Last active
May 1, 2020 15:59
-
-
Save asolntsev/bf4b0ac648c50ed6dfdaf334a6f36912 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import com.codeborne.selenide.SelenideElement; | |
import org.junit.jupiter.api.Test; | |
import static com.codeborne.selenide.CollectionCondition.size; | |
import static com.codeborne.selenide.Selectors.byText; | |
import static com.codeborne.selenide.Selenide.$; | |
import static com.codeborne.selenide.Selenide.$$; | |
public class PageObjectWithoutContainersTest { | |
@Test | |
void containersUsage() { | |
HistoryPage page = new HistoryPage(); | |
page.shouldHaveRecords(8); | |
page.record(1).status().shouldHave(text("Booked")) | |
page.showMore(); | |
page.shouldHaveRecords(42); | |
page.record(41).status().shouldHave(text("Overbooked")) | |
} | |
static class HistoryPage { | |
public void showMore() { | |
$(byText("SHOW MORE")).click(); | |
} | |
public void shouldHaveRecords(int expectedRecordsCount) { | |
$$("div[id^='bookingRef-']").shouldHave(size(expectedRecordsCount)); | |
} | |
public BookingRecord record(int index) { | |
return new BookingRecord($("div[id^='bookingRef-']", index)); | |
} | |
} | |
static class BookingRecord { | |
private final SelenideElement container; | |
public BookingRecord(SelenideElement container) { | |
this.container = container; | |
} | |
public SelenideElement pickupTime() { | |
return container.$("div[id^='bookingPickupTime-']"); | |
} | |
public SelenideElement status() { | |
return container.$("span[id^='bookingStatusTag-'] > span"); | |
} | |
public void openRecord() { | |
container.$("img[id^='bookingId-']").click(); | |
} | |
} | |
} |
Author
asolntsev
commented
Apr 22, 2020
via email
•
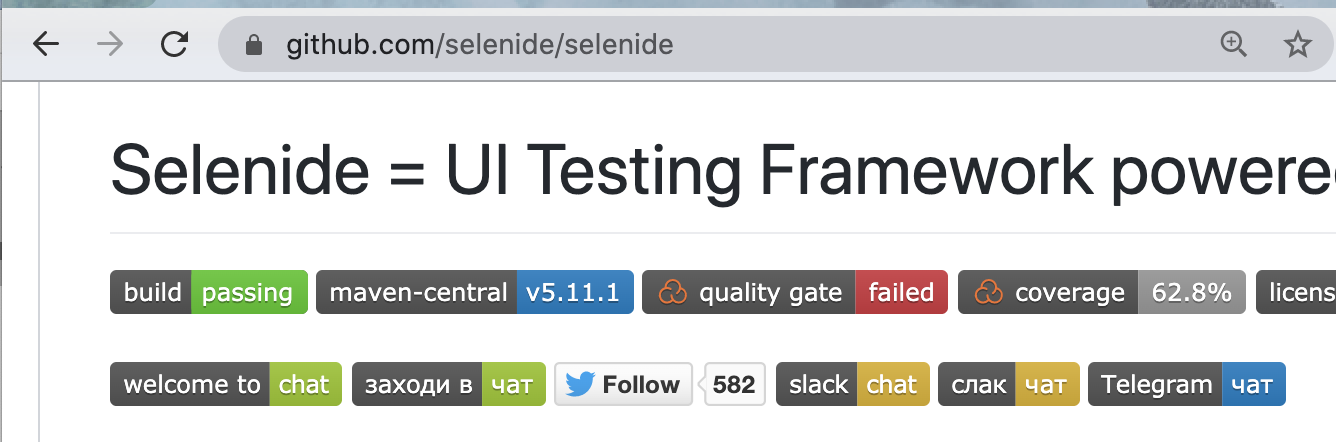
Hi @ansonliao!
You can find links to severals chats on Selenide github page:
[image: image.png]
Andrei Solntsev
сб, 18 апр. 2020 г. в 13:18, ansonliao <notifications@github.com>:
… ***@***.**** commented on this gist.
------------------------------
@asolntsev <https://github.com/asolntsev>, @vinogradoff
<https://github.com/vinogradoff>
or could you provide some contact information so that I can consult you
more convenient? telegram, skype, slack of Selenide, Gitter for Selenide
project, or others.
Thanks.
—
You are receiving this because you were mentioned.
Reply to this email directly, view it on GitHub
<https://gist.github.com/bf4b0ac648c50ed6dfdaf334a6f36912#gistcomment-3259308>,
or unsubscribe
<https://github.com/notifications/unsubscribe-auth/AACEJXMASBFL3LI3RYRLGLLRNF5AHANCNFSM4MI57M2A>
.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment