Last active
November 24, 2019 22:50
-
-
Save blackwatertepes/f768c67da32d1615fdf1fd3ee8cf1859 to your computer and use it in GitHub Desktop.
Render Images from Circle CI Artifacts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
ONE_LINER: Creates images from URL's in the Circle CI Artirfacts tab! | |
FULL_DESCRIPTION: | |
1. Turns '.png' url's in the Circle CI Artifacts tab into viewable images | |
a. Organizes the images into sections, based off of their test file names | |
b. Renders the images with spacing, and outlines based off of their test names | |
- Example: [beforeEach, some named screenshot, some other named screenshot, afterEach] | |
c. Adds buttons to scale up/down the images for individual sections | |
2. Acts as a bookmarket for your Circle CI project | |
a. Replace the PROJECT_NAME below with the name of your CircleCI project | |
*/ | |
var CIRCLECI_URI = "circleci.com"; | |
var GITHUB_URI = "github.com"; | |
var PROJECT_NAME = "Flatbook"; /* << REPLACE WITH THE NAME OF YOUR PROJECT */ | |
var imgHeightMin = 100; | |
var imgHeightMax = 800; | |
if (window.location.href.match(CIRCLECI_URI)) { | |
var getAlt = function(href) { | |
var pre = href.split("/"); | |
pre.pop(); | |
return pre.pop().replace(/%20/g, " "); | |
}; | |
var getClassName = function(href) { | |
return href | |
.split("/") | |
.pop() | |
.split(".") | |
.shift(); | |
}; | |
var getSection = function(href) { | |
var pre = getAlt(href).split(" "); | |
pre.shift(); | |
return pre.shift(); | |
}; | |
var createImage = function(href) { | |
var className = getClassName(href); | |
var alt = getAlt(href); | |
return ( | |
"<img class='" + | |
className + | |
"' src='" + | |
href + | |
"' height='" + | |
imgHeightMin + | |
"' alt='" + | |
alt + | |
"' />" | |
); | |
}; | |
var sections = {}; | |
var artifactList = document.querySelectorAll("#__next main div ol ul")[0]; | |
for (var el of artifactList.getElementsByTagName("a")) { | |
if (el.href.match(".png")) { | |
el.innerHTML = createImage(el.href); | |
el.className = el.className + " " + getSection(el.href); | |
} else { | |
el.innerHTML = ""; | |
} | |
if (getSection(el.href) && getAlt(el.href)) { | |
if (!sections[getSection(el.href)]) { | |
sections[getSection(el.href)] = {}; | |
} | |
if (!sections[getSection(el.href)][getAlt(el.href)]) { | |
sections[getSection(el.href)][getAlt(el.href)] = []; | |
} | |
sections[getSection(el.href)][getAlt(el.href)].push( | |
decodeURI(el.href).toString() | |
); | |
sections[getSection(el.href)][getAlt(el.href)].sort(function(a, b) { | |
if (a.match("beforeEach")) { | |
return 0; | |
} | |
if (a.match("afterEach")) { | |
return 2; | |
} | |
return 1; | |
}); | |
} | |
} | |
artifactList.innerHTML = ""; | |
var createButton = function(text, className, backgroundColor, sectionName) { | |
var button = | |
"<span class='button_" + | |
className + | |
" " + | |
sectionName + | |
"' style='background-color:" + | |
backgroundColor + | |
";width:50px;height:20px;display:inline-block;text-align:center;border-radius:5px;margin-left:10px;'>" + | |
text + | |
"</span>"; | |
return button; | |
}; | |
for (var sectionName in sections) { | |
var buttons = | |
createButton("-", "shrink", "#DAA", sectionName) + | |
createButton("+", "grow", "#ADA", sectionName); | |
artifactList.innerHTML = | |
artifactList.innerHTML + | |
"<li><h4><span>" + | |
sectionName + | |
"</span>" + | |
buttons + | |
"</h4></li>"; | |
for (var testName in sections[sectionName]) { | |
var section = artifactList.querySelectorAll("li:last-of-type")[0]; | |
var hrefs = sections[sectionName][testName]; | |
for (var href of hrefs) { | |
section.innerHTML = | |
section.innerHTML + | |
"<a class='" + | |
getSection(href) + | |
"' href='" + | |
href + | |
"'>" + | |
createImage(href) + | |
"</a>"; | |
} | |
} | |
} | |
for (var el of document.getElementsByClassName("beforeEach")) { | |
el.setAttribute( | |
"style", | |
"border-left:1px solid black;border-top:1px solid black;border-bottom:1px solid black;margin-left:20px;" | |
); | |
} | |
for (var el of document.getElementsByClassName("afterEach")) { | |
el.setAttribute( | |
"style", | |
"border-right:1px solid black;border-top:1px solid black;border-bottom:1px solid black;margin-right:20px;" | |
); | |
} | |
for (var el of document.getElementsByClassName("button_grow")) { | |
el.onclick = function(event) { | |
for (var el of event.currentTarget.parentElement.parentElement.getElementsByTagName( | |
"img" | |
)) { | |
if (el.height < imgHeightMax) { | |
el.setAttribute("height", Math.min(el.height * 4, imgHeightMax)); | |
} | |
} | |
}; | |
} | |
for (var el of document.getElementsByClassName("button_shrink")) { | |
el.onclick = function(event) { | |
for (var el of event.currentTarget.parentElement.parentElement.getElementsByTagName( | |
"img" | |
)) { | |
if (el.height > 0) { | |
el.setAttribute("height", imgHeightMin); | |
} | |
} | |
}; | |
} | |
} else if (window.location.href.match(GITHUB_URI)) { | |
for (var el of document.getElementsByClassName("status-actions")) { | |
if (el.href.match(CIRCLECI_URI)) { | |
el.click(); | |
break; | |
} | |
} | |
} else { | |
window.location.href = "https://" + CIRCLECI_URI + "/gh/" + PROJECT_NAME; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here's what it looks like in action...
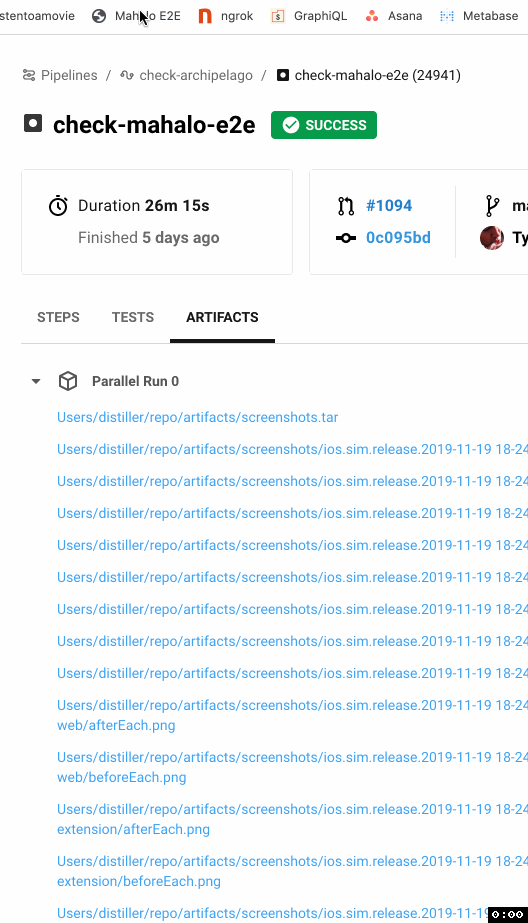