Created
January 13, 2018 01:52
-
-
Save beardordie/40f9e7ed4bd69c6a4a1a239ad0bb1ff9 to your computer and use it in GitHub Desktop.
Unity script component to show debug text on your GameObjects when you need to see it in Game view, not just Scene view.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
/// <summary>DebugTextGameView class | |
/// AUTHOR: Beard or Die | |
/// DATE: 2018-01-12 | |
/// REQUIREMENTS: Add this script onto a GameObject that you want some debug text to | |
/// appear over in Game View. Set up in inspector. You will also need to create | |
/// an empty GameObject, add a TextMesh component, and drag that GameObject into | |
/// Project folder to create a prefab from it. Use that prefab in this script's | |
/// inspector for "Debug Text Prefab". This will allow you to change the style/size | |
/// of your debug text just once, rather than having to set that for every usage. | |
/// INSTRUCTIONS: If you want to specify custom text, choose Override style and | |
/// call the public OverrideText method. Or choose GameObjectToString and override that. | |
/// Example: public override string ToString(){ return "Some custom string"; } | |
/// WARNING: This is for debug purposes, not for production. It's too ugly to use | |
/// for real game usage. | |
/// LICENSE: CC0; change and use this however you like | |
/// </summary> | |
public class DebugTextGameView : MonoBehaviour { | |
private bool isDrawn = false; | |
private string overrideText = "OverrideText method not yet called. See instructions."; | |
private Transform txtMeshTransform; | |
private GameObject txtMeshObject; | |
private TextMesh txtMesh; | |
private float destroyTime; | |
// INSPECTOR SETTINGS | |
[Tooltip("If true, set visibleOnlyWhileKeyHeld to false")] [SerializeField] private bool isVisibleOnAwake = true; | |
[Tooltip("If true, specify heldKey and set isVisibleOnAwake to false")] [SerializeField] private bool visibleOnlyWhileKeyHeld = false; | |
[SerializeField] private Transform debugTextPrefab; // this prefab requires a TextMesh component attached on a child gameobject | |
[SerializeField] private Vector3 textOffset = Vector3.up; | |
[SerializeField] private Color color = Color.green; | |
[Tooltip("In seconds; If 0, never auto-destroy")] [SerializeField] private float autoDestroyTime = 0f; | |
[Tooltip("If visibleOnlyWhileKeyHeld is true, set this key")] [SerializeField] private KeyCode heldKey = KeyCode.LeftShift; | |
[HideInInspector] public enum DebugDataTypes {TransformPosition, GameObjectName, GameObjectToString, Override} | |
[Tooltip("Choose type of data to show")] [SerializeField] private DebugDataTypes useData = DebugDataTypes.TransformPosition; | |
// PRIVATE METHODS | |
private void Awake() | |
{ | |
Setup(); | |
} | |
private void Setup() | |
{ | |
if(txtMeshObject==null) | |
{ | |
isDrawn=false; | |
} | |
if(!isDrawn && txtMeshTransform==null) | |
{ | |
isDrawn=true; | |
txtMeshTransform = (Transform)Instantiate(debugTextPrefab); | |
txtMeshTransform.position = Vector3.zero; // reset the prefab's position | |
txtMeshObject = txtMeshTransform.gameObject; | |
if(!isVisibleOnAwake) txtMeshObject.SetActive(false); | |
txtMesh = txtMeshTransform.GetComponentInChildren<TextMesh>(); | |
txtMesh.color = color; | |
if(autoDestroyTime>0) | |
{ | |
destroyTime = Time.time + autoDestroyTime; | |
StartCoroutine(AutoDestroy()); | |
} | |
} | |
if(isDrawn) | |
{ | |
SetPosition(); | |
UpdateDebugText(); | |
} | |
} | |
private void SetPosition() | |
{ | |
txtMeshTransform.position = gameObject.transform.position + textOffset; | |
} | |
private void UpdateDebugText() | |
{ | |
if(txtMesh==null || txtMeshObject==null) | |
{ | |
return; // prevent null reference exceptions | |
} | |
SetPosition(); | |
// Set text for debugging | |
switch (useData){ | |
case DebugDataTypes.GameObjectName: | |
txtMesh.text = gameObject.name; | |
break; | |
case DebugDataTypes.GameObjectToString: | |
txtMesh.text = gameObject.ToString(); | |
break; | |
case DebugDataTypes.TransformPosition: | |
txtMesh.text = gameObject.transform.position.ToString(); | |
break; | |
case DebugDataTypes.Override: | |
if(overrideText!=null) | |
{ | |
txtMesh.text = overrideText; | |
} else | |
{ | |
txtMesh.text = "OverrideText not yet called. See instructions."; // to prevent this, see instructions | |
} | |
break; | |
default: | |
txtMesh.text = gameObject.ToString(); | |
break; | |
} | |
if(visibleOnlyWhileKeyHeld) | |
{ | |
if(Input.GetKey(heldKey)) | |
{ | |
SetPosition(); | |
txtMeshObject.SetActive(true); | |
} else { | |
txtMeshObject.SetActive(false); | |
} | |
} | |
if(txtMeshObject==null) | |
{ | |
isDrawn=false; | |
} | |
} | |
private void Update() | |
{ | |
UpdateDebugText(); | |
} | |
private IEnumerator AutoDestroy() | |
{ | |
if(Time.time >= destroyTime) | |
{ | |
Hide(); | |
Destroy(txtMeshObject,5f); | |
yield break; | |
} else { | |
yield return new WaitForSeconds(0.1f); | |
StartCoroutine(AutoDestroy()); // recursion | |
} | |
} | |
// PUBLIC METHODS | |
public void OverrideText(string newText) | |
{ | |
overrideText = newText; | |
} | |
public void Show() | |
{ | |
if(txtMeshObject!=null) txtMeshObject.SetActive(true); | |
} | |
public void Hide() | |
{ | |
if(txtMeshObject!=null) txtMeshObject.SetActive(false); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Result:
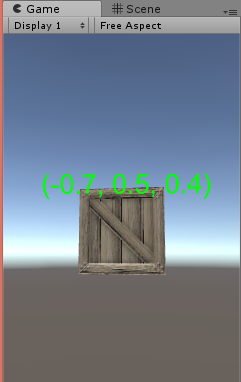