Last active
March 8, 2016 07:03
-
-
Save arkist/3b674ca5723787c7000f to your computer and use it in GitHub Desktop.
git diff 1c14477..HEAD --name-only | grep docs/docs | grep '[^(JP|IT|CN)].md$' | xargs git diff 1c14477..HEAD --
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
diff --git a/docs/docs/02.1-jsx-in-depth.md b/docs/docs/02.1-jsx-in-depth.md | |
index c395f36..8397e50 100644 | |
--- a/docs/docs/02.1-jsx-in-depth.md | |
+++ b/docs/docs/02.1-jsx-in-depth.md | |
@@ -82,10 +82,7 @@ var Nav = React.createClass({ }); | |
var Nav = React.createClass({displayName: "Nav", }); | |
``` | |
-Use the [Babel REPL](https://babeljs.io/repl/) to try out JSX and see how it | |
-desugars into native JavaScript, and the | |
-[HTML to JSX converter](/react/html-jsx.html) to convert your existing HTML to | |
-JSX. | |
+Use the [Babel REPL](https://babeljs.io/repl/) to try out JSX and see how it desugars into native JavaScript, and the [HTML to JSX converter](/react/html-jsx.html) to convert your existing HTML to JSX. | |
If you want to use JSX, the [Getting Started](/react/docs/getting-started.html) guide shows how to set up compilation. | |
@@ -163,8 +160,7 @@ var App = ( | |
### Attribute Expressions | |
-To use a JavaScript expression as an attribute value, wrap the expression in a | |
-pair of curly braces (`{}`) instead of quotes (`""`). | |
+To use a JavaScript expression as an attribute value, wrap the expression in a pair of curly braces (`{}`) instead of quotes (`""`). | |
```javascript | |
// Input (JSX): | |
diff --git a/docs/docs/02.2-jsx-spread.md b/docs/docs/02.2-jsx-spread.md | |
index 4cafa8c..a4b2678 100644 | |
--- a/docs/docs/02.2-jsx-spread.md | |
+++ b/docs/docs/02.2-jsx-spread.md | |
@@ -49,4 +49,4 @@ You can use this multiple times or combine it with other attributes. The specifi | |
## What's with the weird `...` notation? | |
-The `...` operator (or spread operator) is already supported for [arrays in ES6](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_operator). There is also an ES7 proposal for [Object Rest and Spread Properties](https://github.com/sebmarkbage/ecmascript-rest-spread). We're taking advantage of these supported and developing standards in order to provide a cleaner syntax in JSX. | |
+The `...` operator (or spread operator) is already supported for [arrays in ES6](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_operator). There is also an ECMAScript proposal for [Object Rest and Spread Properties](https://github.com/sebmarkbage/ecmascript-rest-spread). We're taking advantage of these supported and developing standards in order to provide a cleaner syntax in JSX. | |
diff --git a/docs/docs/02.3-jsx-gotchas.md b/docs/docs/02.3-jsx-gotchas.md | |
index 6ab5d4a..10ae6bc 100644 | |
--- a/docs/docs/02.3-jsx-gotchas.md | |
+++ b/docs/docs/02.3-jsx-gotchas.md | |
@@ -61,6 +61,12 @@ If you pass properties to native HTML elements that do not exist in the HTML spe | |
<div data-custom-attribute="foo" /> | |
``` | |
+However, arbitrary attributes are supported on custom elements (those with a hyphen in the tag name or an `is="..."` attribute). | |
+ | |
+```javascript | |
+<x-my-component custom-attribute="foo" /> | |
+``` | |
+ | |
[Web Accessibility](http://www.w3.org/WAI/intro/aria) attributes starting with `aria-` will be rendered properly. | |
```javascript | |
diff --git a/docs/docs/03-interactivity-and-dynamic-uis.md b/docs/docs/03-interactivity-and-dynamic-uis.md | |
index dc4a19c..80ec0c8 100644 | |
--- a/docs/docs/03-interactivity-and-dynamic-uis.md | |
+++ b/docs/docs/03-interactivity-and-dynamic-uis.md | |
@@ -36,7 +36,7 @@ ReactDOM.render( | |
## Event Handling and Synthetic Events | |
-With React you simply pass your event handler as a camelCased prop similar to how you'd do it in normal HTML. React ensures that all events behave identically in IE8 and above by implementing a synthetic event system. That is, React knows how to bubble and capture events according to the spec, and the events passed to your event handler are guaranteed to be consistent with [the W3C spec](http://www.w3.org/TR/DOM-Level-3-Events/), regardless of which browser you're using. | |
+With React you simply pass your event handler as a camelCased prop similar to how you'd do it in normal HTML. React ensures that all events behave similarly in all browsers by implementing a synthetic event system. That is, React knows how to bubble and capture events according to the spec, and the events passed to your event handler are guaranteed to be consistent with [the W3C spec](http://www.w3.org/TR/DOM-Level-3-Events/), regardless of which browser you're using. | |
## Under the Hood: Autobinding and Event Delegation | |
diff --git a/docs/docs/04-multiple-components.md b/docs/docs/04-multiple-components.md | |
index f6fa1de..0800974 100644 | |
--- a/docs/docs/04-multiple-components.md | |
+++ b/docs/docs/04-multiple-components.md | |
@@ -14,49 +14,49 @@ By building modular components that reuse other components with well-defined int | |
## Composition Example | |
-Let's create a simple Avatar component which shows a profile picture and username using the Facebook Graph API. | |
+Let's create a simple Avatar component which shows a Facebook page picture and name using the Facebook Graph API. | |
```javascript | |
var Avatar = React.createClass({ | |
render: function() { | |
return ( | |
<div> | |
- <ProfilePic username={this.props.username} /> | |
- <ProfileLink username={this.props.username} /> | |
+ <PagePic pagename={this.props.pagename} /> | |
+ <PageLink pagename={this.props.pagename} /> | |
</div> | |
); | |
} | |
}); | |
-var ProfilePic = React.createClass({ | |
+var PagePic = React.createClass({ | |
render: function() { | |
return ( | |
- <img src={'https://graph.facebook.com/' + this.props.username + '/picture'} /> | |
+ <img src={'https://graph.facebook.com/' + this.props.pagename + '/picture'} /> | |
); | |
} | |
}); | |
-var ProfileLink = React.createClass({ | |
+var PageLink = React.createClass({ | |
render: function() { | |
return ( | |
- <a href={'https://www.facebook.com/' + this.props.username}> | |
- {this.props.username} | |
+ <a href={'https://www.facebook.com/' + this.props.pagename}> | |
+ {this.props.pagename} | |
</a> | |
); | |
} | |
}); | |
ReactDOM.render( | |
- <Avatar username="pwh" />, | |
+ <Avatar pagename="Engineering" />, | |
document.getElementById('example') | |
); | |
``` | |
## Ownership | |
-In the above example, instances of `Avatar` *own* instances of `ProfilePic` and `ProfileLink`. In React, **an owner is the component that sets the `props` of other components**. More formally, if a component `X` is created in component `Y`'s `render()` method, it is said that `X` is *owned by* `Y`. As discussed earlier, a component cannot mutate its `props` — they are always consistent with what its owner sets them to. This fundamental invariant leads to UIs that are guaranteed to be consistent. | |
+In the above example, instances of `Avatar` *own* instances of `PagePic` and `PageLink`. In React, **an owner is the component that sets the `props` of other components**. More formally, if a component `X` is created in component `Y`'s `render()` method, it is said that `X` is *owned by* `Y`. As discussed earlier, a component cannot mutate its `props` — they are always consistent with what its owner sets them to. This fundamental invariant leads to UIs that are guaranteed to be consistent. | |
-It's important to draw a distinction between the owner-ownee relationship and the parent-child relationship. The owner-ownee relationship is specific to React, while the parent-child relationship is simply the one you know and love from the DOM. In the example above, `Avatar` owns the `div`, `ProfilePic` and `ProfileLink` instances, and `div` is the **parent** (but not owner) of the `ProfilePic` and `ProfileLink` instances. | |
+It's important to draw a distinction between the owner-ownee relationship and the parent-child relationship. The owner-ownee relationship is specific to React, while the parent-child relationship is simply the one you know and love from the DOM. In the example above, `Avatar` owns the `div`, `PagePic` and `PageLink` instances, and `div` is the **parent** (but not owner) of the `PagePic` and `PageLink` instances. | |
## Children | |
diff --git a/docs/docs/05-reusable-components.ko-KR.md b/docs/docs/05-reusable-components.ko-KR.md | |
index f7633d5..c89e749 100644 | |
--- a/docs/docs/05-reusable-components.ko-KR.md | |
+++ b/docs/docs/05-reusable-components.ko-KR.md | |
@@ -244,7 +244,7 @@ ReactDOM.render(<HelloMessage name="Sebastian" />, mountNode); | |
아니면 ES6의 화살표 문법을 사용할 수 있습니다. | |
```javascript | |
-var HelloMessage = (props) => <div>Hello {props.name}</div>; | |
+const HelloMessage = (props) => <div>Hello {props.name}</div>; | |
ReactDOM.render(<HelloMessage name="Sebastian" />, mountNode); | |
``` | |
diff --git a/docs/docs/05-reusable-components.md b/docs/docs/05-reusable-components.md | |
index 75e2385..b79e329 100644 | |
--- a/docs/docs/05-reusable-components.md | |
+++ b/docs/docs/05-reusable-components.md | |
@@ -78,6 +78,27 @@ React.createClass({ | |
}); | |
``` | |
+### Single Child | |
+ | |
+With `React.PropTypes.element` you can specify that only a single child can be passed to a component as children. | |
+ | |
+```javascript | |
+var MyComponent = React.createClass({ | |
+ propTypes: { | |
+ children: React.PropTypes.element.isRequired | |
+ }, | |
+ | |
+ render: function() { | |
+ return ( | |
+ <div> | |
+ {this.props.children} // This must be exactly one element or it will warn. | |
+ </div> | |
+ ); | |
+ } | |
+ | |
+}); | |
+``` | |
+ | |
## Default Prop Values | |
React lets you define default values for your `props` in a very declarative way: | |
@@ -115,28 +136,6 @@ ReactDOM.render( | |
); | |
``` | |
-## Single Child | |
- | |
-With `React.PropTypes.element` you can specify that only a single child can be passed to | |
-a component as children. | |
- | |
-```javascript | |
-var MyComponent = React.createClass({ | |
- propTypes: { | |
- children: React.PropTypes.element.isRequired | |
- }, | |
- | |
- render: function() { | |
- return ( | |
- <div> | |
- {this.props.children} // This must be exactly one element or it will throw. | |
- </div> | |
- ); | |
- } | |
- | |
-}); | |
-``` | |
- | |
## Mixins | |
Components are the best way to reuse code in React, but sometimes very different components may share some common functionality. These are sometimes called [cross-cutting concerns](https://en.wikipedia.org/wiki/Cross-cutting_concern). React provides `mixins` to solve this problem. | |
@@ -244,14 +243,15 @@ ReactDOM.render(<HelloMessage name="Sebastian" />, mountNode); | |
Or using the new ES6 arrow syntax: | |
```javascript | |
-var HelloMessage = (props) => <div>Hello {props.name}</div>; | |
+const HelloMessage = (props) => <div>Hello {props.name}</div>; | |
ReactDOM.render(<HelloMessage name="Sebastian" />, mountNode); | |
``` | |
This simplified component API is intended for components that are pure functions of their props. These components must not retain internal state, do not have backing instances, and do not have the component lifecycle methods. They are pure functional transforms of their input, with zero boilerplate. | |
+However, you may still specify `.propTypes` and `.defaultProps` by setting them as properties on the function, just as you would set them on an ES6 class. | |
> NOTE: | |
> | |
> Because stateless functions don't have a backing instance, you can't attach a ref to a stateless function component. Normally this isn't an issue, since stateless functions do not provide an imperative API. Without an imperative API, there isn't much you could do with an instance anyway. However, if a user wants to find the DOM node of a stateless function component, they must wrap the component in a stateful component (eg. ES6 class component) and attach the ref to the stateful wrapper component. | |
-In an ideal world, most of your components would be stateless functions because these stateless components can follow a faster code path within the React core. This is the recommended pattern, when possible. | |
+In an ideal world, most of your components would be stateless functions because in the future we’ll also be able to make performance optimizations specific to these components by avoiding unnecessary checks and memory allocations. This is the recommended pattern, when possible. | |
diff --git a/docs/docs/06-transferring-props.ko-KR.md b/docs/docs/06-transferring-props.ko-KR.md | |
index 02aa86c..1f4b294 100644 | |
--- a/docs/docs/06-transferring-props.ko-KR.md | |
+++ b/docs/docs/06-transferring-props.ko-KR.md | |
@@ -27,16 +27,14 @@ React.createElement(Component, Object.assign({}, this.props, { more: 'values' }) | |
대부분의 경우 명시적으로 프로퍼티를 아래로 전달해야 합니다. 이는 동작을 확신하는 내부 API의 일부만 공개하도록 합니다. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var fancyClass = this.props.checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- return ( | |
- <div className={fancyClass} onClick={this.props.onClick}> | |
- {this.props.children} | |
- </div> | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var fancyClass = props.checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ return ( | |
+ <div className={fancyClass} onClick={props.onClick}> | |
+ {props.children} | |
+ </div> | |
+ ); | |
+} | |
ReactDOM.render( | |
<FancyCheckbox checked={true} onClick={console.log.bind(console)}> | |
세상아 안녕! | |
@@ -59,22 +57,20 @@ ReactDOM.render( | |
소비할 프로퍼티들을 나열하고, 그 뒤에 `...other`를 넣습니다. | |
```javascript | |
-var { checked, ...other } = this.props; | |
+var { checked, ...other } = props; | |
``` | |
이는 지금 소비한 props를 *제외한* 나머지를 아래로 전달합니다. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var { checked, ...other } = this.props; | |
- var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- // `other`에는 { onClick: console.log }가 포함되지만 checked 프로퍼티는 제외됩니다 | |
- return ( | |
- <div {...other} className={fancyClass} /> | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var { checked, ...other } = props; | |
+ var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ // `other`에는 { onClick: console.log }가 포함되지만 checked 프로퍼티는 제외됩니다 | |
+ return ( | |
+ <div {...other} className={fancyClass} /> | |
+ ); | |
+} | |
ReactDOM.render( | |
<FancyCheckbox checked={true} onClick={console.log.bind(console)}> | |
세상아 안녕! | |
@@ -90,15 +86,13 @@ ReactDOM.render( | |
미상의 `other` props을 전달할 때는 항상 구조 해체 패턴을 사용하세요. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var fancyClass = this.props.checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- // 반례: `checked` 또한 내부 컴포넌트로 전달될 것입니다 | |
- return ( | |
- <div {...this.props} className={fancyClass} /> | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var fancyClass = props.checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ // 반례: `checked` 또한 내부 컴포넌트로 전달될 것입니다 | |
+ return ( | |
+ <div {...props} className={fancyClass} /> | |
+ ); | |
+} | |
``` | |
## 같은 Prop을 소비하고 전달하기 | |
@@ -106,23 +100,21 @@ var FancyCheckbox = React.createClass({ | |
컴포넌트가 프로퍼티를 사용하지만 계속 넘기길 원한다면, `checked={checked}`처럼 명시적으로 다시 넘길 수 있습니다. 리팩토링과 린트(lint)하기가 더 쉬우므로 이 방식이 `this.props` 객체 전부를 넘기는 것보다 낫습니다. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var { checked, title, ...other } = this.props; | |
- var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- var fancyTitle = checked ? 'X ' + title : 'O ' + title; | |
- return ( | |
- <label> | |
- <input {...other} | |
- checked={checked} | |
- className={fancyClass} | |
- type="checkbox" | |
- /> | |
- {fancyTitle} | |
- </label> | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var { checked, title, ...other } = props; | |
+ var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ var fancyTitle = checked ? 'X ' + title : 'O ' + title; | |
+ return ( | |
+ <label> | |
+ <input {...other} | |
+ checked={checked} | |
+ className={fancyClass} | |
+ type="checkbox" | |
+ /> | |
+ {fancyTitle} | |
+ </label> | |
+ ); | |
+} | |
``` | |
> 주의: | |
@@ -152,14 +144,12 @@ z; // { a: 3, b: 4 } | |
JSX를 사용하지 않는다면 라이브러리를 사용해 같은 패턴을 쓸 수 있습니다. Underscore에서는 `_.omit`을 사용해 특정 프로퍼티를 제외하거나 `_.extend`를 사용해 새로운 객체로 프로퍼티를 복사할 수 있습니다. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var checked = this.props.checked; | |
- var other = _.omit(this.props, 'checked'); | |
- var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- return ( | |
- React.DOM.div(_.extend({}, other, { className: fancyClass })) | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var checked = props.checked; | |
+ var other = _.omit(props, 'checked'); | |
+ var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ return ( | |
+ React.DOM.div(_.extend({}, other, { className: fancyClass })) | |
+ ); | |
+} | |
``` | |
diff --git a/docs/docs/06-transferring-props.md b/docs/docs/06-transferring-props.md | |
index 0bac12a..6b2280e 100644 | |
--- a/docs/docs/06-transferring-props.md | |
+++ b/docs/docs/06-transferring-props.md | |
@@ -20,23 +20,21 @@ If you don't use JSX, you can use any object helper such as ES6 `Object.assign` | |
React.createElement(Component, Object.assign({}, this.props, { more: 'values' })); | |
``` | |
-The rest of this tutorial explains best practices. It uses JSX and experimental ES7 syntax. | |
+The rest of this tutorial explains best practices. It uses JSX and experimental ECMAScript syntax. | |
## Manual Transfer | |
Most of the time you should explicitly pass the properties down. This ensures that you only expose a subset of the inner API, one that you know will work. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var fancyClass = this.props.checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- return ( | |
- <div className={fancyClass} onClick={this.props.onClick}> | |
- {this.props.children} | |
- </div> | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var fancyClass = props.checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ return ( | |
+ <div className={fancyClass} onClick={props.onClick}> | |
+ {props.children} | |
+ </div> | |
+ ); | |
+} | |
ReactDOM.render( | |
<FancyCheckbox checked={true} onClick={console.log.bind(console)}> | |
Hello world! | |
@@ -58,22 +56,20 @@ Sometimes it's fragile and tedious to pass every property along. In that case yo | |
List out all the properties that you would like to consume, followed by `...other`. | |
```javascript | |
-var { checked, ...other } = this.props; | |
+var { checked, ...other } = props; | |
``` | |
This ensures that you pass down all the props EXCEPT the ones you're consuming yourself. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var { checked, ...other } = this.props; | |
- var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- // `other` contains { onClick: console.log } but not the checked property | |
- return ( | |
- <div {...other} className={fancyClass} /> | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var { checked, ...other } = props; | |
+ var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ // `other` contains { onClick: console.log } but not the checked property | |
+ return ( | |
+ <div {...other} className={fancyClass} /> | |
+ ); | |
+} | |
ReactDOM.render( | |
<FancyCheckbox checked={true} onClick={console.log.bind(console)}> | |
Hello world! | |
@@ -89,39 +85,35 @@ ReactDOM.render( | |
Always use the destructuring pattern when transferring unknown `other` props. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var fancyClass = this.props.checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- // ANTI-PATTERN: `checked` would be passed down to the inner component | |
- return ( | |
- <div {...this.props} className={fancyClass} /> | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var fancyClass = props.checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ // ANTI-PATTERN: `checked` would be passed down to the inner component | |
+ return ( | |
+ <div {...props} className={fancyClass} /> | |
+ ); | |
+} | |
``` | |
## Consuming and Transferring the Same Prop | |
-If your component wants to consume a property but also wants to pass it along, you can repass it explicitly with `checked={checked}`. This is preferable to passing the full `this.props` object since it's easier to refactor and lint. | |
+If your component wants to consume a property but also wants to pass it along, you can repass it explicitly with `checked={checked}`. This is preferable to passing the full `props` object since it's easier to refactor and lint. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var { checked, title, ...other } = this.props; | |
- var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- var fancyTitle = checked ? 'X ' + title : 'O ' + title; | |
- return ( | |
- <label> | |
- <input {...other} | |
- checked={checked} | |
- className={fancyClass} | |
- type="checkbox" | |
- /> | |
- {fancyTitle} | |
- </label> | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var { checked, title, ...other } = props; | |
+ var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ var fancyTitle = checked ? 'X ' + title : 'O ' + title; | |
+ return ( | |
+ <label> | |
+ <input {...other} | |
+ checked={checked} | |
+ className={fancyClass} | |
+ type="checkbox" | |
+ /> | |
+ {fancyTitle} | |
+ </label> | |
+ ); | |
+} | |
``` | |
> NOTE: | |
@@ -132,7 +124,7 @@ var FancyCheckbox = React.createClass({ | |
Rest properties allow you to extract the remaining properties from an object into a new object. It excludes every other property listed in the destructuring pattern. | |
-This is an experimental implementation of an [ES7 proposal](https://github.com/sebmarkbage/ecmascript-rest-spread). | |
+This is an experimental implementation of an [ECMAScript proposal](https://github.com/sebmarkbage/ecmascript-rest-spread). | |
```javascript | |
var { x, y, ...z } = { x: 1, y: 2, a: 3, b: 4 }; | |
@@ -143,21 +135,19 @@ z; // { a: 3, b: 4 } | |
> Note: | |
> | |
-> This proposal has reached stage 2 and is now enabled by default in Babel. Older versions of Babel may need to explicitly enable this transform with `babel --optional es7.objectRestSpread` | |
+> To transform rest and spread properties using Babel 6, you need to install the [`es2015`](https://babeljs.io/docs/plugins/preset-es2015/) preset, the [`transform-object-rest-spread`](https://babeljs.io/docs/plugins/transform-object-rest-spread/) plugin and configure them in the `.babelrc` file. | |
## Transferring with Underscore | |
If you don't use JSX, you can use a library to achieve the same pattern. Underscore supports `_.omit` to filter out properties and `_.extend` to copy properties onto a new object. | |
```javascript | |
-var FancyCheckbox = React.createClass({ | |
- render: function() { | |
- var checked = this.props.checked; | |
- var other = _.omit(this.props, 'checked'); | |
- var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
- return ( | |
- React.DOM.div(_.extend({}, other, { className: fancyClass })) | |
- ); | |
- } | |
-}); | |
+function FancyCheckbox(props) { | |
+ var checked = props.checked; | |
+ var other = _.omit(props, 'checked'); | |
+ var fancyClass = checked ? 'FancyChecked' : 'FancyUnchecked'; | |
+ return ( | |
+ React.DOM.div(_.extend({}, other, { className: fancyClass })) | |
+ ); | |
+} | |
``` | |
diff --git a/docs/docs/07-forms.md b/docs/docs/07-forms.md | |
index af75d4b..2da8e35 100644 | |
--- a/docs/docs/07-forms.md | |
+++ b/docs/docs/07-forms.md | |
@@ -34,7 +34,7 @@ Like all DOM events, the `onChange` prop is supported on all native components a | |
## Controlled Components | |
-An `<input>` with `value` set is a *controlled* component. In a controlled `<input>`, the value of the rendered element will always reflect the `value` prop. For example: | |
+A **controlled** `<input>` has a `value` prop. Rendering a controlled `<input>` will reflect the value of the `value` prop. | |
```javascript | |
render: function() { | |
@@ -42,7 +42,7 @@ An `<input>` with `value` set is a *controlled* component. In a controlled `<inp | |
} | |
``` | |
-This will render an input that always has a value of `Hello!`. Any user input will have no effect on the rendered element because React has declared the value to be `Hello!`. If you wanted to update the value in response to user input, you could use the `onChange` event: | |
+User input will have no effect on the rendered element because React has declared the value to be `Hello!`. To update the value in response to user input, you could use the `onChange` event: | |
```javascript | |
getInitialState: function() { | |
@@ -52,12 +52,17 @@ This will render an input that always has a value of `Hello!`. Any user input wi | |
this.setState({value: event.target.value}); | |
}, | |
render: function() { | |
- var value = this.state.value; | |
- return <input type="text" value={value} onChange={this.handleChange} />; | |
+ return ( | |
+ <input | |
+ type="text" | |
+ value={this.state.value} | |
+ onChange={this.handleChange} | |
+ /> | |
+ ); | |
} | |
``` | |
-In this example, we are simply accepting the newest value provided by the user and updating the `value` prop of the `<input>` component. This pattern makes it easy to implement interfaces that respond to or validate user interactions. For example: | |
+In this example, we are accepting the value provided by the user and updating the `value` prop of the `<input>` component. This pattern makes it easy to implement interfaces that respond to or validate user interactions. For example: | |
```javascript | |
handleChange: function(event) { | |
@@ -65,7 +70,9 @@ In this example, we are simply accepting the newest value provided by the user a | |
} | |
``` | |
-This would accept user input but truncate the value to the first 140 characters. | |
+This would accept user input and truncate the value to the first 140 characters. | |
+ | |
+A **Controlled** component does not maintain its own internal state; the component renders purely based on props. | |
### Potential Issues With Checkboxes and Radio Buttons | |
@@ -73,7 +80,7 @@ Be aware that, in an attempt to normalize change handling for checkbox and radio | |
## Uncontrolled Components | |
-An `<input>` that does not supply a `value` (or sets it to `null`) is an *uncontrolled* component. In an uncontrolled `<input>`, the value of the rendered element will reflect the user's input. For example: | |
+An `<input>` without a `value` property is an *uncontrolled* component: | |
```javascript | |
render: function() { | |
@@ -83,6 +90,8 @@ An `<input>` that does not supply a `value` (or sets it to `null`) is an *uncont | |
This will render an input that starts off with an empty value. Any user input will be immediately reflected by the rendered element. If you wanted to listen to updates to the value, you could use the `onChange` event just like you can with controlled components. | |
+An **uncontrolled** component maintains its own internal state. | |
+ | |
### Default Value | |
If you want to initialize the component with a non-empty value, you can supply a `defaultValue` prop. For example: | |
@@ -95,7 +104,7 @@ If you want to initialize the component with a non-empty value, you can supply a | |
This example will function much like the **Uncontrolled Components** example above. | |
-Likewise, `<input>` supports `defaultChecked` and `<select>` supports `defaultValue`. | |
+Likewise, `<input type="checkbox">` and `<input type="radio">` support `defaultChecked`, and `<select>` supports `defaultValue`. | |
> Note: | |
> | |
diff --git a/docs/docs/08-working-with-the-browser.md b/docs/docs/08-working-with-the-browser.md | |
index 1a7548f..645f668 100644 | |
--- a/docs/docs/08-working-with-the-browser.md | |
+++ b/docs/docs/08-working-with-the-browser.md | |
@@ -12,7 +12,7 @@ React provides powerful abstractions that free you from touching the DOM directl | |
React is very fast because it never talks to the DOM directly. React maintains a fast in-memory representation of the DOM. `render()` methods actually return a *description* of the DOM, and React can compare this description with the in-memory representation to compute the fastest way to update the browser. | |
-Additionally, React implements a full synthetic event system such that all event objects are guaranteed to conform to the W3C spec despite browser quirks, and everything bubbles consistently and efficiently across browsers. You can even use some HTML5 events in IE8! | |
+Additionally, React implements a full synthetic event system such that all event objects are guaranteed to conform to the W3C spec despite browser quirks, and everything bubbles consistently and efficiently across browsers. You can even use some HTML5 events in older browsers that don't ordinarily support them! | |
Most of the time you should stay within React's "faked browser" world since it's more performant and easier to reason about. However, sometimes you simply need to access the underlying API, perhaps to work with a third-party library like a jQuery plugin. React provides escape hatches for you to use the underlying DOM API directly. | |
@@ -53,45 +53,8 @@ _Mounted_ composite components also support the following method: | |
* `component.forceUpdate()` can be invoked on any mounted component when you know that some deeper aspect of the component's state has changed without using `this.setState()`. | |
-## Browser Support and Polyfills | |
+## Browser Support | |
-At Facebook, we support older browsers, including IE8. We've had polyfills in place for a long time to allow us to write forward-thinking JS. This means we don't have a bunch of hacks scattered throughout our codebase and we can still expect our code to "just work". For example, instead of seeing `+new Date()`, we can just write `Date.now()`. Since the open source React is the same as what we use internally, we've carried over this philosophy of using forward thinking JS. | |
+React supports most popular browsers, including Internet Explorer 9 and above. | |
-In addition to that philosophy, we've also taken the stance that we, as authors of a JS library, should not be shipping polyfills as a part of our library. If every library did this, there's a good chance you'd be sending down the same polyfill multiple times, which could be a sizable chunk of dead code. If your product needs to support older browsers, chances are you're already using something like [es5-shim](https://github.com/es-shims/es5-shim). | |
- | |
-### Polyfills Needed to Support Older Browsers | |
- | |
-`es5-shim.js` from [kriskowal's es5-shim](https://github.com/es-shims/es5-shim) provides the following that React needs: | |
- | |
-* `Array.isArray` | |
-* `Array.prototype.every` | |
-* `Array.prototype.forEach` | |
-* `Array.prototype.indexOf` | |
-* `Array.prototype.map` | |
-* `Date.now` | |
-* `Function.prototype.bind` | |
-* `Object.keys` | |
-* `String.prototype.split` | |
-* `String.prototype.trim` | |
- | |
-`es5-sham.js`, also from [kriskowal's es5-shim](https://github.com/es-shims/es5-shim), provides the following that React needs: | |
- | |
-* `Object.create` | |
-* `Object.freeze` | |
- | |
-The unminified build of React needs the following from [paulmillr's console-polyfill](https://github.com/paulmillr/console-polyfill). | |
- | |
-* `console.*` | |
- | |
-When using HTML5 elements in IE8 including `<section>`, `<article>`, `<nav>`, `<header>`, and `<footer>`, it's also necessary to include [html5shiv](https://github.com/aFarkas/html5shiv) or a similar script. | |
- | |
-### Cross-browser Issues | |
- | |
-Although React is pretty good at abstracting browser differences, some browsers are limited or present quirky behaviors that we couldn't find a workaround for. | |
- | |
-#### onScroll event on IE8 | |
- | |
-On IE8 the `onScroll` event doesn't bubble and IE8 doesn't have an API to define handlers to the capturing phase of an event, meaning there is no way for React to listen to these events. | |
-Currently a handler to this event is ignored on IE8. | |
- | |
-See the [onScroll doesn't work in IE8](https://github.com/facebook/react/issues/631) GitHub issue for more information. | |
+(We don't support older browsers that don't support ES5 methods, but you may find that your apps do work in older browsers if polyfills such as [es5-shim and es5-sham](https://github.com/es-shims/es5-shim) are included in the page. You're on your own if you choose to take this path.) | |
diff --git a/docs/docs/08.1-more-about-refs.md b/docs/docs/08.1-more-about-refs.md | |
index 9caf893..5bca86e 100644 | |
--- a/docs/docs/08.1-more-about-refs.md | |
+++ b/docs/docs/08.1-more-about-refs.md | |
@@ -5,7 +5,7 @@ permalink: more-about-refs.html | |
prev: working-with-the-browser.html | |
next: tooling-integration.html | |
--- | |
-After building your component, you may find yourself wanting to "reach out" and invoke methods on component instances returned from `render()`. In most cases, this should be unnecessary because the reactive data flow always ensures that the most recent props are sent to each child that is output from render(). However, there are a few cases where it still might be necessary or beneficial, so React provides an escape hatch known as `refs`. These `refs` (references) are especially useful when you need to: find the DOM markup rendered by a component (for instance, to position it absolutely), use React components in a larger non-React application, or transition your existing codebase to React. | |
+After building your component, you may find yourself wanting to "reach out" and invoke methods on component instances returned from `render()`. In most cases, this should be unnecessary because the reactive data flow always ensures that the most recent props are sent to each child that is output from `render()`. However, there are a few cases where it still might be necessary or beneficial, so React provides an escape hatch known as `refs`. These `refs` (references) are especially useful when you need to: find the DOM markup rendered by a component (for instance, to position it absolutely), use React components in a larger non-React application, or transition your existing codebase to React. | |
Let's look at how to get a ref, and then dive into a complete example. | |
@@ -91,11 +91,13 @@ In order to get a reference to a React component, you can either use `this` to g | |
var MyComponent = React.createClass({ | |
handleClick: function() { | |
// Explicitly focus the text input using the raw DOM API. | |
- this.myTextInput.focus(); | |
+ if (this.myTextInput !== null) { | |
+ this.myTextInput.focus(); | |
+ } | |
}, | |
render: function() { | |
- // The ref attribute adds a reference to the component to | |
- // this.refs when the component is mounted. | |
+ // The ref attribute is a callback that saves a reference to the | |
+ // component to this.myTextInput when the component is mounted. | |
return ( | |
<div> | |
<input type="text" ref={(ref) => this.myTextInput = ref} /> | |
@@ -131,7 +133,7 @@ Refs are a great way to send a message to a particular child instance in a way t | |
### Cautions: | |
-- *Never* access refs inside of any component's render method - or while any component's render method is even running anywhere in the call stack. | |
+- *Never* access refs inside of any component's render method – or while any component's render method is even running anywhere in the call stack. | |
- If you want to preserve Google Closure Compiler advanced-mode crushing resilience, make sure to never access as a property what was specified as a string. This means you must access using `this.refs['myRefString']` if your ref was defined as `ref="myRefString"`. | |
-- If you have not programmed several apps with React, your first inclination is usually going to be to try to use refs to "make things happen" in your app. If this is the case, take a moment and think more critically about where `state` should be owned in the component hierarchy. Often, it becomes clear that the proper place to "own" that state is at a higher level in the hierarchy. Placing the state there often eliminates any desire to use `ref`s to "make things happen" – instead, the data flow will usually accomplish your goal. | |
+- If you have not programmed several apps with React, your first inclination is usually going to be to try to use refs to "make things happen" in your app. If this is the case, take a moment and think more critically about where state should be owned in the component hierarchy. Often, it becomes clear that the proper place to "own" that state is at a higher level in the hierarchy. Placing the state there often eliminates any desire to use `ref`s to "make things happen" – instead, the data flow will usually accomplish your goal. | |
- Refs may not be attached to a [stateless function](/react/docs/reusable-components.html#stateless-functions), because the component does not have a backing instance. You can always wrap a stateless component in a standard composite component and attach a ref to the composite component. | |
diff --git a/docs/docs/09-tooling-integration.md b/docs/docs/09-tooling-integration.md | |
index fe18706..8c90f51 100644 | |
--- a/docs/docs/09-tooling-integration.md | |
+++ b/docs/docs/09-tooling-integration.md | |
@@ -22,7 +22,7 @@ We have instructions for building from `master` [in our GitHub repository](https | |
### In-browser JSX Transform | |
-If you like using JSX, Babel provides an [in-browser ES6 and JSX transformer for development](http://babeljs.io/docs/usage/browser/) called browser.js that can be included from a `babel-core` npm release or from [CDNJS](http://cdnjs.com/libraries/babel-core). Include a `<script type="text/babel">` tag to engage the JSX transformer. | |
+If you like using JSX, Babel 5 provided an in-browser ES6 and JSX transformer for development called browser.js that can be included from [CDNJS](http://cdnjs.com/libraries/babel-core/5.8.34). Include a `<script type="text/babel">` tag to engage the JSX transformer. | |
> Note: | |
> | |
diff --git a/docs/docs/10-addons.md b/docs/docs/10-addons.md | |
index a0a5cc5..1cf0294 100644 | |
--- a/docs/docs/10-addons.md | |
+++ b/docs/docs/10-addons.md | |
@@ -14,10 +14,11 @@ The React add-ons are a collection of useful utility modules for building React | |
- [`createFragment`](create-fragment.html), to create a set of externally-keyed children. | |
- [`update`](update.html), a helper function that makes dealing with immutable data in JavaScript easier. | |
- [`PureRenderMixin`](pure-render-mixin.html), a performance booster under certain situations. | |
+- [`shallowCompare`](shallow-compare.html), a helper function that performs a shallow comparison for props and state in a component to decide if a component should update. | |
The add-ons below are in the development (unminified) version of React only: | |
-- [`TestUtils`](test-utils.html), simple helpers for writing test cases (unminified build only). | |
-- [`Perf`](perf.html), for measuring performance and giving you hint where to optimize. | |
+- [`TestUtils`](test-utils.html), simple helpers for writing test cases. | |
+- [`Perf`](perf.html), a performance profiling tool for finding optimization opportunities. | |
To get the add-ons, install them individually from npm (e.g., `npm install react-addons-pure-render-mixin`). We don't support using the addons if you're not using npm. | |
diff --git a/docs/docs/10.1-animation.ko-KR.md b/docs/docs/10.1-animation.ko-KR.md | |
index ebaf6fb..dc6d5f1 100644 | |
--- a/docs/docs/10.1-animation.ko-KR.md | |
+++ b/docs/docs/10.1-animation.ko-KR.md | |
@@ -45,7 +45,7 @@ var TodoList = React.createClass({ | |
return ( | |
<div> | |
<button onClick={this.handleAdd}>Add Item</button> | |
- <ReactCSSTransitionGroup transitionName="example" transitionEnterTimeout={500} transitionLeaveTimeout={300} > | |
+ <ReactCSSTransitionGroup transitionName="example" transitionEnterTimeout={500} transitionLeaveTimeout={300}> | |
{items} | |
</ReactCSSTransitionGroup> | |
</div> | |
diff --git a/docs/docs/10.1-animation.md b/docs/docs/10.1-animation.md | |
index e0f735a..dcea2d9 100644 | |
--- a/docs/docs/10.1-animation.md | |
+++ b/docs/docs/10.1-animation.md | |
@@ -29,7 +29,7 @@ var TodoList = React.createClass({ | |
this.setState({items: newItems}); | |
}, | |
handleRemove: function(i) { | |
- var newItems = this.state.items; | |
+ var newItems = this.state.items.slice(); | |
newItems.splice(i, 1); | |
this.setState({items: newItems}); | |
}, | |
@@ -44,7 +44,7 @@ var TodoList = React.createClass({ | |
return ( | |
<div> | |
<button onClick={this.handleAdd}>Add Item</button> | |
- <ReactCSSTransitionGroup transitionName="example" transitionEnterTimeout={500} transitionLeaveTimeout={300} > | |
+ <ReactCSSTransitionGroup transitionName="example" transitionEnterTimeout={500} transitionLeaveTimeout={300}> | |
{items} | |
</ReactCSSTransitionGroup> | |
</div> | |
diff --git a/docs/docs/10.10-shallow-compare.md b/docs/docs/10.10-shallow-compare.md | |
new file mode 100644 | |
index 0000000..4ce3ee4 | |
--- /dev/null | |
+++ b/docs/docs/10.10-shallow-compare.md | |
@@ -0,0 +1,32 @@ | |
+--- | |
+id: shallow-compare | |
+title: Shallow Compare | |
+permalink: shallow-compare.html | |
+prev: perf.html | |
+next: advanced-performance.html | |
+--- | |
+ | |
+`shallowCompare` is a helper function to achieve the same functionality as `PureRenderMixin` while using ES6 classes with React. | |
+ | |
+If your React component's render function is "pure" (in other words, it renders the same result given the same props and state), you can use this helper function for a performance boost in some cases. | |
+ | |
+Example: | |
+ | |
+```js | |
+var shallowCompare = require('react-addons-shallow-compare'); | |
+export class SampleComponent extends React.Component { | |
+ shouldComponentUpdate(nextProps, nextState) { | |
+ return shallowCompare(this, nextProps, nextState); | |
+ } | |
+ | |
+ render() { | |
+ return <div className={this.props.className}>foo</div>; | |
+ } | |
+} | |
+``` | |
+ | |
+`shallowCompare` performs a shallow equality check on the current `props` and `nextProps` objects as well as the current `state` and `nextState` objects. | |
+It does this by iterating on the keys of the objects being compared and returning false when the value of a key in each object are not strictly equal. | |
+ | |
+`shallowCompare` returns `true` if the shallow comparison for props or state fails and therefore the component should update. | |
+`shallowCompare` returns `false` if the shallow comparison for props and state both pass and therefore the component does not need to update. | |
diff --git a/docs/docs/10.2-form-input-binding-sugar.md b/docs/docs/10.2-form-input-binding-sugar.md | |
index 8bc3513..7c4ae37 100644 | |
--- a/docs/docs/10.2-form-input-binding-sugar.md | |
+++ b/docs/docs/10.2-form-input-binding-sugar.md | |
@@ -10,7 +10,7 @@ next: test-utils.html | |
> Note: | |
> | |
-> If you're new to the framework, note that `ReactLink` is not needed for most applications and should be used cautiously. | |
+> ReactLink is deprecated as of React v15. The recommendation is to explicitly set the value and change handler, instead of using ReactLink. | |
In React, data flows one way: from owner to child. This is because data only flows one direction in [the Von Neumann model of computing](https://en.wikipedia.org/wiki/Von_Neumann_architecture). You can think of it as "one-way data binding." | |
diff --git a/docs/docs/10.3-class-name-manipulation.ko-KR.md b/docs/docs/10.3-class-name-manipulation.ko-KR.md | |
index 98765f9..463d6a1 100644 | |
--- a/docs/docs/10.3-class-name-manipulation.ko-KR.md | |
+++ b/docs/docs/10.3-class-name-manipulation.ko-KR.md | |
@@ -8,7 +8,7 @@ next: test-utils-ko-KR.html | |
> 주의: | |
> | |
-> 이 모듈은 이제 [JedWatson/classnames](https://github.com/JedWatson/classnames)에 독립적으로 있고 React와 관련없습니다. 그러므로 이 애드온은 제거될 예정입니다. | |
+> 이 모듈은 폐기예정입니다. 대신 [JedWatson/classnames](https://github.com/JedWatson/classnames)를 사용하세요. | |
`classSet()`은 간단히 DOM `class` 문자열을 조작하는 편리한 도구입니다. | |
diff --git a/docs/docs/10.3-class-name-manipulation.md b/docs/docs/10.3-class-name-manipulation.md | |
index d1427a0..4a3567d 100644 | |
--- a/docs/docs/10.3-class-name-manipulation.md | |
+++ b/docs/docs/10.3-class-name-manipulation.md | |
@@ -6,6 +6,6 @@ prev: two-way-binding-helpers.html | |
next: test-utils.html | |
--- | |
-> 주의: | |
+> NOTE: | |
> | |
-> 이 모듈은 폐기예정입니다. 대신 [JedWatson/classnames](https://github.com/JedWatson/classnames)를 사용하세요. | |
+> This module has been deprecated; use [JedWatson/classnames](https://github.com/JedWatson/classnames) instead. | |
diff --git a/docs/docs/10.4-test-utils.md b/docs/docs/10.4-test-utils.md | |
index cbbaa9d..c088990 100644 | |
--- a/docs/docs/10.4-test-utils.md | |
+++ b/docs/docs/10.4-test-utils.md | |
@@ -43,7 +43,7 @@ ReactTestUtils.Simulate.keyDown(node, {key: "Enter", keyCode: 13, which: 13}); | |
*Note that you will have to provide any event property that you're using in your component (e.g. keyCode, which, etc...) as React is not creating any of these for you.* | |
-`Simulate` has a method for every event that React understands. | |
+`Simulate` has a method for [every event that React understands](/react/docs/events.html#supported-events). | |
### renderIntoDocument | |
@@ -232,6 +232,7 @@ After `render` has been called, returns shallowly rendered output. You can then | |
Then you can assert: | |
```javascript | |
+var renderer = ReactTestUtils.createRenderer(); | |
result = renderer.getRenderOutput(); | |
expect(result.type).toBe('div'); | |
expect(result.props.children).toEqual([ | |
diff --git a/docs/docs/10.8-pure-render-mixin.md b/docs/docs/10.8-pure-render-mixin.md | |
index bd5143c..5b8c409 100644 | |
--- a/docs/docs/10.8-pure-render-mixin.md | |
+++ b/docs/docs/10.8-pure-render-mixin.md | |
@@ -21,6 +21,22 @@ React.createClass({ | |
}); | |
``` | |
+Example using ES6 class syntax: | |
+ | |
+```js | |
+import PureRenderMixin from 'react-addons-pure-render-mixin'; | |
+class FooComponent extends React.Component { | |
+ constructor(props) { | |
+ super(props); | |
+ this.shouldComponentUpdate = PureRenderMixin.shouldComponentUpdate.bind(this); | |
+ } | |
+ | |
+ render() { | |
+ return <div className={this.props.className}>foo</div>; | |
+ } | |
+} | |
+``` | |
+ | |
Under the hood, the mixin implements [shouldComponentUpdate](/react/docs/component-specs.html#updating-shouldcomponentupdate), in which it compares the current props and state with the next ones and returns `false` if the equalities pass. | |
> Note: | |
diff --git a/docs/docs/10.9-perf.md b/docs/docs/10.9-perf.md | |
index f4f6582..0a1e213 100644 | |
--- a/docs/docs/10.9-perf.md | |
+++ b/docs/docs/10.9-perf.md | |
@@ -3,7 +3,7 @@ id: perf | |
title: Performance Tools | |
permalink: perf.html | |
prev: pure-render-mixin.html | |
-next: advanced-performance.html | |
+next: shallow-compare.html | |
--- | |
React is usually quite fast out of the box. However, in situations where you need to squeeze every ounce of performance out of your app, it provides a [shouldComponentUpdate](/react/docs/component-specs.html#updating-shouldcomponentupdate) hook where you can add optimization hints to React's diff algorithm. | |
diff --git a/docs/docs/11-advanced-performance.md b/docs/docs/11-advanced-performance.md | |
index 12e7c5b..0d15756 100644 | |
--- a/docs/docs/11-advanced-performance.md | |
+++ b/docs/docs/11-advanced-performance.md | |
@@ -2,7 +2,7 @@ | |
id: advanced-performance | |
title: Advanced Performance | |
permalink: advanced-performance.html | |
-prev: perf.html | |
+prev: shallow-compare.html | |
next: context.html | |
--- | |
diff --git a/docs/docs/12-context.ko-KR.md b/docs/docs/12-context.ko-KR.md | |
index 56c29e2..a15d539 100644 | |
--- a/docs/docs/12-context.ko-KR.md | |
+++ b/docs/docs/12-context.ko-KR.md | |
@@ -124,6 +124,43 @@ var MessageList = React.createClass({ | |
원한다면 전체 React 컴포넌트를 프로퍼티로 전달할 수도 있습니다. | |
+## Referencing context in lifecycle methods | |
+ | |
+If `contextTypes` is defined within a component, the following lifecycle methods will receive an additional parameter, the `context` object: | |
+ | |
+```javascript | |
+void componentWillReceiveProps( | |
+ object nextProps, object nextContext | |
+) | |
+ | |
+boolean shouldComponentUpdate( | |
+ object nextProps, object nextState, object nextContext | |
+) | |
+ | |
+void componentWillUpdate( | |
+ object nextProps, object nextState, object nextContext | |
+) | |
+ | |
+void componentDidUpdate( | |
+ object prevProps, object prevState, object prevContext | |
+) | |
+``` | |
+ | |
+## Referencing context in stateless functional components | |
+ | |
+Stateless functional components are also able to reference `context` if `contextTypes` is defined as a property of the function. The following code shows the `Button` component above written as a stateless functional component. | |
+ | |
+```javascript | |
+function Button(props, context) { | |
+ return ( | |
+ <button style={{'{{'}}background: context.color}}> | |
+ {props.children} | |
+ </button> | |
+ ); | |
+} | |
+Button.contextTypes = {color: React.PropTypes.string}; | |
+``` | |
+ | |
## 컨텍스트를 사용하지 말아야 하는 경우 | |
대부분의 경우, 깔끔한 코드를 위해 전역 변수를 피하는 것과 마찬가지로 컨텍스트의 사용을 피해야 합니다. 특히 "타이핑을 줄이거나" 명시적인 프로퍼티 전달 대신 이를 사용하려는 경우 다시 한번 생각해 보세요. | |
diff --git a/docs/docs/12-context.md b/docs/docs/12-context.md | |
index 6b24d24..4c64736 100644 | |
--- a/docs/docs/12-context.md | |
+++ b/docs/docs/12-context.md | |
@@ -123,6 +123,75 @@ By passing down the relevant info in the `Menu` component, each `MenuItem` can c | |
Recall that you can also pass entire React components in props if you'd like to. | |
+## Referencing context in lifecycle methods | |
+ | |
+If `contextTypes` is defined within a component, the following lifecycle methods will receive an additional parameter, the `context` object: | |
+ | |
+```javascript | |
+void componentWillReceiveProps( | |
+ object nextProps, object nextContext | |
+) | |
+ | |
+boolean shouldComponentUpdate( | |
+ object nextProps, object nextState, object nextContext | |
+) | |
+ | |
+void componentWillUpdate( | |
+ object nextProps, object nextState, object nextContext | |
+) | |
+ | |
+void componentDidUpdate( | |
+ object prevProps, object prevState, object prevContext | |
+) | |
+``` | |
+ | |
+## Referencing context in stateless functional components | |
+ | |
+Stateless functional components are also able to reference `context` if `contextTypes` is defined as a property of the function. The following code shows the `Button` component above written as a stateless functional component. | |
+ | |
+```javascript | |
+function Button(props, context) { | |
+ return ( | |
+ <button style={{'{{'}}background: context.color}}> | |
+ {props.children} | |
+ </button> | |
+ ); | |
+} | |
+Button.contextTypes = {color: React.PropTypes.string}; | |
+``` | |
+ | |
+## Updating context | |
+ | |
+The `getChildContext` function will be called when the state or props changes. In order to update data in the context, trigger a local state update with `this.setState`. This will trigger a new context and changes will be received by the children. | |
+ | |
+```javascript | |
+var MediaQuery = React.createClass({ | |
+ getInitialState: function(){ | |
+ return {type:'desktop'}; | |
+ }, | |
+ childContextTypes: { | |
+ type: React.PropTypes.string | |
+ }, | |
+ getChildContext: function() { | |
+ return {type: this.state.type}; | |
+ }, | |
+ componentDidMount: function(){ | |
+ var checkMediaQuery = function(){ | |
+ var type = window.matchMedia("min-width: 1025px").matches ? 'desktop' : 'mobile'; | |
+ if (type !== this.state.type){ | |
+ this.setState({type:type}); | |
+ } | |
+ }; | |
+ | |
+ window.addEventListener('resize', checkMediaQuery); | |
+ checkMediaQuery(); | |
+ }, | |
+ render: function(){ | |
+ return this.props.children; | |
+ } | |
+}); | |
+``` | |
+ | |
## When not to use context | |
Just as global variables are best avoided when writing clear code, you should avoid using context in most cases. In particular, think twice before using it to "save typing" and using it instead of passing explicit props. | |
diff --git a/docs/docs/complementary-tools.ko-KR.md b/docs/docs/complementary-tools.ko-KR.md | |
index c387883..9807bf0 100644 | |
--- a/docs/docs/complementary-tools.ko-KR.md | |
+++ b/docs/docs/complementary-tools.ko-KR.md | |
@@ -1,9 +1,5 @@ | |
--- | |
-id: complementary-tools-ko-KR | |
-title: 상호 보완적인 도구들 | |
permalink: complementary-tools-ko-KR.html | |
-prev: videos-ko-KR.html | |
-next: examples-ko-KR.html | |
+layout: redirect | |
+dest_url: https://github.com/facebook/react/wiki/Complementary-Tools | |
--- | |
- | |
-이 페이지는 이동되었습니다. [GitHub wiki](https://github.com/facebook/react/wiki/Complementary-Tools). | |
diff --git a/docs/docs/complementary-tools.md b/docs/docs/complementary-tools.md | |
index 0d4190c..10e8dba 100644 | |
--- a/docs/docs/complementary-tools.md | |
+++ b/docs/docs/complementary-tools.md | |
@@ -1,9 +1,5 @@ | |
--- | |
-id: complementary-tools | |
-title: Complementary Tools | |
permalink: complementary-tools.html | |
-prev: videos.html | |
-next: examples.html | |
+layout: redirect | |
+dest_url: https://github.com/facebook/react/wiki/Complementary-Tools | |
--- | |
- | |
-This page has moved to the [GitHub wiki](https://github.com/facebook/react/wiki/Complementary-Tools). | |
diff --git a/docs/docs/conferences.md b/docs/docs/conferences.md | |
index a86915a..cb4a86d 100644 | |
--- a/docs/docs/conferences.md | |
+++ b/docs/docs/conferences.md | |
@@ -17,3 +17,13 @@ January 28 & 29 | |
July 2 & 3 | |
[Website](http://www.react-europe.org/) - [Schedule](http://www.react-europe.org/#schedule) | |
+ | |
+### Reactive 2015 | |
+November 2-4 | |
+ | |
+[Website](https://reactive2015.com/) - [Schedule](https://reactive2015.com/schedule_speakers.html#schedule) | |
+ | |
+### ReactEurope 2016 | |
+June 2 & 3 | |
+ | |
+[Website](http://www.react-europe.org/) - [Schedule](http://www.react-europe.org/#schedule) | |
diff --git a/docs/docs/examples.ko-KR.md b/docs/docs/examples.ko-KR.md | |
index 30b1971..3b46fa5 100644 | |
--- a/docs/docs/examples.ko-KR.md | |
+++ b/docs/docs/examples.ko-KR.md | |
@@ -1,8 +1,5 @@ | |
--- | |
-id: examples-ko-KR | |
-title: 예제들 | |
permalink: examples-ko-KR.html | |
-prev: complementary-tools-ko-KR.html | |
+layout: redirect | |
+dest_url: https://github.com/facebook/react/wiki/Examples | |
--- | |
- | |
-이 페이지는 이동되었습니다. [GitHub wiki](https://github.com/facebook/react/wiki/Examples). | |
diff --git a/docs/docs/examples.md b/docs/docs/examples.md | |
index 2143742..c697ffd 100644 | |
--- a/docs/docs/examples.md | |
+++ b/docs/docs/examples.md | |
@@ -1,8 +1,5 @@ | |
--- | |
-id: examples | |
-title: Examples | |
permalink: examples.html | |
-prev: complementary-tools.html | |
+layout: redirect | |
+dest_url: https://github.com/facebook/react/wiki/Examples | |
--- | |
- | |
-This page has moved to the [GitHub wiki](https://github.com/facebook/react/wiki/Examples). | |
diff --git a/docs/docs/getting-started.md b/docs/docs/getting-started.md | |
index 14f1363..cd93f37 100644 | |
--- a/docs/docs/getting-started.md | |
+++ b/docs/docs/getting-started.md | |
@@ -27,17 +27,33 @@ ReactDOM.render( | |
); | |
``` | |
-To install React DOM and build your bundle after installing browserify: | |
+To install React DOM and build your bundle with browserify: | |
```sh | |
$ npm install --save react react-dom babelify babel-preset-react | |
$ browserify -t [ babelify --presets [ react ] ] main.js -o bundle.js | |
``` | |
+To install React DOM and build your bundle with webpack: | |
+ | |
+```sh | |
+$ npm install --save react react-dom babel-preset-react | |
+$ webpack | |
+``` | |
+ | |
> Note: | |
> | |
> If you are using ES2015, you will want to also use the `babel-preset-es2015` package. | |
+**Note:** by default, React will be in development mode, which is slower, and not advised for production. To use React in production mode, set the environment variable `NODE_ENV` to `production` (using envify or webpack's DefinePlugin). For example: | |
+ | |
+```js | |
+new webpack.DefinePlugin({ | |
+ "process.env": { | |
+ NODE_ENV: JSON.stringify("production") | |
+ } | |
+}); | |
+``` | |
## Quick Start Without npm | |
@@ -73,7 +89,7 @@ In the root directory of the starter kit, create a `helloworld.html` with the fo | |
</html> | |
``` | |
-The XML syntax inside of JavaScript is called JSX; check out the [JSX syntax](/react/docs/jsx-in-depth.html) to learn more about it. In order to translate it to vanilla JavaScript we use `<script type="text/babel">` and include Babel to actually perform the transformation in the browser. | |
+The XML syntax inside of JavaScript is called JSX; check out the [JSX syntax](/react/docs/jsx-in-depth.html) to learn more about it. In order to translate it to vanilla JavaScript we use `<script type="text/babel">` and include Babel to actually perform the transformation in the browser. Open the html from a browser and you should already be able to see the greeting! | |
### Separate File | |
diff --git a/docs/docs/ref-01-top-level-api.md b/docs/docs/ref-01-top-level-api.md | |
index 4fdcdfd..7aeb715 100644 | |
--- a/docs/docs/ref-01-top-level-api.md | |
+++ b/docs/docs/ref-01-top-level-api.md | |
@@ -66,9 +66,7 @@ factoryFunction createFactory( | |
) | |
``` | |
-Return a function that produces ReactElements of a given type. Like `React.createElement`, | |
-the type argument can be either an html tag name string (eg. 'div', 'span', etc), or a | |
-`ReactClass`. | |
+Return a function that produces ReactElements of a given type. Like `React.createElement`, the type argument can be either an html tag name string (eg. 'div', 'span', etc), or a `ReactClass`. | |
### React.isValidElement | |
diff --git a/docs/docs/ref-02-component-api.md b/docs/docs/ref-02-component-api.md | |
index 919e202..216dd2c 100644 | |
--- a/docs/docs/ref-02-component-api.md | |
+++ b/docs/docs/ref-02-component-api.md | |
@@ -106,7 +106,7 @@ boolean isMounted() | |
> Note: | |
> | |
-> This method is not available on ES6 `class` components that extend `React.Component`. It may be removed entirely in a future version of React. | |
+> This method is not available on ES6 `class` components that extend `React.Component`. It will likely be removed entirely in a future version of React, so you might as well [start migrating away from isMounted() now](/react/blog/2015/12/16/ismounted-antipattern.html). | |
### setProps | |
@@ -124,11 +124,11 @@ Calling `setProps()` on a root-level component will change its properties and tr | |
> Note: | |
> | |
+> This method is deprecated and will be removed soon. This method is not available on ES6 `class` components that extend `React.Component`. Instead of calling `setProps`, try invoking ReactDOM.render() again with the new props. For additional notes, see our [blog post about using the Top Level API](/react/blog/2015/10/01/react-render-and-top-level-api.html) | |
+> | |
> When possible, the declarative approach of calling `ReactDOM.render()` again on the same node is preferred instead. It tends to make updates easier to reason about. (There's no significant performance difference between the two approaches.) | |
> | |
> This method can only be called on a root-level component. That is, it's only available on the component passed directly to `ReactDOM.render()` and none of its children. If you're inclined to use `setProps()` on a child component, instead take advantage of reactive updates and pass the new prop to the child component when it's created in `render()`. | |
-> | |
-> This method is not available on ES6 `class` components that extend `React.Component`. It may be removed entirely in a future version of React. | |
### replaceProps | |
@@ -143,4 +143,4 @@ Like `setProps()` but deletes any pre-existing props instead of merging the two | |
> Note: | |
> | |
-> This method is not available on ES6 `class` components that extend `React.Component`. It may be removed entirely in a future version of React. | |
+> This method is deprecated and will be removed soon. This method is not available on ES6 `class` components that extend `React.Component`. Instead of calling `replaceProps`, try invoking ReactDOM.render() again with the new props. For additional notes, see our [blog post about using the Top Level API](/react/blog/2015/10/01/react-render-and-top-level-api.html) | |
diff --git a/docs/docs/ref-03-component-specs.md b/docs/docs/ref-03-component-specs.md | |
index 2855caa..9e5a956 100644 | |
--- a/docs/docs/ref-03-component-specs.md | |
+++ b/docs/docs/ref-03-component-specs.md | |
@@ -148,6 +148,8 @@ componentWillReceiveProps: function(nextProps) { | |
> Note: | |
> | |
+> One common mistake is for code executed during this lifecycle method to assume that props have changed. To understand why this is invalid, read [A implies B does not imply B implies A](/react/blog/2016/01/08/A-implies-B-does-not-imply-B-implies-A.html) | |
+> | |
> There is no analogous method `componentWillReceiveState`. An incoming prop transition may cause a state change, but the opposite is not true. If you need to perform operations in response to a state change, use `componentWillUpdate`. | |
@@ -161,8 +163,7 @@ boolean shouldComponentUpdate( | |
Invoked before rendering when new props or state are being received. This method is not called for the initial render or when `forceUpdate` is used. | |
-Use this as an opportunity to `return false` when you're certain that the | |
-transition to the new props and state will not require a component update. | |
+Use this as an opportunity to `return false` when you're certain that the transition to the new props and state will not require a component update. | |
```javascript | |
shouldComponentUpdate: function(nextProps, nextState) { | |
diff --git a/docs/docs/ref-04-tags-and-attributes.ko-KR.md b/docs/docs/ref-04-tags-and-attributes.ko-KR.md | |
index 4b93ff3..a08d0cd 100644 | |
--- a/docs/docs/ref-04-tags-and-attributes.ko-KR.md | |
+++ b/docs/docs/ref-04-tags-and-attributes.ko-KR.md | |
@@ -59,7 +59,7 @@ controls coords crossOrigin data dateTime defer dir disabled download draggable | |
encType form formAction formEncType formMethod formNoValidate formTarget frameBorder | |
headers height hidden high href hrefLang htmlFor httpEquiv icon id inputMode | |
keyParams keyType label lang list loop low manifest marginHeight marginWidth max | |
-maxLength media mediaGroup method min minlength multiple muted name noValidate open | |
+maxLength media mediaGroup method min minLength multiple muted name noValidate open | |
optimum pattern placeholder poster preload radioGroup readOnly rel required role | |
rows rowSpan sandbox scope scoped scrolling seamless selected shape size sizes | |
span spellCheck src srcDoc srcSet start step style summary tabIndex target title | |
diff --git a/docs/docs/ref-04-tags-and-attributes.md b/docs/docs/ref-04-tags-and-attributes.md | |
index 488c5db..8481c75 100644 | |
--- a/docs/docs/ref-04-tags-and-attributes.md | |
+++ b/docs/docs/ref-04-tags-and-attributes.md | |
@@ -18,11 +18,11 @@ The following HTML elements are supported: | |
a abbr address area article aside audio b base bdi bdo big blockquote body br | |
button canvas caption cite code col colgroup data datalist dd del details dfn | |
dialog div dl dt em embed fieldset figcaption figure footer form h1 h2 h3 h4 h5 | |
-h6 head header hr html i iframe img input ins kbd keygen label legend li link | |
-main map mark menu menuitem meta meter nav noscript object ol optgroup option | |
-output p param picture pre progress q rp rt ruby s samp script section select | |
-small source span strong style sub summary sup table tbody td textarea tfoot th | |
-thead time title tr track u ul var video wbr | |
+h6 head header hgroup hr html i iframe img input ins kbd keygen label legend li | |
+link main map mark menu menuitem meta meter nav noscript object ol optgroup | |
+option output p param picture pre progress q rp rt ruby s samp script section | |
+select small source span strong style sub summary sup table tbody td textarea | |
+tfoot th thead time title tr track u ul var video wbr | |
``` | |
### SVG elements | |
@@ -30,11 +30,11 @@ thead time title tr track u ul var video wbr | |
The following SVG elements are supported: | |
``` | |
-circle clipPath defs ellipse g line linearGradient mask path pattern polygon polyline | |
-radialGradient rect stop svg text tspan | |
+circle clipPath defs ellipse g image line linearGradient mask path pattern | |
+polygon polyline radialGradient rect stop svg text tspan | |
``` | |
-You may also be interested in [react-art](https://github.com/facebook/react-art), a drawing library for React that can render to Canvas, SVG, or VML (for IE8). | |
+You may also be interested in [react-art](https://github.com/facebook/react-art), a cross-browser drawing library for React. | |
## Supported Attributes | |
@@ -53,24 +53,32 @@ These standard attributes are supported: | |
``` | |
accept acceptCharset accessKey action allowFullScreen allowTransparency alt | |
-async autoComplete autoFocus autoPlay capture cellPadding cellSpacing charSet | |
-challenge checked classID className cols colSpan content contentEditable contextMenu | |
-controls coords crossOrigin data dateTime defer dir disabled download draggable | |
-encType form formAction formEncType formMethod formNoValidate formTarget frameBorder | |
-headers height hidden high href hrefLang htmlFor httpEquiv icon id inputMode | |
-keyParams keyType label lang list loop low manifest marginHeight marginWidth max | |
-maxLength media mediaGroup method min minlength multiple muted name noValidate open | |
-optimum pattern placeholder poster preload radioGroup readOnly rel required role | |
-rows rowSpan sandbox scope scoped scrolling seamless selected shape size sizes | |
-span spellCheck src srcDoc srcSet start step style summary tabIndex target title | |
-type useMap value width wmode wrap | |
+async autoComplete autoFocus autoPlay capture cellPadding cellSpacing challenge | |
+charSet checked classID className colSpan cols content contentEditable | |
+contextMenu controls coords crossOrigin data dateTime default defer dir | |
+disabled download draggable encType form formAction formEncType formMethod | |
+formNoValidate formTarget frameBorder headers height hidden high href hrefLang | |
+htmlFor httpEquiv icon id inputMode integrity is keyParams keyType kind label | |
+lang list loop low manifest marginHeight marginWidth max maxLength media | |
+mediaGroup method min minLength multiple muted name noValidate nonce open | |
+optimum pattern placeholder poster preload radioGroup readOnly rel required | |
+reversed role rowSpan rows sandbox scope scoped scrolling seamless selected | |
+shape size sizes span spellCheck src srcDoc srcLang srcSet start step style | |
+summary tabIndex target title type useMap value width wmode wrap | |
+``` | |
+ | |
+These RDFa attributes are supported (several RDFa attributes overlap with standard HTML attributes and thus are excluded from this list): | |
+ | |
+``` | |
+about datatype inlist prefix property resource typeof vocab | |
``` | |
In addition, the following non-standard attributes are supported: | |
- `autoCapitalize autoCorrect` for Mobile Safari. | |
-- `property` for [Open Graph](http://ogp.me/) meta tags. | |
+- `color` for `<link rel="mask-icon" />` in Safari. | |
- `itemProp itemScope itemType itemRef itemID` for [HTML5 microdata](http://schema.org/docs/gs.html). | |
+- `security` for older versions of Internet Explorer. | |
- `unselectable` for Internet Explorer. | |
- `results autoSave` for WebKit/Blink input fields of type `search`. | |
@@ -78,13 +86,6 @@ There is also the React-specific attribute `dangerouslySetInnerHTML` ([more here | |
### SVG Attributes | |
-``` | |
-clipPath cx cy d dx dy fill fillOpacity fontFamily | |
-fontSize fx fy gradientTransform gradientUnits markerEnd | |
-markerMid markerStart offset opacity patternContentUnits | |
-patternUnits points preserveAspectRatio r rx ry spreadMethod | |
-stopColor stopOpacity stroke strokeDasharray strokeLinecap | |
-strokeOpacity strokeWidth textAnchor transform version | |
-viewBox x1 x2 x xlinkActuate xlinkArcrole xlinkHref xlinkRole | |
-xlinkShow xlinkTitle xlinkType xmlBase xmlLang xmlSpace y1 y2 y | |
-``` | |
+Any attributes passed to SVG tags are passed through without changes. | |
+ | |
+React used to support special camelCase aliases for certain SVG attributes, such as `clipPath`. If you use them now you'll see a deprecation warning. These aliases will be removed in the next version in favor of their real names from the SVG specification, such as `clip-path`. Attributes that have a camelCase name in the spec, such as `gradientTransform`, will keep their names. | |
diff --git a/docs/docs/ref-05-events.md b/docs/docs/ref-05-events.md | |
index edb4256..93ad7fe 100644 | |
--- a/docs/docs/ref-05-events.md | |
+++ b/docs/docs/ref-05-events.md | |
@@ -61,8 +61,7 @@ function onClick(event) { | |
## Supported Events | |
-React normalizes events so that they have consistent properties across | |
-different browsers. | |
+React normalizes events so that they have consistent properties across different browsers. | |
The event handlers below are triggered by an event in the bubbling phase. To register an event handler for the capture phase, append `Capture` to the event name; for example, instead of using `onClick`, you would use `onClickCapture` to handle the click event in the capture phase. | |
@@ -140,6 +139,7 @@ DOMEventTarget relatedTarget | |
These focus events work on all elements in the React DOM, not just form elements. | |
+ | |
### Form Events | |
Event names: | |
@@ -183,7 +183,7 @@ boolean shiftKey | |
``` | |
-### Selection events | |
+### Selection Events | |
Event names: | |
@@ -192,7 +192,7 @@ onSelect | |
``` | |
-### Touch events | |
+### Touch Events | |
Event names: | |
@@ -247,6 +247,7 @@ number deltaY | |
number deltaZ | |
``` | |
+ | |
### Media Events | |
Event names: | |
@@ -255,6 +256,7 @@ Event names: | |
onAbort onCanPlay onCanPlayThrough onDurationChange onEmptied onEncrypted onEnded onError onLoadedData onLoadedMetadata onLoadStart onPause onPlay onPlaying onProgress onRateChange onSeeked onSeeking onStalled onSuspend onTimeUpdate onVolumeChange onWaiting | |
``` | |
+ | |
### Image Events | |
Event names: | |
@@ -262,3 +264,37 @@ Event names: | |
``` | |
onLoad onError | |
``` | |
+ | |
+ | |
+### Animation Events | |
+ | |
+Event names: | |
+ | |
+``` | |
+onAnimationStart onAnimationEnd onAnimationIteration | |
+``` | |
+ | |
+Properties: | |
+ | |
+```javascript | |
+string animationName | |
+string pseudoElement | |
+float elapsedTime | |
+``` | |
+ | |
+ | |
+### Transition Events | |
+ | |
+Event names: | |
+ | |
+``` | |
+onTransitionEnd | |
+``` | |
+ | |
+Properties: | |
+ | |
+```javascript | |
+string propertyName | |
+string pseudoElement | |
+float elapsedTime | |
+``` | |
diff --git a/docs/docs/ref-06-dom-differences.md b/docs/docs/ref-06-dom-differences.md | |
index ceeeac9..3d51061 100644 | |
--- a/docs/docs/ref-06-dom-differences.md | |
+++ b/docs/docs/ref-06-dom-differences.md | |
@@ -10,6 +10,7 @@ React has implemented a browser-independent events and DOM system for performanc | |
* All DOM properties and attributes (including event handlers) should be camelCased to be consistent with standard JavaScript style. We intentionally break with the spec here since the spec is inconsistent. **However**, `data-*` and `aria-*` attributes [conform to the specs](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes#data-*) and should be lower-cased only. | |
* The `style` attribute accepts a JavaScript object with camelCased properties rather than a CSS string. This is consistent with the DOM `style` JavaScript property, is more efficient, and prevents XSS security holes. | |
+* Since `class` and `for` are reserved words in JavaScript, the JSX elements for built-in [DOM nodes](http://javascript.info/tutorial/dom-nodes) should use the attribute names `className` and `htmlFor` respectively, (eg. `<div className="foo" />` ). Custom elements should use `class` and `for` directly (eg. `<my-tag class="foo" />` ). | |
* All event objects conform to the W3C spec, and all events (including submit) bubble correctly per the W3C spec. See [Event System](/react/docs/events.html) for more details. | |
* The `onChange` event behaves as you would expect it to: whenever a form field is changed this event is fired rather than inconsistently on blur. We intentionally break from existing browser behavior because `onChange` is a misnomer for its behavior and React relies on this event to react to user input in real time. See [Forms](/react/docs/forms.html) for more details. | |
* Form input attributes such as `value` and `checked`, as well as `textarea`. [More here](/react/docs/forms.html). | |
diff --git a/docs/docs/ref-08-reconciliation.ko-KR.md b/docs/docs/ref-08-reconciliation.ko-KR.md | |
index febf7cb..e8bce33 100644 | |
--- a/docs/docs/ref-08-reconciliation.ko-KR.md | |
+++ b/docs/docs/ref-08-reconciliation.ko-KR.md | |
@@ -3,7 +3,7 @@ id: reconciliation-ko-KR | |
title: 비교조정(Reconciliation) | |
permalink: reconciliation-ko-KR.html | |
prev: special-non-dom-attributes-ko-KR.html | |
-next: glossary-ko-KR.html | |
+next: webcomponents.html | |
--- | |
React의 주요 설계 철학은 업데이트할 때마다 전체 앱을 다시 렌더하는 것처럼 보이게 API를 만드는 것입니다. 이렇게 하면 애플리케이션 작성이 훨씬 쉬워지지만 한편으로는 어려운 도전 과제이기도 합니다. 이 글에서는 강력한 휴리스틱으로 어떻게 O(n<sup>3</sup>) 복잡도의 문제를 O(n)짜리로 바꿀 수 있었는지 설명합니다. | |
diff --git a/docs/docs/ref-08-reconciliation.md b/docs/docs/ref-08-reconciliation.md | |
index 25d20b2..aefd2ed 100644 | |
--- a/docs/docs/ref-08-reconciliation.md | |
+++ b/docs/docs/ref-08-reconciliation.md | |
@@ -3,7 +3,7 @@ id: reconciliation | |
title: Reconciliation | |
permalink: reconciliation.html | |
prev: special-non-dom-attributes.html | |
-next: glossary.html | |
+next: webcomponents.html | |
--- | |
React's key design decision is to make the API seem like it re-renders the whole app on every update. This makes writing applications a lot easier but is also an incredible challenge to make it tractable. This article explains how with powerful heuristics we managed to turn a O(n<sup>3</sup>) problem into a O(n) one. | |
@@ -13,7 +13,7 @@ React's key design decision is to make the API seem like it re-renders the whole | |
Generating the minimum number of operations to transform one tree into another is a complex and well-studied problem. The [state of the art algorithms](http://grfia.dlsi.ua.es/ml/algorithms/references/editsurvey_bille.pdf) have a complexity in the order of O(n<sup>3</sup>) where n is the number of nodes in the tree. | |
-This means that displaying 1000 nodes would require in the order of one billion comparisons. This is far too expensive for our use case. To put this number in perspective, CPUs nowadays execute roughly 3 billion instruction per second. So even with the most performant implementation, we wouldn't be able to compute that diff in less than a second. | |
+This means that displaying 1000 nodes would require in the order of one billion comparisons. This is far too expensive for our use case. To put this number in perspective, CPUs nowadays execute roughly 3 billion instructions per second. So even with the most performant implementation, we wouldn't be able to compute that diff in less than a second. | |
Since an optimal algorithm is not tractable, we implement a non-optimal O(n) algorithm using heuristics based on two assumptions: | |
@@ -76,7 +76,7 @@ After the attributes have been updated, we recurse on all the children. | |
### Custom Components | |
-We decided that the two custom components are the same. Since components are stateful, we cannot just use the new component and call it a day. React takes all the attributes from the new component and calls `component[Will/Did]ReceiveProps()` on the previous one. | |
+We decided that the two custom components are the same. Since components are stateful, we cannot just use the new component and call it a day. React takes all the attributes from the new component and calls `componentWillReceiveProps()` and `componentWillUpdate()` on the previous one. | |
The previous component is now operational. Its `render()` method is called and the diff algorithm restarts with the new result and the previous result. | |
@@ -129,5 +129,5 @@ Because we rely on two heuristics, if the assumptions behind them are not met, p | |
1. The algorithm will not try to match sub-trees of different components classes. If you see yourself alternating between two components classes with very similar output, you may want to make it the same class. In practice, we haven't found this to be an issue. | |
-2. If you don't provide stable keys (by using Math.random() for example), all the sub-trees are going to be re-rendered every single time. By giving the users the choice to choose the key, they have the ability to shoot themselves in the foot. | |
+2. Keys should be stable, predictable, and unique. Unstable keys (like those produced by Math.random()) will cause many nodes to be unnecessarily re-created, which can cause performance degradation and lost state in child components. | |
diff --git a/docs/docs/ref-09-glossary.ko-KR.md b/docs/docs/ref-09-glossary.ko-KR.md | |
deleted file mode 100644 | |
index ad27e6e..0000000 | |
--- a/docs/docs/ref-09-glossary.ko-KR.md | |
+++ /dev/null | |
@@ -1,193 +0,0 @@ | |
---- | |
-id: glossary-ko-KR | |
-title: React (가상) DOM 용어 | |
-permalink: glossary-ko-KR.html | |
-prev: reconciliation-ko-KR.html | |
---- | |
- | |
-다음은 React에서 사용되는 용어들로, 이 다섯 가지의 타입을 구별하는 것은 중요합니다. | |
- | |
-- [ReactElement / ReactElement 팩토리](#react-elements) | |
-- [ReactNode](#react-nodes) | |
-- [ReactComponent / ReactComponent 클래스](#react-components) | |
- | |
-## React 엘리먼트 | |
- | |
-`ReactElement`는 React의 주요 타입입니다. `type`, `props`, `key`, `ref`의 네 가지 프로퍼티를 가집니다. 메소드는 가지지 않으며 프로토타입에는 아무 것도 들어있지 않습니다. | |
- | |
-이러한 객체는 `React.createElement`를 통해 만들 수 있습니다. | |
- | |
-```javascript | |
-var root = React.createElement('div'); | |
-``` | |
- | |
-DOM에 새로운 트리를 렌더링하기 위해서는 `ReactElement`를 만들고 일반적인 DOM `Element` (`HTMLElement` 또는 `SVGElement`)와 함께 `ReactDOM.render`에 넘깁니다. `ReactElement`를 DOM `Element`와 혼동해서는 안됩니다. `ReactElement`는 가볍고, 상태를 갖지 않으며, 변경 불가능한, DOM `Element`의 가상 표현입니다. 즉 가상 DOM입니다. | |
- | |
-```javascript | |
-ReactDOM.render(root, document.getElementById('example')); | |
-``` | |
- | |
-DOM 엘리먼트에 프로퍼티를 추가하려면 두번째 인자로 프로퍼티 객체를, 세번째 인자로 자식을 넘깁니다. | |
- | |
-```javascript | |
-var child = React.createElement('li', null, 'Text Content'); | |
-var root = React.createElement('ul', { className: 'my-list' }, child); | |
-ReactDOM.render(root, document.getElementById('example')); | |
-``` | |
- | |
-React JSX를 사용하면 `ReactElement`가 알아서 만들어집니다. 따라서 다음 코드는 앞의 코드와 같습니다: | |
- | |
-```javascript | |
-var root = <ul className="my-list"> | |
- <li>Text Content</li> | |
- </ul>; | |
-ReactDOM.render(root, document.getElementById('example')); | |
-``` | |
- | |
-### 팩토리 | |
- | |
-`ReactElement` 팩토리는 그저 특정한 `type` 프로퍼티를 가지는 `ReactElement`를 만들어주는 함수입니다. React에는 팩토리를 만드는 헬퍼가 내장되어 있습니다. 그 함수는 사실상 다음과 같습니다: | |
- | |
-```javascript | |
-function createFactory(type) { | |
- return React.createElement.bind(null, type); | |
-} | |
-``` | |
- | |
-이를 이용하면 편리한 단축 함수를 만들 수 있어 항상 `React.createElement('div')`를 입력하지 않아도 됩니다. | |
- | |
-```javascript | |
-var div = React.createFactory('div'); | |
-var root = div({ className: 'my-div' }); | |
-ReactDOM.render(root, document.getElementById('example')); | |
-``` | |
- | |
-React에는 이미 보통의 HTML 태그를 위한 팩토리가 내장되어 있습니다: | |
- | |
-```javascript | |
-var root = React.DOM.ul({ className: 'my-list' }, | |
- React.DOM.li(null, 'Text Content') | |
- ); | |
-``` | |
- | |
-JSX를 사용하면 팩토리가 필요하지 않습니다. 이미 JSX가 `ReactElement`를 만드는 편리한 단축 문법을 제공합니다. | |
- | |
- | |
-## React 노드 | |
- | |
-`ReactNode`는 다음 중 하나가 될 수 있습니다: | |
- | |
-- `ReactElement` | |
-- `string` (`ReactText`로 부르기도 함) | |
-- `number` (`ReactText`로 부르기도 함) | |
-- `ReactNode`의 배열 (`ReactFragment`로 부르기도 함) | |
- | |
-이들은 자식을 표현하기 위해 다른 `ReactElement`의 프로퍼티에 사용됩니다. 사실상 이들이 `ReactElement`의 트리를 형성합니다. | |
- | |
- | |
-## React 컴포넌트 | |
- | |
-`ReactElement`만 가지고도 React를 사용할 수는 있지만, React의 장점을 제대로 활용하려면 `ReactComponent`를 사용하여 상태를 가진 캡슐화된 객체를 만들기를 원할 것입니다. | |
- | |
-`ReactComponent` 클래스는 그냥 JavaScript 클래스 (또는 "생성자 함수")입니다. | |
- | |
-```javascript | |
-var MyComponent = React.createClass({ | |
- render: function() { | |
- ... | |
- } | |
-}); | |
-``` | |
- | |
-이 생성자가 호출될 때 최소한 `render` 메소드를 가진 객체를 리턴해야 합니다. 이 리턴된 객체를 `ReactComponent`라고 부릅니다. | |
- | |
-```javascript | |
-var component = new MyComponent(props); // 절대 하지 마세요. | |
-``` | |
- | |
-테스트 목적이 아니라면 *절대* 이 생성자를 직접 호출하지 마십시오. React가 알아서 호출해줍니다. | |
- | |
-대신 `ReactComponent` 클래스를 `createElement`에 넘겨 `ReactElement`를 받을 수 있습니다. | |
- | |
-```javascript | |
-var element = React.createElement(MyComponent); | |
-``` | |
- | |
-또는 JSX를 사용하면: | |
- | |
-```javascript | |
-var element = <MyComponent />; | |
-``` | |
- | |
-이것이 `ReactDOM.render`에 넘겨지면 React가 알아서 생성자를 호출하여 `ReactComponent`를 만들고 리턴합니다. | |
- | |
-```javascript | |
-var component = ReactDOM.render(element, document.getElementById('example')); | |
-``` | |
- | |
-같은 타입의 `ReactElement`와 같은 컨테이너 DOM `Element`를 가지고 `ReactDOM.render`를 계속 호출하면 항상 같은 인스턴스를 리턴합니다. 이 인스턴스는 상태를 가집니다. | |
- | |
-```javascript | |
-var componentA = ReactDOM.render(<MyComponent />, document.getElementById('example')); | |
-var componentB = ReactDOM.render(<MyComponent />, document.getElementById('example')); | |
-componentA === componentB; // true | |
-``` | |
- | |
-그렇기 때문에 직접 인스턴스를 만들어서는 안됩니다. `ReactComponent`가 생성되기 전에 `ReactElement`가 대신 가상의 `ReactComponent` 역할을 합니다. 이전 `ReactElement`와 새 `ReactElement`를 비교하여 새로운 `ReactComponent`를 만들지, 아니면 기존 것을 재사용할지 결정합니다. | |
- | |
-`ReactComponent`의 `render` 메소드는 또다른 `ReactElement`를 리턴해야 합니다. 이렇게 해서 컴포넌트들이 조합됩니다. 결과적으로 렌더링 과정은 다음과 같습니다. `string` 타입의 태그를 가진 `ReactElement`를 통해 DOM `Element` 인스턴스가 생성되며 문서에 삽입됩니다. | |
- | |
- | |
-## 형식 타입 정의 | |
- | |
-### 진입점 | |
- | |
-``` | |
-ReactDOM.render = (ReactElement, HTMLElement | SVGElement) => ReactComponent; | |
-``` | |
- | |
-### 노드와 엘리먼트 | |
- | |
-``` | |
-type ReactNode = ReactElement | ReactFragment | ReactText; | |
- | |
-type ReactElement = ReactComponentElement | ReactDOMElement; | |
- | |
-type ReactDOMElement = { | |
- type : string, | |
- props : { | |
- children : ReactNodeList, | |
- className : string, | |
- etc. | |
- }, | |
- key : string | boolean | number | null, | |
- ref : string | null | |
-}; | |
- | |
-type ReactComponentElement<TProps> = { | |
- type : ReactClass<TProps>, | |
- props : TProps, | |
- key : string | boolean | number | null, | |
- ref : string | null | |
-}; | |
- | |
-type ReactFragment = Array<ReactNode | ReactEmpty>; | |
- | |
-type ReactNodeList = ReactNode | ReactEmpty; | |
- | |
-type ReactText = string | number; | |
- | |
-type ReactEmpty = null | undefined | boolean; | |
-``` | |
- | |
-### 클래스와 컴포넌트 | |
- | |
-``` | |
-type ReactClass<TProps> = (TProps) => ReactComponent<TProps>; | |
- | |
-type ReactComponent<TProps> = { | |
- props : TProps, | |
- render : () => ReactElement | |
-}; | |
-``` | |
- | |
diff --git a/docs/docs/ref-09-glossary.md b/docs/docs/ref-09-glossary.md | |
deleted file mode 100644 | |
index 50ccc00..0000000 | |
--- a/docs/docs/ref-09-glossary.md | |
+++ /dev/null | |
@@ -1,193 +0,0 @@ | |
---- | |
-id: glossary | |
-title: React (Virtual) DOM Terminology | |
-permalink: glossary.html | |
-prev: reconciliation.html | |
---- | |
- | |
-In React's terminology, there are five core types that are important to distinguish: | |
- | |
-- [ReactElement / ReactElement Factory](#react-elements) | |
-- [ReactNode](#react-nodes) | |
-- [ReactComponent / ReactComponent Class](#react-components) | |
- | |
-## React Elements | |
- | |
-The primary type in React is the `ReactElement`. It has four properties: `type`, `props`, `key` and `ref`. It has no methods and nothing on the prototype. | |
- | |
-You can create one of these objects through `React.createElement`. | |
- | |
-```javascript | |
-var root = React.createElement('div'); | |
-``` | |
- | |
-To render a new tree into the DOM, you create `ReactElement`s and pass them to `ReactDOM.render` along with a regular DOM `Element` (`HTMLElement` or `SVGElement`). `ReactElement`s are not to be confused with DOM `Element`s. A `ReactElement` is a light, stateless, immutable, virtual representation of a DOM `Element`. It is a virtual DOM. | |
- | |
-```javascript | |
-ReactDOM.render(root, document.getElementById('example')); | |
-``` | |
- | |
-To add properties to a DOM element, pass a properties object as the second argument and children to the third argument. | |
- | |
-```javascript | |
-var child = React.createElement('li', null, 'Text Content'); | |
-var root = React.createElement('ul', { className: 'my-list' }, child); | |
-ReactDOM.render(root, document.getElementById('example')); | |
-``` | |
- | |
-If you use React JSX, then these `ReactElement`s are created for you. So this is equivalent: | |
- | |
-```javascript | |
-var root = <ul className="my-list"> | |
- <li>Text Content</li> | |
- </ul>; | |
-ReactDOM.render(root, document.getElementById('example')); | |
-``` | |
- | |
-### Factories | |
- | |
-A `ReactElement`-factory is simply a function that generates a `ReactElement` with a particular `type` property. React has a built-in helper for you to create factories. It's effectively just: | |
- | |
-```javascript | |
-function createFactory(type) { | |
- return React.createElement.bind(null, type); | |
-} | |
-``` | |
- | |
-It allows you to create a convenient short-hand instead of typing out `React.createElement('div')` all the time. | |
- | |
-```javascript | |
-var div = React.createFactory('div'); | |
-var root = div({ className: 'my-div' }); | |
-ReactDOM.render(root, document.getElementById('example')); | |
-``` | |
- | |
-React already has built-in factories for common HTML tags: | |
- | |
-```javascript | |
-var root = React.DOM.ul({ className: 'my-list' }, | |
- React.DOM.li(null, 'Text Content') | |
- ); | |
-``` | |
- | |
-If you are using JSX you have no need for factories. JSX already provides a convenient short-hand for creating `ReactElement`s. | |
- | |
- | |
-## React Nodes | |
- | |
-A `ReactNode` can be either: | |
- | |
-- `ReactElement` | |
-- `string` (aka `ReactText`) | |
-- `number` (aka `ReactText`) | |
-- Array of `ReactNode`s (aka `ReactFragment`) | |
- | |
-These are used as properties of other `ReactElement`s to represent children. Effectively they create a tree of `ReactElement`s. | |
- | |
- | |
-## React Components | |
- | |
-You can use React using only `ReactElement`s but to really take advantage of React, you'll want to use `ReactComponent`s to create encapsulations with embedded state. | |
- | |
-A `ReactComponent` Class is simply just a JavaScript class (or "constructor function"). | |
- | |
-```javascript | |
-var MyComponent = React.createClass({ | |
- render: function() { | |
- ... | |
- } | |
-}); | |
-``` | |
- | |
-When this constructor is invoked it is expected to return an object with at least a `render` method on it. This object is referred to as a `ReactComponent`. | |
- | |
-```javascript | |
-var component = new MyComponent(props); // never do this | |
-``` | |
- | |
-Other than for testing, you would normally *never* call this constructor yourself. React calls it for you. | |
- | |
-Instead, you pass the `ReactComponent` Class to `createElement` you get a `ReactElement`. | |
- | |
-```javascript | |
-var element = React.createElement(MyComponent); | |
-``` | |
- | |
-OR using JSX: | |
- | |
-```javascript | |
-var element = <MyComponent />; | |
-``` | |
- | |
-When this is passed to `ReactDOM.render`, React will call the constructor for you and create a `ReactComponent`, which is returned. | |
- | |
-```javascript | |
-var component = ReactDOM.render(element, document.getElementById('example')); | |
-``` | |
- | |
-If you keep calling `ReactDOM.render` with the same type of `ReactElement` and the same container DOM `Element` it always returns the same instance. This instance is stateful. | |
- | |
-```javascript | |
-var componentA = ReactDOM.render(<MyComponent />, document.getElementById('example')); | |
-var componentB = ReactDOM.render(<MyComponent />, document.getElementById('example')); | |
-componentA === componentB; // true | |
-``` | |
- | |
-This is why you shouldn't construct your own instance. Instead, `ReactElement` is a virtual `ReactComponent` before it gets constructed. An old and new `ReactElement` can be compared to see if a new `ReactComponent` instance is created or if the existing one is reused. | |
- | |
-The `render` method of a `ReactComponent` is expected to return another `ReactElement`. This allows these components to be composed. Ultimately the render resolves into `ReactElement` with a `string` tag which instantiates a DOM `Element` instance and inserts it into the document. | |
- | |
- | |
-## Formal Type Definitions | |
- | |
-### Entry Point | |
- | |
-``` | |
-ReactDOM.render = (ReactElement, HTMLElement | SVGElement) => ReactComponent; | |
-``` | |
- | |
-### Nodes and Elements | |
- | |
-``` | |
-type ReactNode = ReactElement | ReactFragment | ReactText; | |
- | |
-type ReactElement = ReactComponentElement | ReactDOMElement; | |
- | |
-type ReactDOMElement = { | |
- type : string, | |
- props : { | |
- children : ReactNodeList, | |
- className : string, | |
- etc. | |
- }, | |
- key : string | boolean | number | null, | |
- ref : string | null | |
-}; | |
- | |
-type ReactComponentElement<TProps> = { | |
- type : ReactClass<TProps>, | |
- props : TProps, | |
- key : string | boolean | number | null, | |
- ref : string | null | |
-}; | |
- | |
-type ReactFragment = Array<ReactNode | ReactEmpty>; | |
- | |
-type ReactNodeList = ReactNode | ReactEmpty; | |
- | |
-type ReactText = string | number; | |
- | |
-type ReactEmpty = null | undefined | boolean; | |
-``` | |
- | |
-### Classes and Components | |
- | |
-``` | |
-type ReactClass<TProps> = (TProps) => ReactComponent<TProps>; | |
- | |
-type ReactComponent<TProps> = { | |
- props : TProps, | |
- render : () => ReactElement | |
-}; | |
-``` | |
- | |
diff --git a/docs/docs/ref-09-webcomponents.md b/docs/docs/ref-09-webcomponents.md | |
new file mode 100644 | |
index 0000000..3442bcf | |
--- /dev/null | |
+++ b/docs/docs/ref-09-webcomponents.md | |
@@ -0,0 +1,56 @@ | |
+--- | |
+id: webcomponents | |
+title: Web Components | |
+permalink: webcomponents.html | |
+prev: reconciliation.html | |
+next: glossary.html | |
+--- | |
+ | |
+Trying to compare and contrast React with WebComponents inevitably results in specious conclusions, because the two libraries are built to solve different problems. WebComponents provide strong encapsulation for reusable components, while React provides a declarative library that keeps the DOM in sync with your data. The two goals are complementary; engineers can mix-and-match the technologies. As a developer, you are free to use React in your WebComponents, or to use WebComponents in React, or both. | |
+ | |
+## Using Web Components in React | |
+ | |
+```javascript | |
+class HelloMessage extends React.Component{ | |
+ render() { | |
+ return <div>Hello <x-search>{this.props.name}</x-search>!</div>; | |
+ } | |
+} | |
+``` | |
+ | |
+> Note: | |
+> | |
+> The programming models of the two component systems (web components vs. react components) differ in that | |
+> web components often expose an imperative API (for instance, a `video` web component might expose `play()` | |
+> and `pause()` functions). To the extent that web components are declarative functions of their attributes, | |
+> they should work, but to access the imperative APIs of a web component, you will need to attach a ref to the | |
+> component and interact with the DOM node directly. If you are using third-party web components, the | |
+> recommended solution is to write a React component that behaves as a wrapper for your web component. | |
+> | |
+> At this time, events emitted by a web component may not properly propagate through a React render tree. | |
+> You will need to manually attach event handlers to handle these events within your React components. | |
+ | |
+ | |
+## Using React in your Web Components | |
+ | |
+ | |
+```javascript | |
+var proto = Object.create(HTMLElement.prototype, { | |
+ createdCallback: { | |
+ value: function() { | |
+ var mountPoint = document.createElement('span'); | |
+ this.createShadowRoot().appendChild(mountPoint); | |
+ | |
+ var name = this.getAttribute('name'); | |
+ var url = 'https://www.google.com/search?q=' + encodeURIComponent(name); | |
+ ReactDOM.render(<a href={url}>{name}</a>, mountPoint); | |
+ } | |
+ } | |
+}); | |
+document.registerElement('x-search', {prototype: proto}); | |
+``` | |
+ | |
+## Complete Example | |
+ | |
+Check out the `webcomponents` example in the [starter kit](/react/downloads.html) for a complete example. | |
+ | |
diff --git a/docs/docs/ref-10-glossary.ko-KR.md b/docs/docs/ref-10-glossary.ko-KR.md | |
new file mode 100644 | |
index 0000000..0113104 | |
--- /dev/null | |
+++ b/docs/docs/ref-10-glossary.ko-KR.md | |
@@ -0,0 +1,193 @@ | |
+--- | |
+id: glossary-ko-KR | |
+title: React (가상) DOM 용어 | |
+permalink: glossary-ko-KR.html | |
+prev: webcomponents-ko-KR.html | |
+--- | |
+ | |
+다음은 React에서 사용되는 용어들로, 이 다섯 가지의 타입을 구별하는 것은 중요합니다. | |
+ | |
+- [ReactElement / ReactElement 팩토리](#react-elements) | |
+- [ReactNode](#react-nodes) | |
+- [ReactComponent / ReactComponent 클래스](#react-components) | |
+ | |
+## React 엘리먼트 | |
+ | |
+`ReactElement`는 React의 주요 타입입니다. `type`, `props`, `key`, `ref`의 네 가지 프로퍼티를 가집니다. 메소드는 가지지 않으며 프로토타입에는 아무 것도 들어있지 않습니다. | |
+ | |
+이러한 객체는 `React.createElement`를 통해 만들 수 있습니다. | |
+ | |
+```javascript | |
+var root = React.createElement('div'); | |
+``` | |
+ | |
+DOM에 새로운 트리를 렌더링하기 위해서는 `ReactElement`를 만들고 일반적인 DOM `Element` (`HTMLElement` 또는 `SVGElement`)와 함께 `ReactDOM.render`에 넘깁니다. `ReactElement`를 DOM `Element`와 혼동해서는 안됩니다. `ReactElement`는 가볍고, 상태를 갖지 않으며, 변경 불가능한, DOM `Element`의 가상 표현입니다. 즉 가상 DOM입니다. | |
+ | |
+```javascript | |
+ReactDOM.render(root, document.getElementById('example')); | |
+``` | |
+ | |
+DOM 엘리먼트에 프로퍼티를 추가하려면 두번째 인자로 프로퍼티 객체를, 세번째 인자로 자식을 넘깁니다. | |
+ | |
+```javascript | |
+var child = React.createElement('li', null, 'Text Content'); | |
+var root = React.createElement('ul', { className: 'my-list' }, child); | |
+ReactDOM.render(root, document.getElementById('example')); | |
+``` | |
+ | |
+React JSX를 사용하면 `ReactElement`가 알아서 만들어집니다. 따라서 다음 코드는 앞의 코드와 같습니다: | |
+ | |
+```javascript | |
+var root = <ul className="my-list"> | |
+ <li>Text Content</li> | |
+ </ul>; | |
+ReactDOM.render(root, document.getElementById('example')); | |
+``` | |
+ | |
+### 팩토리 | |
+ | |
+`ReactElement` 팩토리는 그저 특정한 `type` 프로퍼티를 가지는 `ReactElement`를 만들어주는 함수입니다. React에는 팩토리를 만드는 헬퍼가 내장되어 있습니다. 그 함수는 사실상 다음과 같습니다: | |
+ | |
+```javascript | |
+function createFactory(type) { | |
+ return React.createElement.bind(null, type); | |
+} | |
+``` | |
+ | |
+이를 이용하면 편리한 단축 함수를 만들 수 있어 항상 `React.createElement('div')`를 입력하지 않아도 됩니다. | |
+ | |
+```javascript | |
+var div = React.createFactory('div'); | |
+var root = div({ className: 'my-div' }); | |
+ReactDOM.render(root, document.getElementById('example')); | |
+``` | |
+ | |
+React에는 이미 보통의 HTML 태그를 위한 팩토리가 내장되어 있습니다: | |
+ | |
+```javascript | |
+var root = React.DOM.ul({ className: 'my-list' }, | |
+ React.DOM.li(null, 'Text Content') | |
+ ); | |
+``` | |
+ | |
+JSX를 사용하면 팩토리가 필요하지 않습니다. 이미 JSX가 `ReactElement`를 만드는 편리한 단축 문법을 제공합니다. | |
+ | |
+ | |
+## React 노드 | |
+ | |
+`ReactNode`는 다음 중 하나가 될 수 있습니다: | |
+ | |
+- `ReactElement` | |
+- `string` (`ReactText`로 부르기도 함) | |
+- `number` (`ReactText`로 부르기도 함) | |
+- `ReactNode`의 배열 (`ReactFragment`로 부르기도 함) | |
+ | |
+이들은 자식을 표현하기 위해 다른 `ReactElement`의 프로퍼티에 사용됩니다. 사실상 이들이 `ReactElement`의 트리를 형성합니다. | |
+ | |
+ | |
+## React 컴포넌트 | |
+ | |
+`ReactElement`만 가지고도 React를 사용할 수는 있지만, React의 장점을 제대로 활용하려면 `ReactComponent`를 사용하여 상태를 가진 캡슐화된 객체를 만들기를 원할 것입니다. | |
+ | |
+`ReactComponent` 클래스는 그냥 JavaScript 클래스 (또는 "생성자 함수")입니다. | |
+ | |
+```javascript | |
+var MyComponent = React.createClass({ | |
+ render: function() { | |
+ ... | |
+ } | |
+}); | |
+``` | |
+ | |
+이 생성자가 호출될 때 최소한 `render` 메소드를 가진 객체를 리턴해야 합니다. 이 리턴된 객체를 `ReactComponent`라고 부릅니다. | |
+ | |
+```javascript | |
+var component = new MyComponent(props); // 절대 하지 마세요. | |
+``` | |
+ | |
+테스트 목적이 아니라면 *절대* 이 생성자를 직접 호출하지 마십시오. React가 알아서 호출해줍니다. | |
+ | |
+대신 `ReactComponent` 클래스를 `createElement`에 넘겨 `ReactElement`를 받을 수 있습니다. | |
+ | |
+```javascript | |
+var element = React.createElement(MyComponent); | |
+``` | |
+ | |
+또는 JSX를 사용하면: | |
+ | |
+```javascript | |
+var element = <MyComponent />; | |
+``` | |
+ | |
+이것이 `ReactDOM.render`에 넘겨지면 React가 알아서 생성자를 호출하여 `ReactComponent`를 만들고 리턴합니다. | |
+ | |
+```javascript | |
+var component = ReactDOM.render(element, document.getElementById('example')); | |
+``` | |
+ | |
+같은 타입의 `ReactElement`와 같은 컨테이너 DOM `Element`를 가지고 `ReactDOM.render`를 계속 호출하면 항상 같은 인스턴스를 리턴합니다. 이 인스턴스는 상태를 가집니다. | |
+ | |
+```javascript | |
+var componentA = ReactDOM.render(<MyComponent />, document.getElementById('example')); | |
+var componentB = ReactDOM.render(<MyComponent />, document.getElementById('example')); | |
+componentA === componentB; // true | |
+``` | |
+ | |
+그렇기 때문에 직접 인스턴스를 만들어서는 안됩니다. `ReactComponent`가 생성되기 전에 `ReactElement`가 대신 가상의 `ReactComponent` 역할을 합니다. 이전 `ReactElement`와 새 `ReactElement`를 비교하여 새로운 `ReactComponent`를 만들지, 아니면 기존 것을 재사용할지 결정합니다. | |
+ | |
+`ReactComponent`의 `render` 메소드는 또다른 `ReactElement`를 리턴해야 합니다. 이렇게 해서 컴포넌트들이 조합됩니다. 결과적으로 렌더링 과정은 다음과 같습니다. `string` 타입의 태그를 가진 `ReactElement`를 통해 DOM `Element` 인스턴스가 생성되며 문서에 삽입됩니다. | |
+ | |
+ | |
+## 형식 타입 정의 | |
+ | |
+### 진입점 | |
+ | |
+``` | |
+ReactDOM.render = (ReactElement, HTMLElement | SVGElement) => ReactComponent; | |
+``` | |
+ | |
+### 노드와 엘리먼트 | |
+ | |
+``` | |
+type ReactNode = ReactElement | ReactFragment | ReactText; | |
+ | |
+type ReactElement = ReactComponentElement | ReactDOMElement; | |
+ | |
+type ReactDOMElement = { | |
+ type : string, | |
+ props : { | |
+ children : ReactNodeList, | |
+ className : string, | |
+ etc. | |
+ }, | |
+ key : string | boolean | number | null, | |
+ ref : string | null | |
+}; | |
+ | |
+type ReactComponentElement<TProps> = { | |
+ type : ReactClass<TProps>, | |
+ props : TProps, | |
+ key : string | boolean | number | null, | |
+ ref : string | null | |
+}; | |
+ | |
+type ReactFragment = Array<ReactNode | ReactEmpty>; | |
+ | |
+type ReactNodeList = ReactNode | ReactEmpty; | |
+ | |
+type ReactText = string | number; | |
+ | |
+type ReactEmpty = null | undefined | boolean; | |
+``` | |
+ | |
+### 클래스와 컴포넌트 | |
+ | |
+``` | |
+type ReactClass<TProps> = (TProps) => ReactComponent<TProps>; | |
+ | |
+type ReactComponent<TProps> = { | |
+ props : TProps, | |
+ render : () => ReactElement | |
+}; | |
+``` | |
+ | |
diff --git a/docs/docs/ref-10-glossary.md b/docs/docs/ref-10-glossary.md | |
new file mode 100644 | |
index 0000000..f5e5533 | |
--- /dev/null | |
+++ b/docs/docs/ref-10-glossary.md | |
@@ -0,0 +1,193 @@ | |
+--- | |
+id: glossary | |
+title: React (Virtual) DOM Terminology | |
+permalink: glossary.html | |
+prev: webcomponents.html | |
+--- | |
+ | |
+In React's terminology, there are five core types that are important to distinguish: | |
+ | |
+- [ReactElement / ReactElement Factory](#react-elements) | |
+- [ReactNode](#react-nodes) | |
+- [ReactComponent / ReactComponent Class](#react-components) | |
+ | |
+## React Elements | |
+ | |
+The primary type in React is the `ReactElement`. It has four properties: `type`, `props`, `key` and `ref`. It has no methods and nothing on the prototype. | |
+ | |
+You can create one of these objects through `React.createElement`. | |
+ | |
+```javascript | |
+var root = React.createElement('div'); | |
+``` | |
+ | |
+To render a new tree into the DOM, you create `ReactElement`s and pass them to `ReactDOM.render` along with a regular DOM `Element` (`HTMLElement` or `SVGElement`). `ReactElement`s are not to be confused with DOM `Element`s. A `ReactElement` is a light, stateless, immutable, virtual representation of a DOM `Element`. It is a virtual DOM. | |
+ | |
+```javascript | |
+ReactDOM.render(root, document.getElementById('example')); | |
+``` | |
+ | |
+To add properties to a DOM element, pass a properties object as the second argument and children to the third argument. | |
+ | |
+```javascript | |
+var child = React.createElement('li', null, 'Text Content'); | |
+var root = React.createElement('ul', { className: 'my-list' }, child); | |
+ReactDOM.render(root, document.getElementById('example')); | |
+``` | |
+ | |
+If you use React JSX, then these `ReactElement`s are created for you. So this is equivalent: | |
+ | |
+```javascript | |
+var root = <ul className="my-list"> | |
+ <li>Text Content</li> | |
+ </ul>; | |
+ReactDOM.render(root, document.getElementById('example')); | |
+``` | |
+ | |
+### Factories | |
+ | |
+A `ReactElement`-factory is simply a function that generates a `ReactElement` with a particular `type` property. React has a built-in helper for you to create factories. It's effectively just: | |
+ | |
+```javascript | |
+function createFactory(type) { | |
+ return React.createElement.bind(null, type); | |
+} | |
+``` | |
+ | |
+It allows you to create a convenient short-hand instead of typing out `React.createElement('div')` all the time. | |
+ | |
+```javascript | |
+var div = React.createFactory('div'); | |
+var root = div({ className: 'my-div' }); | |
+ReactDOM.render(root, document.getElementById('example')); | |
+``` | |
+ | |
+React already has built-in factories for common HTML tags: | |
+ | |
+```javascript | |
+var root = React.DOM.ul({ className: 'my-list' }, | |
+ React.DOM.li(null, 'Text Content') | |
+ ); | |
+``` | |
+ | |
+If you are using JSX you have no need for factories. JSX already provides a convenient short-hand for creating `ReactElement`s. | |
+ | |
+ | |
+## React Nodes | |
+ | |
+A `ReactNode` can be either: | |
+ | |
+- `ReactElement` | |
+- `string` (aka `ReactText`) | |
+- `number` (aka `ReactText`) | |
+- Array of `ReactNode`s (aka `ReactFragment`) | |
+ | |
+These are used as properties of other `ReactElement`s to represent children. Effectively they create a tree of `ReactElement`s. | |
+ | |
+ | |
+## React Components | |
+ | |
+You can use React using only `ReactElement`s but to really take advantage of React, you'll want to use `ReactComponent`s to create encapsulations with embedded state. | |
+ | |
+A `ReactComponent` Class is simply just a JavaScript class (or "constructor function"). | |
+ | |
+```javascript | |
+var MyComponent = React.createClass({ | |
+ render: function() { | |
+ ... | |
+ } | |
+}); | |
+``` | |
+ | |
+When this constructor is invoked it is expected to return an object with at least a `render` method on it. This object is referred to as a `ReactComponent`. | |
+ | |
+```javascript | |
+var component = new MyComponent(props); // never do this | |
+``` | |
+ | |
+Other than for testing, you would normally *never* call this constructor yourself. React calls it for you. | |
+ | |
+Instead, you pass the `ReactComponent` Class to `createElement` you get a `ReactElement`. | |
+ | |
+```javascript | |
+var element = React.createElement(MyComponent); | |
+``` | |
+ | |
+OR using JSX: | |
+ | |
+```javascript | |
+var element = <MyComponent />; | |
+``` | |
+ | |
+When this is passed to `ReactDOM.render`, React will call the constructor for you and create a `ReactComponent`, which is returned. | |
+ | |
+```javascript | |
+var component = ReactDOM.render(element, document.getElementById('example')); | |
+``` | |
+ | |
+If you keep calling `ReactDOM.render` with the same type of `ReactElement` and the same container DOM `Element` it always returns the same instance. This instance is stateful. | |
+ | |
+```javascript | |
+var componentA = ReactDOM.render(<MyComponent />, document.getElementById('example')); | |
+var componentB = ReactDOM.render(<MyComponent />, document.getElementById('example')); | |
+componentA === componentB; // true | |
+``` | |
+ | |
+This is why you shouldn't construct your own instance. Instead, `ReactElement` is a virtual `ReactComponent` before it gets constructed. An old and new `ReactElement` can be compared to see if a new `ReactComponent` instance should be created or if the existing one should be reused. | |
+ | |
+The `render` method of a `ReactComponent` is expected to return another `ReactElement`. This allows these components to be composed. Ultimately the render resolves into `ReactElement` with a `string` tag which instantiates a DOM `Element` instance and inserts it into the document. | |
+ | |
+ | |
+## Formal Type Definitions | |
+ | |
+### Entry Point | |
+ | |
+``` | |
+ReactDOM.render = (ReactElement, HTMLElement | SVGElement) => ReactComponent; | |
+``` | |
+ | |
+### Nodes and Elements | |
+ | |
+``` | |
+type ReactNode = ReactElement | ReactFragment | ReactText; | |
+ | |
+type ReactElement = ReactComponentElement | ReactDOMElement; | |
+ | |
+type ReactDOMElement = { | |
+ type : string, | |
+ props : { | |
+ children : ReactNodeList, | |
+ className : string, | |
+ etc. | |
+ }, | |
+ key : string | boolean | number | null, | |
+ ref : string | null | |
+}; | |
+ | |
+type ReactComponentElement<TProps> = { | |
+ type : ReactClass<TProps>, | |
+ props : TProps, | |
+ key : string | boolean | number | null, | |
+ ref : string | null | |
+}; | |
+ | |
+type ReactFragment = Array<ReactNode | ReactEmpty>; | |
+ | |
+type ReactNodeList = ReactNode | ReactEmpty; | |
+ | |
+type ReactText = string | number; | |
+ | |
+type ReactEmpty = null | undefined | boolean; | |
+``` | |
+ | |
+### Classes and Components | |
+ | |
+``` | |
+type ReactClass<TProps> = (TProps) => ReactComponent<TProps>; | |
+ | |
+type ReactComponent<TProps> = { | |
+ props : TProps, | |
+ render : () => ReactElement | |
+}; | |
+``` | |
+ | |
diff --git a/docs/docs/thinking-in-react.ko-KR.md b/docs/docs/thinking-in-react.ko-KR.md | |
index 5c023db..6476545 100644 | |
--- a/docs/docs/thinking-in-react.ko-KR.md | |
+++ b/docs/docs/thinking-in-react.ko-KR.md | |
@@ -61,7 +61,7 @@ React의 많은 뛰어난 점들 중 하나는 생각을 하면서 애플리케 | |
## 2단계: 정적 버전을 만드세요. | |
-<iframe width="100%" height="300" src="https://jsfiddle.net/reactjs/yun1vgqb/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
+<iframe width="100%" height="600" src="https://jsfiddle.net/reactjs/yun1vgqb/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
계층구조의 컴포넌트들을 가지고 있으니, 이젠 애플리케이션을 구현할 시간입니다. 가장 쉬운 방법은 상호작용을 하지 않는 채로 자료 모델을 이용해 UI를 그리는 것입니다. 정적 버전을 만드는 데에는 적은 생각과 많은 노동이 필요하고, 상호작용을 추가하는 데에는 많은 생각과 적은 노동이 필요하기 때문에 둘을 분리하는 것이 가장 좋습니다. 왜 그런지 봅시다. | |
@@ -105,7 +105,7 @@ product 들의 원본 목록은 props를 통해서 전달되기 때문에, state | |
## 4단계: 어디서 state가 유지되어야 하는지 확인하세요. | |
-<iframe width="100%" height="300" src="https://jsfiddle.net/reactjs/zafjbw1e/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
+<iframe width="100%" height="600" src="https://jsfiddle.net/reactjs/zafjbw1e/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
이제 최소한의 state가 무엇인지 알아냈습니다. 다음은, 어떤 컴포넌트가 이 state를 변형하거나 만들어낼지 알아내야 합니다. | |
@@ -130,7 +130,7 @@ product 들의 원본 목록은 props를 통해서 전달되기 때문에, state | |
## 5단계: 반대방향 자료 흐름을 추가하세요. | |
-<iframe width="100%" height="300" src="https://jsfiddle.net/reactjs/n47gckhr/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
+<iframe width="100%" height="600" src="https://jsfiddle.net/reactjs/n47gckhr/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
앞서 우리는 계층적으로 아랫방향 흐름의 props, state전달로 잘 동작하는 애플리케이션을 만들었습니다. 이제 다른방향의 자료 흐름을 지원할 시간입니다: form 컴포넌트들은 `FilterableProductTable`의 state를 업데이트할 필요성이 있죠. | |
diff --git a/docs/docs/thinking-in-react.md b/docs/docs/thinking-in-react.md | |
index 85cee61..bdaf5ad 100644 | |
--- a/docs/docs/thinking-in-react.md | |
+++ b/docs/docs/thinking-in-react.md | |
@@ -31,7 +31,7 @@ Our JSON API returns some data that looks like this: | |
]; | |
``` | |
-## Step 1: break the UI into a component hierarchy | |
+## Step 1: Break the UI into a component hierarchy | |
The first thing you'll want to do is to draw boxes around every component (and subcomponent) in the mock and give them all names. If you're working with a designer, they may have already done this, so go talk to them! Their Photoshop layer names may end up being the names of your React components! | |
@@ -61,7 +61,7 @@ Now that we've identified the components in our mock, let's arrange them into a | |
## Step 2: Build a static version in React | |
-<iframe width="100%" height="300" src="https://jsfiddle.net/reactjs/yun1vgqb/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
+<iframe width="100%" height="600" src="https://jsfiddle.net/reactjs/yun1vgqb/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
Now that you have your component hierarchy, it's time to implement your app. The easiest way is to build a version that takes your data model and renders the UI but has no interactivity. It's best to decouple these processes because building a static version requires a lot of typing and no thinking, and adding interactivity requires a lot of thinking and not a lot of typing. We'll see why. | |
@@ -81,7 +81,7 @@ There are two types of "model" data in React: props and state. It's important to | |
To make your UI interactive, you need to be able to trigger changes to your underlying data model. React makes this easy with **state**. | |
-To build your app correctly, you first need to think of the minimal set of mutable state that your app needs. The key here is DRY: *Don't Repeat Yourself*. Figure out what the absolute minimal representation of the state of your application needs to be and compute everything else you need on-demand. For example, if you're building a TODO list, just keep an array of the TODO items around; don't keep a separate state variable for the count. Instead, when you want to render the TODO count, simply take the length of the TODO items array. | |
+To build your app correctly, you first need to think of the minimal set of mutable state that your app needs. The key here is DRY: *Don't Repeat Yourself*. Figure out the absolute minimal representation of the state your application needs and compute everything else you need on-demand. For example, if you're building a TODO list, just keep an array of the TODO items around; don't keep a separate state variable for the count. Instead, when you want to render the TODO count, simply take the length of the TODO items array. | |
Think of all of the pieces of data in our example application. We have: | |
@@ -105,7 +105,7 @@ So finally, our state is: | |
## Step 4: Identify where your state should live | |
-<iframe width="100%" height="300" src="https://jsfiddle.net/reactjs/zafjbw1e/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
+<iframe width="100%" height="600" src="https://jsfiddle.net/reactjs/zafjbw1e/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
OK, so we've identified what the minimal set of app state is. Next, we need to identify which component mutates, or *owns*, this state. | |
@@ -130,7 +130,7 @@ You can start seeing how your application will behave: set `filterText` to `"bal | |
## Step 5: Add inverse data flow | |
-<iframe width="100%" height="300" src="https://jsfiddle.net/reactjs/n47gckhr/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
+<iframe width="100%" height="600" src="https://jsfiddle.net/reactjs/n47gckhr/embedded/" allowfullscreen="allowfullscreen" frameborder="0"></iframe> | |
So far, we've built an app that renders correctly as a function of props and state flowing down the hierarchy. Now it's time to support data flowing the other way: the form components deep in the hierarchy need to update the state in `FilterableProductTable`. | |
diff --git a/docs/docs/tutorial.ko-KR.md b/docs/docs/tutorial.ko-KR.md | |
old mode 100644 | |
new mode 100755 | |
index 83c1b00..fa29741 | |
--- a/docs/docs/tutorial.ko-KR.md | |
+++ b/docs/docs/tutorial.ko-KR.md | |
@@ -44,7 +44,7 @@ next: thinking-in-react-ko-KR.html | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/{{site.react_version}}/react.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/{{site.react_version}}/react-dom.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.8.23/browser.min.js"></script> | |
- <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> | |
+ <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.2.0/jquery.min.js"></script> | |
</head> | |
<body> | |
<div id="content"></div> | |
@@ -234,7 +234,7 @@ Markdown은 텍스트를 포맷팅하는 간단한 방식입니다. 예를 들 | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/{{site.react_version}}/react-dom.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.8.23/browser.min.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> | |
- <script src="https://cdnjs.cloudflare.com/ajax/libs/marked/0.3.2/marked.min.js"></script> | |
+ <script src="https://cdnjs.cloudflare.com/ajax/libs/marked/0.3.5/marked.min.js"></script> | |
</head> | |
``` | |
@@ -262,7 +262,7 @@ var Comment = React.createClass({ | |
React는 이런 식으로 [XSS 공격](https://en.wikipedia.org/wiki/Cross-site_scripting)을 예방합니다. 우회할 방법이 있긴 하지만 프레임워크는 사용하지 않도록 경고하고 있습니다: | |
-```javascript{4,14} | |
+```javascript{3-6,14} | |
// tutorial7.js | |
var Comment = React.createClass({ | |
rawMarkup: function() { | |
@@ -662,7 +662,7 @@ var CommentBox = React.createClass({ | |
우리의 애플리케이션은 이제 모든 기능을 갖추었습니다. 하지만 댓글이 목록에 업데이트되기 전에 완료요청을 기다리는 게 조금 느린듯한 느낌이 드네요. 우리는 낙관적 업데이트를 통해 댓글이 목록에 추가되도록 함으로써 앱이 좀 더 빨라진 것처럼 느껴지도록 할 수 있습니다. | |
-```javascript{17-19} | |
+```javascript{17-19,29} | |
// tutorial20.js | |
var CommentBox = React.createClass({ | |
loadCommentsFromServer: function() { | |
@@ -691,6 +691,7 @@ var CommentBox = React.createClass({ | |
this.setState({data: data}); | |
}.bind(this), | |
error: function(xhr, status, err) { | |
+ this.setState({data: comments}); | |
console.error(this.props.url, status, err.toString()); | |
}.bind(this) | |
}); | |
diff --git a/docs/docs/tutorial.md b/docs/docs/tutorial.md | |
old mode 100644 | |
new mode 100755 | |
index 83bab0d..0878bd6 | |
--- a/docs/docs/tutorial.md | |
+++ b/docs/docs/tutorial.md | |
@@ -31,7 +31,7 @@ For sake of simplicity, the server we will run uses a `JSON` file as a database. | |
### Getting started | |
-For this tutorial, we're going to make it as easy as possible. Included in the server package discussed above is an HTML file which we'll work in. Open up `public/index.html` in your favorite editor. It should look something like this (with perhaps some minor differences, we'll add an additional `<script>` tag later): | |
+For this tutorial, we're going to make it as easy as possible. Included in the server package discussed above is an HTML file which we'll work in. Open up `public/index.html` in your favorite editor. It should look something like this: | |
```html | |
<!-- index.html --> | |
@@ -43,7 +43,8 @@ For this tutorial, we're going to make it as easy as possible. Included in the s | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/{{site.react_version}}/react.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/{{site.react_version}}/react-dom.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.8.23/browser.min.js"></script> | |
- <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> | |
+ <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.2.0/jquery.min.js"></script> | |
+ <script src="https://cdnjs.cloudflare.com/ajax/libs/marked/0.3.5/marked.min.js"></script> | |
</head> | |
<body> | |
<div id="content"></div> | |
@@ -129,6 +130,8 @@ You do not have to return basic HTML. You can return a tree of components that y | |
The `ReactDOM` module exposes DOM-specific methods, while `React` has the core tools shared by React on different platforms (e.g., [React Native](http://facebook.github.io/react-native/)). | |
+It is important that `ReactDOM.render` remain at the bottom of the script for this tutorial. `ReactDOM.render` should only be called after the composite components have been defined. | |
+ | |
## Composing components | |
Let's build skeletons for `CommentList` and `CommentForm` which will, again, be simple `<div>`s. Add these two components to your file, keeping the existing `CommentBox` declaration and `ReactDOM.render` call: | |
@@ -221,22 +224,7 @@ Note that we have passed some data from the parent `CommentList` component to th | |
Markdown is a simple way to format your text inline. For example, surrounding text with asterisks will make it emphasized. | |
-First, add the third-party library **marked** to your application. This is a JavaScript library which takes Markdown text and converts it to raw HTML. This requires a script tag in your head (which we have already included in the React playground): | |
- | |
-```html{9} | |
-<!-- index.html --> | |
-<head> | |
- <meta charset="utf-8" /> | |
- <title>React Tutorial</title> | |
- <script src="https://cdnjs.cloudflare.com/ajax/libs/react/{{site.react_version}}/react.js"></script> | |
- <script src="https://cdnjs.cloudflare.com/ajax/libs/react/{{site.react_version}}/react-dom.js"></script> | |
- <script src="https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.8.23/browser.min.js"></script> | |
- <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> | |
- <script src="https://cdnjs.cloudflare.com/ajax/libs/marked/0.3.2/marked.min.js"></script> | |
-</head> | |
-``` | |
- | |
-Next, let's convert the comment text to Markdown and output it: | |
+In this tutorial we use a third-party library **marked** which takes Markdown text and converts it to raw HTML. We already included this library with the original markup for the page, so we can just start using it. Let's convert the comment text to Markdown and output it: | |
```javascript{9} | |
// tutorial6.js | |
@@ -260,7 +248,7 @@ But there's a problem! Our rendered comments look like this in the browser: "`<p | |
That's React protecting you from an [XSS attack](https://en.wikipedia.org/wiki/Cross-site_scripting). There's a way to get around it but the framework warns you not to use it: | |
-```javascript{4,14} | |
+```javascript{3-6,14} | |
// tutorial7.js | |
var Comment = React.createClass({ | |
rawMarkup: function() { | |
@@ -292,8 +280,8 @@ So far we've been inserting the comments directly in the source code. Instead, l | |
```javascript | |
// tutorial8.js | |
var data = [ | |
- {author: "Pete Hunt", text: "This is one comment"}, | |
- {author: "Jordan Walke", text: "This is *another* comment"} | |
+ {id: 1, author: "Pete Hunt", text: "This is one comment"}, | |
+ {id: 2, author: "Jordan Walke", text: "This is *another* comment"} | |
]; | |
``` | |
@@ -325,9 +313,9 @@ Now that the data is available in the `CommentList`, let's render the comments d | |
// tutorial10.js | |
var CommentList = React.createClass({ | |
render: function() { | |
- var commentNodes = this.props.data.map(function (comment) { | |
+ var commentNodes = this.props.data.map(function(comment) { | |
return ( | |
- <Comment author={comment.author}> | |
+ <Comment author={comment.author} key={comment.id}> | |
{comment.text} | |
</Comment> | |
); | |
@@ -388,7 +376,7 @@ var CommentBox = React.createClass({ | |
`getInitialState()` executes exactly once during the lifecycle of the component and sets up the initial state of the component. | |
#### Updating state | |
-When the component is first created, we want to GET some JSON from the server and update the state to reflect the latest data. We're going to use jQuery to make an asynchronous request to the server we started earlier to fetch the data we need. It will look something like this: | |
+When the component is first created, we want to GET some JSON from the server and update the state to reflect the latest data. We're going to use jQuery to make an asynchronous request to the server we started earlier to fetch the data we need. The data is already included in the server you started (based on the `comments.json` file), so once it's fetched, `this.state.data` will look something like this: | |
```json | |
[ | |
@@ -428,7 +416,7 @@ var CommentBox = React.createClass({ | |
}); | |
``` | |
-Here, `componentDidMount` is a method called automatically by React when a component is rendered. The key to dynamic updates is the call to `this.setState()`. We replace the old array of comments with the new one from the server and the UI automatically updates itself. Because of this reactivity, it is only a minor change to add live updates. We will use simple polling here but you could easily use WebSockets or other technologies. | |
+Here, `componentDidMount` is a method called automatically by React after a component is rendered for the first time. The key to dynamic updates is the call to `this.setState()`. We replace the old array of comments with the new one from the server and the UI automatically updates itself. Because of this reactivity, it is only a minor change to add live updates. We will use simple polling here but you could easily use WebSockets or other technologies. | |
```javascript{3,15,20-21,35} | |
// tutorial14.js | |
@@ -492,28 +480,91 @@ var CommentForm = React.createClass({ | |
}); | |
``` | |
-Let's make the form interactive. When the user submits the form, we should clear it, submit a request to the server, and refresh the list of comments. To start, let's listen for the form's submit event and clear it. | |
+#### Controlled components | |
-```javascript{3-14,16-19} | |
+With the traditional DOM, `input` elements are rendered and the browser manages the state (its rendered value). As a result, the state of the actual DOM will differ from that of the component. This is not ideal as the state of the view will differ from that of the component. In React, components should always represent the state of the view and not only at the point of initialization. | |
+ | |
+Hence, we will be using `this.state` to save the user's input as it is entered. We define an initial `state` with two properties `author` and `text` and set them to be empty strings. In our `<input>` elements, we set the `value` prop to reflect the `state` of the component and attach `onChange` handlers to them. These `<input>` elements with a `value` set are called controlled components. Read more about controlled components on the [Forms article](/react/docs/forms.html#controlled-components). | |
+ | |
+```javascript{3-11,15-26} | |
// tutorial16.js | |
var CommentForm = React.createClass({ | |
+ getInitialState: function() { | |
+ return {author: '', text: ''}; | |
+ }, | |
+ handleAuthorChange: function(e) { | |
+ this.setState({author: e.target.value}); | |
+ }, | |
+ handleTextChange: function(e) { | |
+ this.setState({text: e.target.value}); | |
+ }, | |
+ render: function() { | |
+ return ( | |
+ <form className="commentForm"> | |
+ <input | |
+ type="text" | |
+ placeholder="Your name" | |
+ value={this.state.author} | |
+ onChange={this.handleAuthorChange} | |
+ /> | |
+ <input | |
+ type="text" | |
+ placeholder="Say something..." | |
+ value={this.state.text} | |
+ onChange={this.handleTextChange} | |
+ /> | |
+ <input type="submit" value="Post" /> | |
+ </form> | |
+ ); | |
+ } | |
+}); | |
+``` | |
+ | |
+#### Events | |
+ | |
+React attaches event handlers to components using a camelCase naming convention. We attach `onChange` handlers to the two `<input>` elements. Now, as the user enters text into the `<input>` fields, the attached `onChange` callbacks are fired and the `state` of the component is modified. Subsequently, the rendered value of the `input` element will be updated to reflect the current component `state`. | |
+ | |
+#### Submitting the form | |
+ | |
+Let's make the form interactive. When the user submits the form, we should clear it, submit a request to the server, and refresh the list of comments. To start, let's listen for the form's submit event and clear it. | |
+ | |
+```javascript{12-21,24} | |
+// tutorial17.js | |
+var CommentForm = React.createClass({ | |
+ getInitialState: function() { | |
+ return {author: '', text: ''}; | |
+ }, | |
+ handleAuthorChange: function(e) { | |
+ this.setState({author: e.target.value}); | |
+ }, | |
+ handleTextChange: function(e) { | |
+ this.setState({text: e.target.value}); | |
+ }, | |
handleSubmit: function(e) { | |
e.preventDefault(); | |
- var author = this.refs.author.value.trim(); | |
- var text = this.refs.text.value.trim(); | |
+ var author = this.state.author.trim(); | |
+ var text = this.state.text.trim(); | |
if (!text || !author) { | |
return; | |
} | |
// TODO: send request to the server | |
- this.refs.author.value = ''; | |
- this.refs.text.value = ''; | |
- return; | |
+ this.setState({author: '', text: ''}); | |
}, | |
render: function() { | |
return ( | |
<form className="commentForm" onSubmit={this.handleSubmit}> | |
- <input type="text" placeholder="Your name" ref="author" /> | |
- <input type="text" placeholder="Say something..." ref="text" /> | |
+ <input | |
+ type="text" | |
+ placeholder="Your name" | |
+ value={this.state.author} | |
+ onChange={this.handleAuthorChange} | |
+ /> | |
+ <input | |
+ type="text" | |
+ placeholder="Say something..." | |
+ value={this.state.text} | |
+ onChange={this.handleTextChange} | |
+ /> | |
<input type="submit" value="Post" /> | |
</form> | |
); | |
@@ -521,24 +572,18 @@ var CommentForm = React.createClass({ | |
}); | |
``` | |
-##### Events | |
- | |
-React attaches event handlers to components using a camelCase naming convention. We attach an `onSubmit` handler to the form that clears the form fields when the form is submitted with valid input. | |
+We attach an `onSubmit` handler to the form that clears the form fields when the form is submitted with valid input. | |
Call `preventDefault()` on the event to prevent the browser's default action of submitting the form. | |
-##### Refs | |
- | |
-We use the `ref` attribute to assign a name to a child component and `this.refs` to reference the DOM node. | |
- | |
-##### Callbacks as props | |
+#### Callbacks as props | |
When a user submits a comment, we will need to refresh the list of comments to include the new one. It makes sense to do all of this logic in `CommentBox` since `CommentBox` owns the state that represents the list of comments. | |
We need to pass data from the child component back up to its parent. We do this in our parent's `render` method by passing a new callback (`handleCommentSubmit`) into the child, binding it to the child's `onCommentSubmit` event. Whenever the event is triggered, the callback will be invoked: | |
```javascript{16-18,31} | |
-// tutorial17.js | |
+// tutorial18.js | |
var CommentBox = React.createClass({ | |
loadCommentsFromServer: function() { | |
$.ajax({ | |
@@ -575,28 +620,45 @@ var CommentBox = React.createClass({ | |
}); | |
``` | |
-Let's call the callback from the `CommentForm` when the user submits the form: | |
+Now that `CommentBox` has made the callback available to `CommentForm` via the `onCommentSubmit` prop, the `CommentForm` can call the callback when the user submits the form: | |
-```javascript{10} | |
-// tutorial18.js | |
+```javascript{19} | |
+// tutorial19.js | |
var CommentForm = React.createClass({ | |
+ getInitialState: function() { | |
+ return {author: '', text: ''}; | |
+ }, | |
+ handleAuthorChange: function(e) { | |
+ this.setState({author: e.target.value}); | |
+ }, | |
+ handleTextChange: function(e) { | |
+ this.setState({text: e.target.value}); | |
+ }, | |
handleSubmit: function(e) { | |
e.preventDefault(); | |
- var author = this.refs.author.value.trim(); | |
- var text = this.refs.text.value.trim(); | |
+ var author = this.state.author.trim(); | |
+ var text = this.state.text.trim(); | |
if (!text || !author) { | |
return; | |
} | |
this.props.onCommentSubmit({author: author, text: text}); | |
- this.refs.author.value = ''; | |
- this.refs.text.value = ''; | |
- return; | |
+ this.setState({author: '', text: ''}); | |
}, | |
render: function() { | |
return ( | |
<form className="commentForm" onSubmit={this.handleSubmit}> | |
- <input type="text" placeholder="Your name" ref="author" /> | |
- <input type="text" placeholder="Say something..." ref="text" /> | |
+ <input | |
+ type="text" | |
+ placeholder="Your name" | |
+ value={this.state.author} | |
+ onChange={this.handleAuthorChange} | |
+ /> | |
+ <input | |
+ type="text" | |
+ placeholder="Say something..." | |
+ value={this.state.text} | |
+ onChange={this.handleTextChange} | |
+ /> | |
<input type="submit" value="Post" /> | |
</form> | |
); | |
@@ -607,7 +669,7 @@ var CommentForm = React.createClass({ | |
Now that the callbacks are in place, all we have to do is submit to the server and refresh the list: | |
```javascript{17-28} | |
-// tutorial19.js | |
+// tutorial20.js | |
var CommentBox = React.createClass({ | |
loadCommentsFromServer: function() { | |
$.ajax({ | |
@@ -659,8 +721,8 @@ var CommentBox = React.createClass({ | |
Our application is now feature complete but it feels slow to have to wait for the request to complete before your comment appears in the list. We can optimistically add this comment to the list to make the app feel faster. | |
-```javascript{17-19} | |
-// tutorial20.js | |
+```javascript{17-23,33} | |
+// tutorial21.js | |
var CommentBox = React.createClass({ | |
loadCommentsFromServer: function() { | |
$.ajax({ | |
@@ -677,6 +739,10 @@ var CommentBox = React.createClass({ | |
}, | |
handleCommentSubmit: function(comment) { | |
var comments = this.state.data; | |
+ // Optimistically set an id on the new comment. It will be replaced by an | |
+ // id generated by the server. In a production application you would likely | |
+ // not use Date.now() for this and would have a more robust system in place. | |
+ comment.id = Date.now(); | |
var newComments = comments.concat([comment]); | |
this.setState({data: newComments}); | |
$.ajax({ | |
@@ -688,6 +754,7 @@ var CommentBox = React.createClass({ | |
this.setState({data: data}); | |
}.bind(this), | |
error: function(xhr, status, err) { | |
+ this.setState({data: comments}); | |
console.error(this.props.url, status, err.toString()); | |
}.bind(this) | |
}); | |
diff --git a/docs/docs/videos.ko-KR.md b/docs/docs/videos.ko-KR.md | |
index de9b460..b2270f6 100644 | |
--- a/docs/docs/videos.ko-KR.md | |
+++ b/docs/docs/videos.ko-KR.md | |
@@ -169,6 +169,6 @@ Server-side rendering을 위해 [SoundCloud](https://developers.soundcloud.com/b | |
### Introducing React Native (+Playlist) - React.js Conf 2015 | |
-<iframe width="650" height="366" src="https://www.youtube-nocookie.com/watch?v=KVZ-P-ZI6W4&index=1&list=PLb0IAmt7-GS1cbw4qonlQztYV1TAW0sCr" frameborder="0" allowfullscreen></iframe> | |
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/v/KVZ-P-ZI6W4&index=1&list=PLb0IAmt7-GS1cbw4qonlQztYV1TAW0sCr" frameborder="0" allowfullscreen></iframe> | |
2015년에 [Tom Occhino](https://twitter.com/tomocchino)님이 React의 과거와 현재를 리뷰하고 나아갈 방향을 제시했습니다. | |
diff --git a/docs/docs/videos.md b/docs/docs/videos.md | |
index b656f78..89f69b5 100644 | |
--- a/docs/docs/videos.md | |
+++ b/docs/docs/videos.md | |
@@ -29,7 +29,7 @@ A [tagtree.tv](http://tagtree.tv/) video conveying the principles of [Thinking i | |
* * * | |
-### Going big with React ### | |
+### Going big with React | |
"On paper, all those JS frameworks look promising: clean implementations, quick code design, flawless execution. But what happens when you stress test Javascript? What happens when you throw 6 megabytes of code at it? In this talk, we'll investigate how React performs in a high stress situation, and how it has helped our team build safe code on a massive scale." | |
<figure>[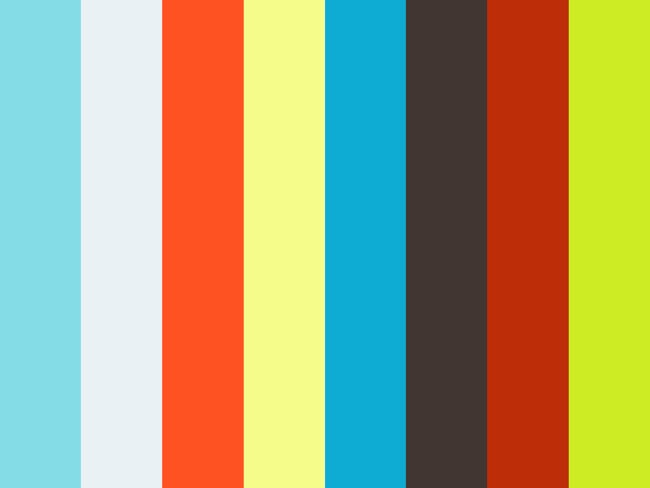](https://skillsmatter.com/skillscasts/5429-going-big-with-react#video)</figure> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment