Last active
October 1, 2019 08:32
-
-
Save andrey-legayev/48098af0f4cefe4481c133554f7a3f11 to your computer and use it in GitHub Desktop.
PHP benchmark: in_array() vs. isset() vs. array_key_exists()
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
function test($size, $iterations) | |
{ | |
// prepare arrays | |
$plain = []; | |
$assoc = []; | |
for ($i = 0; $i < $size; $i++) { | |
$value = rand(); | |
array_push($plain, $value); | |
$assoc[$value] = true; | |
} | |
// run tests | |
$t1 = microtime(true); | |
for ($j = 0; $j < $iterations; $j++) { | |
$x = in_array($j, $plain); | |
} | |
$t1 = microtime(true) - $t1; | |
$t2 = microtime(true); | |
for ($j = 0; $j < $iterations; $j++) { | |
$x = isset($assoc[$j]); | |
} | |
$t2 = microtime(true) - $t2; | |
$t3 = microtime(true); | |
for ($j = 0; $j < $iterations; $j++) { | |
$x = array_key_exists($j, $assoc); | |
} | |
$t3 = microtime(true) - $t3; | |
// echo results as CSV | |
printf("%s, %d, %d, %f, %f, %f\n", phpversion(), $size, $iterations, $t1, $t2, $t3); | |
} | |
echo "# PHP Version, Array Size, Iterations, in_array(), isset(), array_key_exists()\n"; | |
for ($i = 1; $i <= 100000; $i = $i * 10) { | |
test($i, 10000); | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/bin/bash | |
for ver in 7.0 7.1 7.2 7.3; do | |
echo "# running tests for version $ver" | |
image=php:$ver-cli | |
docker run --rm -v "$PWD":/src $image \ | |
bash -c "php /src/perf-test.php" | |
done |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Results of benchmark: isset() is fastest function in all mentioned php versions.
Data as a table:
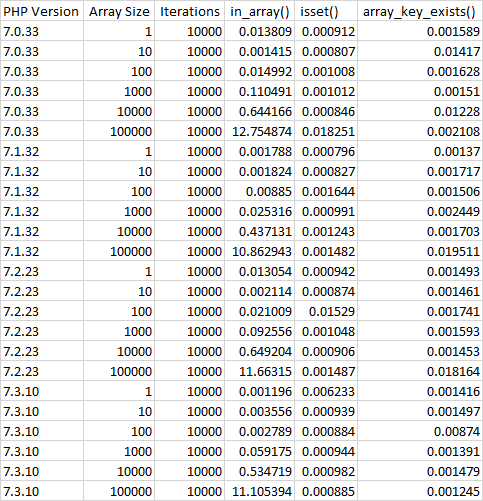
Chart with linear time axis:
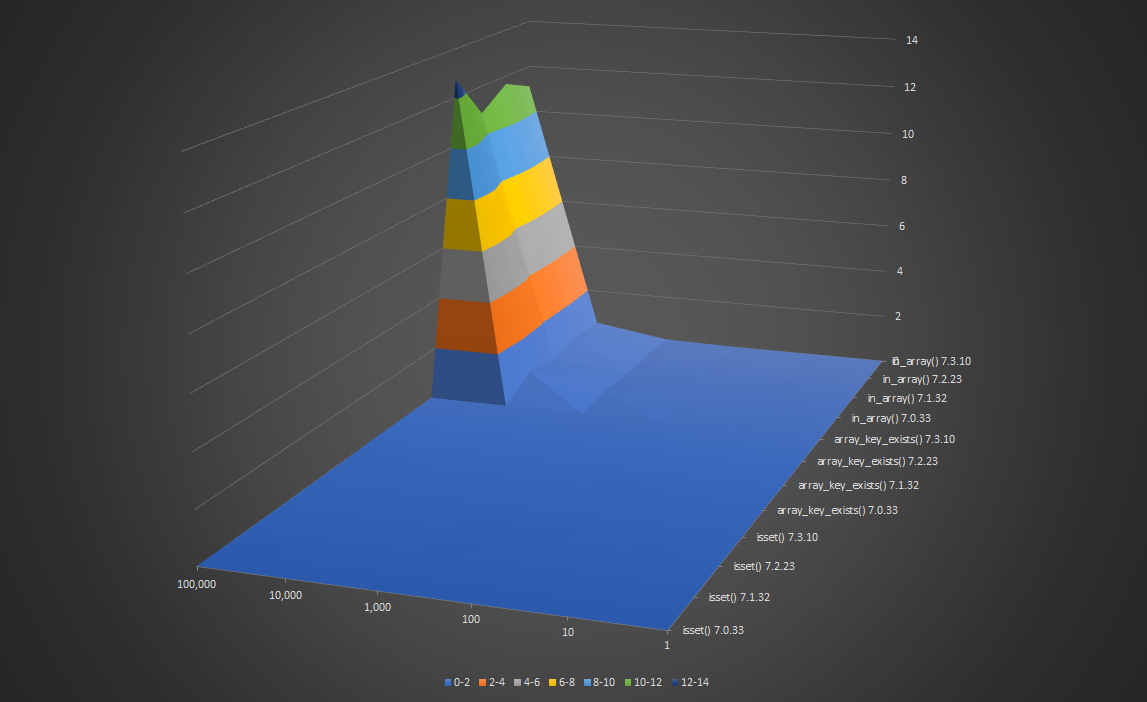
Chart with logarifmic time axis (log base = 2):
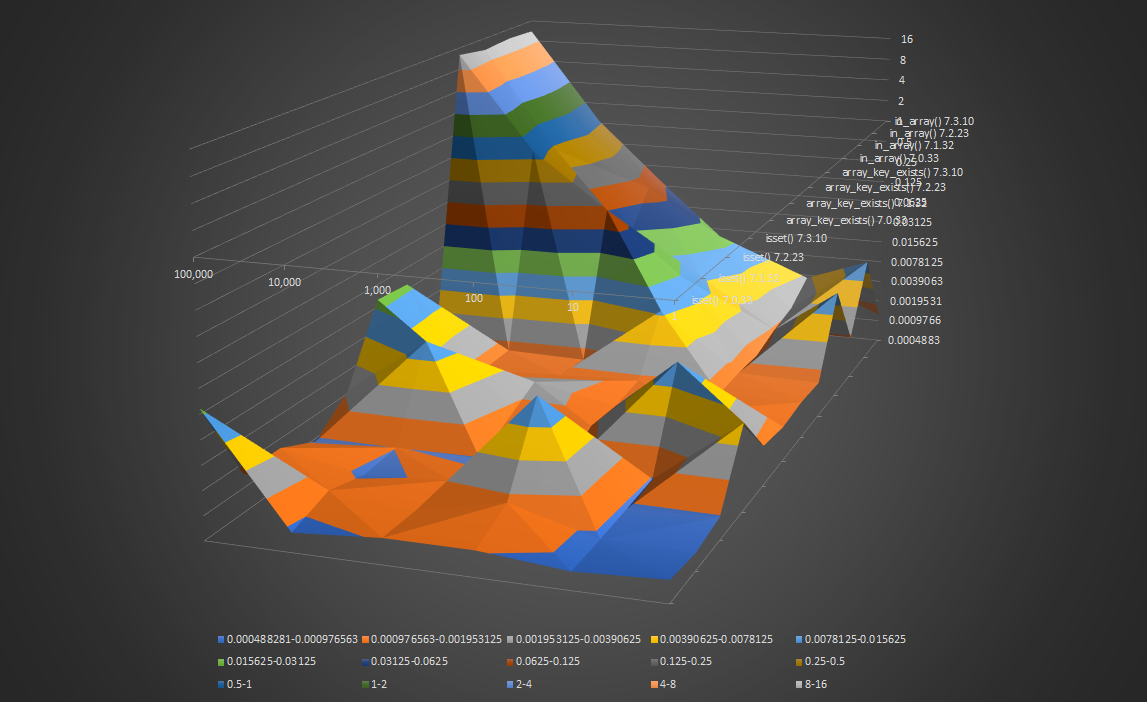