Last active
December 31, 2023 05:11
-
-
Save anilsathyan7/ffb35601483ac46bd72790fde55f5c04 to your computer and use it in GitHub Desktop.
Convert all images in a directory to ".npy" format
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from PIL import Image | |
import os, sys | |
import cv2 | |
import numpy as np | |
''' | |
Converts all images in a directory to '.npy' format. | |
Use np.save and np.load to save and load the images. | |
Use it for training your neural networks in ML/DL projects. | |
''' | |
# Path to image directory | |
path = "/path/to/image/directory/" | |
dirs = os.listdir( path ) | |
dirs.sort() | |
x_train=[] | |
def load_dataset(): | |
# Append images to a list | |
for item in dirs: | |
if os.path.isfile(path+item): | |
im = Image.open(path+item).convert("RGB") | |
im = np.array(im) | |
x_train.append(im) | |
if __name__ == "__main__": | |
load_dataset() | |
# Convert and save the list of images in '.npy' format | |
imgset=np.array(x_train) | |
np.save("imgds.npy",imgset) |
Sorry, that was an unnecessary import(code works without it). Also, the scipy.misc image I/O functionalities seems to be deprecated; use imageio instead.
thanks for posting this code. I need .npy folder which has a shape like this (200, 50, 50, 3). with the help of your code I am only getting the shape of (200). can you please tell me what should I edit in this code?
did you figure it out ? I'm having the same problem
where can I find the folder where the images are converted, please?
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Thanks for posting this code! I'm getting an import error. I was wondering if you have a suggestion for fixing it.
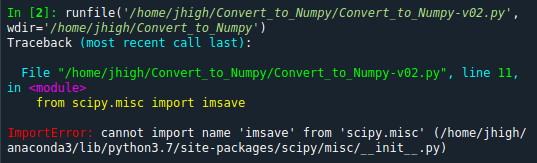
I checked and I have scipy installed in the environment. I opened init.py and this is what I found:
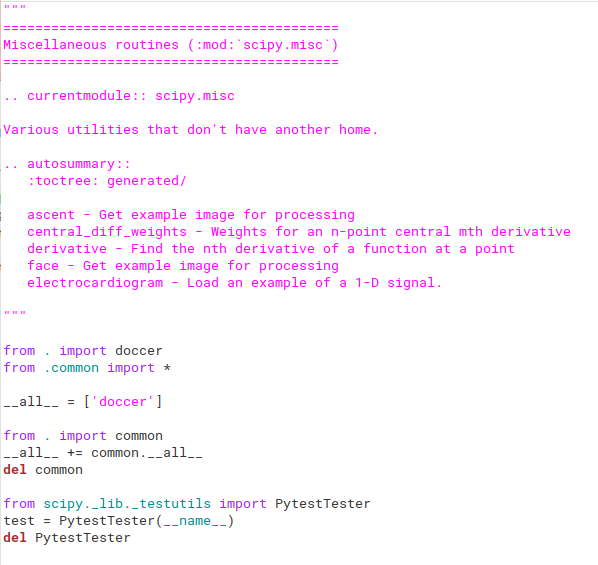
My line 11 matches with your line 3.
Any help would be much appreciated!