Created
November 29, 2021 00:50
-
-
Save asehmi/35db009466a010bd1c89d5346a71aea2 to your computer and use it in GitHub Desktop.
Using pandas profiling with streamlit_pandas_profiling component in Streamlit
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import streamlit as st | |
import pandas_profiling | |
from streamlit_pandas_profiling import st_profile_report | |
import pandas as pd | |
st.set_page_config( | |
page_title='Data Profiler', | |
layout='wide', | |
page_icon='🔍' | |
) | |
state = st.session_state | |
if 'profile_report' not in state: | |
state['profile_report'] = None | |
def generate_profile_report(data_file, delimiter, minimal): | |
file_info = {"Filename": data_file.name, "FileType": data_file.type, "FileSize": data_file.size} | |
st.write(file_info) | |
df = pd.read_csv(data_file, sep=delimiter, engine="python") | |
pr = df.profile_report(lazy=True, minimal=minimal) | |
state.profile_report = {'data': df, 'pr': pr} | |
def file_handler(): | |
seperators = {" ": " ", "pipe (|)": "|", r"tab (\t)": "\t", "comma (,)":",", "semicolon (;)":";"} | |
file_upload_form = st.form(key="file_upload") | |
with file_upload_form: | |
data_file = st.file_uploader("Upload CSV File", type=['csv'], key="upload") | |
delimiter = seperators[st.selectbox("Select delimiter", seperators.keys(), key="delims")] | |
minimal = st.checkbox('Minimal report', value=True) | |
if file_upload_form.form_submit_button(label='Submit') and data_file: | |
generate_profile_report(data_file, delimiter, minimal) | |
if (data_file and delimiter and state.profile_report): | |
data = st.session_state.profile_report['data'] | |
pr = st.session_state.profile_report['pr'] | |
col = st.selectbox("Select column for summary stats", options=['']+list(data.columns)) | |
if col != '': | |
st.write(pd.DataFrame(data[col].unique(), columns=[col]).T) | |
st_profile_report(pr) | |
else: | |
st.info('Please upload a CSV data file, select a delimiter and hit submit.') | |
state.profile_report = None | |
if __name__ =="__main__": | |
file_handler() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Demo
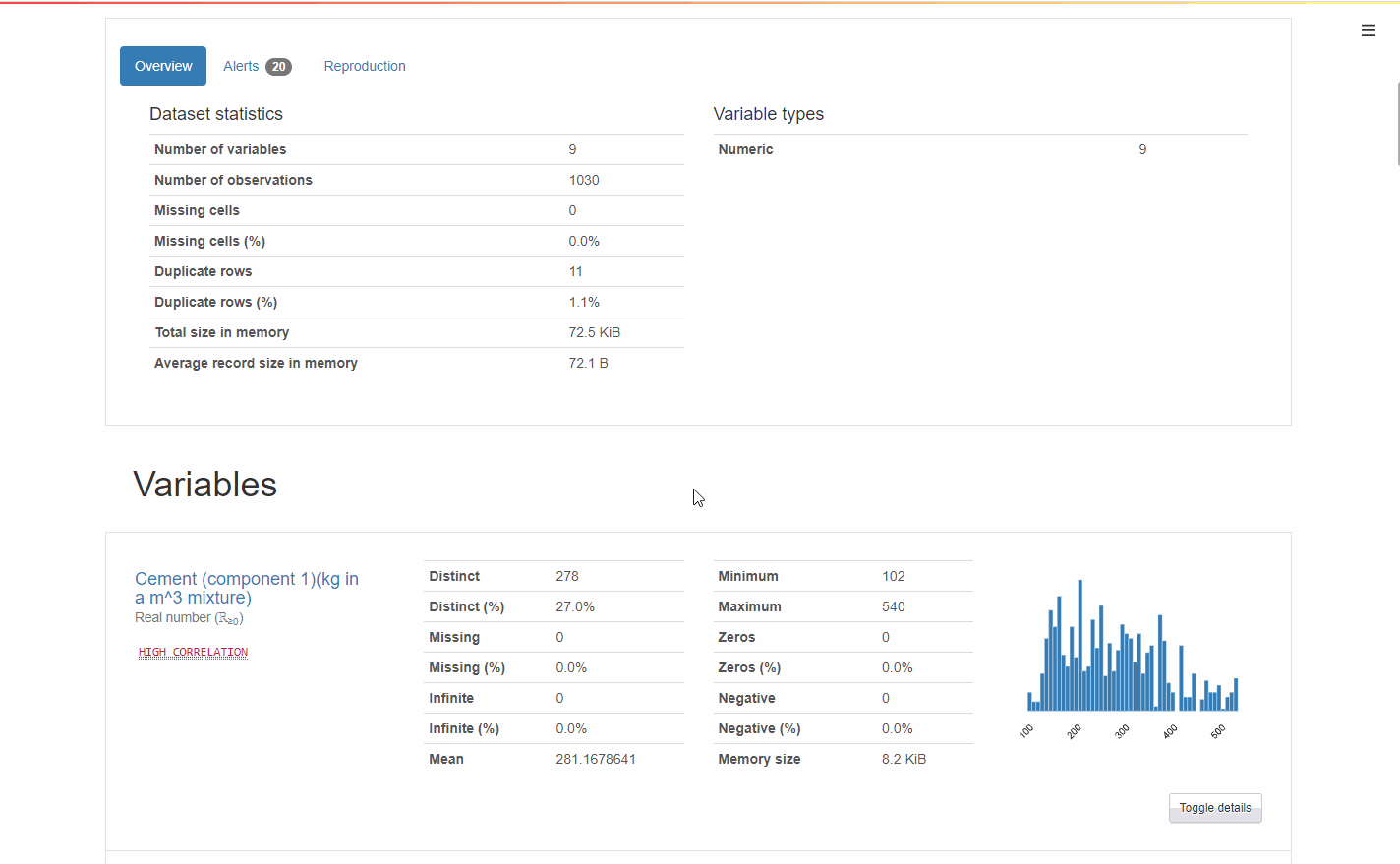