You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Why is looking at runtime not a reliable method of calculating time complexity?
Not all computers are made equal( some may be stronger and therefore boost our runtime speed )
How many background processes ran concurrently with our program that was being tested?
We also need to ask if our code remains performant if we increase the size of the input.
The real question we need to answering is: How does our performance scale?.
big ‘O’ notation
Big O Notation is a tool for describing the efficiency of algorithms with respect to the size of the input arguments.
Since we use mathematical functions in Big-O, there are a few big picture ideas that we’ll want to keep in mind:
The function should be defined by the size of the input.
Smaller Big O is better (lower time complexity)
Big O is used to describe the worst case scenario.
Big O is simplified to show only its most dominant mathematical term.
Simplifying Math Terms
We can use the following rules to simplify the our Big O functions:
Simplify Products : If the function is a product of many terms, we drop the terms that don't depend on n.
Simplify Sums : If the function is a sum of many terms, we drop the non-dominant terms.
n : size of the input
T(f) : unsimplified math function
O(f) : simplified math function.
Putting it all together
- First we apply the product rule to drop all constants.
- Then we apply the sum rule to select the single most dominant term.
Complexity Classes
Common Complexity Classes
There are 7 major classes in Time Complexity
#### `O(1) Constant`
The algorithm takes roughly the same number of steps for any input size.
O(log(n)) Logarithmic
In most cases our hidden base of Logarithmic time is 2, log complexity algorithm’s will typically display ‘halving’ the size of the input (like binary search!)
O(n) Linear
Linear algorithm’s will access each item of the input “once”.
O(nlog(n)) Log Linear Time
Combination of linear and logarithmic behavior, we will see features from both classes.
Algorithm’s that are log-linear will use both recursion AND iteration.
O(nc) Polynomial
C is a fixed constant.
O(c^n) Exponential
C is now the number of recursive calls made in each stack frame.
Algorithm’s with exponential time are VERY SLOW.
Memoization
Memoization : a design pattern used to reduce the overall number of calculations that can occur in algorithms that use recursive strategies to solve.
MZ stores the results of the sub-problems in some other data structure, so that we can avoid duplicate calculations and only ‘solve’ each problem once.
Two features that comprise memoization:
FUNCTION MUST BE RECURSIVE.
Our additional Data Structure is usually an object (we refer to it as our memo… or sometimes cache!)
### Memoizing Factorial
Our memo object is mapping out our arguments of factorial to it’s return value.
Keep in mind we didn’t improve the speed of our algorithm.
Memoizing Fibonacci
- Our time complexity for Fibonacci goes from O(2^n) to O(n) after applying memoization.
The Memoization Formula
Rules:
Write the unoptimized brute force recursion (make sure it works);
Add memo object as an additional argument .
Add a base case condition that returns the stored value if the function’s argument is in the memo.
Before returning the result of the recursive case, store it in the memo as a value and make the function’s argument it’s key.
Things to remember
When solving DP problems with Memoization, it is helpful to draw out the visual tree first.
When you notice duplicate sub-tree’s that means we can memoize.
Tabulation
Tabulation Strategy
Use When:
The function is iterative and not recursive.
The accompanying DS is usually an array.
Steps for tabulation
Create a table array based off the size of the input.
Initialize some values in the table to ‘answer’ the trivially small subproblem.
Iterate through the array and fill in the remaining entries.
Your final answer is usually the last entry in the table.
Memo and Tab Demo with Fibonacci
Normal Recursive Fibonacci
function fibonacci(n) {
if (n <= 2) return 1;
return fibonacci(n - 1) + fibonacci(n - 2);
}
Memoization Fibonacci 1
Memoization Fibonacci 2
Tabulated Fibonacci
Example of Linear Search
Worst Case Scenario: The term does not even exist in the array.
Meaning: If it doesn’t exist then our for loop would run until the end therefore making our time complexity O(n).
Sorting Algorithms
Bubble Sort
Time Complexity: Quadratic O(n^2)
The inner for-loop contributes to O(n), however in a worst case scenario the while loop will need to run n times before bringing all n elements to their final resting spot.
Space Complexity: O(1)
Bubble Sort will always use the same amount of memory regardless of n.
- The first major sorting algorithm one learns in introductory programming courses.
- Gives an intro on how to convert unsorted data into sorted data.
It’s almost never used in production code because:
It’s not efficient
It’s not commonly used
There is stigma attached to it
Bubbling Up* : Term that infers that an item is in motion, moving in some direction, and has some final resting destination.*
Bubble sort, sorts an array of integers by bubbling the largest integer to the top.
Worst Case & Best Case are always the same because it makes nested loops.
Double for loops are polynomial time complexity or more specifically in this case Quadratic (Big O) of: O(n²)
Selection Sort
Time Complexity: Quadratic O(n^2)
Our outer loop will contribute O(n) while the inner loop will contribute O(n / 2) on average. Because our loops are nested we will get O(n²);
Space Complexity: O(1)
Selection Sort will always use the same amount of memory regardless of n.
- Selection sort organizes the smallest elements to the start of the array.
Summary of how Selection Sort should work:
Set MIN to location 0
Search the minimum element in the list.
Swap with value at location Min
Increment Min to point to next element.
Repeat until list is sorted.
Insertion Sort
Time Complexity: Quadratic O(n^2)
Our outer loop will contribute O(n) while the inner loop will contribute O(n / 2) on average. Because our loops are nested we will get O(n²);
Space Complexity: O(n)
Because we are creating a subArray for each element in the original input, our Space Comlexity becomes linear.
### Merge Sort
Time Complexity: Log Linear O(nlog(n))
Since our array gets split in half every single time we contribute O(log(n)). The while loop contained in our helper merge function contributes O(n) therefore our time complexity is O(nlog(n)); Space Complexity: O(n)
We are linear O(n) time because we are creating subArrays.
### Example of Merge Sort
- **Merge sort is O(nlog(n)) time.**
- *We need a function for merging and a function for sorting.*
Steps:
If there is only one element in the list, it is already sorted; return the array.
Otherwise, divide the list recursively into two halves until it can no longer be divided.
Merge the smallest lists into new list in a sorted order.
Quick Sort
Time Complexity: Quadratic O(n^2)
Even though the average time complexity O(nLog(n)), the worst case scenario is always quadratic.
Space Complexity: O(n)
Our space complexity is linear O(n) because of the partition arrays we create.
QS is another Divide and Conquer strategy.
Some key ideas to keep in mind:
It is easy to sort elements of an array relative to a particular target value.
An array of 0 or 1 elements is already trivially sorted.
### Binary Search
Time Complexity: Log Time O(log(n))
Space Complexity: O(1)
*Recursive Solution*
Min Max Solution
Must be conducted on a sorted array.
Binary search is logarithmic time, not exponential b/c n is cut down by two, not growing.
Binary Search is part of Divide and Conquer.
Insertion Sort
Works by building a larger and larger sorted region at the left-most end of the array.
Steps:
If it is the first element, and it is already sorted; return 1.
Pick next element.
Compare with all elements in the sorted sub list
Shift all the elements in the sorted sub list that is greater than the value to be sorted.
Insert the value
Repeat until list is sorted.
If you found this guide helpful feel free to checkout my GitHub/gists where I host similar content:
- way we analyze how efficient algorithms are without getting too mired in details
- can model how much time any function will take given `n` inputs
- interested in order of magnitude of number of the exact figure
- O absorbs all fluff and n = biggest term
- Big O of `3x^2 +x + 1` = `O(n^2)`
recursive: as you add more terms, increase in time as you add input diminishes recursion: when you define something in terms of itself, a function that calls itself
used because of ability to maintain state at diffferent levels of recursion
inherently carries large footprint
every time function called, you add call to stack
iterative: use loops instead of recursion (preferred)
favor readability over performance
O(n log(n)) & O(log(n)): dividing/halving
if code employs recursion/divide-and-conquer strategy
what power do i need to power my base to get n
Time Definitions
constant: does not scale with input, will take same amount of time
for any input size n, constant time performs same number of operations every time
logarithmic: increases number of operations it performs as logarithmic function of input size n
function log n grows very slowly, so as n gets longer, number of operations the algorithm needs to perform doesn’t increase very much
halving
linear: increases number of operations it performs as linear function of input size n
number of additional operations needed to perform grows in direct proportion to increase in input size n
log-linear: increases number of operations it performs as log-linear function of input size n
looking over every element and doing work on each one
quadratic: increases number of operations it performs as quadratic function of input size n
exponential: increases number of operations it performs as exponential function of input size n
number of nested loops increases as function of n
polynomial: as size of input increases, runtime/space used will grow at a faster rate
factorial: as size of input increases, runtime/space used will grow astronomically even with relatively small inputs
rate of growth: how fast a function grows with input size
### Space Complexity
How does the space usage scale/change as input gets very large?
What auxiliary space does your algorithm use or is it in place (constant)?
Runtime stack space counts as part of space complexity unless told otherwise.
I have this innate desire to make everything available all in one place and it’s usually an unnecessary waste of time… but here I will…
All The Things You Can Embed In A Medium Article
I have this innate desire to make everything available all in one place and it’s usually an unnecessary waste of time… but here I will conduct and ‘experiment’ where I intentionally indulge that tendency.
Here you can see in just the first frame of my blog site 5 different embedded widgets that I inject onto nearly every page of the site using javascript to append my widgets to various anchor points in the html.
This topic is meant to give you a very basic overview of how Markdown works, showing only some of the most common operations you use most frequently. Keep in mind that you can also use the Edit menus to inject markdown using the toolbar, which serves as a great way to see how Markdown works. However, Markdown’s greatest strength lies in its simplicity and keyboard friendly approach that lets you focus on writing your text and staying on the keyboard.
What is Markdown
Markdown is very easy to learn and get comfortable with due it’s relatively small set of markup ‘commands’. It uses already familiar syntax to represent common formatting operations. Markdown understands basic line breaks so you can generally just type text.
Markdown also allows for raw HTML inside of a markdown document, so if you want to embed something more fancy than what Markdowns syntax can do you can always fall back to HTML. However to keep documents readable that’s generally not recommended.
Basic Markdown Syntax
The following are a few examples of the most common things you are likely to do with Markdown while building typical documentation.
Bold and Italic
markdown
This text **is bold**.
This text *is italic*.
By default Markdown adds paragraphs at double line breaks. Single line breaks by themselves are simply wrapped together into a single line. If you want to have soft returns that break a single line, add two spaces at the end of the line.
markdown
This line has a paragraph break at the end (empty line after).
Theses two lines should display as a single
line because there's no double space at the end.
The following line has a soft break at the end (two spaces at end)
This line should be following on the very next line.
This line has a paragraph break at the end (empty line after).
Theses two lines should display as a single line because there’s no double space at the end.
The following line has a soft break at the end (two spaces at end)
This line should be following on the very next line.
Links
markdown
[Help Builder Web Site](http://helpbuilder.west-wind.com/)
If you need additional image tags like targets or title attributes you can also embed HTML directly:
markdown
Go the Help Builder sitest Wind site: <a href="http://west-wind.com/" target="_blank">Help Builder Site</a>.
Images
markdown
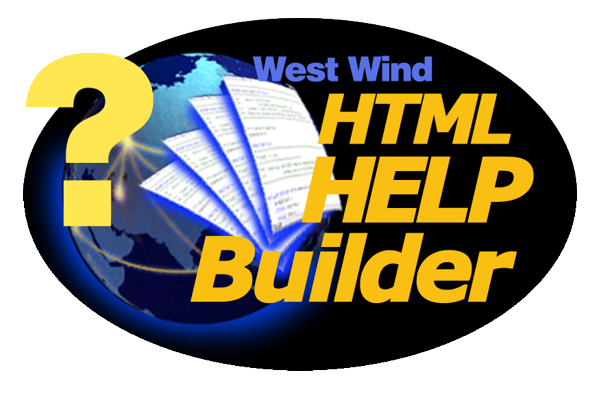
### Block Quotes
Block quotes are callouts that are great for adding notes or warnings into documentation.
markdown
> ### Headers break on their own
> Note that headers don't need line continuation characters
as they are block elements and automatically break. Only text
lines require the double spaces for single line breaks.
Headers break on their own
Note that headers don’t need line continuation characters as they are block elements and automatically break. Only text lines require the double spaces for single line breaks.
Fontawesome Icons
Help Builder includes a custom syntax for FontAwesome icons in its templates. You can embed a @ icon- followed by a font-awesome icon name to automatically embed that icon without full HTML syntax.
markdown
Gear: Configuration
Configuration
HTML Markup
You can also embed plain HTML markup into the page if you like. For example, if you want full control over fontawesome icons you can use this:
markdown
This text can be **embedded** into Markdown:
<i class="fa fa-refresh fa-spin fa-lg"></i> Refresh Page
This text can be embedded into Markdown:
Refresh Page
Unordered Lists
markdown
* Item 1
* Item 2
* Item 3
This text is part of the third item. Use two spaces at end of the the list item to break the line.
A double line break, breaks out of the list.
Item 1
Item 2
Item 3
This text is part of the third item. Use two spaces at end of the the list item to break the line.
A double line break, breaks out of the list.
Ordered Lists
markdown
1. **Item 1**
Item 1 is really something
2. **Item 2**
Item two is really something else
If you want lines to break using soft returns use two spaces at the end of a line.
Item 1 Item 1 is really something
Item 2
Item two is really something else
If you want to lines to break using soft returns use to spaces at the end of a line.
Inline Code
If you want to embed code in the middle of a paragraph of text to highlight a coding syntax or class/member name you can use inline code syntax:
markdown
Structured statements like `for x =1 to 10` loop structures
can be codified using single back ticks.
Structured statements like for x =1 to 10 loop structures can be codified using single back ticks.
Code Blocks with Syntax Highlighting
Markdown supports code blocks syntax in a variety of ways:
markdown
The following code demonstrates:
// This is code by way of four leading spaces
// or a leading tab
More text here
The following code demonstrates:
pgsql
// This is code by way of four leading spaces
// or a leading tab
More text here
Code Blocks
You can also use triple back ticks plus an optional coding language to support for syntax highlighting (space injected before last ` to avoid markdown parsing):
markdown
`` `csharp
// this code will be syntax highlighted
for(var i=0; i++; i < 10)
{
Console.WriteLine(i);
}
`` `
csharp
// this code will be syntax highlighted
for(var i=0; i++; i < 10)
{
Console.WriteLine(i);
}
Many languages are supported: html, xml, javascript, css, csharp, foxpro, vbnet, sql, python, ruby, php and many more. Use the Code drop down list to get a list of available languages.
You can also leave out the language to get no syntax coloring but the code box:
Function that takes in a value and two callbacks. The function should return the result of the callback who’s invocation results in a larger value.
function greaterValue(value, cb1, cb2) {
// compare cb1 invoked with value to cb2 invoked with value
// return the greater result
let res1 = cb1(value);
let res2 = cb2(value);
if (res1 > res2) {
// if this is false, we move out of if statement
return res1;
}
return res2;
}
let negate = function(num) {
return num * -1;
};
let addOne = function(num) {
return num + 1;
};
console.log(greaterValue(3, negate, addOne));
console.log(greaterValue(-2, negate, addOne));
Note: we do not invokenegateoraddOne(by using()to call them), we are passing the function itself.
Write a function, myMap, that takes in an array and a callback as arguments. The function should mimic the behavior of Array.prototype.map.
function myMap(arr, callback) {
// iterate through the array, perform the cb on each element
// return a new array with those new values
let mapped = [];
for (let i = 0; i < arr.length; i++) {
// remember that map passes three args with each element.
let val = callback(arr[i], i, arr);
mapped.push(val);
}
return mapped;
}
let double = function(num) {
return num * 2;
};
console.log(myMap([1, 2, 3], double));
Write a function, myFilter, that takes in an array and a callback as arguments. The function should mimic the behavior of Array.prototype.filter.
function myFilter(arr, callback) {
let filtered = [];
for (let i = 0; i < arr.length; i++) {
let element = arr[i];
if (callback(element, i, arr)) {
filtered.push(element);
}
}
return filtered;
}
Write a function, myEvery, that takes in an array and a callback as arguments. The function should mimic the behavior of Array.prototype.every.
function myEvery(arr, callback) {
for (let i = 0; i < arr.length; i++) {
let element = arr[i];
if (callback(element, i, arr) === false) {
return false;
}
}
return true;
}
Further Examples of the above concepts
const createMeowValue = () => {
console.log(this.name);
let meow = function () {
console.log(this);
console.log(this.name + ' meows');
}
meow = meow.bind(this);
return meow;
};
const name = 'Fluffy';
const cat = {
name: name,
age: 12,
createMeow: function () {
console.log(this.name);
let meow = () => {
const hello = 'hello';
console.log(this.name + ' meows');
};
let world = '';
if (true) {
world = 'world';
}
console.log(world);
// meow = meow.bind(this);
return meow;
}
};
cat.newKey = function () {
const outermostContext = this;
const innerFunc = () => {
secondContext = this;
console.log(secondContext === outermostContext)
return function () {
innermostContext = this;
}
};
return innerFunc.bind(outermostContext);
};
const meow = cat.createMeow(); // method-style invocation
meow(); // function-style invocation
console.log('-------')
const createMeow = cat.createMeow;
const globalMeow = createMeow(); // function-style
globalMeow(); // function-style
function createSmoothie(ingredient) {
const ingredients = [ingredient];
return ingredients;
}
// console.log(createSmoothie('banana'));
// console.log(createSmoothie('apple'));
// one parameter only
// first argument is a string
// return an array
// DO NOT USE forEach
Quantum Computing — Computing which utilizes quantum mechanics and qubits on quantum computers.
Theoretical Computer Science — The interplay of computer science and pure mathematics, distinguished by its emphasis on mathematical rigour and technique.
This is going to be a running list of youtube videos and channels that I discover as I learn web development. It will not be strictly…
Awesome Web Development Youtube Video Archive
This is going to be a running list of youtube videos and channels that I discover as I learn web development. It will not be strictly confined to web development but that will be it’s focus.
Tutorials:
Inspiration:
Channels:
Free Code Camp:
Our mission: to help people learn to code for free. We accomplish this by creating thousands of videos, articles, and interactive coding lessons — all freely available to the public. We also have thousands of freeCodeCamp study groups around the world.
Chris Coyier
This is the official YouTube channel for CSS-Tricks, a web design community curated by Chris Coyier. https://css-tricks.com
Computer History Museum:
Welcome to the Computer History Museum channel on YouTube. We’re committed to preserving and presenting the history and stories of the Information Age. Here on YouTube we offer videos of the many lectures and events at the museum and also historic computer films. We also feature video from our well–known Revolutionaries television series. Be sure to check out the Computer History Museum website for even more information including online exhibits, upcoming events and our collection of computing artifacts: http://www.computerhistory.org.
Computerphile
Videos all about computers and computer stuff. Sister channel of Numberphile.
Hello! My name is David Xiang. I am a software developer based in New York City. I am a self-proclaimed micro-influencer, focused on helping software developers understand their industry better. To see what I look like and get a feel for my content, please check out some of my YouTube videos. Besides coding, I also enjoy writing and am lucky enough to have some generous tech friends who have shared their experiences with me. I’ve published their stories — and some of my own — in my book, “Software Developer Life.”
Google TechTalks
Google Tech Talks is a grass-roots program at Google for sharing information of interest to the technical community. At its best, it’s part of an ongoing discussion about our world featuring top experts in diverse fields. Presentations range from the broadest of perspective overviews to the most technical of deep dives, on topics well-established to wildly speculative.
Hello World, it’s Siraj! I’m a technologist on a mission to spread data literacy. Artificial Intelligence, Mathematics, Science, Technology, I simplify these topics to help you understand how they work. Using this knowledge you can build wealth and live a happier, more meaningful life. I live to serve this community. We are the fastest growing AI community in the world! Co-Founder of Sage Health (www.sage-health.org) Twitter: http://www.twitter.com/sirajraval Instagram: https://instagram.com/sirajraval/ Facebook: https://www.facebook.com/sirajology/
suckerpinch
This is Tom 7’s youtube!
Scaler Academy
Scaler is a tech-focused upskilling and reskilling platform for all of us that may have written anywhere between zero to a few million lines of code but want to get better. The brainchild of Abhimanyu Saxena and Anshuman Singh, founders of InterviewBit, one of India’s largest interview preparation platforms for top tech companies, started out with Academy, a course for working professionals with more than 1 year of experience, and has now grown to include Edge which is for college students. Scaler’s courses have industry-relevant curriculums which are vetted by CTOs, regular doubt solving support through Teaching Assistants and 1:1 personal mentorship from people who currently work at some top tech companies and startups. True to our mission, we have already empowered thousands of students, with our alumni working in companies such as Google, Microsoft and Amazon. But we believe this is only the beginning. Come along, as we build a future together.
$ cat /var/log/maillog | grep 'lost connection after AUTH from unknown' | tail -n 5
May 10 11:19:49 srv4 postfix/smtpd[1486]: lost connection after AUTH from unknown[185.36.81.145]
May 10 11:21:41 srv4 postfix/smtpd[1762]: lost connection after AUTH from unknown[185.36.81.164]
May 10 11:21:56 srv4 postfix/smtpd[1762]: lost connection after AUTH from unknown[175.139.231.129]
May 10 11:23:51 srv4 postfix/smtpd[1838]: lost connection after AUTH from unknown[185.211.245.170]
May 10 11:24:02 srv4 postfix/smtpd[1838]: lost connection after AUTH from unknown[185.211.245.170]
$ for ip in $(cat /var/log/maillog | grep 'lost connection after AUTH from unknown' | cut -d'[' -f3 | cut -d ']' -f1 | sort | uniq); do iptables -I INPUT -s ${ip} -p tcp --dport 25 -j DROP; done
If Statements
Check if args are passed
if [[ $# -eq 0 ]] ; then
echo 'need to pass args'
exit 0
fi
Check if required variables exist
if [ $1 == "one" ] || [ $1 == "two" ]
then
echo "argument 1 has the value one or two"
exit 0
else
echo "I require argument 1 to be one or two"
exit 1
fi
Check if environment variables exists
if [ -z ${OWNER} ] || [ -z ${NAME} ]
then
echo "does not meet requirements of both environment variables"
exit 1
else
echo "required environment variables exists"
fi
While Loops
Run process for 5 Seconds
set -ex
count=0
echo "boot"
ping localhost &
while [ $count -le 5 ]
do
sleep 1
count=$((count + 1))
echo $count
done
ps aux | grep ping
echo "tear down"
kill $!
sleep 2
Redirecting Outputs
Stdout, Stderr
Redirect stderr to /dev/null:
grep -irl faker . 2>/dev/null
Redirect stdout to one file and stderr to another file:
grep -irl faker . > out 2>error
Redirect stderr to stdout (&1), and then redirect stdout to a file:
This article will be accompanied by the followinggithub repositorywhich will contain all the commands listed as well as folders that demonstrate before and after usage!
The readme for this git repo will provide a much more condensed list… whereas this article will break up the commands with explanations… images & links!
I will include the code examples as both github gists (for proper syntax highlighting) and as code snippets adjacent to said gists so that they can easily be copied and pasted… or … if you’re like me for instance; and like to use an extension to grab the markdown content of a page… the code will be included rather than just a link to the gist!
Here’s a Cheatsheet:
Getting Started (Advanced Users Skip Section):
✔ Check the Current Directory ➡ pwd:
On the command line, it’s important to know the directory we are currently working on. For that, we can use pwd command.
It shows that I’m working on my Desktop directory.
✔ Display List of Files ➡ ls:
To see the list of files and directories in the current directory use ls command in your CLI.
Shows all of my files and directories of my Desktop directory.
To show the contents of a directory pass the directory name to the ls command i.e. ls directory_name.
Some useful ls command options:-
OptionDescriptionls -alist all files including hidden file starting with ‘.’ls -llist with the long formatls -lalist long format including hidden files
✔ Create a Directory ➡ mkdir:
We can create a new folder using the mkdir command. To use it type mkdir folder_name.
Use `ls` command to see the directory is created or not.
I created a cli-practice directory in my working directory i.e. Desktop directory.
✔ Move Between Directories ➡ cd:
It’s used to change directory or to move other directories. To use it type cd directory_name.
Can use `pwd` command to confirm your directory name.
Changed my directory to the cli-practice directory. And the rest of the tutorial I’m gonna work within this directory.
✔ Parent Directory ➡ ..:
We have seen cd command to change directory but if we want to move back or want to move to the parent directory we can use a special symbol .. after cd command, like cd ..
✔ Create Files ➡ touch:
We can create an empty file by typing touch file_name. It's going to create a new file in the current directory (the directory you are currently in) with your provided name.
I created a hello.txt file in my current working directory. Again you can use `ls` command to see the file is created or not.
Now open your hello.txt file in your text editor and write Hello Everyone! into your hello.txt file and save it.
✔ Display the Content of a File ➡ cat:
We can display the content of a file using the cat command. To use it type cat file_name.
Shows the content of my hello.txt file.
✔ Move Files & Directories ➡ mv:
To move a file and directory, we use mv command.
By typing mv file_to_move destination_directory, you can move a file to the specified directory.
By entering mv directory_to_move destination_directory, you can move all the files and directories under that directory.
Before using this command, we are going to create two more directories and another txt file in our cli-practice directory.
mkdir html css touch bye.txt
Yes, we can use multiple directories & files names one after another to create multiple directories & files in one command.
Moved my bye.txt file into my css directory and then moved my css directory into my html directory.
✔ Rename Files & Directories ➡ mv:
mv command can also be used to rename a file and a directory.
You can rename a file by typing mv old_file_name new_file_name & also rename a directory by typing mv old_directory_name new_directory_name.
Renamed my hello.txt file to the hi.txt file and html directory to the folder directory.
✔ Copy Files & Directories ➡ cp:
To do this, we use the cp command.
You can copy a file by entering cp file_to_copy new_file_name.
Copied my hi.txt file content into hello.txt file. For confirmation open your hello.txt file in your text editor.
You can also copy a directory by adding the -r option, like cp -r directory_to_copy new_directory_name.
The-roption for "recursive" means that it will copy all of the files including the files inside of subfolders.
Here I copied all of the files from the folder to folder-copy.
✔ Remove Files & Directories ➡ rm:
To do this, we use the rm command.
To remove a file, you can use the command like rm file_to_remove.
Here I removed my hi.txt file.
To remove a directory, use the command like rm -r directory_to_remove.
I removed my folder-copy directory from my cli-practice directory i.e. current working directory.
✔ Clear Screen ➡ clear:
Clear command is used to clear the terminal screen.
✔ Home Directory ➡ ~:
The Home directory is represented by ~. The Home directory refers to the base directory for the user. If we want to move to the Home directory we can use cd ~ command. Or we can only use cd command.
MY COMMANDS:
1.) Recursively unzip zip files and then delete the archives when finished:
here is afolder containing the before and after… I had to change folder names slightly due to a limit on the length of file-paths in a github repo.
find . -name "*.zip" | while read filename; do unzip -o -d "`dirname "$filename"`" "$filename"; done;
find . -name "*.zip" -type f -print -delete
2.) Install node modules recursively:
npm i -g recursive-install
npm-recursive-install
3.) Clean up unnecessary files/folders in git repo:
find . -empty -type f -print -delete #Remove empty files
# -------------------------------------------------------
find . -empty -type d -print -delete #Remove empty folders
# -------------------------------------------------------
# This will remove .git folders... .gitmodule files as well as .gitattributes and .gitignore files.
find . \( -name ".git" -o -name ".gitignore" -o -name ".gitmodules" -o -name ".gitattributes" \) -exec rm -rf -- {} +
# -------------------------------------------------------
# This will remove the filenames you see listed below that just take up space if a repo has been downloaded for use exclusively in your personal file system (in which case the following files just take up space)# Disclaimer... you should not use this command in a repo that you intend to use with your work as it removes files that attribute the work to their original creators!
find . \( -name "*SECURITY.txt" -o -name "*RELEASE.txt" -o -name "*CHANGELOG.txt" -o -name "*LICENSE.txt" -o -name "*CONTRIBUTING.txt" -name "*HISTORY.md" -o -name "*LICENSE" -o -name "*SECURITY.md" -o -name "*RELEASE.md" -o -name "*CHANGELOG.md" -o -name "*LICENSE.md" -o -name "*CODE_OF_CONDUCT.md" -o -name "\*CONTRIBUTING.md" \) -exec rm -rf -- {} +
In Action:
The following output from my bash shell corresponds to the directory:
The command seen below deletes most SECURITY, RELEASE, CHANGELOG, LICENSE, CONTRIBUTING, & HISTORY files that take up pointless space in repo’s you wish to keep exclusively for your own reference.
!!!Use with caution as this command removes the attribution of the work from it’s original authors!!!!!
!!!Use with caution as this command removes the attribution of the work from it’s original authors!!!!!find . \( -name "*SECURITY.txt" -o -name "*RELEASE.txt" -o -name "*CHANGELOG.txt" -o -name "*LICENSE.txt" -o -name "*CONTRIBUTING.txt" -name "*HISTORY.md" -o -name "*LICENSE" -o -name "*SECURITY.md" -o -name "*RELEASE.md" -o -name "*CHANGELOG.md" -o -name "*LICENSE.md" -o -name "*CODE_OF_CONDUCT.md" -o -name "*CONTRIBUTING.md" \) -exec rm -rf -- {} +
4.) Generate index.html file that links to all other files in working directory:
6.)Recursively remove lines of text containing the string badFolder from files in the working directory.
find . -type f -exec sed -i '/badFolder/d' ./* {} \;
# OR
find . -name 'result.md' -type f -exec sed -i '/badFolder/d' ./* {} \;
As an example I will run this command on a file containing the text:
Hacks Blog
Read more at hacks.mozilla.org
badFolder
badFolder Implementing Private Fields for JavaScript
When implementing a language feature for JavaScript, an implementer must make decisions about how the language in the specification maps to the implementation. Private fields is an example of where the specification language and implementation reality diverge, at least in SpiderMonkey– the JavaScript engine which powers Firefox. To understand more, I’ll explain what private fields are, a couple of models for thinking about them, and explain why our implementation diverges from the specification language.The post Implementing Private Fields for JavaScript appeared first on Mozilla Hacks - the Web developer blog.
Posted Tuesday, June 8, 2021 by Matthew Gaudet
Looking fine with Firefox 89
Firefox 89 has smartened up and brings with it a slimmed-down, slightly more minimalist interface.badFolder Along with this new look, we get some great styling features including a force-colours feature for media queries and better control over how fonts are displayed. The long-awaited top-level await keyword for JavaScript modules is now enabled, as well as the PerformanceEventTiming interface, which is another addition to the performance suite of APIs: 89 really has been working out!The post Looking fine with Firefox 89 appeared first on Mozilla Hacks - the Web developer blog.
badFolder
Posted Tuesday, June 1, 2021 by Chris Mills
badFolder
Improving Firefox stability on Linux
Roughly a year ago at Mozilla we started an effort to improve Firefox stability on Linux. This effort quickly became an example of good synergies between FOSS projects.The post Improving Firefox stability on Linux appeared first on Mozilla Hacks - the Web developer blog.
Posted Wednesday, May 19, 2021 by Gabriele Svelto badFolder
Introducing Firefox’s new Site Isolation Security Architecture
Like any web browser, Firefox loads code from untrusted and potentially hostile websites and runs it on your computer. To protect you against new types of attacks from malicious sites and to meet the security principles of Mozilla, we set out to redesign Firefox on desktop.The post Introducing Firefox’s new Site Isolation Security Architecture appeared first on Mozilla Hacks - the Web developer blog.
Posted Tuesday, May 18, 2021 by Anny Gakhokidze
Pyodide Spin Out and 0.17 Release
We are happy to announce that Pyodide has become an independent and community-driven project. We are also pleased to announce the 0.17 release for Pyodide with many new features and improvements. Pyodide consists of the CPython 3.8 interpreter compiled to WebAssembly which allows Python to run in the browser.The post Pyodide Spin Out and 0.17 Release appeared first on Mozilla Hacks - the Web developer blog. badFolder
Posted Thursday, April 22, 2021 by Teon Brooks
I modified the command slightly to apply only to files called ‘result.md’:
The result is :
Hacks Blog
Read more at hacks.mozilla.org
When implementing a language feature for JavaScript, an implementer must make decisions about how the language in the specification maps to the implementation. Private fields is an example of where the specification language and implementation reality diverge, at least in SpiderMonkey– the JavaScript engine which powers Firefox. To understand more, I’ll explain what private fields are, a couple of models for thinking about them, and explain why our implementation diverges from the specification language.The post Implementing Private Fields for JavaScript appeared first on Mozilla Hacks - the Web developer blog.
Posted Tuesday, June 8, 2021 by Matthew Gaudet
Looking fine with Firefox 89
Posted Tuesday, June 1, 2021 by Chris Mills
Improving Firefox stability on Linux
Roughly a year ago at Mozilla we started an effort to improve Firefox stability on Linux. This effort quickly became an example of good synergies between FOSS projects.The post Improving Firefox stability on Linux appeared first on Mozilla Hacks - the Web developer blog.
Introducing Firefox’s new Site Isolation Security Architecture
Like any web browser, Firefox loads code from untrusted and potentially hostile websites and runs it on your computer. To protect you against new types of attacks from malicious sites and to meet the security principles of Mozilla, we set out to redesign Firefox on desktop.The post Introducing Firefox’s new Site Isolation Security Architecture appeared first on Mozilla Hacks - the Web developer blog.
Posted Tuesday, May 18, 2021 by Anny Gakhokidze
Pyodide Spin Out and 0.17 Release
Posted Thursday, April 22, 2021 by Teon Brooks
the test.txt and result.md files can be found here:
Here I have modified the command I wish to run recursively to account for the fact that the ‘find’ command already works recursively, by appending the -maxdepth 1 flag…
I am essentially removing the recursive action of the find command…
That way, if the command affects the more deeply nested folders we know the outer RecurseDirs function we are using to run the find/pandoc line once in every subfolder of the working directory… is working properly!
**Run in the folder shown to the left… we would expect every .md file to be accompanied by a newly generated html file by the same name.**
If you want to run any bash script recursively all you have to do is substitue out line #9 with the command you want to run once in every sub-folder.
function RecurseDirs ()
{
oldIFS=$IFS
IFS=$'\n'
for f in "$@"
do
#Replace the line below with your own command!
#find ./ -iname "*.md" -maxdepth 1 -type f -exec sh -c 'pandoc --standalone "${0}" -o "${0%.md}.html"' {} \;
#####################################################
# YOUR CODE BELOW!
#####################################################
if [[ -d "${f}" ]]; then
cd "${f}"
RecurseDirs $(ls -1 ".")
cd ..
fi
done
IFS=$oldIFS
}
RecurseDirs "./"
TBC….
Here are some of the other commands I will cover in greater detail… at a later time:
9. Copy any text between <script> tags in a file called example.html to be inserted into a new file: out.js
sed -n -e '/<script>/,/<\/script>/p' example.html >out.js
13. Add closing body and script tags to each html file in working directory.
for f in * ; do
mv "$f" "$f.html"
doneecho "<form>
<input type="button" value="Go back!" onclick="history.back()">
</form>
</body></html>" | tee -a *.html
14. Batch Download Videos
#!/bin/bash
link="#insert url here#"
#links were a set of strings with just the index of the video as the variable
num=3
#first video was numbered 3 - weird.
ext=".mp4"
while [ $num -le 66 ]
do
wget $link$num$ext -P ~/Downloads/
num=$(($num+1))
done
15. Change File Extension from ‘.txt’ to .doc for all files in working directory.
Cheat sheet and in-depth explanations located below main article contents… The UNIX shell program interprets user commands, which are…
Bash Proficiency In Under 15 Minutes
Cheat sheet and in-depth explanations located below main article contents… The UNIX shell program interprets user commands, which are either directly entered by the user, or which can be read from a file called the shell script or shell program. Shell scripts are interpreted, not compiled. The shell reads commands from the script line per line and searches for those commands on the system while a compiler converts a program into machine readable form, an executable file.
LIFE SAVING PROTIP:
A nice thing to do is to add on the first line
#!/bin/bash -x
I will go deeper into the explanations behind some of these examples at the bottom of this article.
Here’s some previous articles I’ve written for more advanced users.
Apart from passing commands to the kernel, the main task of a shell is providing a user environment through which they can issue the computer commands via a command line instead of the graphical user interfaces most software consumers are familiar with.
fire meme
Here’s a REPL with some examples for you to practice….
Remember: learning is an effortful activity… it’s not comfortable… practice might be unpleasant but if you don’t you might as well skip reading too because without application… reading articles just provides a false sense of accomplishment….
cd <file path here>
# to go up a directory from your current directory
cd ..
List a directory’s contents
ls
# for more details, add -l (long)
ls -l
# this will output something like this:
# -rw-r--r-- 1 cameronnokes staff 1237 Jun 2 22:46 index.js
# in order, those columns are:
# permissions for you, your group, all
# number of links (hardlinks & softlinks)
# owner user
# owner group
# file size
# last modified time
# file name
# to see hidden files/folders (like .git or .npmignore)
ls -a
# Note, flags can be combined like so
ls -la
cat <file name>
# shows it with line numbers
cat -n <file name>
View a file in bash
# view the file without dumping it all onto your screen
less <file name>
# Some useful shortcuts in less
# Shift+g (jump to end)
# g (go back to top)
# / (search)
# q (quit/close)
View file/folder in default application associated with it
open <file/folder name>
# view current directory in Finder
open .
# specify an application to use
open <file name> -a TextEdit
# set the file's contents
echo 'hi' > file.txt
# append to file's contents
echo 'hi' >> file.txt
# note that if you pass a file name that doesn't exist, it'll get created on the fly
Create a directory
mkdir <folder name>
# make intermediary directories as needed
mkdir -p parent/child/grandchild
Remove a file
# Note, this permanently deletes a file
rm <file name>
# Remove a folder and it's contents, recursively
rm -rf <folder name>
find# find all the PNGs in a folder
find -name "*.png"
# find all the JPGs (case insensitive) in a folder
find -iname "*.jpg"
# find only directories
find -type d
# delete all matching files
find -name "*.built.js" -delete
# execute an arbitrary action on each match
# remember `{}` will be replaced with the file name
find -name "*.png" -exec pngquant {} \;
httpThe test server is available in the `curl-practice-server` directory. Run `npm install && npm start` to run it.
curl <url>
# Useful flags
# -i (show response headers only)
# -L (follow redirects)
# -H (header flag)
# -X (set HTTP method)
# -d (request body)
# -o (output to a file)
# to POST JSON
# (technically you don't need -X POST because -d will make it POST automatically, but I like to be explicit)
curl -X POST -H "Content-Type: application/json" -d '{ "title": "Curling" }' http://localhost:3000/api/posts
# POST a url encoded form
curl -X POST --data-urlencode title="Curling again" http://localhost:3000/api/posts
# multiline curl (applies to any bash command)
curl -i -X PUT \
-d '{ "title": "Changed title" }' \
-H "Content-Type: application/json" \
http://localhost:3000/api/posts
# pretty print JSON with jsome
curl https://swapi.co/api/people/1/ | jsome
# no spaces between name, =, and value
var=123
echo $var
# to make it accessible to all child processes of current shell, export it
export var
# this deletes the variable
unset var
To see all environment variables
env
clone-to-temp.sh script:
temp=$(mktemp -d)
git clone --branch $1 $PWD $temp
echo "branch $1 cloned to $temp"
# run some tasks, tests, etc here
greet() {
echo "$1 world"
}
greeting=$(greet "howdy")
echo "the greeting is $greeting"
global=123
test() {
echo "global = $global"
local local_var="i'm a local"
echo "local_var = $local_var"
}
test
echo "global = $global"
echo "local_var = $local_var" # will be empty because it's out of scope
# Some conditional primaries that can be used in the if expression:
# =, != string (in)equality
# -eq, -ne numeric (in)equality
# -lt, -gt less/greater than
# -z check variable is not set
# -e check file/folder exists
if [[ $USER = 'cameronnokes' ]]; then
echo "true"
else
echo "false"
fi
Conditionals can be used inline in a more ternary-like format
# ps ax will list all running processes
ps ax | grep Chrome | less
# get the file size after uglify + gzip
uglifyjs -c -m -- index.js | gzip -9 | wc -c
Redirection
# redirect stdout to a file
ls > ls.txt
# append stdout to a file
echo "hi" >> ls.txt
Update(Utility Commands):
Find files that have been modified on your system in the past 60 minutes
Pipes let you use the output of a program as the input of another one
simple pipe with sed
This is very simple way to use pipes.
ls -l | sed -e "s/[aeio]/u/g"
Here, the following happens: first the command ls -l is executed, and it’s output, instead of being printed, is sent (piped) to the sed program, which in turn, prints what it has to.
an alternative to ls -l *.txt
Probably, this is a more difficult way to do ls -l *.txt, but this is for educational purposes.
ls -l | grep "\.txt$"
Here, the output of the program ls -l is sent to the grep program, which, in turn, will print lines which match the regex “\.txt$”.
Variables
You can use variables as in any programming languages. There are no data types. A variable in bash can contain a number, a character, a string of characters.
You have no need to declare a variable, just assigning a value to its reference will create it.
Hello World! using variables
#!/bin/bash
STR="Hello World!"
echo $STR
Line 2 creates a variable called STR and assigns the string “Hello World!” to it. Then the VALUE of this variable is retrieved by putting the ‘$’ in at the beginning. Please notice (try it!) that if you don’t use the ‘$’ sign, the output of the program will be different, and probably not what you want it to be.
A very simple backup script (little bit better)
#!/bin/bash
OF=/var/my-backup-$(date +%Y%m%d).tgz
tar -cZf $OF /home/me/
This script introduces another thing. First of all, you should be familiarized with the variable creation and assignation on line 2. Notice the expression ‘$(date +%Y%m%d)’. If you run the script you’ll notice that it runs the command inside the parenthesis, capturing its output.
Notice that in this script, the output filename will be different every day, due to the format switch to the date command(+%Y%m%d). You can change this by specifying a different format.
examples:
echo ls
echo $(ls)
Local variables
Local variables can be created by using the keyword local.
#!/bin/bash
HELLO=Hello
function hello {
local HELLO=World
echo $HELLO
}
echo $HELLO
hello
echo $HELLO
Basic conditional example if .. then
#!/bin/bash
if [ "foo" = "foo" ]; then
echo expression evaluated as true
fi
The code to be executed if the expression within braces is true can be found after the ‘then’ word and before ‘fi’ which indicates the end of the conditionally executed code.
Basic conditional example if .. then … else
#!/bin/bash
if [ "foo" = "foo" ]; then
echo expression evaluated as true
else
echo expression evaluated as false
fi
Conditionals with variables
#!/bin/bash
T1="foo"
T2="bar"
if [ "$T1" = "$T2" ]; then
echo expression evaluated as true
else
echo expression evaluated as false
fi
Loops
for
while
(there’s another loop called until but I don’t use it so you can look it up if you’d like)
The until loop is almost equal to the while loop, except that the code is executed while thecontrol expressionevaluates to false.
The for loop is a little bit different from other programming languages. Basically, it let’s you iterate over a series of ‘words’ within a string.
The while executes a piece of code if the control expression is true, and only stops when it is false …or a explicit break is found within the executed code.
For
#!/bin/bash
for i in $( ls ); do
echo item: $i
done
On the second line, we declare i to be the variable that will take the different values contained in $( ls ).
The third line could be longer if needed, or there could be more lines before the done (4).
‘done’ (4) indicates that the code that used the value of $i has finished and $i can take a new value.
A more useful way to use the for loop would be to use it to match only certain files on the previous example
While
#!/bin/bash
COUNTER=0
while [ $COUNTER -lt 10 ]; do
echo The counter is $COUNTER
let COUNTER=COUNTER+1
done
Functions
As in almost any programming language, you can use functions to group pieces of code in a more logical way or practice the divine art of recursion.
Declaring a function is just a matter of writing function my_func { my_code }.
Calling a function is just like calling another program, you just write its name.
Functions ex.)
#!/bin/bash
function quit {
exit
}
function hello {
echo Hello!
}
hello
quit
echo foo
Lines 2–4 contain the ‘quit’ function. Lines 5–7 contain the ‘hello’ function If you are not absolutely sure about what this script does, please try it!.
Notice that a functions don’t need to be declared in any specific order.
When running the script you’ll notice that first: the function ‘hello’ is called, second the ‘quit’ function, and the program never reaches line 10.
Functions with parameters
#!/bin/bash
function quit {
exit
}
function e {
echo $1
}
e Hello
e World
quit
echo foo
Backup Directory Script:
#!/bin/bash
SRCD="/home/"
TGTD="/var/backups/"
OF=home-$(date +%Y%m%d).tgz
tar -cZf $TGTD$OF $SRCD
File Renamer:
Bonus Commands:
Included in a gist below (so you can see them syntax highlighted..) I am also including them in text so that they might turn up as a result of google searches … I have a hunch that google’s SEO rankings don’t put much emphasis on the content of github gists.
CodeDescription${FOO%suffix}Remove suffix${FOO#prefix}Remove prefix------${FOO%%suffix}Remove long suffix${FOO##prefix}Remove long prefix------${FOO/from/to}Replace first match${FOO//from/to}Replace all------${FOO/%from/to}Replace suffix${FOO/#from/to}Replace prefix
Comments
# Single line comment
: '
This is a
multi line
comment
'
Substrings
ExpressionDescription${FOO:0:3}Substring (position, length)${FOO:(-3):3}Substring from the right
ExpressionDescription${FOO:-val}$FOO, or val if unset (or null)${FOO:=val}Set $FOO to val if unset (or null)${FOO:+val}val if $FOO is set (and not null)${FOO:?message}Show error message and exit if $FOO is unset (or null)
Omitting the : removes the (non)nullity checks, e.g. ${FOO-val} expands to val if unset otherwise $FOO.
Loops
{: .-three-column}
Basic for loop
for i in /etc/rc.*; do
echo $i
done
C-like for loop
for ((i = 0 ; i < 100 ; i++)); do
echo $i
done
Ranges
for i in {1..5}; do
echo "Welcome $i"
done
With step size
for i in {5..50..5}; do
echo "Welcome $i"
done
Reading lines
cat file.txt | while read line; do
echo $line
done
Forever
while true; do
···
done
Functions
{: .-three-column}
Defining functions
myfunc() {
echo "hello $1"
}
# Same as above (alternate syntax)
function myfunc() {
echo "hello $1"
}
myfunc "John"
Returning values
myfunc() {
local myresult='some value'
echo $myresult
}
result="$(myfunc)"
Raising errors
myfunc() {
return 1
}
if myfunc; then
echo "success"
else
echo "failure"
fi
Arguments
ExpressionDescription$#Number of arguments$*All postional arguments (as a single word)$@All postitional arguments (as separate strings)$1First argument$_Last argument of the previous command
Note: $@ and $* must be quoted in order to perform as described. Otherwise, they do exactly the same thing (arguments as separate strings).
Note that [[ is actually a command/program that returns either 0 (true) or 1 (false). Any program that obeys the same logic (like all base utils, such as grep(1) or ping(1)) can be used as condition, see examples.
ConditionDescription[[ -z STRING ]]Empty string[[ -n STRING ]]Not empty string[[ STRING == STRING ]]Equal[[ STRING != STRING ]]Not Equal------[[ NUM -eq NUM ]]Equal[[ NUM -ne NUM ]]Not equal[[ NUM -lt NUM ]]Less than[[ NUM -le NUM ]]Less than or equal[[ NUM -gt NUM ]]Greater than[[ NUM -ge NUM ]]Greater than or equal------[[ STRING =~ STRING ]]Regexp------(( NUM < NUM ))Numeric conditions
More conditions
ConditionDescription[[ -o noclobber ]]If OPTIONNAME is enabled------[[ ! EXPR ]]Not[[ X && Y ]]And`[[ X
File conditions
ConditionDescription[[ -e FILE ]]Exists[[ -r FILE ]]Readable[[ -h FILE ]]Symlink[[ -d FILE ]]Directory[[ -w FILE ]]Writable[[ -s FILE ]]Size is > 0 bytes[[ -f FILE ]]File[[ -x FILE ]]Executable------[[ FILE1 -nt FILE2 ]]1 is more recent than 2[[ FILE1 -ot FILE2 ]]2 is more recent than 1[[ FILE1 -ef FILE2 ]]Same files
Example
# String
if [[ -z "$string" ]]; then
echo "String is empty"
elif [[ -n "$string" ]]; then
echo "String is not empty"
else
echo "This never happens"
fi
# Combinations
if [[ X && Y ]]; then
...
fi
# Equal
if [[ "$A" == "$B" ]]
# Regex
if [[ "A" =~ . ]]
if (( $a < $b )); then
echo "$a is smaller than $b"
fi
if [[ -e "file.txt" ]]; then
echo "file exists"
fi
echo ${Fruits[0]} # Element #0
echo ${Fruits[-1]} # Last element
echo ${Fruits[@]} # All elements, space-separated
echo ${#Fruits[@]} # Number of elements
echo ${#Fruits} # String length of the 1st element
echo ${#Fruits[3]} # String length of the Nth element
echo ${Fruits[@]:3:2} # Range (from position 3, length 2)
echo ${!Fruits[@]} # Keys of all elements, space-separated
Operations
Fruits=("${Fruits[@]}" "Watermelon") # Push
Fruits+=('Watermelon') # Also Push
Fruits=( ${Fruits[@]/Ap*/} ) # Remove by regex match
unset Fruits[2] # Remove one item
Fruits=("${Fruits[@]}") # Duplicate
Fruits=("${Fruits[@]}" "${Veggies[@]}") # Concatenate
lines=(`cat "logfile"`) # Read from file
Iteration
for i in "${arrayName[@]}"; do
echo $i
done
Dictionaries
{: .-three-column}
Defining
declare -A sounds
sounds[dog]="bark"
sounds[cow]="moo"
sounds[bird]="tweet"
sounds[wolf]="howl"
Declares sound as a Dictionary object (aka associative array).
Working with dictionaries
echo ${sounds[dog]} # Dog's sound
echo ${sounds[@]} # All values
echo ${!sounds[@]} # All keys
echo ${#sounds[@]} # Number of elements
unset sounds[dog] # Delete dog
Iteration
Iterate over values
for val in "${sounds[@]}"; do
echo $val
done
Iterate over keys
for key in "${!sounds[@]}"; do
echo $key
done
Options
Options
set -o noclobber # Avoid overlay files (echo "hi" > foo)
set -o errexit # Used to exit upon error, avoiding cascading errors
set -o pipefail # Unveils hidden failures
set -o nounset # Exposes unset variables
Set GLOBIGNORE as a colon-separated list of patterns to be removed from glob matches.
History
Commands
CommandDescriptionhistoryShow historyshopt -s histverifyDon't execute expanded result immediately
Expansions
ExpressionDescription!$Expand last parameter of most recent command!*Expand all parameters of most recent command!-nExpand nth most recent command!nExpand nth command in history!<command>Expand most recent invocation of command <command>
Operations
CodeDescription!!Execute last command again!!:s/<FROM>/<TO>/Replace first occurrence of <FROM> to <TO> in most recent command!!:gs/<FROM>/<TO>/Replace all occurrences of <FROM> to <TO> in most recent command!$:tExpand only basename from last parameter of most recent command!$:hExpand only directory from last parameter of most recent command
!! and !$ can be replaced with any valid expansion.
Slices
CodeDescription!!:nExpand only nth token from most recent command (command is 0; first argument is 1)!^Expand first argument from most recent command!$Expand last token from most recent command!!:n-mExpand range of tokens from most recent command!!:n-$Expand nth token to last from most recent command
!! can be replaced with any valid expansion i.e. !cat, !-2, !42, etc.
Miscellaneous
Numeric calculations
$((a + 200)) # Add 200 to $a
$(($RANDOM%200)) # Random number 0..199
Subshells
(cd somedir; echo "I'm now in $PWD")
pwd # still in first directory
Redirection
python hello.py > output.txt # stdout to (file)
python hello.py >> output.txt # stdout to (file), append
python hello.py 2> error.log # stderr to (file)
python hello.py 2>&1 # stderr to stdout
python hello.py 2>/dev/null # stderr to (null)
python hello.py &>/dev/null # stdout and stderr to (null)
python hello.py < foo.txt # feed foo.txt to stdin for python
Inspecting commands
command -V cd
#=> "cd is a function/alias/whatever"
Trap errors
trap 'echo Error at about $LINENO' ERR
or
traperr() {
echo "ERROR: ${BASH_SOURCE[1]} at about ${BASH_LINENO[0]}"
}
set -o errtrace
trap traperr ERR
Case/switch
case "$1" in
start | up)
vagrant up
;;
*)
echo "Usage: $0 {start|stop|ssh}"
;;
esac
Source relative
source "${0%/*}/../share/foo.sh"
printf
printf "Hello %s, I'm %s" Sven Olga
#=> "Hello Sven, I'm Olga
printf "1 + 1 = %d" 2
#=> "1 + 1 = 2"
printf "This is how you print a float: %f" 2
#=> "This is how you print a float: 2.000000"
Directory of script
DIR="${0%/*}"
Getting options
while [[ "$1" =~ ^- && ! "$1" == "--" ]]; do case $1 in
-V | --version )
echo $version
exit
;;
-s | --string )
shift; string=$1
;;
-f | --flag )
flag=1
;;
esac; shift; done
if [[ "$1" == '--' ]]; then shift; fi
Heredoc
cat <<END
hello world
END
Reading input
echo -n "Proceed? [y/n]: "
read ans
echo $ans
read -n 1 ans # Just one character
Special variables
ExpressionDescription$?Exit status of last task$!PID of last background task$$PID of shell$0Filename of the shell script
Using () implicitly returns components.Role of index.js is to render your application.The reference to root comes from a div in the body of your public html file.State of a component is simply a regular JS Object.Class Components require render() method to return JSX.Functional Components directly return JSX.Class is className in React.When parsing for an integer just chain Number.parseInt("123")
- **Use ternary operator if you want to make a conditional inside a fragment.**
{ x === y ? <div>Naisu</div> : <div>Not Naisu</div>; }
Purpose of React.Fragment is to allow you to create groups of children without adding an extra dom element.
React manages the creation and updating of DOM nodes in your Web page.
All it does is dynamically render stuff into your DOM.
What it doesn’t do:
Ajax
Services
Local Storage
Provide a CSS framework
React is unopinionated
Just contains a few rules for developers to follow, and it just works.
JSX : Javascript Extension is a language invented to help write React Applications (looks like a mixture of JS and HTML)
Here is an overview of the difference between rendering out vanilla JS to create elements, and JSX:
This may seem like a lot of code but when you end up building many components, it becomes nice to put each of those functions/classes into their own files to organize your code. Using tools with React
React DevTools : New tool in your browser to see ow React is working in the browser
create-react-app : Extensible command-line tool to help generate standard React applications.
Webpack : In between tool for dealing with the extra build step involved.
- **HMR** : (Hot Module Replacement) When you make changes to your source code the changes are delivered in real-time.
- React Developers created something called `Flux Architecture` to moderate how their web page consumes and modifies data received from back-end API's.
- **Choosing React**
- Basically, React is super important to learn and master.
React Concepts and Features
There are many benefits to using React over just Vanilla JS.
Modularity
To avoid the mess of many event listeners and template strings, React gives you the benefit of a lot of modularity.
Easy to start
No specials tools are needed to use Basic React.
You can start working directly with createElement method in React.
Declarative Programming
React is declarative in nature, utilizing either it’s build in createElement method or the higher-level language known as JSX.
Reusability
Create elements that can be re-used over and over. One-flow of data
React apps are built as a combination of parent and child components.
Parents can have one or more child components, all children have parents.
Data is never passed from child to the parent.
Virtual DOM : React provides a Virtual DOM that acts as an agent between the real DOM and the developer to help debug, maintain, and provide general use.
Due to this usage, React handles web pages much more intelligently; making it one of the speediest Front End Libraries available.
Test if you have Ubuntu installed by typing “Ubuntu” in the search box in the bottom app bar that reads “Type here to search”. If you see a search result that reads **“Ubuntu 20.04 LTS”** with “App” under it, then you have it installed.
In the application search box in the bottom bar, type “PowerShell” to find the application named “Windows PowerShell”
Right-click on “Windows PowerShell” and choose “Run as administrator” from the popup menu
In the blue PowerShell window, type the following: Enable-WindowsOptionalFeature -Online -FeatureName Microsoft-Windows-Subsystem-Linux
Restart your computer
In the application search box in the bottom bar, type “Store” to find the application named “Microsoft Store”
Click “Microsoft Store”
Click the “Search” button in the upper-right corner of the window
Type in “Ubuntu”
Click “Run Linux on Windows (Get the apps)”
Click the orange tile labeled “Ubuntu” Note that there are 3 versions in the Microsoft Store… you want the one just entitled ‘Ubuntu’
Click “Install”
After it downloads, click “Launch”
If you get the option, pin the application to the task bar. Otherwise, right-click on the orange Ubuntu icon in the task bar and choose “Pin to taskbar”
When prompted to “Enter new UNIX username”, type your first name with no spaces
When prompted, enter and retype a password for this UNIX user (it can be the same as your Windows password)
Confirm your installation by typing the command whoami ‘as in who-am-i'followed by Enter at the prompt (it should print your first name)
You need to update your packages, so type sudo apt update (if prompted for your password, enter it)
You need to upgrade your packages, so type sudo apt upgrade (if prompted for your password, enter it)
Git
Git comes with Ubuntu, so there’s nothing to install. However, you should configure it using the following instructions.
Open an Ubuntu terminal if you don’t have one open already.
You need to configure Git, so type git config --global user.name "Your Name" with replacing "Your Name" with your real name.
You need to configure Git, so type git config --global user.email your@email.com with replacing "your@email.com" with your real email.
Note: if you want git to remember your login credentials type:
$ git config --global credential.helper store
Google Chrome
Test if you have Chrome installed by typing “Chrome” in the search box in the bottom app bar that reads “Type here to search”. If you see a search result that reads “Chrome” with “App” under it, then you have it installed. Otherwise, follow these instructions to install Google Chrome.
Open Microsoft Edge, the blue “e” in the task bar, and type in http://chrome.google.com. Click the “Download Chrome” button. Click the “Accept and Install” button after reading the terms of service. Click “Save” in the “What do you want to do with ChromeSetup.exe” dialog at the bottom of the window. When you have the option to “Run” it, do so. Answer the questions as you’d like. Set it as the default browser.
Right-click on the Chrome icon in the task bar and choose “Pin to taskbar”.
Node.js
Test if you have Node.js installed by opening an Ubuntu terminal and typing node --version. If it reports "Command 'node' not found", then you need to follow these directions.
In the Ubuntu terminal, type sudo apt update and press Enter
In the Ubuntu terminal, type sudo apt install build-essential and press Enter
In the Ubuntu terminal, type curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.35.2/install.sh | bash and press Enter
In the Ubuntu terminal, type . ./.bashrc and press Enter
In the Ubuntu terminal, type nvm install --lts and press Enter
Confirm that node is installed by typing node --version and seeing it print something that is not "Command not found"!
Unzip
You will often have to download a zip file and unzip it. It is easier to do this from the command line. So we need to install a linux unzip utility.
In the Ubuntu terminal type: sudo apt install unzip and press Enter
Mocha.js
Test if you have Mocha.js installed by opening an Ubuntu terminal and typing which mocha. If it prints a path, then you're good. Otherwise, if it prints nothing, install Mocha.js by typing npm install -g mocha.
Python 3
Ubuntu does not come with Python 3. Install it using the command sudo apt install python3. Test it by typing python3 --version and seeing it print a number.
Note about WSL
As of the time of writing of this document, WSL has an issue renaming or deleting files if Visual Studio Code is open. So before doing any linux commands which manipulate files, make sure you close Visual Studio Code before running those commands in the Ubuntu terminal.
As I learn to build web applications in React I will blog about it in this series in an attempt to capture the questions that a complete…
Beginner’s Guide To React Part 2
As I learn to build web applications in React I will blog about it in this series in an attempt to capture the questions that a complete beginner might encounter that a more seasoned developer would take for granted!
Here I will walk through a demo…. skip down below for more fundamental examples and resources…
React Demo
ex1 — A Basic React Component
ex2 — A Basic React Class Component
ex3 — A Class Component with State
ex4 — A Class Component that Updates State
ex5 — A Class Component that Iterates through State
ex6 — An Example of Parent and Child Components
With regards to converting an existing HTML, CSS, and JS site into React, first you’ll want to think about how to break up your site into components,
as well as think about what the general hierarchical component structure of your site will look like.
From there, it’s a simple matter of copying the relevant HTML for that component and throwing it into the render method of your component file.
Any methods that are needed for that component to function properly can added onto your new component.
Once you’ve refactored your HTML components into React components, you’ll want to lay them out in the desired hierarchical structure
with children components being rendered by their parents, as well as ensuring that the parent components are passing down the necessary data as props to their children components.
ex.)
<!-- Hello world -->
<div class="awesome" style="border: 1px solid red">
<label for="name">Enter your name: </label>
<input type="text" id="name" />
</div>
<p>Enter your HTML here</p>
Is equivalent to:
A Basic Component
A component is some thing that is being rendered in the browser. It could be a button, a form with a bunch of fields in it…etc.…
React doesn’t place any restrictions on how large or small a component can be.
You could have an entire static site encapsulated in a single React component, but that would defeat the purpose of using React.
So the first thing to remember about a component is that a component must render something.
If nothing is being rendered from a component, then React will throw an error.
Inside of BasicComponent.js , first import React at the top of the file. Our most basic of components looks like this:
This is a component that simply returns a div tag with the words Hello World! inside.
The last line simply exports our component so that it can be imported
by another file.
Notice that this component looks exactly like an anonymous arrow function that we’ve named BasicComponent .
In fact, that is literally what this is.
The arrow function then is simply returning the div tag. When a component is written as a function like this one is, it is called a functional component.
A Basic Class Component
The above component is an example of a functional component, which is appropriate since that component is literally nothing more than a function that returns some HTML.
Functional components are great when all you want a component to do is to render some stuff.
Components can also be written as classes (although this paradigm is becoming outdated and you should strive to write your components functionally!
For this exercise, we’re going to write a class component that does exactly the same thing as the functional component we just wrote.
We’ll again need to import React at the top of the file, but we’ll also need to add a little something. Our import statement will look like this:
import React, { Component } from 'react';
So, in addition to importing React, we’re also importing the base Component class that is included in the React library.
React lets you define components as classes or functions.
Components defined as classes currently provide more features . To define a React component class, you need to extend React.Component:
The only method you must define in aReact.Componentsubclass is calledrender().
render()
The render() method is the only required method in a class component.
When called, it should examine this.props and this.state and return one of the following types:
React elements. Typically created via JSX. For example, <div /> and <MyComponent /> are React elements that instruct React to render a DOM node, or another user-defined component, respectively.
Arrays and fragments. Let you return multiple elements from render. See the documentation on fragments for more details.
Portals. Let you render children into a different DOM subtree. See the documentation on portals for more details.
String and numbers. These are rendered as text nodes in the DOM.
Booleans ornull. Render nothing. (Mostly exists to support return test && <Child /> pattern, where test is boolean.)
The render() function should be pure, meaning that it does not modify component state, it returns the same result each time it’s invoked, and it does not directly interact with the browser.
If you need to interact with the browser, perform your work in componentDidMount() or the other lifecycle methods instead. Keeping render() pure makes components easier to think about.
Note
render()will not be invoked ifshouldComponentUpdate()returns false.
The export statement at the bottom of the file also stays, completely unchanged. Our class component will thus look like this:
Notice that ourBasicClassComponentinherits from the baseComponentclass that we imported from the React library, by virtue of the 'extends' keyword.
That being said, there's nothing in this minimal component that takes advantage of any of those inherited methods.
All we have is a method on our component class calledrenderthat returns the same div tag.
If we really were deciding between whether to use a functional component versus a class component to render a simple div tag, then the functional style is more appropriate to use.
This is because class components are much better suited for handling component state and triggering events based on the component’s lifecycle.
The important takeaways at this point are that there are two types of components, functional and class components, and that functional components are well-suited if you’re just looking to render some HTML.
Class components, on the other hand, are much better suited for handling components that require more complex functionality, need to exhibit more varied behavior, and/or need to keep track of some state that may change throughout said component’s lifecycle.
A Class Component with Some State
Component state is any dynamic data that we want the component to keep track of.
For example, let’s say we have a form component. This form has some input fields that we’d like users to fill out. When a user types characters into an input field, how is that input persisted from the point of view of our form component?
The answer is by using component state!
There are a few important concepts regarding component state, such as how to update it, pass it to another component, render it, etc.
Only class components have the ability to persist state, so if at any time you realize that a component needs to keep track of some state, you know that you’ll automatically need a class component instead of a functional component.
It is possible to handle state with functional components but that requires the use of something called the useState() hook. Hooks were added in React 16.8; prior to this release, there was no mechanism to add state to functional components.
Here’s what the above component looks like as a functional component:
Our class component with state will look a lot like the basic class component we just wrote, but with some exceptions:
So far, the only new thing going on here is the constructor block. If you recall how classes in JavaScript work, classes need constructors.
Additionally, if a class is extending off of another class and wants access to its parent class’s methods and properties, then thesuperfunction needs to be called inside the class's constructor function.
Point being, the constructor function and the call to the super function are not associated with React, they are associated with all JavaScript classes.
Then there is the this.stateproperty inside the constructor function that is set as an empty object.
We're adding a property called state to our class and setting it to an empty object.
State objects in React are always just plain old objects.
So why is it that the basic class component we wrote in the previous exercise had no constructor function within its body?
That is because we had no need for them since all our class component was doing was rendering some HTML.
The constructor is needed here because that is where we need to initialize our state object.
The call tosuperis needed because we can't referencethisinside of our constructor without a call tosuperfirst.
Ok, now let’s actually use this state object.
One very common application of state objects in React components is to render the data being stored inside them within our component’s render function.
Refactoring our component class to do that:
We added a key-value pair to our state object inside our constructor.
Then we changed the contents of the render function.
Now, it’s actually rendering the data that we have inside the state object.
Notice that inside the div tags we’re using a template string literal so that we can access the value of this.state.someData straight inside of our rendered content.
With Reacts newest version, we can actually now add state to a component without explicitly defining a constructor on the class. We can refactor our class component to look like this:
This new syntax is what is often referred to as ‘syntactic sugar’: under the hood, the React library translates this back into the old constructor code that we first started with, so that the JavaScript remains valid to the JavaScript interpreter.
The clue to this is the fact that when we want to access some data from the state object, we still need to call it with this.state.someData ; changing it to just state.someData does not work.
Class Component Updating State
Great, so we can render some state that our component persists for us.
However, we said an important use case of component state is to handle dynamic data.
A single static number isn’t very dynamic at all.
So now let’s walk through how to update component state.
Notice that we’ve added two methods to our class: increment and decrement .
increment and decrement are methods that we are adding to our class component.
Unlike the render method, increment and decrement were not already a part of our class component.
This is why increment and decrement are written as arrow functions, so that they are automatically bound to our class component.
The alternative is using a declaration syntax function with the bind method to bind the context of our methods to the class component.
The more interesting thing is what is going on within the bodies of these methods.
Each calls the setState function.
setState in fact is provided to us by React.
It is the standard way to update a component's state.
It's the only way you should ever update a component's state. It may seem more verbose than necessary, but there are good reasons for why you should be doing it this way.
So the way to use setState to update a component's state is to pass it an object with each of the state keys you wish to update, along with the updated value.
In our increment method we said "I would like to update the aNumber property on my component state by adding one to it and then setting the new value as my new aNumber ".
The same thing happens in our decrement method, only we're subtracting instead of adding.
Then the other new concept we’re running into here is how to actually call these methods we’ve added to our class.
We added two HTML button tags within our `render` function, then in their respective `onClick` handlers, we specify the method that should be called whenever this button gets clicked. So whenever we click either of the buttons, our state gets updated appropriately and our component will re-render to show the correct value we're expecting.
Class Component Iterating State
Another common state pattern you’ll see being used in React components is iterating over an array in our state object and rendering each array element in its own tag.
This is often used in order to render lists.
Additionally, we want to be able to easily update lists and have React re-render our updated list.
We’ll see how both of these are done and how they work together within a single component in order to create the behavior of a dynamic list.
The first change to note is that our state object now has an ‘ingredients’ array, and a ‘newIngredient’ field that has been initialized to an empty string.
The ingredients array contains the elements that we’ll want to render in our list.
The addIngredient and handleIngredientInput methods we've added to our class receives a parameter called 'event'.
This event object is part of the browser's API.
When we interact with some DOM element, such as clicking on an HTML button, the function that is invoked upon that button being clicked actually receives the event object.
So when we type some input into an input tag, we're able grab each character that was typed into the input field through the event object parameter.
The handleIngredientInput method is what gets invoked every time the user presses a key to enter text in the input box for adding a new ingredient.
Every character the user types gets persisted in the newIngredient field on the state object.
We're able to grab the text in the input box using event.target.value
Which holds the value of the string text that is currently in the input box.
We use that to update our newIngredient string field.
Breaking down the addIngredient method, we see this event.preventDefault() invocation.
This is because this method will be used upon submitting a form, and it turns out that submitting a form triggers some default form behavior that we don't want to trigger when we submit the form (namely refreshing the entire page).
event.preventDefault() will prevent this default form behavior, meaning our form will only do what we want it to do when it is submitted.
Next, we store a reference to `this.state.ingredients` in a variable called `ingredientsList` .
So we now have a copy of the array that is stored in our state object.
We want to update the copy of the ingredients array first instead of directly updating the actual array itself in state.
Now we push whatever value is being stored at our newIngredient field onto the ingredientsList array so that our ingredientsList array is now more up-to-date than our this.state.ingredients array.
So all we have to do now is call setState appropriately in order to update the value in our state object.
Additionally, we also set the newIngredient field back to an empty string in order to clear out the input field once we submit a new ingredient.
Now it's ready to accept more user input!
Looking at our render function, first note the `this.state.ingredients.map` call.
This is looping through each ingredient in our ingredients array and returning each one within its own div tag.
This is a very common pattern for rendering everything inside an array.
Then we have an HTML form which contains an input field.
The purpose of this form is to allow a user to add new ingredients to the list. Note that we’re passing our addIngredient method to the form's onSubmit handler.
This means that our addIngredient method gets invoked whenever our form is submitted.
Lastly, the input field has an onChange handler that invokes our handleIngredientInput method whenever there is some sort of change in the input field, namely when a user types into it.
Notice that the `value` field in our input tag reads off of `this.state.newIngredient` in order to know what value to display.
So when a user enters text into the input field, the onChange handler is invoked every time, which updates our this.state.newIngredient field, which the input field and then renders.
Parent and Child Components
A single isolated component isn’t going to do us much good.
The beauty of React lies in the fact that it allows us to compose modular components together.
Let’s start off with the component we just saw, but let’s change its name to ParentComponent .
The only two other differences in this component are that we’re importing a ChildComponent and then using it inside our this.state.ingredients.map call.
ChildComponent is another React component.
Notice that we're using it just as if it were any other HTML tag.
This is how we lay out our component hierarchy: the ChildComponent is rendered within the ParentComponent.
We can see this to be the case if we open up the developer console and inspect these elements.
child-left: parent-right
Note also that we’re passing each ingredient as a ‘thing’ to the ChildComponent component.
This is how a parent component passes data to a child component. It doesn’t need to be called ‘thing’; you can call it whatever you want.
Conceptually though, every piece of data that a parent component passes down to a child component is called a ‘prop’ in React lingo.
Let’s take a look now at the Child Component. It serves two purposes:
to render the props data that it gets from a parent component,
to add the ability for a user to click on it and have it toggle a strikethrough, indicating that the item is ‘complete’.
The overall structure of the child component is nothing we haven’t seen. It’s just another class component with its own state object and a method calledhandleClick** .**
A component accesses its props via thethis.propsobject.
Any prop a parent component passes down to a child component is accessible inside the child component'sthis.propobject.
So our child component keeps its own state that tracks whether the component has been clicked or not.
Then at the top of the render function, it uses a ternary condition to determine whether the div tag that is being rendered should have a strikethrough or not.
The handleClick method is then invoked via an onClick handler on the div tag; it does the work of toggling the this.state.clicked Boolean.
The overall structure of React applications can be represented as a hierarchical tree structure, just like how the DOM itself is structure. There is an overarching root component at the top of the hierarchy that every other component sits underneath. Specifying that a component should be a child of some parent component is as simple as throwing it in the parent component’s render function, just like how we did it in this example
### **Core Concepts:**
1. What is react?
React is a declarative, efficient, and flexible JavaScript library for building user interfaces. It uses components to update and render as your data changes.
React manages the creation and continuous updating of DOM nodes in your Web page.
It does not handleAJAXrequests, Local Storage or style your website. IT is just a tool to dynamically render content on a webpage as a result of changes in ‘state’. Because it’s function is so limited in scope you may hear it referred to as a library… (not a framework … like Angular for example) and you may also hear it described as unopinionated.
2. Why use react?
Works for teams and helps UI workflow patterns
The components can be reusable
Componentized UI is the future of web dev
### Declarative programming
In the same way that you use HTML to declare what the user interface should
look like, React provides the same mechanism in its createElement method or the higher-level language known as JSX.
Declarative programming is a non-imperative style of programming in which programs describe their desired results without explicitly listing commands or steps that must be performed.
Its return value is only determined by its input values
Its return value is always the same for the same input values
A React component is considered pure if it renders the same output for the same state and props. For class components like this, React provides the PureComponent base class. Class components that extend the React.PureComponent class are treated as pure components.
Pure components have some performance improvements and render optimizations since React implements the shouldComponentUpdate() method for them with a shallow comparison for props and state.
Are React functional components pure?
Functional components are very useful in React, especially when you want to isolate state management from the component. That’s why they are often called stateless components.
However, functional components cannot leverage the performance improvements and render optimizations that come with React.PureComponent since they are not classes by definition.
If you want React to treat a functional component as a pure component, you’ll have to convert the functional component to a class component that extends React.PureComponent.
Reusability
React encourages you to think in terms of reusability as you construct the user
interface from elements and components that you create. When you
make a list or a button, you can then reuse those components to show different data ‘state’ in the same UI structure as you have built for different data previously.
#### Component-Based
Build encapsulated components that manage their own state, then compose them to make complex UIs.
Since component logic is written in JavaScript instead of templates, you can easily pass rich data through your app and keep state out of the DOM.
Learn Once, Write Anywhere
We don’t make assumptions about the rest of your technology stack, so you can develop new features in React without rewriting existing code.
React can also render on the server using Node and power mobile apps using React Native.
Speed
Due to the use of a virtual DOM, React handles changes to a Web page more
intelligently than just string manipulation. It is constantly monitors the
virtual DOM for changes. It very efficiently reconciles changes in the virtual
DOM with what it has already produced in the real DOM. This is what
makes React one of the speediest front-end libraries available.
#### 3. Who uses react?
Companies such as Facebook app for android and Instagram
Here is a link to a list of other companies who use react.
Who uses react#### 4. Setting up react
React can be set up in CodePen for quick practice development by adding react.js, react-dom and babel.
It can also be set up by downloading a react starter project from GitHub installing node and following these instructions.
Alternatively it can be set up through NPM like this.
5. Intro to eco system
Composition, being able to wrap up sections of code into there own containers so they can be re used.
How to make a large application? by combining small components to create a larger complex application.
They are similar to a collection of HTML,CSS, JS and data specific to that component.
They can be defined in pure JavaScript or JSX.
Data is either received from a component’s parent component, or it’s contained in the component itself.
Applications can be separated into smaller components like this…
React components can be created using ES6 class like this.
import React from 'react';
class Hello extends React.Component {
render () {
return <h1>Hello, {this.props.name}!</h1>;
}
}
export default Hello;
At the top with have the code to bring react and react dom libraries in.
React library is used for the react syntax.
React DOM is used to update the DOM.
We then have the Class section which creates the component.
Render() describes the specific UI for the component.
Return is used to return the JSX
And Finally ReactDOM.render is used to update the DOM.
8. Data flow with props
Small examples of data flow, see if you can get the code to work.
9. Creating lists with map
The parent component passes down to the child component as props.
Using props to access names and map to loop through each list item. Then passing this by using props.
Checking data to see if Boolean is true then adding detail to the list.
10. Prop types
PropTypes allow you to declare the type (string, number, function, etc) of each prop being passed to a component. Then if a prop passed in isn’t of the declared type you’ll get a warning in the console.
Excerpt from the React website:
React — A JavaScript library for building user interfaces
A JavaScript library for building user interfaces
Declarative
React makes it painless to create interactive UIs. Design simple views for each state in your application, and React will efficiently update and render just the right components when your data changes.
Declarative views make your code more predictable and easier to debug.
A Simple Component
React components implement a render() method that takes input data and returns what to display. This example uses an XML-like syntax called JSX. Input data that is passed into the component can be accessed by render() via this.props.
JSX is optional and not required to use React. Try the Babel REPL to see the raw JavaScript code produced by the JSX compilation step.
In addition to taking input data (accessed via this.props), a component can maintain internal state data (accessed via this.state). When a component’s state data changes, the rendered markup will be updated by re-invoking render().
An Application
Using props and state, we can put together a small Todo application. This example uses state to track the current list of items as well as the text that the user has entered. Although event handlers appear to be rendered inline, they will be collected and implemented using event delegation.
A Component Using External Plugins
React allows you to interface with other libraries and frameworks. This example uses remarkable, an external Markdown library, to convert the <textarea>’s value in real time.
My favorite language for maintainability is Python. It has simple, clean syntax, object encapsulation, good library support, and optional…
Beginners Guide To Python
My favorite language for maintainability is Python. It has simple, clean syntax, object encapsulation, good library support, and optional named parameters.
Bram Cohen
Article on basic web development setup… it is geared towards web but VSCode is an incredibly versatile editor and this stack really could suit just about anyone working in the field of computer science.
the high-level data types allow you to express complex operations in a single statement;
statement grouping is done by indentation instead of beginning and ending brackets;
no variable or argument declarations are necessary.
Installing Python:
Windows
To determine if your Windows computer already has Python 3:
Open a command prompt by entering command prompt in the Windows 10 search box and selecting the Command Prompt App in the Best match section of the results.
Enter the following command and then select the Enter key:
ConsoleCopy
python --version
1. Running `python --version` may not return a value, or may return an error message stating *'python' is not recognized as an internal or external command, operable program or batch file.* This indicates Python is not installed on your Windows system.
2. If you see the word `Python` with a set of numbers separated by `.` characters, some version of Python is installed.
i.e.
Python 3.8.0
As long as the first number is3, you have Python 3 installed.
if condition:
pass
elif condition2:
pass
else:
pass
Loops
Python has two primitive loop commands:
while loops
for loops
While loop
With the while loop we can execute a set of statements as long as a condition is true.
Example: Print i as long as i is less than 6
i = 1
while i < 6:
print(i)
i += 1
The while loop requires relevant variables to be ready, in this example we need to define an indexing variable, i, which we set to 1.
With the break statement we can stop the loop even if the while condition is true
With the continue statement we can stop the current iteration, and continue with the next.
With the else statement we can run a block of code once when the condition no longer is true.
For loop
A for loop is used for iterating over a sequence (that is either a list, a tuple, a dictionary, a set, or a string).
This is less like the for keyword in other programming languages, and works more like an iterator method as found in other object-orientated programming languages.
With the for loop we can execute a set of statements, once for each item in a list, tuple, set etc.
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)
The for loop does not require an indexing variable to set beforehand.
To loop through a set of code a specified number of times, we can use the range() function.
The range() function returns a sequence of numbers, starting from 0 by default, and increments by 1 (by default), and ends at a specified number.
The range() function defaults to increment the sequence by 1, however it is possible to specify the increment value by adding a third parameter: range(2, 30, 3).
The else keyword in a for loop specifies a block of code to be executed when the loop is finished.
A nested loop is a loop inside a loop.
The “inner loop” will be executed one time for each iteration of the “outer loop”:
adj = ["red", "big", "tasty"]
fruits = ["apple", "banana", "cherry"]
for x in adj:
for y in fruits:
print(x, y)
for loops cannot be empty, but if you for some reason have a for loop with no content, put in the pass statement to avoid getting an error.
for x in [0, 1, 2]:
pass
Function definition
def function_name():
return
Function call
function_name()
We need not to specify the return type of the function.
Functions by default return None
We can return any datatype.
Python Syntax
Python syntax was made for readability, and easy editing. For example, the python language uses a : and indented code, while javascript and others generally use {} and indented code.
First Program
Lets create a python 3 repl, and call it Hello World. Now you have a blank file called main.py. Now let us write our first line of code:
print('Hello world!')
Brian Kernighan actually wrote the first “Hello, World!” program as part of the documentation for the BCPL programming language developed by Martin Richards.
Now, press the run button, which obviously runs the code. If you are not using replit, this will not work. You should research how to run a file with your text editor.
Command Line
If you look to your left at the console where hello world was just printed, you can see a >, >>>, or $ depending on what you are using. After the prompt, try typing a line of code.
Python 3.6.1 (default, Jun 21 2017, 18:48:35)
[GCC 4.9.2] on linux
Type "help", "copyright", "credits" or "license" for more information.
> print('Testing command line')
Testing command line
> print('Are you sure this works?')
Are you sure this works?
>
The command line allows you to execute single lines of code at a time. It is often used when trying out a new function or method in the language.
New: Comments!
Another cool thing that you can generally do with all languages, are comments. In python, a comment starts with a #. The computer ignores all text starting after the #.
# Write some comments!
If you have a huge comment, do not comment all the 350 lines, just put ''' before it, and ''' at the end. Technically, this is not a comment but a string, but the computer still ignores it, so we will use it.
New: Variables!
Unlike many other languages, there is no var, let, or const to declare a variable in python. You simply go name = 'value'.
Remember, there is a difference between integers and strings. Remember: String ="". To convert between these two, you can put an int in a str() function, and a string in a int() function. There is also a less used one, called a float. Mainly, these are integers with decimals. Change them using the float() command.
x = 5
x = str(x)
b = '5'
b = int(b)
print('x = ', x, '; b = ', str(b), ';') # => x = 5; b = 5;
Instead of using the , in the print function, you can put a + to combine the variables and string.
Operators
There are many operators in python:
+
-
/
*
These operators are the same in most languages, and allow for addition, subtraction, division, and multiplicaiton.
Now, we can look at a few more complicated ones:
*simpleops.py*
x = 4
a = x + 1
a = x - 1
a = x * 2
a = x / 2
You should already know everything shown above, as it is similar to other languages. If you continue down, you will see more complicated ones.
complexop.py
a += 1
a -= 1
a *= 2
a /= 2
The ones above are to edit the current value of the variable.
Sorry to JS users, as there is no i++; or anything.
Fun Fact: The python language was named after Monty Python.
If you really want to know about the others, view Py Operators
More Things With Strings
Like the title?
Anyways, a ' and a " both indicate a string, but do not combine them!
quotes.py
x = 'hello' # Good
x = "hello" # Good
x = "hello' # ERRORRR!!!
slicing.py
String Slicing
You can look at only certain parts of the string by slicing it, using [num:num].
The first number stands for how far in you go from the front, and the second stands for how far in you go from the back.
Input is a function that gathers input entered from the user in the command line. It takes one optional parameter, which is the users prompt.
inp.py
print('Type something: ')
x = input()
print('Here is what you said: ', x)
If you wanted to make it smaller, and look neater to the user, you could do…
inp2.py
print('Here is what you said: ', input('Type something: '))
Running: inp.py
Type something:
Hello World
Here is what you said: Hello World
inp2.py
Type something: Hello World
Here is what you said: Hello World
New: Importing Modules
Python has created a lot of functions that are located in other .py files. You need to import these modules to gain access to the,, You may wonder why python did this. The purpose of separate modules is to make python faster. Instead of storing millions and millions of functions, , it only needs a few basic ones. To import a module, you must write input <modulename>. Do not add the .py extension to the file name. In this example , we will be using a python created module named random.
module.py
import random
Now, I have access to all functions in the random.py file. To access a specific function in the module, you would do <module>.<function>. For example:
module2.py
import random
print(random.randint(3,5)) # Prints a random number between 3 and 5
Pro Tip:
Dofrom random import randintto not have to dorandom.randint(), justrandint()*
To import all functions from a module, you could do* from random import *
New: Loops!
Loops allow you to repeat code over and over again. This is useful if you want to print Hi with a delay of one second 100 times.
for Loop
The for loop goes through a list of variables, making a seperate variable equal one of the list every time.
Let’s say we wanted to create the example above.
loop.py
from time import sleep
for i in range(100):
print('Hello')
sleep(.3)
This will print Hello with a .3 second delay 100 times. This is just one way to use it, but it is usually used like this:
loop2.py
import time
for number in range(100):
print(number)
time.sleep(.1)
The while loop runs the code while something stays true. You would put while <expression>. Every time the loop runs, it evaluates if the expression is True. It it is, it runs the code, if not it continues outside of the loop. For example:
while.py
while True: # Runs forever
print('Hello World!')
Or you could do:
while2.py
import random
position = '<placeholder>'
while position != 1: # will run at least once
position = random.randint(1, 10)
print(position)
New: if Statement
The if statement allows you to check if something is True. If so, it runs the code, if not, it continues on. It is kind of like a while loop, but it executes only once. An if statement is written:
if.py
import random
num = random.randint(1, 10)
if num == 3:
print('num is 3. Hooray!!!')
if num > 5:
print('Num is greater than 5')
if num == 12:
print('Num is 12, which means that there is a problem with the python language, see if you can figure it out. Extra credit if you can figure it out!')
Now, you may think that it would be better if you could make it print only one message. Not as many that are True. You can do that with an elif statement:
elif.py
import random
num = random.randint(1, 10)
if num == 3:
print('Num is three, this is the only msg you will see.')
elif num > 2:
print('Num is not three, but is greater than 1')
Now, you may wonder how to run code if none work. Well, there is a simple statement called else:
else.py
import random
num = random.randint(1, 10)
if num == 3:
print('Num is three, this is the only msg you will see.')
elif num > 2:
print('Num is not three, but is greater than 1')
else:
print('No category')
New: Functions (def)
So far, you have only seen how to use functions other people have made. Let use the example that you want to print the a random number between 1 and 9, and print different text every time.
It is quite tiring to type:
Characters: 389
nofunc.py
import random
print(random.randint(1, 9))
print('Wow that was interesting.')
print(random.randint(1, 9))
print('Look at the number above ^')
print(random.randint(1, 9))
print('All of these have been interesting numbers.')
print(random.randint(1, 9))
print("these random.randint's are getting annoying to type")
print(random.randint(1, 9))
print('Hi')
print(random.randint(1, 9))
print('j')
Now with functions, you can seriously lower the amount of characters:
Characters: 254
functions.py
import random
def r(t):
print(random.randint(1, 9))
print(t)
r('Wow that was interesting.')
r('Look at the number above ^')
r('All of these have been interesting numbers.')
r("these random.randint's are getting annoying to type")
r('Hi')
r('j')
Project Based Learning:
The following is a modified version of a tutorial posted By: InvisibleOne
I would cite the original tutorial it’s self but at the time of this writing I can no longer find it on his repl.it profile and so the only reference I have are my own notes from following the tutorial when I first found it.
1. Adventure Story
The first thing you need with an adventure story is a great storyline, something that is exciting and fun. The idea is, that at each pivotal point in the story, you give the player the opportunity to make a choice.
First things first, let’s import the stuff that we need, like this:
import os #very useful for clearing the screen
import random
Now, we need some variables to hold some of the player data.
name = input(“Name Please: “) #We’ll use this to get the name from the user
nickname = input(“Nickname: “)
Ok, now we have the player’s name and nickname, let’s welcome them to the game
print(“Hello and welcome “ + name)
Now for the story. The most important part of all stories is the introduction, so let’s print our introduction
print(“Long ago, there was a magical meal known as Summuh and Spich Atip”) #We can drop a line by making a new print statement, or we can use the escape code \n
print(“It was said that this meal had the power to save lives, restore peace, and stop evil\nBecuase it was so powerful, it was hidden away on a mountain that could not be climbed\nBut it’s power brought unwanted attention, and a great war broke out.\nFinally, the leaders of the good side chose a single hero to go and find the Summah and Spich Atip, that hero was “ + name + “\n so ” + nickname + ‘ headed out to find this great power, and stop the war…’)
Now, we’ll give the player their first choice
print(“After hiking through the wastelands for a long time, you come to a massive ravine, there is only a single way across\nA rickety old bridge, taking that could be very dangerous, but… maybe you could jump across?”)
choice1 = input(“[1] Take the bridge [2] Try and jump over”)
#Now we check to see what the player chose
If choice1 == ‘1’:
print(“You slowly walk across the bride, it creakes ominously, then suddenly breaks! You flail through the air before hitting the ground a thousand feet below. Judging by the fact that you hit the ground with the equivalent force of being hit by a cement truck moving at 125 miles an hour, you are dead…”)
#The player lost, so now we’ll boot them out of the program with the exit command
exit()
#Then we check to see if they made the other choice, we can do with with else if, written as elif
elif choice1 == ‘2’:
print(“You make the jump! You see a feather hit the bridge, the weight breakes it and sends it to the bottom of the ravine\nGood thing you didn’t use that bridge.”)
#Now we can continue the story
print(“A few more hours of travel and you come to the unclimbable mountain.”)
choice2 == input(“[1] Give up [2] Try and climb the mountain”)
if choice2 == ‘1’:
print(“You gave up and lost…”)
#now we exit them again
exit()
elif choice2 == ‘1’:
print(“you continue up the mountain. Climbing is hard, but finally you reach the top.\nTo your surprise there is a man standing at the top of the mountain, he is very old.”)
print(“Old Man: Hey “ + nickname)
print(“You: How do you know my name!?!”)
print(“Old Man: Because you have a name tag on…”)
print(“You: Oh, well, were is the Summuh and Spich Atip?”)
print(“Old Man: Summuh and Spich Atip? You must mean the Pita Chips and Hummus”)
print(“You: Pita…chips…humus, what power do those have?”)
print(“Old Man: Pretty simple kid, their organic…”)
#Now let’s clear the screen
os.system(‘clear’)
print(“YOU WON!!!”)
There you have it, a pretty simple choose your own ending story. You can make it as complex or uncomplex as you like.
2. TEXT ENCODER
Ever make secret messages as a kid? I used to. Anyways, here’s the way you can make a program to encode messages! It’s pretty simple. First things first, let’s get the message the user wants to encode, we’ll use input() for that:
message = input(“Message you would like encoded: “)
Now we need to split that string into a list of characters, this part is a bit more complicated.
#We’ll make a function, so we can use it later
def split(x):
return (char for char in x)
#now we’ll call this function with our text
L_message = message.lower() #This way we can lower any of their input
encode = split(l_message)
Now we need to convert the characters into code, well do this with a for loop:
out = []
for x in encode:
if x == ‘a’:
out.append(‘1’)
elif x == ‘b’:
out.append(‘2’)
#And we’ll continue on though this with each letter of the alphabet
Once we’ve encoded the text, we’ll print it back for the user
x = ‘ ‘.join(out)
#this will turn out into a string that we can print
print(x)
And if you want to decode something, it is this same process but in reverse!
3. Guess my Number
Number guessing games are fun and pretty simple, all you need are a few loops. To start, we need to import random.
import random
That is pretty simple. Now we’ll make a list with the numbers were want available for the game
num_list = [1,2,3,4,5,6,7,8,9,10]
Next, we get a random number from the list
num = random.choice(num_list)
Now, we need to ask the user for input, we’ll to this with a while loop
while True:
# We could use guess = input(“What do you think my number is? “), but that would produce a string, and numbers are integers, so we’ll convert the input into an integer
guess = int(input(“What do you think my number is? “))
#Next, we’ll check if that number is equal to the number we picked
if guess == num:
break #this will remove us from the loop, so we can display the win message
else:
print(“Nope, that isn’t it”)
#outside our loop, we’ll have the win message that is displayed if the player gets the correct number.
print(“You won!”)
Have fun with this!
4. Notes
Here is a more advanced project, but still pretty easy. This will be using a txt file to save some notes. The first thing we need to do is to create a txt file in your repl, name it ‘notes.txt’
Now, to open a file in python we use open(‘filename’, type) The type can be ‘r’ for read, or ‘w’ for write. There is another option, but we won’t be using that here. Now, the first thing we are going to do is get what the user would like to save:
message = input(“What would you like to save?”)
Now we’ll open our file and save that text
o = open(‘notes.txt’, ‘w’)
o.write(message)
#this next part is very important, you need to always remember to close your file or what you wrote to it won’t be saved
o.close()
There we go, now the information is in the file. Next, we’ll retrieve it
read = open(‘notes.txt’, ‘r’)
out = read.read()
# now we need to close the file
read.close()
# and now print what we read
print(out)
There we go, that’s how you can open files and close files with python
5. Random Dare Generator
Who doesn’t love a good dare? Here is a program that can generate random dares. The first thing we’ll need to do is as always, import random. Then we’ll make some lists of dares
import random
list1 = [‘jump on’, ‘sit on’, ‘rick roll on’, ‘stop on’, ‘swing on’]
list2 = [‘your cat’, ‘your neighbor’, ‘a dog’, ‘a tree’, ‘a house’]
list3 = [‘your mom’, ‘your best friend’, ‘your dad’, ‘your teacher’]
#now we’ll generate a dare
while True:
if input() == ‘’: #this will trigger if they hit enter
print(“I dare you to “ + random.choice(list1) + ‘ ‘ + random.choice(list2) + ‘ in front of ‘ + random.choice(list3)
Breaking Down Scope, Context, And Closure In JavaScript In Simple Terms.
“JavaScript’s global scope is like a public toilet. You can’t avoid going in there, but try to limit your contact with surfaces when you…
Breaking Down Scope, Context, And Closure In JavaScript In Simple Terms.
Photo by Florian Olivo on Unsplash“JavaScript’s global scope is like a public toilet. You can’t avoid going in there, but try to limit your contact with surfaces when you do.”
― **Dmitry Baranowski**
Here’s another (much) more simple article I wrote on the subject:
Vocab (most of these will be detailed many times over in this article!)
Scope: “Scope is the set of rules that determines where and how a variable (identifier) can be looked-up.” — Kyle Simpson, You Don’t Know JS: Scope & Closure
Function Scope: Every variable defined in a function, is available for the entirety of that function.
Global Scope: “The scope that is visible in all other scopes.” — MDN
Global Variable: A variable defined at the Global Scope.
IIFE: Imediatly-Invoked Function Expression — a function wrapped in () to create an expression, and immediatly followed by a pair of () to invoke that function imediatly.
Closure: “Closures are functions that refer to independent (free) variables. In other words, the function defined in the closure ‘remembers’ the environment in which it was created.” — MDN
Variable Shadowing: “occurs when a variable declared within a certain scope … has the same name as a variable declared in an outer scope.” — Wikipedia
for statement: “The for statement creates a loop that consists of three optional expressions, enclosed in parentheses and separated by semicolons, followed by a statement or a set of statements executed in the loop.” — MDN
initialization: “An expression (including assignment expressions) or variable declaration. Typically used to initialize a counter variable. This expression may optionally declare new variables with the var keyword. These variables are not local to the loop, i.e. they are in the same scope the for loop is in. The result of this expression is discarded.” — MDN
condition: “An expression to be evaluated before each loop iteration. If this expression evaluates to true, statement is executed. This conditional test is optional. If omitted, the condition always evaluates to true. If the expression evaluates to false, execution skips to the first expression following the for construct.” — MDN
final-expression: “An expression to be evaluated at the end of each loop iteration. This occurs before the next evaluation of condition. Generally used to update or increment the counter variable.” — MDN
statement: “A statement that is executed as long as the condition evaluates to true. To execute multiple statements within the loop, use a block statement ({ … }) to group those statements.” — MDN
Arrays: “JavaScript arrays are used to store multiple values in a single variable.” — W3Schools
I am going to try something new this article… it’s called spaced repetition.
“Spaced repetition is an evidence-based learning technique that is usually performed with flashcards. Newly introduced and more difficult flashcards are shown more frequently, while older and less difficult flashcards are shown less frequently in order to exploit the psychological spacing effect. The use of spaced repetition has been proven to increase rate of learning.”
Open it in another tab… it will only display the html file that existed when I pasted it into this article… for access to the JavaScript file and the most up to date html page you need to Open the sandbox… feel free to create a fork of it if you like!
SCOPE:
The scope of a program in JS is the set of variables that are available for use within the program.
Scope in JavaScript defines which variables and functions you have access to, depending on where you are (a physical position) within your code.
The current context of execution. The context in which values and expressions are “visible” or can be referenced. If a variable or other expression is not “in the current scope,” then it is unavailable for use. Scopes can also be layered in a hierarchy, so that child scopes have access to parent scopes, but not vice versa.
- A function serves as a **closure** in JavaScript, and thus creates a scope, so that (for example) a variable defined exclusively within the function cannot be accessed from outside the function or within other functions:https://developer.mozilla.org/en-US/docs/Glossary/Scope
- In computer programming, the **scope** of a name binding — an association of a name to an entity, such as a variable — is the part of a program where the name binding is valid, that is where the name can be used to refer to the entity. In other parts of the program the name may refer to a different entity (it may have a different binding), or to nothing at all (it may be unbound). The scope of a name binding is also known as the **visibility** of an entity, particularly in older or more technical literature — this is from the perspective of the referenced entity, not the referencing name:https://en.wikipedia.org/wiki/Scope_(computer_science)
Advantages of utilizing scope
Security : Adds security by ensuring variables can only be access by pre-defined parts of our program.
Lexical scope is the ability of the inner function to access the outer scope in which it is defined.
Lexing Time : When you run a piece of JS code that is parsed before it is run.
Lexical environment is a place where variables and functions live during the program execution.
Different Variables in JavaScript
A variable always evaluates to the value it contains no matter how you declare it.
The different ways to declare variables
let : can be re-assigned; block-scoped.
const : no re-assignment; block scoped.
var : May or may not be re-assigned; scoped to a function.
Hoisting and Scoping with Variables
Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their scope before code execution.
I am afraid … sometimes… one simply does… at least analogous-ley### !!!Only function declarations are hoisted in JavaScript, function expressions are not hoisted!!!
&&
!!! Only variables declared with var are hoisted!!!!
The first Execution Context ( a word that we don’t have to worry about the exact meaning of yet) that gets created when the JavaScript engine runs your code is called the “Global Execution Context”.
Initially this Execution Context will consist of two things —
a global object
and
a variable calledthis.
By default thethis keyword will act as a reference to global object which will be window if you’re running JavaScript in the browser or global if you’re running it in a Node environment.
REMEMBER:
Node: this references a global object called window (like the window that comprises the content of a tab in chrome)
Browsers:this references a global object called global
Above we can see that even without any code, the Global Execution Context will still consist of two things — `window` and `this`. This is the Global Execution Context in its most basic form.
Let’s step things up and see what happens when we start actually adding code to our program. Let’s start with adding a few variables.
The key take away is that each Execution Context has two separate phases, a `Creation` phase and an `Execution` phase and each phase has its own unique responsibilities.
Exaction Context:
Execution Context ≠(NOT EQUAL)≠≠≠Scope
The global execution context is created before any code is executed.
Whenever a function is executed invoked (this means the function is told to run… i.e. after the doSomething function has been declared … calling ‘doSomething()’, a new execution context gets created.
Every execution context provides this keyword, which points to an object to which the current code that’s being executed belongs.
In JavaScript, execution context is an abstract concept that holds information about the environment within which the current code is being executed.
Remember: the JavaScript engine creates the global execution context before it starts to execute any code. From that point on, a new execution context gets created every time a function is executed, as the engine parses through your code. In fact, the global execution context is nothing special. It’s just like any other execution context, except that it gets created by default.
In the Global Creation phase, the JavaScript engine will
Create a global object.
Create an object called “this”.
Set up memory space for variables and functions.
Assign variable declarations a default value of “undefined” while placing any function declarations in memory.
It’s not until theExecutionphase where the JavaScript engine starts running your code line by line and executing it.
We can see this flow from Creationphase toExecutionphase in the GIF below. 🠗🠗🠗🠗🠗🠗
During the `Creation` phase:
The JavaScript engine said ‘let there bewindowandthis‘
and there was window and this… and it was good
Once the window and this are created;
Variable declarations are assigned a default value of undefined
Any function declarations (getUser) are placed entirely into memory.
Exaction Phase:
Then once we enter the Execution phase, the JavaScript engine starts executing the code line by line and assigns the real values to the variables already living in memory.
Function-Scoped Variables
Function scope means that parameters and variables defined in a function are visible everywhere within the function, but are not visible outside of the function.
Consider the next function that auto-executes, called IIFE.
(function autoexecute() {
let x = 1;
})();
console.log(x);
//Uncaught ReferenceError: x is not defined
IIFE stands for Immediately Invoked Function Expression and is a function that runs immediately after its definition.
Variables declared with var have only function scope. Even more, variables declared with var are hoisted to the top of their scope. This way they can be accessed before being declared. Take a look at the code below:
function doSomething(){
console.log(x);
var x = 1;
}
doSomething(); //undefined
This does not happen for let. A variable declared with let can be accessed only after its definition.
function doSomething(){
console.log(x);
let x = 1;
}
doSomething();
//Uncaught ReferenceError: x is not defined
A variable declared with var can be re-declared multiple times in the same scope. The following code is just fine:
function doSomething(){
var x = 1
var x = 2;
console.log(x);
}
doSomething();
Variables declared with let or const cannot be re-declared in the same scope:
function doSomething(){
let x = 1
let x = 2;
}
//Uncaught SyntaxError: Identifier 'x' has already been declared
Maybe we don’t even have to care about this, as var has started to become obsolete.
Only the var keyword creates function-scoped variables (however a var declared variable may be globally scoped if it is declared outside of a nested function in the global scope), this means our declared var keyword variable will be confined to the scope of our current function.
Why you shouldn’t use var:
No error is thrown if you declare the same variable twice using var (conversely, both let and const will throw an error if a variable is declared twice)
Variables declared with var are not block scoped (although they are function scoped), while with let and const they are. This is important because what’s the point of block scoping if you’re not going to use it. So using var in this context would require a situation in which a variable declared inside a function would need to be used in the global scope. I’m not able to think of any situations where that would be absolutely necessary, but perhaps they exist.
REMEMBER:
Var===🗑🚮👎🏽🤮
My response to anyone who might comment in defense of using varAlso on a more serious note… if you have var in your projects in 2021 …hiring managers who peek at your projects to see your code quality will assume it was copy pasted from someone else’s legacy code circa 2014.
**Hoisting with function-scoped variables**
function test() {
// var hoistedVar;
console.log(hoistedVar); // => undefined
var hoistedVar = 10;
}
Even though we initially declared & initialized our variable underneath the console.log var variables are “hoisted” to the top, but only in declaration (default value undefined until the exaction arrives at the line on which it was initialized.
Scope Spaced Repetition:
Scope is defined as which variables we currently have access to and where. So far in this course, we have mostly been working in global scope, in that we can access any variable we have created, anywhere in our code. There are a couple different levels of scope: block level scope is used in if statements and for loops. In block level scope, a variable declared using either let or const is only available within the statement or loop (like i in a for loop). Similarly, there is function scope, where temperature exists inside the function, but not elsewhere in your code file.
helloWorld(); // prints 'Hello World!' to the consolefunction helloWorld(){
console.log('Hello World!');
}
Function declarations are added to the memory during the compile stage, so we are able to access it in our code before the actual function declaration
When the JavaScript engine encounters a call to helloWorld(), it will look into the lexical environment, finds the function and will be able to execute it.
Hoisting Function Expressions
Only function declarations are hoisted in JavaScript, function expressions are not hoisted. For example: this code won’t work.
helloWorld(); // TypeError: helloWorld is not a functionvar helloWorld = function(){
console.log('Hello World!');
}
As JavaScript only hoist declarations, not initializations (assignments), so the helloWorld will be treated as a variable, not as a function. Because helloWorld is a var variable, so the engine will assign is the undefined value during hoisting.
So this code will work.
var helloWorld = function(){
console.log('Hello World!'); prints 'Hello World!'
}helloWorld();
Hoisting var variables:
Let’s look at some examples to understand hoisting in case of var variables.
console.log(a); // outputs 'undefined'
var a = 3;
We expected 3 but instead got undefined. Why?
Remember JavaScript only hoist declarations, not initializations*.* That is, during compile time, JavaScript only stores function and variable declarations in the memory, not their assignments (value).
But whyundefined?
When JavaScript engine finds a var variable declaration during the compile phase, it will add that variable to the lexical environment and initialize it with undefined and later during the execution when it reaches the line where the actual assignment is done in the code, it will assign that value to the variable.
let and const in their block scopes do not get their declarations hoisted.
Instead they are not initialized until their definitions are evaluated instead of undefined we will get an error.
Temporal Dead Zone** : The time before a let or const variable is declared.**
Function Scope vs Block Scope
The downside of the flexibility of var is that it can easily overwrite previously declared variables.
The block-scope limitations of let and const were introduced to easily avoid accidentally overwriting variable values.
Global Variables
Any variables declared without a declaration term will be considered global scope.
Every time a variable is declared on the global scope, the change of collision increases.
Use the proper declarations to manage your code: Avoid being a sloppy programmer!
Variables defined outside any function, block, or module scope have global scope. Variables in global scope can be accessed from everywhere in the application.
When a module system is enabled it’s harder to make global variables, but one can still do it. By defining a variable in HTML, outside any function, a global variable can be created:
When there is no module system in place, it is a lot easier to create global variables. A variable declared outside any function, in any file, is a global variable.
Global variables are available for the lifetime of the application.
Another way for creating a global variable is to use the window global object anywhere in the application:
window.GLOBAL_DATA = { value: 1 };
At this point, the GLOBAL_DATA variable is visible everywhere.
console.log(GLOBAL_DATA)
As you can imagine these practices are bad practices.
*Module scope
Before modules, a variable declared outside any function was a global variable. In modules, a variable declared outside any function is hidden and not available to other modules unless it is explicitly exported.
Exporting makes a function or object available to other modules. In the next example, I export a function from the sequence.js module file:
// in sequence.js
export { sequence, toList, take };
Importing makes a function or object, from other modules, available to the current module.
import { sequence, toList, toList } from "./sequence";
In a way, we can imagine a module as a self-executing function that takes the import data as inputs and returns the export data.
Closure : The combination of a function and the lexical environment within which that function is declared.
Use : A closure is when an inner function uses, or changes, variables in an outer function.
Very important for creativity, flexibility, and security of your code.
Lexical Environment : Consists of any variables available within the scope in which a closure was declared (local inner, outer, and global).
Closures and Scope Basic Closure Rules:
- Closures have access to all variables in it’s lexical environment.
- A closure will keep reference to all the variables when it was defined **even if the outer function has returned**.
Applications of Closures
Private State
JS does not have a way of declaring a function as exclusively private, however we can use closures to make a private state.
Passing Arguments Implicitly
We can use closures to pass down arguments to helper functions.
OVERVIEW
Let’s look at the Window/Console in a browser/node environment. Type window to your chrome console. JavaScript is executed in this context, also known as the global scope.
There are two types of scope in javascript, Global Vs. Local and that this is important to understand in terms of a computer program written in JavaScript.
When a function is declared and created, a new scope is also created. Any variables declared within that function's scope will be enclosed in a lexical/private scope that belongs to that function. Also, it is important to remember that functions look outward for context. If some variable isn't defined in a function's scope, the function will look outside the scope chain and search for a variable being referenced in the outer scope. This is what closure is all about.
In it’s most simplest of forms this is a closure:
const foo = 'bar';
function returnFoo () {
return foo;
}
returnFoo();
// -> reaches outside its scope to find foo because it doesn't exist inside of return Foo's scope when foo is referenced.
The function body (code inside returnFoo) has access to the outside scope (code outside of returnFoo) and can access that ‘foo’ variable.
Not much different than the idea presented above, but the thought remains the same. When ‘greet’ is called, it has no context in its local scope of finding lastName so, it looks outside its scope to find it and use the lastName that is found there.
Let’s take this a step further. In the given examples, we’ve seen that we have created two environments for our code. The first would be the global environment where lastName and greet exist. The second would be the local environment where the alert function is called. Let's represent those two environments like this:
### What is a closure?
A closure is an inner function that has access to the outer (enclosing) function’s variables — scope chain. The closure has three scope chains: it has access to its own scope (variables defined between its curly brackets), it has access to the outer function’s variables, and it has access to the global variables.
The inner function has access not only to the outer function’s variables, but also to the outer function’s parameters. Note that the inner function cannot call the outer function’s arguments object, however, even though it can call the outer function’s parameters directly.
You create a closure by adding a function inside another function.
A Basic Example:
Scope chain
Every scope has a link to the parent scope. When a variable is used, JavaScript looks down the scope chain until it either finds the requested variable or until it reaches the global scope, which is the end of the scope chain.
Context in JavaScript
Scope : Refers to the visibility and availability of variables.
Context : Refers to the value of the this keyword when code is executed.
Every function invocation has both a scope and a context. Even though technically functions are objects in JS, you can think of scope as pertaining to functions in which a variable was defined, and context as the object on which some variable or method (function) may exist. Scope describes a function has access to when it is invoked (unique to each invocation). Context is always the value of the this keyword which in turn is a reference to the object that a piece of code exists within.
Context is most often determined by how a function is invoked. When a function is called as a method of an object, this is set to the object the method is called on:
this** ?**
This : Keyword that exists in every function and evaluates to the object that is currently invoking that function.
Method-Style Invocation : syntax goes like object.method(arg). (i.e. array.push, str.toUpperCase()
Context refers to the value of this within a function and this refers to where a function is invoked.
Issues with Scope and Context
If this is called using normal function style invocation, our output will be the contents of the global object.
When Methods have an Unexpected Context
In the above example we get undefined when we assign our this function to a variable bc there is no obj to reference except the global one!
Strictly Protecting the Global Object
We can run JS in strict mode by tagging use strict at the top of our program.
If we try to invoke this on our global function in strict mode we will no longer be able to access it and instead just get undefined.
Changing Context using Bind
“The simplest use of bind() is to make a function that, no matter how it is called, is called with a particular this value".
Binding with Arguments
We can also use bind() to bind arguments to a function.
Arrow Functions (Fat Arrows)
side note … if you don’t know what this means ignore it… but if you write an arrow function that utilizes an implicit return… that’s roughly equivalent to what is referred to as a lambda function in other languages.
=> : A more concise way of declaring a function and also considers the behavior of this and context.
Arrow Functions Solving Problems
As you can see the arrow function is shorter and easier to read.
Anatomy of an Arrow Function
If there is only a single parameter there is no need to add parenthesis before the arrow function.
However if there are zero parameters then you must add an empty set of parentheses.
Single Expression Arrow Functions
Arrow functions, unlike normal functions, carry over context, binding this lexically.
Value of this inside an arrow function is not dependent on how it is invoked.
Because arrow functions already have a bound context, you can’t reassign this.
Phewh… that’s a lot… let’s go over some of that again!
Block Scope Review:
Block scope is defined with curly braces. It is separated by { and }.
Variables declared with let and const can have block scope. They can only be accessed in the block in which they are defined.
Consider the next code that emphasizes let block scope:
let x = 1;
{
let x = 2;
}
console.log(x); //1
In contrast, the var declaration has no block scope:
var x = 1;
{
var x = 2;
}
console.log(x); //2
Closures Spaced Repetition
1. Closures have access to the outer function’s variable even after the outer function returns:
One of the most important features with closures is that the inner function still has access to the outer function’s variables even after the outer function has returned.
When functions in JavaScript execute, they use the same scope chain that was in effect when they were created.
This means that even after the outer function has returned, the inner function still has access to the outer function’s variables. Therefore, you can call the inner function later in your program.
See here:
2. Closures store references to the outer function’s variables:
They do not store the actual value.
Closures get more interesting when the value of the outer function’s variable changes before the closure is called.
And this powerful feature can be harnessed in creative ways…namely private variables.
3. Closures Gone Bad
Because closures have access to the updated values of the outer function’s variables, they can also lead to bugs when the outer function’s variable changes with a for loop.
For example:
Cclosure is a word we use to refer to the context of a given function. Normally our function starts from scratch every time we run it.
However, if we were to return a function from addOne() that referenced counter, counter would become part of the context of that new function, even after addOne() finishes executing. This is easier to see in code than to explain in words:
This works! we only instantiate counter when createAdder() is called, but it's value gets updated whenever the function it returns is called.
We say that this inner function is closed around the variable counter
Definition*: (According to MDN) A closure is the combination of a function and the lexical environment within which that function was declared.*
Global Scope Spaced Repetition:
Scope in JavaScript works like it does in most languages. If something is defined at the root level, that’s global scope — we can access (and modify) that variable from anywhere. If it’s a function we defined, we can call it from anywhere.
The Problem with Global Scope
So it seems we should declare all of our variables at the global scope.
Why could this be a problem?
It seems reasonable to want counter to only be accessed/modified through our addOne() function, but if our variable is defined within the global scope, it's simply not so.
This may not seem like a major concern — we can just make sure we don’t access it directly.
We need some form of encapsulation — i.e. the data our function relies on is completely contained within the logic of that function
Inner Scope
OK, this seems to work as expected, however
What about inside of our addOne() function?
Every function creates it’s own local scope.
Compared to it’s context (i.e. where our function is defined), we call this the inner scope
Our function can access/modify anything outside of it’s scope, so the body of our function, { counter++; }, has an effect that persists in the outside scope.
What about the other way around…Can global scope modify inner scope?
Because counter is defined within our function's scope, it doesn't exist within the global scope, so referencing it there doesn't make sense.
Context vs. Scope
The first important thing to clear up is that context and scope are not the same. I have noticed many developers over the years often confuse the two terms (myself included), incorrectly describing one for the other.
Every function invocation has both a scope and a context associated with it.
To condense it down to a single concept, scope is function-based while context is object-based.
In other words, scope pertains to the variable access of a function when it is invoked and is unique to each invocation. Context is always the value of the this keyword which is a reference to the object that "owns" the currently executing code.
Variable Scope
A variable can be defined in either local or global scope, which establishes the variables’ accessibility from different scopes during runtime.
Local variables exist only within the function body of which they are defined and will have a different scope for every call of that function. There it is subject for value assignment, retrieval, and manipulation only within that call and is not accessible outside of that scope.
ECMAScript 6 (ES6/ES2015) introduced the let and const keywords that support the declaration of block scope local variables.
This means the variable will be confined to the scope of a block that it is defined in, such as an if statement or for loop and will not be accessible outside of the opening and closing curly braces of the block.
This is contrary to var declarations which are accessible outside blocks they are defined in.
The difference between let and const is that a const declaration is, as the name implies, constant - a read-only reference to a value.
This does not mean the value is immutable, just that the variable identifier cannot be reassigned.
Closure Spaced Repetition:
recall:
Lexical scope is the ability for a function scope to access variables from the parent scope. We call the child function to be lexically bound by that of the parent function.
### AND
A lexical environment is an abstraction that holds identifier-variable mapping. Identifier refers to the name of variables/functions, and the variable is the reference to actual object [including function object] or primitive value.
This is similar to an object with a method on it…
In the picture below… sayHi (and name) are identifiers and the function and (string “bryan”) are variable values.
#### Accessing variables outside of the immediate lexical scope creates a closure.
A closure is to put it simply, when a nested function is defined inside of another function gains access to the outer functions variables.
Returning the nested function from the ‘parent function’ that enclosed the nested function definition, allows you to maintain access to the local variables, (arguments, and potentially even more inner function declarations) of its outer function… without exposing the variables or arguments of outer function…. to any of the other functions that were not declared inside of it.
What is “this” Context
Context is most often determined by how a function is invoked.
When a function is called as a method of an object, this is set to the object the method is called on:
The same principle applies when invoking a function with the new operator to create an instance of an object.
When invoked in this manner, the value of this within the scope of the function will be set to the newly created instance:
When called as an unbound function, this will default to the global context or window object in the browser. However, if the function is executed in strict mode, the context will default to undefined.
Execution Context Spaced Repetition:
JavaScript is a single threaded language, meaning only one task can be executed at a time. When the JavaScript interpreter initially executes code, it first enters into a global execution context by default. Each invocation of a function from this point on will result in the creation of a new execution context.
This is where confusion often sets in, the term “execution context” is actually for all intents and purposes referring more to scope and not context.
It is an unfortunate naming convention, however it is the terminology as defined by the ECMAScript specification, so we’re kind of stuck with it.
Each time a new execution context is created it is appended to the top of the execution stack (call stack).
The browser will always execute the current execution context that is atop the execution stack. Once completed, it will be removed from the top of the stack and control will return to the execution context below.
An execution context can be divided into a creation and execution phase. In the creation phase, the interpreter will first create a variable object that is composed of all the variables, function declarations, and arguments defined inside the execution context.
From there the scope chain is initialized next, and the value of this is determined last. Then in the execution phase, code is interpreted and executed.
#### Q1. In the following example, which selector has the highest specificity ranking for selecting the anchor link element?
ul li a
a
.example a
div a
[x] .example a
[ ] div a
[ ] a
[ ] ul li a
Q2. Using an attribute selector, how would you select an <a> element with a "title" attribute?
[x] a[title]{…}
[ ] a > title {…}
[ ] a.title {…}
[ ] a=title {…}
Q3. CSS grid and flexbox are now becoming a more popular way to create page layouts. However, floats are still commonly used, especially when working with an older code base, or it you need to support older browser version. What are two valid techniques used to clear floats?
[ ] Use the “clearfix hack” on the floated element and add a float to the parent element.
[ ] Use the overflow property on the floated element or the “clearfix hack” on either the floated or parent element.
[ ] Use the “clearfix hack” on the floated element or the overflow property on the parent element.
[x] Use the “clearfix hack” on the parent element or use the overflow property with a value other than “visible.”
Q4. What element(s) do the following selectors match to?
1) .nav {...}2) nav {...}3) #nav {...}
[ ]
1. An element with an ID of "nav"
2. A nav element
3. An element with a class of "nav"
[ ]
They all target the same nav element.
[x]
1. An element with an class of "nav"
2. A nav element
3. An element with a id of "nav"
[ ]
1. An element with an class of "nav"
2. A nav element
3. An div with a id of "nav"
Q5. When adding transparency styles, what is the difference between using the opacity property versus the background property with an rgba() value?
[ ] Opacity specifies the level of transparency of the child elements. Background with an rgba() value applies transparency to the background color only.
[ ] Opacity applies transparency to the background color only. Background with an rgba() value specifies the level of transparency of an element, as a whole, including its content.
[x] Opacity specifies the level of transparency of an element, including its content. Background with an rgba() value applies transparency to the background color only.
[ ] Opacity applies transparency to the parent and child elements. Background with an rgba() value specifies the level of transparency of the parent element only.
Q6. What is true of block and inline elements? (Alternative: Which statement about block and inline elements is true?)
[ ] By default, block elements are the same height and width as the content container between their tags; inline elements span the entire width of its container.
[x] By default, block elements span the entire width of its container; inline elements are the same height and width as the content contained between their tags.
[ ] A <nav> element is an example of an inline element. <header> is an example of a block element.
[ ] A <span> is an example of a block element. <div> is an example of an inline element.
Q7. CSS grid introduced a new length unit, fr, to create flexible grid tracks. Referring to the code sample below, what will the widths of the three columns be?
[ ] The first column will have a width of 50px. The second column will be 50px wide and the third column will be 100px wide.
[x] The first column will have a width of 50px. The second column will be 150px wide and the third column will be 300px wide.
[ ] The first column will have a width of 50px. The second column will be 300px wide and the third column will be 150px wide.
[ ] The first column will have a width of 50px. The second column will be 500px wide and the third column will be 1000px wide.
Q8. What is the line-height property primarily used for?
[x] to control the height of the space between two lines of content
[ ] to control the height of the space between heading elements
[ ] to control the height of the character size
[ ] to control the width of the space between characters
Q9. Three of these choices are true about class selectors. Which is NOT true?
[ ] Multiple classes can be used within the same element.
[ ] The same class can be used multiple times per page.
[ ] Class selectors with a leading period
[x] Classes can be used multiple times per page but not within the same element.
Q10. There are many properties that can be used to align elements and create page layouts such as float, position, flexbox and grid. Of these four properties, which one should be used to align a global navigation bar which stays fixed at the top of the page?
[x] position
[ ] flexbox
[ ] grid
[ ] float
Q11. In the shorthand example below, which individual background properties are represented?
background: blue url(image.jpg) no-repeat scroll 0px 0px;
Q12. In the following example, according to cascading and specificity rules, what color will the link be?
.example {
color: yellow;
}
ul li a {
color: blue;
}
ul a {
color: green;
}
a {
color: red;
}
<ul>
<li><a href="#" class="example">link</a></li>
<li>list item</li>
<li>list item</li>
</ul>
[ ] green
[x] yellow
[ ] blue
[ ] red
Q13. When elements overlap, they are ordered on the z-axis (i.e., which element covers another). The z-index property can be used to specify the z-order of overlapping elements. Which set of statements about the z-index property are true?
[x] Larger z-index values appear on top of elements with a lower z-index value. Negative and positive numbers can be used. z-index can only be used on positioned elements.
[ ] Smaller z-index values appear on top of elements with a larger z-index value. Negative and positive numbers can be used. z-index must also be used with positioned elements.
[ ] Larger z-index values appear on top of elements with a lower z-index value. Only positive numbers can be used. z-index must also be used with positioned elements.
[ ] Smaller z-index values appear on top of elements with a larger z-index value. Negative and positive numbers can be used. z-index can be used with or without positioned elements.
Q14. What is the difference between the following line-height settings?
line-height: 20px
line-height: 2
[x] The value of 20px will set the line-height to 20px. The value of 2 will set the line-height to twice the size of the corresponding font-size value.
[ ] The value of 20px will set the line-height to 20px. The value of 2 is not valid.
[ ] The value of 20px will set the line-height to 20px. The value of 2 will default to a value of 2px.
[ ] The value of 20px will set the line-height to 20px. The value of 2 will set the line-height to 20% of the corresponding font-size value.
Q15. In the following example, what color will paragraph one and paragraph two be? (Alternative: In this example, what color will paragraphs one and two be?)
<section>
<p>paragraph one</p>
</section>
<p>paragraph two</p>
section p {
color: red;
}
section + p {
color: blue;
}
[ ] Paragraph one will be blue, paragraph two will be red.
[ ] Both paragraphs will be blue.
[x] Paragraphs one will be red, paragraph two will be blue.
[ ] Both paragraphs will be red.
Q16.What are three valid ways of adding CSS to an HTML page?
[ ]
1. External; CSS is written in a separate file.
2. Inline; CSS is added to the <head> of the HTML page.
3. Internal; CSS is included within the HTML tags.
[ ]
1. External; CSS is written in a separate file and is linked within the <header> element of the HTML file.
2. Inline; CSS is added to the HTML tag.
3. Internal; CSS is included within the <header> element of the HTML file.
[ ]
1. External; CSS is written in a separate file and is linked within the <head> element of the HTML file.
2. Internal; CSS is included within the <header> element of the HTML file.
3. Inline; CSS is added to the HTML tag.
[x]
1. External; CSS is written in a separate file and is linked within the <head> element of the HTML file .
2. Inline; CSS is added to the HTML tag.
3. Internal; CSS is included within the <head> element of the HTML file.
Q17. Which of the following is true of the SVG image format? (Alternative: Which statement about the SVG image format is true?)
[ ] CSS can be applied to SVGs but JavaScript cannot be.
[ ] SVGs work best for creating 3D graphics.
[x] SVGs can be created as a vector graphic or coded using SVG specific elements such as <svg>, <line>, and <ellipse>.
[ ] SVGs are a HAML-based markup language for creating vector graphics.
Q18. In the example below, when will the color pink be applied to the anchor element?
a:active {
color: pink;
}
[ ] The color of the link will display as pink after its been clicked or if the mouse is hovering over the link.
[ ] The color of the link will display as pink on mouse hover.
[x] The color of the link will display as pink while the link is being clicked but before the mouse click is released.
[ ] The color of the link will display as pink before it has been clicked.
Q19. To change the color of an SVG using CSS, which property is used?
[ ] Use background-fill to set the color inside the object and stroke or border to set the color of the border.
[ ] The color cannot be changed with CSS.
[ ] Use fill or background to set the color inside the object and stroke to set the color of the border.
[x] Use fill to set the color inside the object and stroke to set the color of the border.
Q20. When using position: fixed, what will the element always be positioned relative to?
[ ] the closest element with position: relative
[x] the viewport
[ ] the parent element
[ ] the wrapper element
Q21. By default, a background image will repeat \_\_\_
[ ] only if the background-repeat property is set to repeat
[x] indefinitely, vertically, and horizontally
[ ] indefinitely on the horizontal axis only
[ ] once, on the x and y axis
Q22. When using media queries, media types are used to target a device category. Which choice lists current valid media types?
[ ] print, screen, aural
[ ] print, screen, television
[x] print, screen, speech
[ ] print, speech, device
Q23. How would you make the first letter of every paragraph on the page red?
[x] p::first-letter { color: red; }
[ ] p:first-letter { color: red; }
[ ] first-letter::p { color: red; }
[ ] first-letter:p { color: red; }
Q24. In this example, what is the selector, property, and value?
p {
color: #000000;
}
[ ]
"p" is the selector
"#000000" is the property
"color" is the value
[x]
"p" is the selector
"color" is the property
"#000000" is the value
[ ]
"color" is the selector
"#000000" is the property
"#p" is the value
[ ]
"color" is the selector
"p" is the property
"#000000" is the value
Q25. What is the rem unit based on?
[ ] The rem unit is relative to the font-size of the p element.
[ ] You have to set the value for the rem unit by writing a declaration such as rem { font-size: 1 Spx; }
[ ] The rem unit is relative to the font-size of the containing (parent) element.
[x] The rem unit is relative to the font-size of the root element of the page.
Q26.Which of these would give a block element rounded corners?
[ ] corner-curve: 10px
[ ] border-corner: 10px
[x] border-radius: 10px
[ ] corner-radius: 10px
Q27. In the following media query example, what conditions are being targeted?
@media (min-width: 1024px), screen and (orientation: landscape) { … }
[x] The rule will apply to a device that has either a width of 1024px or wider, or is a screen device in landscape mode.
[ ] The rule will apply to a device that has a width of 1024px or narrower and is a screen device in landscape mode.
[ ] The rule will apply to a device that has a width of 1024px or wider and is a screen device in landscape mode.
[ ] The rule will apply to a device that has a width of 1024px or narrower, or is a screen device in landscape mode.
Q28. CSS transform properties are used to change the shape and position of the selected objects. The transform-origin property specifies the location of the element’s transformation origin. By default, what is the location of the origin?
[x] the top left corner of the element
[ ] the center of the element
[ ] the top right corner of the element
[ ] the bottom left of the element
Q29. Which of the following is not a valid color value?
[ ] color: #000
[ ] color: rgb(0,0,0)
[ ] color: #000000
[x] color: 000000
Q30. What is the vertical gap between the two elements below?
Q31. When using the Flexbox method, what property and value is used to display flex items in a column?
[x] flex-flow: column; or flex-direction: column
[ ] flex-flow: column;
[ ] flex-column: auto;
[ ] flex-direction: column;
Q32. Which type of declaration will take precedence?
[ ] any declarations in user-agent stylesheets
[x] important declarations in user stylesheets
[ ] normal declarations in author stylesheets
[ ] important declarations in author stylesheets
Q33. The flex-direction property is used to specify the direction that flex items are displayed. What are the values used to specify the direction of the items in the following examples?
[x] Example 1: flex-direction: row; Example 2: flex-direction: row-reverse; Example 3: flex-direction: column; Example 4: flex-direction: column-reverse;
[ ] Example 1: flex-direction: row-reverse; Example 2: flex-direction: row; Example 3: flex-direction: column-reverse; Example 4: flex-direction: column;
[ ] Example 1: flex-direction: row; Example 2: flex-direction: row-reverse; Example 3: flex-direction: column; Example 4: flex-direction: reverse-column;
[ ] Example 1: flex-direction: column; Example 2: flex-direction: column-reverse; Example 3: flex-direction: row; Example 4: flex-direction: row-reverse;
Q34. There are two sibling combinators that can be used to select elements contained within the same parent element; the general sibling combinator (~) and the adjacent sibling combinator (+). Referring to example below, which elements will the styles be applied to?
[ ] Paragraphs 2 and 3 will be blue. The h2 and paragraph 2 will have a beige background.
[x] Paragraphs 2, and 3 will be blue, and paragraph 2 will have a beige background.
[x] Paragraphs 2 and 3 will be blue. Paragraph 2 will have a beige background.
[ ] Paragraph 2 will be blue. Paragraphs 2 and 3 will have a beige background.
Q35. When using flexbox, the “justify-content” property can be used to distribute the space between the flex items along the main axis. Which value should be used to evenly distribute the flex items within the container shown below?
[x] justify-content: space-around;
[ ] justify-content: center;
[ ] justify-content: auto;
[ ] justify-content: space-between;
Q36. There are many advantages to using icon fonts. What is one of those advantages?
[ ] Icon fonts increase accessibility.
[ ] Icon fonts can be used to replace custom fonts.
[x] Icon fonts can be styled with typography related properties such as font-size and color.
[ ] Icon fonts are also web safe fonts.
Q37. What is the difference between display:none and visibility:hidden?
[ ] Both will hide the element on the page, but display:none has greater browser support. visibility:hidden is a new property and does not have the best browser support
[ ] display:none hides the elements but maintains the space it previously occupied. visibility:hidden will hide the element from view and remove it from the normal flow of the document
[x] display:none hides the element from view and removes it from the normal flow of the document. visibility:hidden will hide the element but maintains the space it previously occupied.
[ ] There is no difference; both will hide the element on the page
Q38. What selector and property would you use to scale an element to be 50% smaller on hover?
[ ] element:hover {scale: 0.5;}
[x] element:hover {transform: scale(0.5);}
[ ] element:hover {scale: 50%;}
[ ] element:hover {transform: scale(50%);}
Q39. Which statement regarding icon fonts is true?
[ ] Icon fonts can be inserted only using JavaScript.
[ ] Icon fonts are inserted as inline images.
[ ] Icon fonts require browser extensions.
[x] Icon fonts can be styled with typography-related properties such as font-size and color.
Q40. The values for the font-weight property can be keywords or numbers. For each numbered value below, what is the associated keyword?
font-weight: 400; font-weight: 700;
[ ] bold; normal
[x] normal; bold
[ ] light; normal
[ ] normal; bolder
Q41. If the width of the container is 500 pixels, what would the width of the three columns be in this layout?
Q42. Using the :nth-child pseudo class, what would be the most efficient way to style every third item in a list, no matter how many items are present, starting with item 2?
Q53. What is the ::placeholder pseudo-element used for?
[x] It is used to format the appearance of placeholder text within a form control.
[ ] It specifies the default input text for a form control.
[ ] It writes text content into a hyperlink tooltip.
[ ] It writes text content into any page element.
Q54. Which statement is true of the single colon (:) or double colon (::) notations for pseudo-elements-for example, ::before and :before?
[ ] All browsers support single and double colons for new and older pseudo-elements. So you can use either but it is convention to use single colons for consistency.
[ ] In CSS3, the double colon notation (::) was introduced to create a consistency between pseudo-elements from pseudo-classes. For newer browsers, use the double colon notation. For IE8 and below, using single colon notation (:).
[ ] Only the new CSS3 pseudo-elements require the double colon notation while the CSS2 pseudo-elements do not.
[x] In CSS3, the double colon notation (::) was introduced to differentiate pseudo-elements from pseudo-classes. However, modern browsers support both formats. Older browsers such as IE8 and below do not.
Q55. Which choice is not valid value for the font-style property?
[ ] normal
[ ] italic
[x] none
[ ] oblique
Q56. When would you use the @font-face method?
[ ] to set the font size of the text
[x] to load custom fonts into stylesheet
[ ] to change the name of the font declared in the font-family
[ ] to set the color of the text
Q57. When elements within a container overlap, the z-index property can be used to indicate how those items are stacked on top of each other. Which set of statements is true?
[x]
1. Larger z-index values appear on top elements with a lower z-index value.
2. Negative and positive number can be used.
3. z-index can be used only on positioned elements.
[ ]
1. Smaller z-index values appear on top of elements with a larger z-index value.
2. Negative and positive numbers can be used.
3. z-index can be used with or without positioned elements.
[ ]
1. Smaller z-index values appear on top of elements with a larger z-index value.
2. Negative and positive number can be used.
3. z-index must also be used with positioned elements.
[ ]
1. Larger z-index values appear on top of elements with a lower z-index value.
2. Only positive number can be used.
3. z-index must also be used with positioned elements.
Q58. You have a large image that needs to fit into a 400 x 200 pixel area. What should you resize the image to if your users are using Retina displays?
[ ] 2000 x 1400 pixels
[ ] 200 x 100 pixels
[x] 800 x 400 pixels
[ ] 400 x 200 pixels
Q59. In Chrome’s Developer Tools view, where are the default styles listed?
[x] under the User Agent Stylesheet section on the right
[ ] in the third panel under the Layout tab
[ ] under the HTML view on the left
[ ] in the middle panel
Q60. While HTML controls document structure, CSS controls _.
[ ] semantic meaning
[ ] content meaning
[ ] document structure
[x] content appearance
Q61. What is the recommended name you should give the folder that holds your project’s images?
[x] images
[ ] #images
[ ] Images
[ ] my images
Q62. What is an advantage of using inline CSS?
[ ] It is easier to manage.
[x] It is easier to add multiple styles through it.
[ ] It can be used to quickly test local CSS overrides.
[ ] It reduces conflict with other CSS definition methods.
Q63.Which W3C status code represents a CSS specification that is fully implemented by modern browsers?
[ ] Proposed Recommendation
[ ] Working Draft
[x] Recommendation
[ ] Candidate Recommendation
Q64. Are any of the following declarations invalid?
color: red; /* declaration A */
font-size: 1em; /* declaration B */
padding: 10px 0; /* declaration C */
[ ] Declaration A is invalid.
[ ] Declaration B is invalid.
[ ] Declaration C is invalid.
[x] All declarations are valid.
Q65. Which CSS will cause your links to have a solid blue background that changes to semitransparent on hover?
Q66. Which CSS rule takes precedence over the others listed?
[ ] div.sidebar {}
[ ] * {}
[x] div#sidebar2 p {}
[ ] .sidebar p {}
Q67. The body of your page includes some HTML sections. How will it look with the following CSS applied?
body {
background: #ffffff; /* white */
}
section {
background: #0000ff; /* blue */
height: 200px;
}
[x] blue sections on a white background
[ ] Yellow sections on a blue background
[ ] Green sections on a white background
[ ] blue sections on a red background
Q68. Which CSS keyword can you use to override standard source order and specificity rules?
[ ] !elevate!
[ ] *prime
[ ] override
[x] !important
Q69. You can use the _ pseudo-class to set a different color on a link if it was clicked on.
[x] a:visited
[ ] a:hover
[ ] a:link
[ ] a:focus
Q70. Which color will look the brightest on your screen, assuming the background is white?
[ ] background-color: #aaa;
[ ] background-color: #999999;
[ ] background-color: rgba(170,170,170,0.5);
[x] background-color: rgba(170,170,170,0.2);
Q71. Which CSS selector can you use to select all elements on your page associated with the two classes header and clear?
[ ] ."header clear" {}
[ ] header#clear {}
[x] .header.clear {}
[ ] .header clear {}
Q72. A universal selector is specified using a(n) _.
[ ] “h1” string
[ ] “a” character
[ ] “p” character
[x] “*” character
Q73. In the following CSS code, 'h1' is the _, while 'color' is the _.
h1 {
color: red;
}
[ ] property; declaration
[ ] declaration; rule
[ ] “p” character
[x] selector; property
Q74. What is an alternate way to define the following CSS rule?
font-weight: bold;
[ ] font-weight: 400;
[ ] font-weight: medium;
[x] font-weight: 700;
[ ] font-weight: Black;
Q75. You want your styling to be based on a font stack consisting of three fonts. Where should the generic font for your font family be specified?
[ ] It should be the first one on the list.
[ ] Generic fonts are discouraged from this list.
[x] It should be the last one on the list.
[ ] It should be the second one on the list.
Q76. What is one disadvantage of using a web font service?
[ ] It requires you to host font files on your own server.
[ ] It uses more of your site’s bandwidth.
[ ] It offers a narrow selection of custom fonts.
[x] It is not always a free service.
Q77. How do you add Google fonts to your project?
[x] by using an HTML link element referring to a Google-provided CSS
[ ] by embedding the font file directly into the project’s master JavaScript
[ ] by using a Google-specific CSS syntax that directly links to the desired font file
[ ] by using a standard font-face CSS definition sourcing a font file on Google’s servers
Q78. which choice is not a valid color?
[ ] color: #000;
[ ] color: rgb(0,0,0);
[ ] color: #000000;
[x] color: 000000;
Q79. Using the following HTML and CSS example, what will equivalent pixel value be for .em and .rem elements?
html {font-size: 10px}
body {font-size: 2rem;}
.rem {font-size: 1.5rem;}
.em {font-size: 2em;}
<body>
<p class="rem"></p>
<p class="em"></p>
</body>
[ ] The .rem will be equivalent to 25px; the .em value will be 20px.
[ ] The .rem will be equivalent to 15px; the .em value will be 20px.
[ ] The .rem will be equivalent to 15px; the .em value will be 40px.
[ ] The .rem will be equivalent to 20px; the .em value will be 40px.
Q80. In this example, according to cascading and specificity rules, what color will the link be?
.example {color: yellow;}
ul li a {color: blue;}
ul a {color: green;}
a {color: red;}
<ul>
<li><a href="#" class="example">link</a></li>
<li>list item</li>
<li>list item</li>
</ul>
[ ] blue
[ ] red
[x] yellow
[ ] green
Q81. What property is used to adjust the space between text characters?
[ ] font-style
[ ] text-transform
[ ] font-variant
[x] letter-spacing
#### Q82. What is the correct syntax for changing the curse from an arrow to a pointing hand when it interacts with a named element?
Elementary Algorithms — Introduces elementary algorithms and data structures. Includes side-by-side comparisons of purely functional realization and their imperative counterpart.
Open Data Structures — Provide a high-quality open content data structures textbook that is both mathematically rigorous and provides complete implementations. (Code)
Since node 3 has edges to nodes 1 and 2, graph[3][1] and graph[3][2] have value 1.
a = LinkedListNode(5) b = LinkedListNode(1) c = LinkedListNode(9) a.next = b b.next = c
Arrays
Ok, so we know how to store individual numbers. Let’s talk about storing several numbers.
That’s right, things are starting to heat up.
Suppose we wanted to keep a count of how many bottles of kombucha we drink every day.
Let’s store each day’s kombucha count in an 8-bit, fixed-width, unsigned integer. That should be plenty — we’re not likely to get through more than 256 (2⁸) bottles in a single day, right?
And let’s store the kombucha counts right next to each other in RAM, starting at memory address 0:
Bam. That’s an **array**. RAM is *basically* an array already.
Just like with RAM, the elements of an array are numbered. We call that number the index of the array element (plural: indices). In this example, each array element’s index is the same as its address in RAM.
But that’s not usually true. Suppose another program like Spotify had already stored some information at memory address 2:
We’d have to start our array below it, for example at memory address 3. So index 0 in our array would be at memory address 3, and index 1 would be at memory address 4, etc.:
Suppose we wanted to get the kombucha count at index 4 in our array. How do we figure out what *address in memory* to go to? Simple math:
Take the array’s starting address (3), add the index we’re looking for (4), and that’s the address of the item we’re looking for. 3 + 4 = 7. In general, for getting the nth item in our array:
\text{address of nth item in array} = \text{address of array start} + n
This works out nicely because the size of the addressed memory slots and the size of each kombucha count are both 1 byte. So a slot in our array corresponds to a slot in RAM.
But that’s not always the case. In fact, it’s usually not the case. We usually use 64-bit integers.
So how do we build an array of 64-bit (8 byte) integers on top of our 8-bit (1 byte) memory slots?
We simply give each array index 8 address slots instead of 1:
So we can still use simple math to grab the start of the nth item in our array — just gotta throw in some multiplication:
\text{address of nth item in array} = \text{address of array start} + (n * \text{size of each item in bytes})
Don’t worry — adding this multiplication doesn’t really slow us down. Remember: addition, subtraction, multiplication, and division of fixed-width integers takes time. So all the math we’re using here to get the address of the nth item in the array takes time.
And remember how we said the memory controller has a direct connection to each slot in RAM? That means we can read the stuff at any given memory address in time.
**Together, this means looking up the contents of a given array index is time.** This fast lookup capability is the most important property of arrays.
But the formula we used to get the address of the nth item in our array only works if:
Each item in the array is the same size (takes up the same
number of bytes).
The array is uninterrupted (contiguous) in memory. There can’t
be any gaps in the array…like to “skip over” a memory slot Spotify was already using.
These things make our formula for finding the nth item work because they make our array predictable. We can predict exactly where in memory the nth element of our array will be.
But they also constrain what kinds of things we can put in an array. Every item has to be the same size. And if our array is going to store a lot of stuff, we’ll need a bunch of uninterrupted free space in RAM. Which gets hard when most of our RAM is already occupied by other programs (like Spotify).
That’s the tradeoff. Arrays have fast lookups ( time), but each item in the array needs to be the same size, and you need a big block of uninterrupted free memory to store the array.
## Pointers
Remember how we said every item in an array had to be the same size? Let’s dig into that a little more.
Suppose we wanted to store a bunch of ideas for baby names. Because we’ve got some really cute ones.
Each name is a string. Which is really an array. And now we want to store those arrays in an array. Whoa.
Now, what if our baby names have different lengths? That’d violate our rule that all the items in an array need to be the same size!
We could put our baby names in arbitrarily large arrays (say, 13 characters each), and just use a special character to mark the end of the string within each array…
“Wigglesworth” is a cute baby name, right?
But look at all that wasted space after “Bill”. And what if we wanted to store a string that was more than 13 characters? We’d be out of luck.
There’s a better way. Instead of storing the strings right inside our array, let’s just put the strings wherever we can fit them in memory. Then we’ll have each element in our array hold the address in memory of its corresponding string. Each address is an integer, so really our outer array is just an array of integers. We can call each of these integers a pointer, since it points to another spot in memory.
The pointers are marked with a \* at the beginning.
Pretty clever, right? This fixes both the disadvantages of arrays:
The items don’t have to be the same length — each string can be as
long or as short as we want.
We don’t need enough uninterrupted free memory to store all our
strings next to each other — we can place each of them separately, wherever there’s space in RAM.
We fixed it! No more tradeoffs. Right?
Nope. Now we have a new tradeoff:
Remember how the memory controller sends the contents of nearby memory addresses to the processor with each read? And the processor caches them? So reading sequential addresses in RAM is faster because we can get most of those reads right from the cache?
Our original array was very **cache-friendly**, because everything was sequential. So reading from the 0th index, then the 1st index, then the 2nd, etc. got an extra speedup from the processor cache.
But the pointers in this array make it not cache-friendly, because the baby names are scattered randomly around RAM. So reading from the 0th index, then the 1st index, etc. doesn’t get that extra speedup from the cache.
That’s the tradeoff. This pointer-based array requires less uninterrupted memory and can accommodate elements that aren’t all the same size, but it’s slower because it’s not cache-friendly.
This slowdown isn’t reflected in the big O time cost. Lookups in this pointer-based array are still time.
Linked lists
Our word processor is definitely going to need fast appends — appending to the document is like the main thing you do with a word processor.
Can we build a data structure that can store a string, has fast appends, and doesn’t require you to say how long the string will be ahead of time?
Let’s focus first on not having to know the length of our string ahead of time. Remember how we used pointers to get around length issues with our array of baby names?
What if we pushed that idea even further?
What if each character in our string were a two-index array with:
the character itself 2. a pointer to the next character
We would call each of these two-item arrays a **node** and we’d call this series of nodes a **linked list**.
Here’s how we’d actually implement it in memory:
Notice how we’re free to store our nodes wherever we can find two open slots in memory. They don’t have to be next to each other. They don’t even have to be *in order*:
“But that’s not cache-friendly, “ you may be thinking. Good point! We’ll get to that.
The first node of a linked list is called the head, and the last node is usually called the tail.
Confusingly, some people prefer to use “tail” to refer to everything after the head of a linked list. In an interview it’s fine to use either definition. Briefly say which definition you’re using, just to be clear.
It’s important to have a pointer variable referencing the head of the list — otherwise we’d be unable to find our way back to the start of the list!
We’ll also sometimes keep a pointer to the tail. That comes in handy when we want to add something new to the end of the linked list. In fact, let’s try that out:
Suppose we had the string “LOG” stored in a linked list:
Suppose we wanted to add an “S” to the end, to make it “LOGS”. How would we do that?
Easy. We just put it in a new node:
And tweak some pointers:
1. Grab the last letter, which is “G”. Our tail pointer lets us do this in time.
2. Point the last letter’s next to the letter we’re appending (“S”).
3. Update the tail pointer to point to our *new* last letter, “S”.
That’s time.
Why is it time? Because the runtime doesn’t get bigger if the string gets bigger. No matter how many characters are in our string, we still just have to tweak a couple pointers for any append.
Now, what if instead of a linked list, our string had been a dynamic array? We might not have any room at the end, forcing us to do one of those doubling operations to make space:
So with a dynamic array, our append would have a *worst-case* time cost of .
Linked lists have worst-case -time appends, which is better than the worst-case time of dynamic arrays.
That worst-case part is important. The average case runtime for appends to linked lists and dynamic arrays is the same: .
Now, what if we wanted to *pre*pend something to our string? Let’s say we wanted to put a “B” at the beginning.
For our linked list, it’s just as easy as appending. Create the node:
And tweak some pointers:
Point “B”’s next to “L”. 2. Point the head to “B”.
Bam. time again.
But if our string were a dynamic array…
And we wanted to add in that “B”:
Eep. We have to *make room* for the “B”!
We have to move each character one space down:
*Now* we can drop the “B” in there:
What’s our time cost here?
It’s all in the step where we made room for the first letter. We had to move all n characters in our string. One at a time. That’s time.
So linked lists have faster *pre*pends ( time) than dynamic arrays ( time).
No “worst case” caveat this time — prepends for dynamic arrays are always time. And prepends for linked lists are always time.
These quick appends and prepends for linked lists come from the fact that linked list nodes can go anywhere in memory. They don’t have to sit right next to each other the way items in an array do.
So if linked lists are so great, why do we usually store strings in an array? Becausearrays have -time lookups. And those constant-time lookups come from the fact that all the array elements are lined up next to each other in memory.
Lookups with a linked list are more of a process, because we have no way of knowing where the ith node is in memory. So we have to walk through the linked list node by node, counting as we go, until we hit the ith item.
def get_ith_item_in_linked_list(head, i): if i < 0: raise ValueError(“i can’t be negative: %d” % i) current_node = head current_position = 0 while current_node: if current_position == i: # Found it! return current_node # Move on to the next node current_node = current_node.next current_position += 1 raise ValueError(‘List has fewer than i + 1 (%d) nodes’ % (i + 1))
That’s i + 1 steps down our linked list to get to the ith node (we made our function zero-based to match indices in arrays). So linked lists have -time lookups. Much slower than the -time lookups for arrays and dynamic arrays.
Not only that — walking down a linked list is not cache-friendly. Because the next node could be anywhere in memory, we don’t get any benefit from the processor cache. This means lookups in a linked list are even slower.
So the tradeoff with linked lists is they have faster prepends and faster appends than dynamic arrays, but they have slower lookups.
## Doubly Linked Lists
In a basic linked list, each item stores a single pointer to the next element.
In a doubly linked list, items have pointers to the next and the previous nodes.
Doubly linked lists allow us to traverse our list *backwards*. In a *singly* linked list, if you just had a pointer to a node in the *middle* of a list, there would be *no way* to know what nodes came before it. Not a problem in a doubly linked list.
Array items are always located right next to each other in computer memory, but linked list nodes can be scattered all over.
So iterating through a linked list is usually quite a bit slower than iterating through the items in an array, even though they’re both theoretically time.
Hash tables
Quick lookups are often really important. For that reason, we tend to use arrays (-time lookups) much more often than linked lists (-time lookups).
For example, suppose we wanted to count how many times each ASCII character appears in Romeo and Juliet. How would we store those counts?
We can use arrays in a clever way here. Remember — characters are just numbers. In ASCII (a common character encoding) ‘A’ is 65, ‘B’ is 66, etc.
So we can use the character(‘s number value) as the index in our array, and store the count for that character at that index in the array:
With this array, we can look up (and edit) the count for any character in constant time. Because we can access any index in our array in constant time.
Something interesting is happening here — this array isn’t just a list of values. This array is storing two things: characters and counts. The characters are implied by the indices.
So we can think of an array as a table with two columns…except you don’t really get to pick the values in one column (the indices) — they’re always 0, 1, 2, 3, etc.
But what if we wanted to put any value in that column and still get quick lookups?
Suppose we wanted to count the number of times each word appears in Romeo and Juliet. Can we adapt our array?
Translating a character into an array index was easy. But we’ll have to do something more clever to translate a word (a string) into an array index…
Here’s one way we could do it:
Grab the number value for each character and add those up.
The result is 429. But what if we only have *30* slots in our array? We’ll use a common trick for forcing a number into a specific range: the modulus operator (%). Modding our sum by 30 ensures we get a whole number that’s less than 30 (and at least 0):
429 \: \% \: 30 = 9
Bam. That’ll get us from a word (or any string) to an array index.
This data structure is called a hash table or hash map. In our hash table, the counts are the values and the words (“lies, “ etc.) are the keys (analogous to the indices in an array). The process we used to translate a key into an array index is called a hashing function.
![A blank array except for a 20, labeled as the value, stored at index
To the left the array is the word “lies,” labeled as the key, with an
The hashing functions used in modern systems get pretty complicated — the one we used here is a simplified example.
Note that our quick lookups are only in one direction — we can quickly get the value for a given key, but the only way to get the key for a given value is to walk through all the values and keys.
Same thing with arrays — we can quickly look up the value at a given index, but the only way to figure out the index for a given value is to walk through the whole array.
One problem — what if two keys hash to the same index in our array? Look at “lies” and “foes”:
They both sum up to 429! So of course they’ll have the same answer when we mod by 30:
429 \: \% \: 30 = 9
So our hashing function gives us the same answer for “lies” and “foes.” This is called a hash collision. There are a few different strategies for dealing with them.
Here’s a common one: instead of storing the actual values in our array, let’s have each array slot hold a pointer to a linked list holding the counts for all the words that hash to that index:
One problem — how do we know which count is for “lies” and which is for “foes”? To fix this, we’ll store the *word* as well as the count in each linked list node:
“But wait!” you may be thinking, “Now lookups in our hash table take time in the worst case, since we have to walk down a linked list.” That’s true! You could even say that in the worst case *every* key creates a hash collision, so our whole hash table *degrades to a linked list*.
In industry though, we usually wave our hands and say collisions are rare enough that on average lookups in a hash table are time. And there are fancy algorithms that keep the number of collisions low and keep the lengths of our linked lists nice and short.
But that’s sort of the tradeoff with hash tables. You get fast lookups by key…except some lookups could be slow. And of course, you only get those fast lookups in one direction — looking up the key for a given value still takes time
Breadth-First Search (BFS) and Breadth-First Traversal
Breadth-first search (BFS) is a method for exploring a tree or graph. In a BFS, you first explore all the nodes one step away, then all the nodes two steps away, etc.
Breadth-first search is like throwing a stone in the center of a pond. The nodes you explore “ripple out” from the starting point.
Here’s a how a BFS would traverse this tree, starting with the root:
We’d visit all the immediate children (all the nodes that’re one step away from our starting node):
Then we’d move on to all *those* nodes’ children (all the nodes that’re *two steps* away from our starting node):
And so on:
Until we reach the end.
Breadth-first search is often compared with depth-first search.
Advantages:
A BFS will find the shortest path between the starting point and
any other reachable node. A depth-first search will not necessarily find the shortest path.
Disadvantages
A BFS on a binary tree generally requires more memory than a DFS.
### Binary Search Tree
A binary tree is a tree where <==(every node has two or fewer children)==>.
The children are usually called left and right.
class BinaryTreeNode(object):
This lets us build a structure like this:
That particular example is special because every level of the tree is completely full. There are no “gaps.” We call this kind of tree “**perfect**.”
Binary trees have a few interesting properties when they’re perfect:
Property 1: the number of total nodes on each “level” doubles as we move down the tree.
**Property 2: the number of nodes on the last level is equal to the sum of the number of nodes on all other levels (plus 1).** In other words, about *half* of our nodes are on the last level.
<==(*Let’s call the number of nodes n, *)==>
<==(**_**and the height of the tree h. _)==>
h can also be thought of as the “number of levels.”
If we had h, how could we calculate n?
Let’s just add up the number of nodes on each level!
If we zero-index the levels, the number of nodes on the xth level is exactly 2^x.
Level 0: 2⁰ nodes,
2. Level 1: 2¹ nodes,
3. Level 2: 2² nodes,
4. Level 3: 2³ nodes,
5. etc
So our total number of nodes is:
n = 2⁰ + 2¹ + 2² + 2³ + … + 2^{h-1}
Why only up to 2^{h-1}?
Notice that we started counting our levels at 0.
So if we have h levels in total,
the last level is actually the “h-1”-th level.
That means the number of nodes on the last level is 2^{h-1}.
But we can simplify.
Property 2 tells us that the number of nodes on the last level is (1 more than) half of the total number of nodes,
so we can just take the number of nodes on the last level, multiply it by 2, and subtract 1 to get the number of nodes overall.
We know the number of nodes on the last level is 2^{h-1},
So:
n = 2^{h-1} * 2–1
n = 2^{h-1} * 2¹ — 1
n = 2^{h-1+1}- 1
n = 2^{h} — 1
So that’s how we can go from h to n. What about the other direction?
We need to bring the h down from the exponent.
That’s what logs are for!
First, some quick review.
<==(log_{10} (100) )==>
simply means,
“What power must you raise 10 to in order to get 100?”.
Which is 2,
because .
<==(10² = 100 )==>
Graph Data Structure: Directed, Acyclic, etc
Graph =====
Binary numbers
Let’s put those bits to use. Let’s store some stuff. Starting with numbers.
The number system we usually use (the one you probably learned in elementary school) is called base 10, because each digit has ten possible values (1, 2, 3, 4, 5, 6, 7, 8, 9, and 0).
But computers don’t have digits with ten possible values. They have bits with two possible values. So they use base 2 numbers.
Base 10 is also called decimal. Base 2 is also called binary.
To understand binary, let’s take a closer look at how decimal numbers work. Take the number “101” in decimal:
Notice we have two “1”s here, but they don’t *mean* the same thing. The leftmost “1” *means* 100, and the rightmost “1” *means* 1. That’s because the leftmost “1” is in the hundreds place, while the rightmost “1” is in the ones place. And the “0” between them is in the tens place.
**So this “101” in base 10 is telling us we have “1 hundred, 0 tens, and 1 one.”**
Notice how the *places* in base 10 (ones place, tens place, hundreds place, etc.) are *sequential powers of 10*:
10⁰=1 * 10¹=10 * 10²=100 * 10³=1000 * etc.
The places in binary (base 2) are sequential powers of 2:
2⁰=1 * 2¹=2 * 2²=4 * 2³=8 * etc.
So let’s take that same “101” but this time let’s read it as a binary number:
Reading this from right to left: we have a 1 in the ones place, a 0 in the twos place, and a 1 in the fours place. So our total is 4 + 0 + 1 which is 5.
Deploy React App To Heroku Using Postgres & Express
Heroku is an web application that makes deploying applications easy for a beginner.
Deploy React App To Heroku Using Postgres & Express
Heroku is an web application that makes deploying applications easy for a beginner.
Before you begin deploying, make sure to remove any console.log's or debugger's in any production code. You can search your entire project folder if you are using them anywhere.
You will set up Heroku to run on a production, not development, version of your application. When a Node.js application like yours is pushed up to Heroku, it is identified as a Node.js application because of the package.json file. It runs npm install automatically. Then, if there is a heroku-postbuild script in the package.json file, it will run that script. Afterwards, it will automatically run npm start.
In the following phases, you will configure your application to work in production, not just in development, and configure the package.json scripts for install, heroku-postbuild and start scripts to install, build your React application, and start the Express production server.
Phase 1: Heroku Connection
If you haven’t created a Heroku account yet, create one here.
Add a new application in your Heroku dashboard named whatever you want. Under the “Resources” tab in your new application, click “Find more add-ons” and add the “Heroku Postgres” add-on with the free Hobby Dev setting.
In your terminal, install the Heroku CLI. Afterwards, login to Heroku in your terminal by running the following:
heroku login
Add Heroku as a remote to your project’s git repository in the following command and replace <name-of-Heroku-app> with the name of the application you created in the Heroku dashboard.
heroku git:remote -a <name-of-Heroku-app>
Next, you will set up your Express + React application to be deployable to Heroku.
Phase 2: Setting up your Express + React application
Right now, your React application is on a different localhost port than your Express application. However, since your React application only consists of static files that don’t need to bundled continuously with changes in production, your Express application can serve the React assets in production too. These static files live in the frontend/build folder after running npm run build in the frontend folder.
Add the following changes into your backend/routes.index.js file.
At the root route, serve the React application’s static index.html file along with XSRF-TOKEN cookie. Then serve up all the React application's static files using the express.static middleware. Serve the index.html and set the XSRF-TOKEN cookie again on all routes that don't start in /api. You should already have this set up in backend/routes/index.js which should now look like this:
// backend/routes/index.js
const express = require('express');
const router = express.Router();
const apiRouter = require('./api');
router.use('/api', apiRouter);
// Static routes
// Serve React build files in production
if (process.env.NODE_ENV === 'production') {
const path = require('path');
// Serve the frontend's index.html file at the root route
router.get('/', (req, res) => {
res.cookie('XSRF-TOKEN', req.csrfToken());
res.sendFile(
path.resolve(__dirname, '../../frontend', 'build', 'index.html')
);
});
// Serve the static assets in the frontend's build folder
router.use(express.static(path.resolve("../frontend/build")));
// Serve the frontend's index.html file at all other routes NOT starting with /api
router.get(/^(?!\/?api).*/, (req, res) => {
res.cookie('XSRF-TOKEN', req.csrfToken());
res.sendFile(
path.resolve(__dirname, '../../frontend', 'build', 'index.html')
);
});
}
// Add a XSRF-TOKEN cookie in development
if (process.env.NODE_ENV !== 'production') {
router.get('/api/csrf/restore', (req, res) => {
res.cookie('XSRF-TOKEN', req.csrfToken());
res.status(201).json({});
});
}
module.exports = router;
Your Express backend’s package.json should include scripts to run the sequelize CLI commands.
The backend/package.json's scripts should now look like this:
Initialize a package.json file at the very root of your project directory (outside of both the backend and frontend folders). The scripts defined in this package.json file will be run by Heroku, not the scripts defined in the backend/package.json or the frontend/package.json.
When Heroku runs npm install, it should install packages for both the backend and the frontend. Overwrite the install script in the root package.json with:
This will run npm install in the backend folder then run npm install in the frontend folder.
Next, define a heroku-postbuild script that will run the npm run build command in the frontend folder. Remember, Heroku will automatically run this script after running npm install.
Define a sequelize script that will run npm run sequelize in the backend folder.
Finally, define a start that will run npm start in the `backend folder.
The root package.json's scripts should look like this:
The dev:backend and dev:frontend scripts are optional and will not be used for Heroku.
Finally, commit your changes.
Phase 3: Deploy to Heroku
Once you’re finished setting this up, navigate to your application’s Heroku dashboard. Under “Settings” there is a section for “Config Vars”. Click the Reveal Config Vars button to see all your production environment variables. You should have a DATABASE_URL environment variable already from the Heroku Postgres add-on.
Add environment variables for JWT_EXPIRES_IN and JWT_SECRET and any other environment variables you need for production.
You can also set environment variables through the Heroku CLI you installed earlier in your terminal. See the docs for Setting Heroku Config Variables.
Push your project to Heroku. Heroku only allows the master branch to be pushed. But, you can alias your branch to be named master when pushing to Heroku. For example, to push a branch called login-branch to master run:
git push heroku login-branch:master
If you do want to push the master branch, just run:
git push heroku master
You may want to make two applications on Heroku, the master branch site that should have working code only. And your staging site that you can use to test your work in progress code.
Now you need to migrate and seed your production database.
Using the Heroku CLI, you can run commands inside of your production application just like in development using the heroku run command.
For example to migrate the production database, run:
heroku run npm run sequelize db:migrate
To seed the production database, run:
heroku run npm run sequelize db:seed:all
Note: You can interact with your database this way as you’d like, but beware that db:drop cannot be run in the Heroku environment. If you want to drop and create the database, you need to remove and add back the "Heroku Postgres" add-on.
Another way to interact with the production application is by opening a bash shell through your terminal by running:
heroku bash
In the opened shell, you can run things like npm run sequelize db:migrate.
Open your deployed site and check to see if you successfully deployed your Express + React application to Heroku!
If you see an Application Error or are experiencing different behavior than what you see in your local environment, check the logs by running:
heroku logs
If you want to open a connection to the logs to continuously output to your terminal, then run:
heroku logs --tail
The logs may clue you into why you are experiencing errors or different behavior.
If you found this guide helpful feel free to checkout my github/gists where I host similar content:
Numbering can be done using $
You can use this inside tag or contents.
h${This is so awesome $}*6
<h1>This is so awesome 1</h1>
<h2>This is so awesome 2</h2>
<h3>This is so awesome 3</h3>
<h4>This is so awesome 4</h4>
<h5>This is so awesome 5</h5>
<h6>This is so awesome 6</h6>
Many developers and aspiring students misinterpret React to be a fully functional framework. It is because we often compare React with major frameworks such as Angular and Ember. This comparison is not to compare the best frameworks but to focus on the differences and similarities of React and Angular’s approach that makes their offerings worth studying. Angular works on the MVC model to support the Model, View, and Controller layers of an app. React focuses only on the ‘V,’ which is the view layer of an application and how to make handling it easier to integrate smoothly into a project.
React’s Virtual DOM is faster than DOM.
React uses a Virtual DOM, which is essentially a tree of JavaScript objects representing the actual browser DOM. The advantage of using this for the developers is that they don’t manipulate the DOM directly as developers do with jQuery when they write React apps. Instead, they would tell React how they want the DOM to make changes to the state object and allow React to make the necessary updates to the browser DOM. This helps create a comprehensive development model for developers as they don’t need to track all DOM changes. They can modify the state object, and React would use its algorithms to understand what part of UI changed compared to the previous DOM. Using this information updates the actual browser DOM. Virtual DOM provides an excellent API for creating UI and minimizes the update count to be made on the browser DOM.
However, it is not faster than the actual DOM. You just read that it needs to pull extra strings to figure out what part of UI needs to be updated before actually performing those updates. Hence, Virtual DOM is beneficial for many things, but it isn’t faster than DOM.
1. Explain how React uses a tree data structure called the virtual DOM to model the DOM
The virtual DOM is a copy of the actual DOM tree. Updates in React are made to the virtual DOM. React uses a diffing algorithm to reconcile the changes and send the to the DOM to commit and paint.
2. Create virtual DOM nodes using JSX To create a React virtual DOM node using JSX, define HTML syntax in a JavaScript file.
Here, the JavaScript hello variable is set to a React virtual DOM h1 element with the text “Hello World!”.
You can also nest virtual DOM nodes in each other just like how you do it in HTML with the real DOM.
3. Use debugging tools to determine when a component is rendering
#### We use the React DevTools extension as an extension in our Browser DevTools to debug and view when a component is rendering
4. Describe how JSX transforms into actual DOM nodes
To transfer JSX into DOM nodes, we use the ReactDOM.render method. It takes a React virtual DOM node’s changes allows Babel to transpile it and sends the JS changes to commit to the DOM.
5. Use theReactDOM.rendermethod to have React render your virtual DOM nodes under an actual DOM node
6. Attach an event listener to an actual DOM node using a virtual node
The virtual DOM (VDOM) is a programming concept where an ideal, or “virtual”, representation of a UI is kept in memory and synced with the “real” DOM by a library such as ReactDOM. This process is called reconciliation.
This approach enables the declarative API of React: You tell React what state you want the UI to be in, and it makes sure the DOM matches that state. This abstracts out the attribute manipulation, event handling, and manual DOM updating that you would otherwise have to use to build your app.
Since “virtual DOM” is more of a pattern than a specific technology, people sometimes say it to mean different things. In React world, the term “virtual DOM” is usually associated with React elements since they are the objects representing the user interface. React, however, also uses internal objects called “fibers” to hold additional information about the component tree. They may also be considered a part of “virtual DOM” implementation in React.
Is the Shadow DOM the same as the Virtual DOM?
No, they are different. The Shadow DOM is a browser technology designed primarily for scoping variables and CSS in web components. The virtual DOM is a concept implemented by libraries in JavaScript on top of browser APIs.
To add an event listener to an element, define a method to handle the event and associate that method with the element event you want to listen for:
7. Usecreate-react-appto initialize a new React app and import required dependencies
Create the default create-react-application by typing in our terminal
npm (node package manager) is the dependency/package manager you get out of the box when you install Node.js. It provides a way for developers to install packages both globally and locally.
Sometimes you might want to take a look at a specific package and try out some commands. But you cannot do that without installing the dependencies in your local node_modules folder.
npm the package manager
npm is a couple of things. First and foremost, it is an online repository for the publishing of open-source Node.js projects.
Second, it is a CLI tool that aids you to install those packages and manage their versions and dependencies. There are hundreds of thousands of Node.js libraries and applications on npm and many more are added every day.
npm by itself doesn’t run any packages. If you want to run a package using npm, you must specify that package in your package.json file.
When executables are installed via npm packages, npm creates links to them:
local installs have links created at the ./node_modules/.bin/ directory
global installs have links created from the global bin/ directory (for example: /usr/local/bin on Linux or at %AppData%/npm on Windows)
To execute a package with npm you either have to type the local path, like this:
$ ./node_modules/.bin/your-package
or you can run a locally installed package by adding it into your package.json file in the scripts section, like this:
You can see that running a package with plain npm requires quite a bit of ceremony.
Fortunately, this is where npx comes in handy.
npx the package runner
Since npm version 5.2.0 npx is pre-bundled with npm. So it’s pretty much a standard nowadays.
npx is also a CLI tool whose purpose is to make it easy to install and manage dependencies hosted in the npm registry.
It’s now very easy to run any sort of Node.js-based executable that you would normally install via npm.
You can run the following command to see if it is already installed for your current npm version:
$ which npx
If it’s not, you can install it like this:
$ npm install -g npx
Once you make sure you have it installed, let’s see a few of the use cases that make npx extremely helpful.
Run a locally installed package easily
If you wish to execute a locally installed package, all you need to do is type:
$ npx your-package
npx will check whether <command> or <package> exists in $PATH, or in the local project binaries, and if so it will execute it.
Execute packages that are not previously installed
Another major advantage is the ability to execute a package that wasn’t previously installed.
Sometimes you just want to use some CLI tools but you don’t want to install them globally just to test them out. This means you can save some disk space and simply run them only when you need them. This also means your global variables will be less polluted.
Now, where were we?
npx create-react-app <name of app> --use-npm
npx gives us the latest version. --use-npm just means to use npm instead of yarn or some other package manager
8. Pass props into a React component
props is an object that gets passed down from the parent component to the child component. The values can be of any data structure including a function (which is an object)
Set a variable to the string, “world”, and replace the string of “world” in the NavLinks JSX element with the variable wrapped in curly braces:
Accessing props:
To access our props object in another component we pass it the props argument and React will invoke the functional component with the props object.
Reminder:
Conceptually, components are like JavaScript functions. They accept arbitrary inputs (called “props”) and return React elements describing what should appear on the screen.
Function and Class Components
The simplest way to define a component is to write a JavaScript function:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
This function is a valid React component because it accepts a single “props” (which stands for properties) object argument with data and returns a React element. We call such components “function components” because they are literally JavaScript functions.
You can also use an ES6 class to define a component:
The above two components are equivalent from React’s point of view.
You can pass down as many props keys as you want.
9. Destructure props
You can destructure the props object in the function component’s parameter.
10. Create routes using components from the react-router-dom package
a. Import the react-router-dom package:
npm i react-router-dom
In your index.js:
Above you import your BrowserRouter with which you can wrap your entire route hierarchy. This makes routing information from React Router available to all its descendent components.
Then in the component of your choosing, usually top tier such as App.js, you can create your routes using the Route and Switch Components
This list of resources is specifically targeted at Web Developers and Data Scientists…. so do with it what you will…This list borrows…
Everything You Need To Become A Machine Learner
This list of resources is specifically targeted at Web Developers and Data Scientists…. so do with it what you will…This list borrows heavily from multiple lists created by :sindresorhus
Machine learning is a subfield of artificial intelligence, which is broadly defined as the capability of a machine to imitate intelligent human behavior. Artificial intelligence systems are used to perform complex tasks in a way that is similar to how humans solve problems.
The goal of AI is to create computer models that exhibit “intelligent behaviors” like humans, according toBoris Katz, a principal research scientist and head of the InfoLab Group at CSAIL. This means machines that can recognize a visual scene, understand a text written in natural language, or perform an action in the physical world.
Machine learning is one way to use AI. It was defined in the 1950s by AI pioneerArthur Samuelas “the field of study that gives computers the ability to learn without explicitly being programmed.”
Natural language processing is a field of machine learning in which machines learn to understand natural language as spoken and written by humans, instead of the data and numbers normally used to program computers. This allows machines to recognize language, understand it, and respond to it, as well as create new text and translate between languages. Natural language processing enables familiar technology like chatbots and digital assistants like Siri or Alexa.
### Neural networks
Neural networks are a commonly used, specific class of machine learning algorithms. Artificial neural networks are modeled on the human brain, in which thousands or millions of processing nodes are interconnected and organized into layers.
In an artificial neural network, cells, or nodes, are connected, with each cell processing inputs and producing an output that is sent to other neurons. Labeled data moves through the nodes, or cells, with each cell performing a different function. In a neural network trained to identify whether a picture contains a cat or not, the different nodes would assess the information and arrive at an output that indicates whether a picture features a cat.
Be familiar with how Machine Learning is applied at other companies
*This list of resources is specifically targeted at Web Developers and Data Scientists…. so do with it what you will…*
This list borrows heavily from multiple lists created by :sindresorhus
Machine learning is a subfield of artificial intelligence, which is broadly defined as the capability of a machine to imitate intelligent human behavior. Artificial intelligence systems are used to perform complex tasks in a way that is similar to how humans solve problems.
The goal of AI is to create computer models that exhibit “intelligent behaviors” like humans, according toBoris Katz, a principal research scientist and head of the InfoLab Group at CSAIL. This means machines that can recognize a visual scene, understand a text written in natural language, or perform an action in the physical world.
Machine learning is one way to use AI. It was defined in the 1950s by AI pioneerArthur Samuelas “the field of study that gives computers the ability to learn without explicitly being programmed.”
Natural language processing is a field of machine learning in which machines learn to understand natural language as spoken and written by humans, instead of the data and numbers normally used to program computers. This allows machines to recognize language, understand it, and respond to it, as well as create new text and translate between languages. Natural language processing enables familiar technology like chatbots and digital assistants like Siri or Alexa.
Neural networks
Neural networks are a commonly used, specific class of machine learning algorithms. Artificial neural networks are modeled on the human brain, in which thousands or millions of processing nodes are interconnected and organized into layers.
In an artificial neural network, cells, or nodes, are connected, with each cell processing inputs and producing an output that is sent to other neurons. Labeled data moves through the nodes, or cells, with each cell performing a different function. In a neural network trained to identify whether a picture contains a cat or not, the different nodes would assess the information and arrive at an output that indicates whether a picture features a cat.
Be familiar with how Machine Learning is applied at other companies