Created
July 5, 2021 03:32
-
-
Save anjali-001/e0b01a98829fb322fd6479b1c0160c8d to your computer and use it in GitHub Desktop.
Chai with Mocha: Unit testing in Node.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
//Chai Syntax | |
const expect = require('chai').expect; | |
describe("tests", () => { | |
it("should be true", () => { | |
const res = false; | |
//some code | |
expect(res).to.be.true; //will give a failing test(obviously) | |
}) | |
}) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
//passing and failing tests | |
describe("mocha", () => { | |
it("should have tests that pass", () => { | |
//some code | |
}) | |
it("should have tests that fail", () => { | |
//some code | |
throw new Error('Failed test'); | |
}) | |
}) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
//can use the watch flag like this | |
"scripts": { | |
"test": "mocha --watch" | |
} | |
//or like this | |
"mocha": { | |
"watch":true | |
} | |
//or can make a separate file altogether and write the configurations in that |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
//Mocha Syntax | |
describe("arithmetic operations", () => { | |
describe("add", () => { | |
it("should give sum of two numbers", () => { | |
//some code | |
}) | |
it('should give sum of five numbers',() => { | |
//some code | |
}) | |
}) | |
describe("subtract", () => { | |
it("should give the difference of two numbers", () => { | |
//some code | |
}) | |
}) | |
}) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
module.exports = { | |
getSum: (n1,n2) => { | |
return n1+n2; | |
}, | |
isValidEmail: (email) => { | |
let reg = /^\w*(\-\w)?(\.\w*)?@\w*(-\w*)?\.\w{2,3}(\.\w{2,3})?$/ | |
if (reg.test(email)) | |
return true; | |
else | |
return false; | |
}, | |
getName: (email) => { | |
const res = (email.split("@")[1] || '').split('.'); | |
return res[0]; | |
}, | |
getFactorial: (num) => { | |
let res=1; | |
while(num!=1) | |
{ | |
res*=num; | |
num--; | |
} | |
return res; | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const expect = require('chai').expect; | |
const {getName, getFactorial, isValidEmail, getSum} = require('./testing') | |
describe('testing', () => { | |
describe('getSum', () => { | |
it('should return the sum of two numbers', () => { | |
const n1 = 5, n2 = 6; | |
expect(getSum(n1,n2)).to.eq(11); | |
}) | |
}) | |
describe('isValidEmail', () => { | |
it('should be true if given a valid email',() => { | |
const email = "anjali@mochajs.org"; | |
expect(isValidEmail(email)).to.be.true; | |
}) | |
}) | |
describe('getName', () => { | |
it('should give the name on receiving an email id', () => { | |
const email = "anjali@mochajs.org"; | |
expect(getName(email)).to.eq('mochajs') | |
}) | |
}) | |
describe('getFactorial', () => { | |
it('should give factorial of the number provided', () => { | |
const num = 3; | |
expect(getFactorial(num)).to.eq(6) | |
}) | |
}) | |
}) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Passing and Failing tests
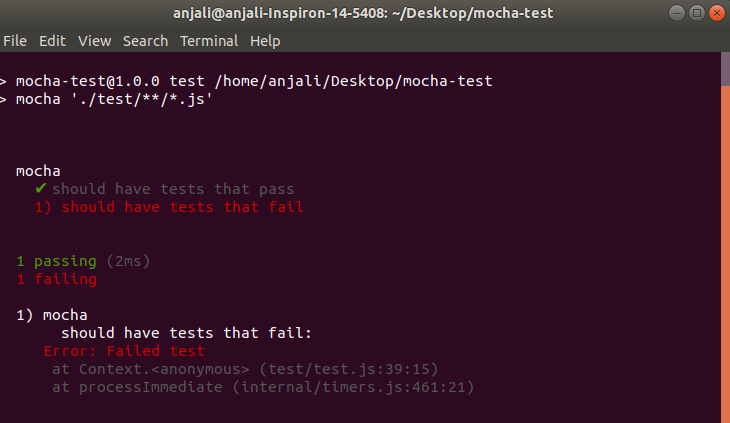
Testing.spec.js result:
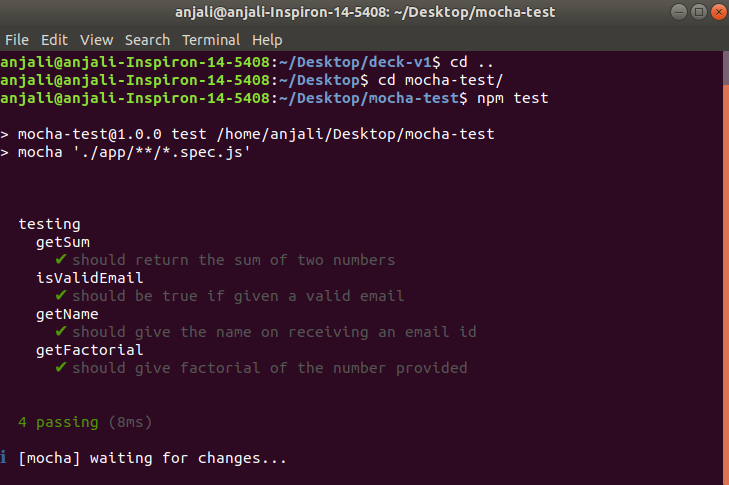