Last active
May 5, 2023 17:52
-
-
Save boppreh/3607a043aa1fb9f539df26f67a89b64b to your computer and use it in GitHub Desktop.
Creates two GIFs, where the second one swaps the Y and time axis.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import sys | |
from PIL import Image, ImageDraw | |
if len(sys.argv) > 1: | |
gif_path, = sys.argv[1:] | |
im = Image.open(gif_path) | |
width, before_height = im.size | |
images_before = [im.copy()] | |
while im.tell() < im.n_frames-1: | |
im.seek(im.tell()+1) | |
images_before.append(im.copy()) | |
else: | |
width = before_height = 200 | |
images_before = [] | |
# Default pre-rotation animation adapted from https://note.nkmk.me/en/python-pillow-gif/ | |
center = width // 2 | |
color_1 = (0, 0, 0) | |
color_2 = (255, 255, 255) | |
max_radius = int(center * 1.5) | |
step = 2 | |
for i in range(0, max_radius, step): | |
im = Image.new('RGB', (width, before_height), color_1) | |
draw = ImageDraw.Draw(im) | |
draw.ellipse((center - i, center - i, center + i, center + i), fill=color_2) | |
images_before.append(im) | |
for i in range(0, max_radius, step): | |
im = Image.new('RGB', (width, before_height), color_2) | |
draw = ImageDraw.Draw(im) | |
draw.ellipse((center - i, center - i, center + i, center + i), fill=color_1) | |
images_before.append(im) | |
images_before[0].save('time_rotation_before.gif', | |
save_all=True, append_images=images_before[1:], optimize=False, duration=40, loop=0) | |
# Create a new stack of images swapping the Y and time axis. | |
after_height = len(images_before) | |
after_n_frames = before_height | |
pixels_after = [[(0, 0, 0)]*width*after_height for _ in range(after_n_frames)] | |
for after_y, image_before in enumerate(images_before): | |
pixels = list(image_before.getdata()) | |
assert len(pixels) == width * before_height | |
for before_y in range(before_height): | |
row = pixels[width*before_y:width*(before_y+1)] | |
pixels_after[before_y][width*after_y:width*(after_y+1)] = row | |
images_after = [] | |
for pixels in pixels_after: | |
img = Image.new('RGB', (width, after_height)) | |
img.putdata(pixels) | |
images_after.append(img) | |
images_after[0].save('time_rotation_after.gif', | |
save_all=True, append_images=images_after[1:], optimize=False, duration=40, loop=0) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Before:
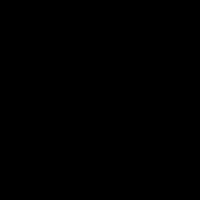
After:
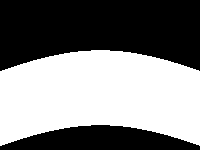
Before:
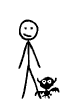
After:
