iOS 7.0 and iOS 8 (Beta) do not have support for minimal-ui
viewport keyword, nor do they response to window.slideTo(0,1)
or support Fullscreen API, which means there is no easy way to tell Mobile Safari to hide address bar/menu without user interaction.
This is a problem for Web App that mimics Native App design, where html/body are commonly set to height: 100%
, so browser viewport = available screen estate. There are currently no solution besides adding apple-mobile-web-app-capable
meta and ask users to manually Save to Homescreen
.
Frustrated by this limit, we present a soft-fullscreen prompt design, which, coupled with proper CSS hack, can achieve soft-fullscreen with relatively small effort from users.
Below is a demo page for such design, basically, by using calc()
for height, we are able to target Mobile Safari address bar height, and trigger a soft-fullscreen on user scroll.
html {
height: calc(100% + 72px);
}
72px
isn't a random number, iOS7+ UI has 44px address bar and 44px menu bar for Mobile Safari. When soft-fullscreen is triggered, its address bar collapses to 20px. So the theoretical minimum document height required to trigger hide address bar is 88px - 20px = 68px, plus the viewport height.
After testing on iOS7.1, we found that if such value is below 72px, Mobile Safari does not always hide address bar after user scroll. Thus 72px is chosen as baseline.
(Obviously there are a lot of testing needed to be done for this demo to be usable cross-device, but this demo shows we can achieve soft-fullscreen with relatively small impact on user.)
And and preventing touchmove the screen will now act more like an app.
Ex. if we were to use the above demo.html file, chagne the script part to this:
Final code here, try in your iPhone/iPad and tell us if it works:
http://repos.codehot.tech/misc/ios-webapp-example.html
(scan this qr-code in camera to get the above link)
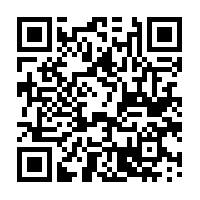