-
-
Save asus4/09b1f5403c63ceab5ae34710cbe2809e to your computer and use it in GitHub Desktop.
#!/usr/bin/env python | |
# coding: UTF-8 | |
import cv2 | |
import numpy as np | |
def make_lut256x16(exportPath): | |
''' 256 x 16 LUT ''' | |
colors = [] | |
for y in range(0, 16): | |
rows = [] | |
for x in range(0, 256): | |
rows.append([ | |
(x / 16) * 16, # blue | |
y * 16, # green | |
(x % 16) * 16 # red | |
]) | |
colors.append(rows) | |
image = np.array(colors) | |
if exportPath: | |
cv2.imwrite(exportPath, image) | |
return image | |
def make_lut1024x32(exportPath): | |
''' 1024 x 32 LUT ''' | |
colors = [] | |
for y in range(0, 32): | |
rows = [] | |
for x in range(0, 1024): | |
rows.append([ | |
(x / 32) * 8, # blue | |
y * 8, # green | |
(x % 32) * 8 # red | |
]) | |
colors.append(rows) | |
image = np.array(colors) | |
if exportPath: | |
cv2.imwrite(exportPath, image) | |
return image | |
def make_lut512x512(exportPath): | |
''' 512 x 512 Basic LUT ''' | |
colors = [] | |
for y in range(0, 512): | |
rows = [] | |
for x in range(0, 512): | |
i = (x % 64, y % 64) | |
rows.append([ # BGR | |
(y / 2 + x / 16), # blue | |
i[1] * 4, # green | |
i[0] * 4 # red | |
]) | |
colors.append(rows) | |
image = np.array(colors) | |
if exportPath: | |
cv2.imwrite(exportPath, image) | |
return image | |
if __name__ == '__main__': | |
import argparse | |
import sys | |
parser = argparse.ArgumentParser(description='LUT Texture maker') | |
parser.add_argument('path', | |
type=str, | |
nargs='?', | |
default='lut.png', | |
help='output filename') | |
parser.add_argument('-s', '--size', | |
type=str, | |
nargs='?', | |
default='512x512', | |
help='256x16 or 1024x32 or 512x512') | |
args = parser.parse_args() | |
if args.size == '512x512': | |
make_lut512x512(args.path) | |
elif args.size == '256x16': | |
make_lut256x16(args.path) | |
elif args.size == '1024x32': | |
make_lut1024x32(args.path) | |
else: | |
sys.exit('Unsupported size') |
The colors do not go all the way to 255 (only 240 in case of 256x16), which will lead to slightly desaturated images.
Here is a proposed workaround, which will work with both 256x16, 1024x32 and also 4096x64 - though not 64 arranged in a 512x512:
samples = 16 # int, 16, 32 or 64
s = float(samples)
mult = 255.0 / (s - 1)
colors = []
for y in range(0, samples):
rows = []
for x in range(0, samples * samples):
rows.append([
int(round((x / samples) * mult)), # blue
int(round(y * mult)), # green
int(round((x % samples) * mult)) # red
])
colors.append(rows)
./lut_maker.py lut.png
./lut_maker.py lut512x512.png --size 512x512
./lut_maker.py lut256x16.png --size 256x16
./lut_maker.py lut1024x32.png --size 1024x32
#!/usr/bin/env python
coding: UTF-8
import cv2
import numpy as np
def make_lut256x16(exportPath):
''' 256 x 16 LUT '''
colors = []
for y in range(0, 16):
rows = []
for x in range(0, 256):
rows.append([
(x / 16) * 16, # blue
y * 16, # green
(x % 16) * 16 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
def make_lut1024x32(exportPath):
''' 1024 x 32 LUT '''
colors = []
for y in range(0, 32):
rows = []
for x in range(0, 1024):
rows.append([
(x / 32) * 8, # blue
y * 8, # green
(x % 32) * 8 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
def make_lut512x512(exportPath):
''' 4096 x 4096 Basic LUT '''
colors = []
for y in range(0, 4096):
rows = []
for x in range(0, 4096):
i = (x % 64, y % 64)
rows.append([ # BGR
(y / 2 + x / 16), # blue
i[1] * 4, # green
i[0] * 4 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
if name == 'main':
import argparse
import sys
parser = argparse.ArgumentParser(description='LUT Texture maker')
parser.add_argument('path',
type=str,
nargs='?',
default='lut.png',
help='output filename')
parser.add_argument('-s', '--size',
type=str,
nargs='?',
default='512x512',
help='256x16 or 1024x32 or 512x512')
args = parser.parse_args()
if args.size == '4096x4096':
make_lut4096x4096(args.path)
elif args.size == '256x16':
make_lut256x16(args.path)
elif args.size == '1024x32':
make_lut1024x32(args.path)
else:
sys.exit('Unsupported size')
#!/usr/bin/env python
coding: UTF-8
import cv2
import numpy as np
def make_lut256x16(exportPath):
''' 256 x 16 LUT '''
colors = []
for y in range(0, 16):
rows = []
for x in range(0, 256):
rows.append([
(x / 16) * 16, # blue
y * 16, # green
(x % 16) * 16 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
def make_lut1024x32(exportPath):
''' 1024 x 32 LUT '''
colors = []
for y in range(0, 32):
rows = []
for x in range(0, 1024):
rows.append([
(x / 32) * 8, # blue
y * 8, # green
(x % 32) * 8 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
def make_lut512x512(exportPath):
''' 512 x 512 Basic LUT '''
colors = []
for y in range(0, 512):
rows = []
for x in range(0, 512):
i = (x % 64, y % 64)
rows.append([ # BGR
(y / 2 + x / 16), # blue
i[1] * 4, # green
i[0] * 4 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
if name == 'main':
import argparse
import sys
parser = argparse.ArgumentParser(description='LUT Texture maker')
parser.add_argument('path',
type=str,
nargs='?',
default='lut.png',
help='output filename')
parser.add_argument('-s', '--size',
type=str,
nargs='?',
default='512x512',
help='256x16 or 1024x32 or 512x512')
args = parser.parse_args()
if args.size == '512x512':
make_lut512x512(args.path)
elif args.size == '256x16':
make_lut256x16(args.path)
elif args.size == '1024x32':
make_lut1024x32(args.path)
else:
sys.exit('Unsupported size')
samples = 16 # int, 16, 32 or 64
s = float(samples)
mult = 255.0 / (s - 1)
colors = []
for y in range(0, samples):
rows = []
for x in range(0, samples * samples):
rows.append([
int(round((x / samples) * mult)), # blue
int(round(y * mult)), # green
int(round((x % samples) * mult)) # red
])
colors.append(rows)
!/usr/bin/env python
coding: UTF-8
import cv2
import numpy as np
def make_lut256x16(exportPath):
''' 256 x 16 LUT '''
colors = []
for y in range(0, 16):
rows = []
for x in range(0, 256):
rows.append([
(x / 16) * 16, # blue
y * 16, # green
(x % 16) * 16 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
def make_lut1024x32(exportPath):
''' 1024 x 32 LUT '''
colors = []
for y in range(0, 32):
rows = []
for x in range(0, 1024):
rows.append([
(x / 32) * 8, # blue
y * 8, # green
(x % 32) * 8 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
def make_lut512x512(exportPath):
''' 512 x 512 Basic LUT '''
colors = []
for y in range(0, 512):
rows = []
for x in range(0, 512):
i = (x % 64, y % 64)
rows.append([ # BGR
(y / 2 + x / 16), # blue
i[1] * 4, # green
i[0] * 4 # red
])
colors.append(rows)
image = np.array(colors)
if exportPath:
cv2.imwrite(exportPath, image)
return image
if name == 'main':
import argparse
import sys
parser = argparse.ArgumentParser(description='LUT Texture maker')
parser.add_argument('path',
type=str,
nargs='?',
default='lut.png',
help='output filename')
parser.add_argument('-s', '--size',
type=str,
nargs='?',
default='512x512',
help='256x16 or 1024x32 or 512x512')
args = parser.parse_args()
if args.size == '512x512':
make_lut512x512(args.path)
elif args.size == '256x16':
make_lut256x16(args.path)
elif args.size == '1024x32':
make_lut1024x32(args.path)
else:
sys.exit('Unsupported size')
requirements.txt
cv2
numpy
USAGE
Output
LUT 256x16.png

LUT 1024x32.png

LUT 512x512
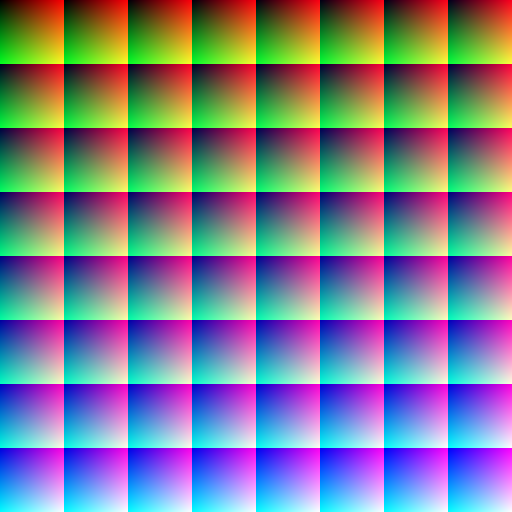