Last active
May 3, 2020 02:26
-
-
Save axelerator/0fc300493b0de188bbffb3f462ba44b7 to your computer and use it in GitHub Desktop.
animation_navigation.elm
The problem seems to be related to the issue @mdgriffith mentions in one of his examples:
https://github.com/mdgriffith/elm-animator/blob/master/examples/Pages.elm#L134
I added the fix as a comment in line 116
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
After the first
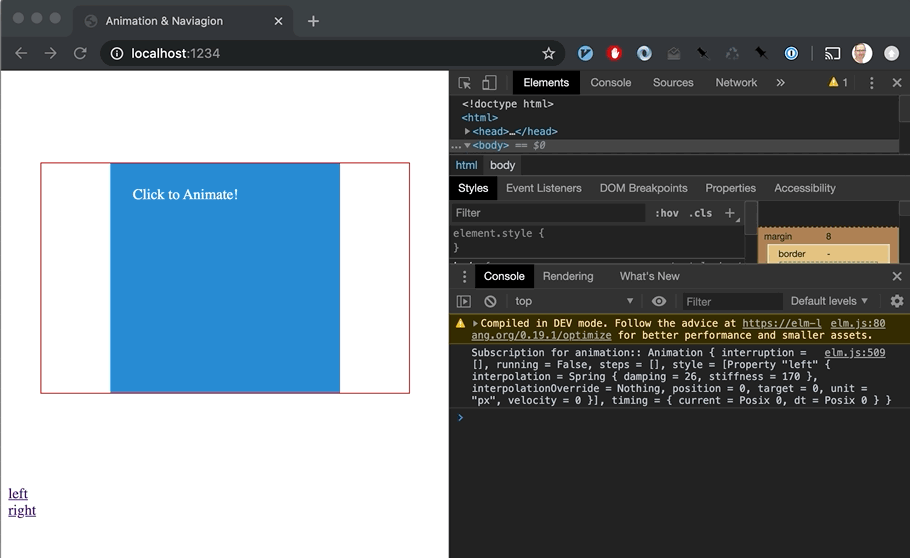
UrlChanged
a newstyle
gets applied to the model. But the subscription does not trigger newAnimate
messages to update the position. However if I click the link again the animation gets kicked off.What am I missing?