Created
June 4, 2018 22:02
-
-
Save branflake2267/eaf371b29bfdc4e3210a17615c4559a5 to your computer and use it in GitHub Desktop.
GXT 4, Example of a grid in a flex layout. Using the HtmllayoutContainer
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<style> | |
.main { | |
width: 100%; | |
height: 300px; | |
border: 1px solid coral; | |
display: flex; | |
flex-direction: row; | |
} | |
.left { | |
border: 1px solid blue; | |
width: 150px; | |
} | |
.middle { | |
border: 1px solid red; | |
flex-grow: 1; | |
} | |
.right { | |
border: 1px solid black; | |
width: 150px; | |
} | |
</style> | |
<div class="main"> | |
<div class="left">Left</div> | |
<div class="middle"></div> | |
<div class="right">Right</div> | |
</div> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package com.sencha.gxt.test.client.layout; | |
import java.util.ArrayList; | |
import java.util.Date; | |
import java.util.List; | |
import com.google.gwt.core.client.EntryPoint; | |
import com.google.gwt.core.client.GWT; | |
import com.google.gwt.event.logical.shared.ResizeEvent; | |
import com.google.gwt.event.logical.shared.ResizeHandler; | |
import com.google.gwt.safehtml.shared.SafeHtml; | |
import com.google.gwt.user.client.Window; | |
import com.google.gwt.user.client.ui.RootPanel; | |
import com.sencha.gxt.core.client.XTemplates; | |
import com.sencha.gxt.core.client.dom.XDOM; | |
import com.sencha.gxt.data.shared.ListStore; | |
import com.sencha.gxt.test.client.TestSample; | |
import com.sencha.gxt.test.client.data.StockDto; | |
import com.sencha.gxt.test.client.data.StockDtoProperties; | |
import com.sencha.gxt.test.client.data.TestSampleData; | |
import com.sencha.gxt.widget.core.client.container.AbstractHtmlLayoutContainer.HtmlData; | |
import com.sencha.gxt.widget.core.client.container.HtmlLayoutContainer; | |
import com.sencha.gxt.widget.core.client.grid.ColumnConfig; | |
import com.sencha.gxt.widget.core.client.grid.ColumnModel; | |
import com.sencha.gxt.widget.core.client.grid.Grid; | |
@TestSample( | |
name = "HtmlLayoutContainer Fluid Sizing", | |
description = { "Test in Modern Browsers Only", "Resize Grid", "Verify the grid will resize." }, | |
jiraIds = 4307) | |
public class HtmlLayoutContainerFluidSizing implements EntryPoint { | |
public interface HtmlLayoutContainerTemplate extends XTemplates { | |
@XTemplate(source = "HtmlFluidSizing.html") | |
SafeHtml getTemplate(); | |
} | |
public int width = 0; | |
@Override | |
public void onModuleLoad() { | |
final Grid<StockDto> grid = createGrid(); | |
HtmlLayoutContainerTemplate templates = GWT.create(HtmlLayoutContainerTemplate.class); | |
HtmlLayoutContainer htmlLayoutContainer = new HtmlLayoutContainer(templates.getTemplate()); | |
htmlLayoutContainer.add(grid, new HtmlData(".middle")); | |
RootPanel.get().add(htmlLayoutContainer); | |
width = XDOM.getViewportWidth(); | |
// resize grid | |
Window.addResizeHandler(new ResizeHandler() { | |
@Override | |
public void onResize(ResizeEvent event) { | |
int newWidth = event.getWidth(); | |
int delta = newWidth - width; | |
if (delta < 0) { // shrinking | |
int w = grid.getOffsetWidth() + delta; | |
grid.setWidth(w + "px"); | |
} else { // growing | |
grid.setSize("100%", "100%"); | |
} | |
width = newWidth; | |
} | |
}); | |
} | |
private Grid<StockDto> createGrid() { | |
StockDtoProperties props = GWT.create(StockDtoProperties.class); | |
ColumnConfig<StockDto, String> nameCol = new ColumnConfig<StockDto, String>(props.name(), 100, "Company"); | |
ColumnConfig<StockDto, String> symbolCol = new ColumnConfig<StockDto, String>(props.symbol(), 100, "Symbol"); | |
ColumnConfig<StockDto, Double> lastCol = new ColumnConfig<StockDto, Double>(props.last(), 100, "Last"); | |
ColumnConfig<StockDto, Double> changeCol = new ColumnConfig<StockDto, Double>(props.change(), 100, "Change"); | |
ColumnConfig<StockDto, Date> lastTransCol = new ColumnConfig<StockDto, Date>(props.lastTrans(), 100, | |
"Last Updated"); | |
List<ColumnConfig<StockDto, ?>> columns = new ArrayList<ColumnConfig<StockDto, ?>>(); | |
columns.add(nameCol); | |
columns.add(symbolCol); | |
columns.add(lastCol); | |
columns.add(changeCol); | |
columns.add(lastTransCol); | |
ColumnModel<StockDto> cm = new ColumnModel<StockDto>(columns); | |
ListStore<StockDto> store = new ListStore<StockDto>(props.key()); | |
store.addAll(TestSampleData.getStocks()); | |
Grid<StockDto> grid = new Grid<StockDto>(store, cm) { | |
@Override | |
protected void onResize(int width, int height) { | |
super.onResize(width, height); | |
// GWT.log("Grid onResize() w=" + width + " h=" + height); | |
} | |
}; | |
grid.setBorders(true); | |
grid.getView().setColumnLines(true); | |
grid.setSize("100%", "100%"); | |
grid.getView().setAutoExpandColumn(nameCol); | |
grid.getView().setAutoExpandMax(5000); | |
return grid; | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Example:
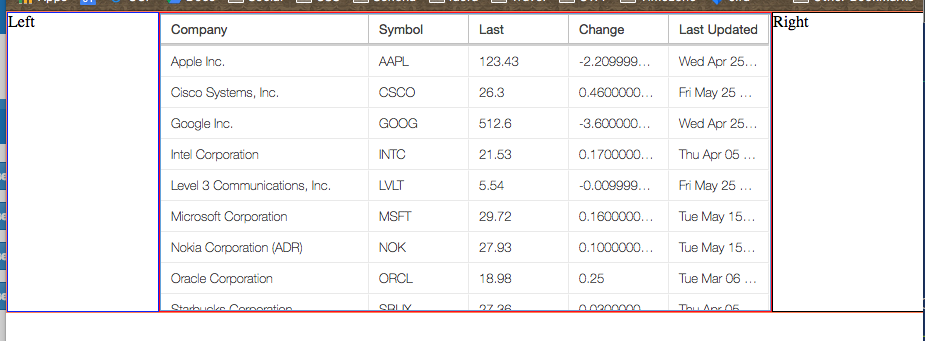