Last active
January 8, 2022 09:07
-
-
Save atetlaw/c8dec1d9842750f2136af1844fc88abb to your computer and use it in GitHub Desktop.
A simple pure SwiftUI card-style action sheet, that also blurs the background content, with animated transition
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// | |
// CardSheet.swift | |
// | |
// Created by Andrew Tetlaw on 4/12/21. | |
// | |
import SwiftUI | |
struct CardSheet<SheetContent: View>: ViewModifier { | |
@Binding var show: Bool | |
@ViewBuilder let sheetContent: () -> SheetContent | |
func body(content: Content) -> some View { | |
ZStack(alignment: .bottom) { | |
content | |
if show { | |
ContainerRelativeShape() | |
.ignoresSafeArea() | |
.background(.thinMaterial) | |
.onTapGesture { | |
withCardSheetAnimation { | |
show.toggle() | |
} | |
} | |
sheetContent() | |
.transition(.opacity.combined(with: .move(edge: .bottom))) | |
.zIndex(1) | |
} | |
} | |
} | |
} | |
extension View { | |
func cardSheet<SheetContent: View>(show: Binding<Bool>, sheetContent: @escaping () -> SheetContent) -> some View { | |
modifier(CardSheet(show: show, sheetContent: sheetContent)) | |
} | |
} | |
extension Animation { | |
static var defaultCardSheetAnimation: Animation { | |
Animation.easeOut(duration: 0.18) | |
} | |
} | |
func withCardSheetAnimation<Result>(_ body: () throws -> Result) rethrows -> Result { | |
try withAnimation(.defaultCardSheetAnimation, body) | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
In your View:
You need to set the
show
binding variable using animation, so it slides up from the bottom, and is dismissed by sliding down:Show:
Hide:
It's automatically dismissed by tapping the background.
And it looks like this:
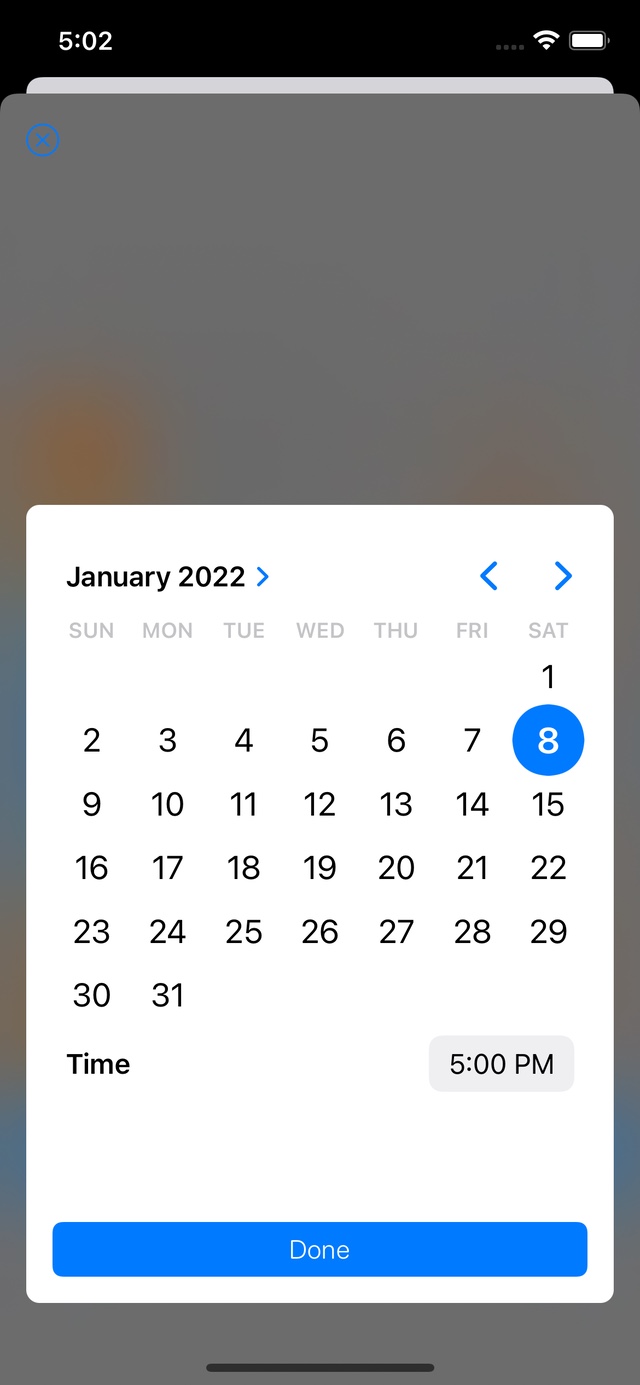
No need to dive into UIKit and do crazy things...