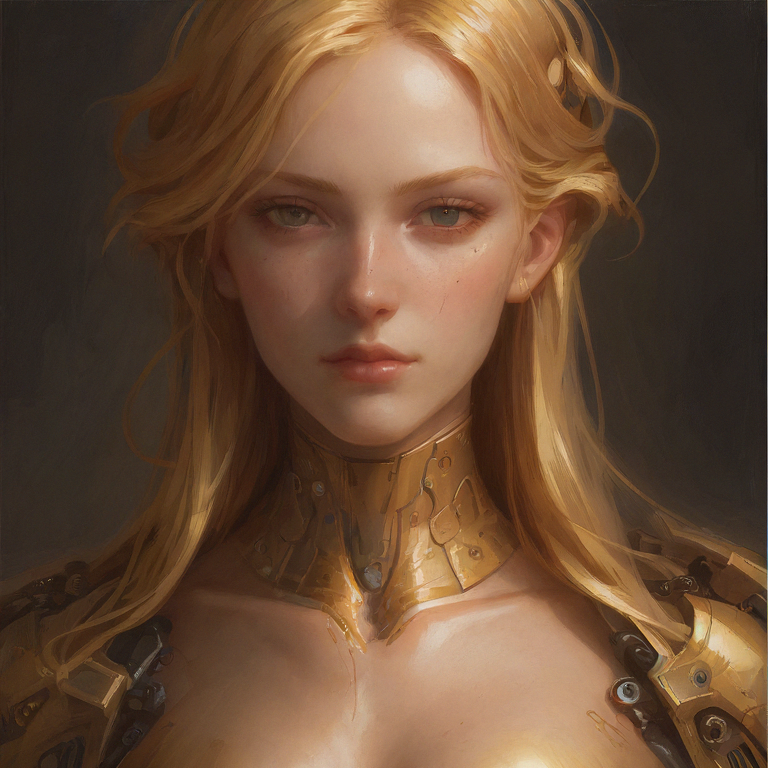