Last active
October 17, 2020 11:06
-
-
Save andi34/4d8c7c652efca33fa2867b15af54c063 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <ESP8266WiFi.h> | |
#include <ESP8266WebServer.h> | |
#include "DHT.h" | |
// Uncomment one of the lines below for whatever DHT sensor type you're using! | |
//#define DHTTYPE DHT11 // DHT 11 | |
//#define DHTTYPE DHT21 // DHT 21 (AM2301) | |
#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321 | |
/*Put your SSID & Password*/ | |
const char* ssid = ""; // Enter SSID here | |
const char* password = ""; //Enter Password here | |
ESP8266WebServer server(80); | |
// DHT Sensor | |
// NOTE: On ESP8266 avoid using "D8" as the ESP8266 won't boot and | |
// you won't be able to upload a Sketch if D8 is connected. | |
uint8_t DHTPin = D2; | |
// Initialize DHT sensor. | |
DHT dht(DHTPin, DHTTYPE); | |
float Temperature; | |
float Fahrenheit; | |
float Humidity; | |
float HeatindexC; | |
float HeatindexF; | |
void setup() { | |
Serial.begin(115200); | |
delay(100); | |
pinMode(DHTPin, INPUT); | |
dht.begin(); | |
Serial.println("Connecting to "); | |
Serial.println(ssid); | |
//connect to your local wi-fi network | |
WiFi.begin(ssid, password); | |
//check wi-fi is connected to wi-fi network | |
while (WiFi.status() != WL_CONNECTED) { | |
delay(1000); | |
Serial.print("."); | |
} | |
Serial.println(""); | |
Serial.println("WiFi connected..!"); | |
Serial.print("Got IP: "); Serial.println(WiFi.localIP()); | |
server.on("/", handle_OnConnect); | |
server.onNotFound(handle_NotFound); | |
server.begin(); | |
Serial.println("HTTP server started"); | |
} | |
void loop() { | |
server.handleClient(); | |
} | |
void handle_OnConnect() { | |
Temperature = dht.readTemperature(); // Gets the values of the temperature | |
Fahrenheit = dht.readTemperature(true); // Gets the values of the temperature in Fahrenheit | |
Humidity = dht.readHumidity(); // Gets the values of the humidity | |
HeatindexC = dht.computeHeatIndex(Temperature, Humidity, false); | |
HeatindexF = dht.computeHeatIndex(Fahrenheit, Humidity); | |
server.send(200, "text/html", SendHTML(Temperature, Fahrenheit, Humidity, HeatindexC, HeatindexF)); | |
} | |
void handle_NotFound() { | |
server.send(404, "text/plain", "Not found"); | |
} | |
String SendHTML(float Temperaturestat, float Fahrenheitstat, float Humiditystat, float HeatindexCstat, float HeatindexFstat) { | |
String ptr = "<!DOCTYPE html> <html>\n"; | |
ptr += "<head><meta name=\"viewport\" content=\"width=device-width, initial-scale=1.0, user-scalable=no\">\n"; | |
ptr += "<meta charset=\"UTF-8\" />\n"; | |
ptr += "<link href=\"https://fonts.googleapis.com/css?family=Open+Sans:300,400,600\" rel=\"stylesheet\">\n"; | |
ptr += "<title>Weather Report</title>\n"; | |
ptr += "<style>html { font-family: 'Open Sans', sans-serif; display: block; margin: 0px auto; text-align: center;color: #333333;}\n"; | |
ptr += "body{margin-top: 50px;}\n"; | |
ptr += "h1 {margin: 20px auto 0px;}\n"; | |
ptr += "hr {width: 25%; margin-top: 1em; margin-bottom: 2em;}\n"; | |
ptr += "@media (max-width: 767px) {hr {width: 50%;}}\n"; | |
ptr += ".side-by-side{display: inline-block;vertical-align: middle;position: relative;}\n"; | |
ptr += ".humidity-icon{background-color: #3498db;width: 30px;height: 30px;border-radius: 50%;line-height: 36px;}\n"; | |
ptr += ".humidity-text{font-weight: 600;padding-left: 15px;font-size: 19px;width: 160px;text-align: left;}\n"; | |
ptr += ".humidity{font-weight: 300;font-size: 60px;color: #3498db;}\n"; | |
ptr += ".temperature-icon{background-color: #f39c12;width: 30px;height: 30px;border-radius: 50%;line-height: 40px;}\n"; | |
ptr += ".temperature-text{font-weight: 600;padding-left: 15px;font-size: 19px;width: 160px;text-align: left;}\n"; | |
ptr += ".temperature{font-weight: 300;font-size: 60px;color: #f39c12;}\n"; | |
ptr += ".superscript{font-size: 17px;font-weight: 600;position: absolute;right: -20px;top: 15px;}\n"; | |
ptr += ".data{padding: 10px;}\n"; | |
ptr += "</style>\n"; | |
// Add date and time | |
ptr += "<script type=\"text/javascript\">\n"; | |
ptr += "var monNames = new Array('January', 'February', 'March',\n"; | |
ptr += "'April', 'May', 'June',\n"; | |
ptr += "'July', 'August', 'September',\n"; | |
ptr += "'October', 'November', 'December');\n"; | |
ptr += "var dayNames = new Array('Sunday', 'Monday', 'Tuesday', 'Wednesday',\n"; | |
ptr += "'Thursday', 'Friday', 'Saturday');\n"; | |
ptr += "function t4(j) {\n"; | |
ptr += "return (j < 1000 ? j+1900 : j);\n"; | |
ptr += "}\n"; | |
ptr += "// Freeware (c) 1999 by Thomas Salvador, http://www.brauchbar.de\n"; | |
ptr += "var now = new Date();\n"; | |
ptr += "var x= now.getHours();\n"; | |
ptr += "if (x <10) {x=\"0\"+x;}\n"; | |
ptr += "var y= now.getMinutes();\n"; | |
ptr += "if (y <10) {y=\"0\"+y;}\n"; | |
ptr += "var z= now.getSeconds();\n"; | |
ptr += "if (z <10) {z=\"0\"+z;}\n"; | |
ptr += "\n"; | |
ptr += "document.write('<h1>Weather Report</h1>');\n"; | |
ptr += "document.write('</br>');\n"; | |
ptr += "document.write(dayNames[now.getDay()]);\n"; | |
ptr += "document.write(', ', now.getDate());\n"; | |
ptr += "document.write('. ', monNames[now.getMonth()]);\n"; | |
ptr += "document.write(' ', t4(now.getYear()));\n"; | |
ptr += "document.write('</br>');\n"; | |
ptr += "document.write('Time: ', x+\":\"+y+\":\"+z);\n"; | |
ptr += "document.write('<hr>');\n"; | |
ptr += "</script>\n"; | |
// Add date and time end | |
ptr += "</head>\n"; | |
ptr += "<body>\n"; | |
ptr += "<div id=\"webpage\">\n"; | |
ptr += "<div class=\"data\">\n"; | |
ptr += "<div class=\"side-by-side temperature-icon\">\n"; | |
ptr += "<svg version=\"1.1\" id=\"Layer_1\" xmlns=\"http://www.w3.org/2000/svg\" xmlns:xlink=\"http://www.w3.org/1999/xlink\" x=\"0px\" y=\"0px\"\n"; | |
ptr += "width=\"9.915px\" height=\"22px\" viewBox=\"0 0 9.915 22\" enable-background=\"new 0 0 9.915 22\" xml:space=\"preserve\">\n"; | |
ptr += "<path fill=\"#FFFFFF\" d=\"M3.498,0.53c0.377-0.331,0.877-0.501,1.374-0.527C5.697-0.04,6.522,0.421,6.924,1.142\n"; | |
ptr += "c0.237,0.399,0.315,0.871,0.311,1.33C7.229,5.856,7.245,9.24,7.227,12.625c1.019,0.539,1.855,1.424,2.301,2.491\n"; | |
ptr += "c0.491,1.163,0.518,2.514,0.062,3.693c-0.414,1.102-1.24,2.038-2.276,2.594c-1.056,0.583-2.331,0.743-3.501,0.463\n"; | |
ptr += "c-1.417-0.323-2.659-1.314-3.3-2.617C0.014,18.26-0.115,17.104,0.1,16.022c0.296-1.443,1.274-2.717,2.58-3.394\n"; | |
ptr += "c0.013-3.44,0-6.881,0.007-10.322C2.674,1.634,2.974,0.955,3.498,0.53z\"/>\n"; | |
ptr += "</svg>\n"; | |
ptr += "</div>\n"; | |
ptr += "<div class=\"side-by-side temperature-text\">Temperature</div>\n"; | |
ptr += "</br>\n"; | |
ptr += "<div class=\"side-by-side temperature\">"; | |
ptr += Temperaturestat; | |
ptr += "<span class=\"superscript\">°C</span></div>\n"; | |
ptr += "</br>\n"; | |
ptr += "<div class=\"side-by-side temperature\">"; | |
ptr += Fahrenheitstat; | |
ptr += "<span class=\"superscript\">°F</span></div>\n"; | |
ptr += "</div>\n"; | |
ptr += "<div class=\"data\">\n"; | |
ptr += "<div class=\"side-by-side humidity-icon\">\n"; | |
ptr += "<svg version=\"1.1\" id=\"Layer_2\" xmlns=\"http://www.w3.org/2000/svg\" xmlns:xlink=\"http://www.w3.org/1999/xlink\" x=\"0px\" y=\"0px\"\n"; | |
ptr += "width=\"12px\" height=\"17.955px\" viewBox=\"0 0 13 17.955\" enable-background=\"new 0 0 13 17.955\" xml:space=\"preserve\">\n"; | |
ptr += "<path fill=\"#FFFFFF\" d=\"M1.819,6.217C3.139,4.064,6.5,0,6.5,0s3.363,4.064,4.681,6.217c1.793,2.926,2.133,5.05,1.571,7.057\n"; | |
ptr += "c-0.438,1.574-2.264,4.681-6.252,4.681c-3.988,0-5.813-3.107-6.252-4.681C-0.313,11.267,0.026,9.143,1.819,6.217\"></path>\n"; | |
ptr += "</svg>\n"; | |
ptr += "</div>\n"; | |
ptr += "<div class=\"side-by-side humidity-text\">Humidity</div>\n"; | |
ptr += "</br>\n"; | |
ptr += "<div class=\"side-by-side humidity\">"; | |
ptr += Humiditystat; | |
ptr += "<span class=\"superscript\">%</span></div>\n"; | |
ptr += "</div>\n"; | |
ptr += "<div class=\"data\">\n"; | |
ptr += "<div class=\"side-by-side temperature-icon\">\n"; | |
ptr += "<svg version=\"1.1\" id=\"Layer_1\" xmlns=\"http://www.w3.org/2000/svg\" xmlns:xlink=\"http://www.w3.org/1999/xlink\" x=\"0px\" y=\"0px\"\n"; | |
ptr += "width=\"9.915px\" height=\"22px\" viewBox=\"0 0 9.915 22\" enable-background=\"new 0 0 9.915 22\" xml:space=\"preserve\">\n"; | |
ptr += "<path fill=\"#FFFFFF\" d=\"M3.498,0.53c0.377-0.331,0.877-0.501,1.374-0.527C5.697-0.04,6.522,0.421,6.924,1.142\n"; | |
ptr += "c0.237,0.399,0.315,0.871,0.311,1.33C7.229,5.856,7.245,9.24,7.227,12.625c1.019,0.539,1.855,1.424,2.301,2.491\n"; | |
ptr += "c0.491,1.163,0.518,2.514,0.062,3.693c-0.414,1.102-1.24,2.038-2.276,2.594c-1.056,0.583-2.331,0.743-3.501,0.463\n"; | |
ptr += "c-1.417-0.323-2.659-1.314-3.3-2.617C0.014,18.26-0.115,17.104,0.1,16.022c0.296-1.443,1.274-2.717,2.58-3.394\n"; | |
ptr += "c0.013-3.44,0-6.881,0.007-10.322C2.674,1.634,2.974,0.955,3.498,0.53z\"/>\n"; | |
ptr += "</svg>\n"; | |
ptr += "</div>\n"; | |
ptr += "<div class=\"side-by-side temperature-text\">Heatindex</div>\n"; | |
ptr += "</br>\n"; | |
ptr += "<div class=\"side-by-side temperature\">"; | |
ptr += HeatindexCstat; | |
ptr += "<span class=\"superscript\">°C</span></div>\n"; | |
ptr += "</br>\n"; | |
ptr += "<div class=\"side-by-side temperature\">"; | |
ptr += HeatindexFstat; | |
ptr += "<span class=\"superscript\">°F</span></div>\n"; | |
ptr += "</div>\n"; | |
ptr += "</div>\n"; | |
ptr += "</body>\n"; | |
ptr += "</html>\n"; | |
return ptr; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
ESP8266 Weather Station
Based on instructions from:
Needed libraries:
Used parts:
NOTE: ESP8266 does not boot, also can't get skatches uploaded, if
D8
is connected.Screenshot:
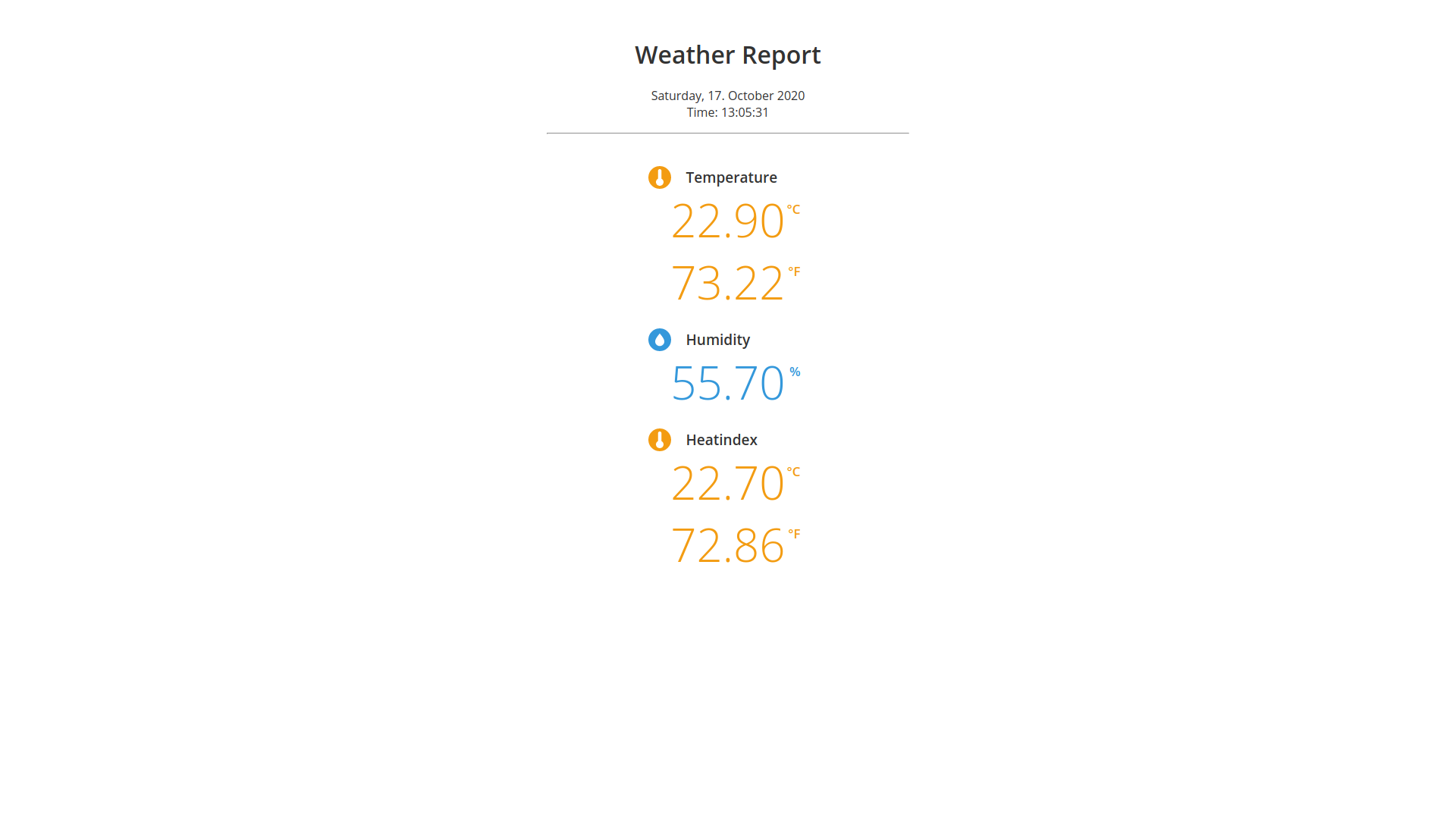
Fritzing:
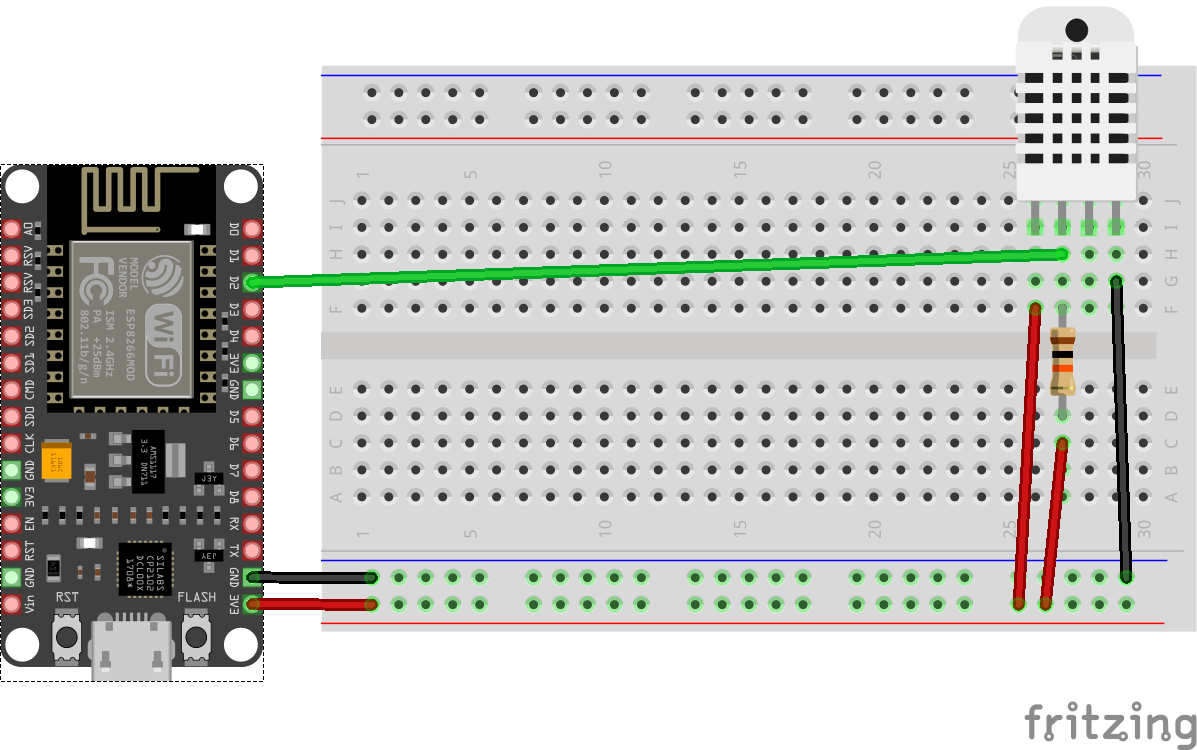