This covers the basic directions to get up and running with webpack.
If you're creating your webpack configuration from scratch, these plugins will be needed.
- Run
npm install --save-dev webpack
- Run
npm install --save-dev webpack webpack-cli
- Run
npm install --save-dev path
The html-webpack-plugin will copy the index.html
template to the dist folder and add the bundle.js resource to it.
- Run
npm install --save-dev html-webpack-plugin
- Run
npm install --save-dev clean-webpack-plugin
- Run
npm install --save-dev style-loader
- Run
npm install --save-dev css-loader
- Run
npm install froala-editor
If you copy or delete the ./node_modules
folder, download the dependencies first.
- Run
npm install
This will run webpack and it observes the changes and will output the changes to the ./dist
directory.
- Run
npm run dev
- Open the webpack generated ./dist/index.html.
https://www.froala.com/wysiwyg-editor/download
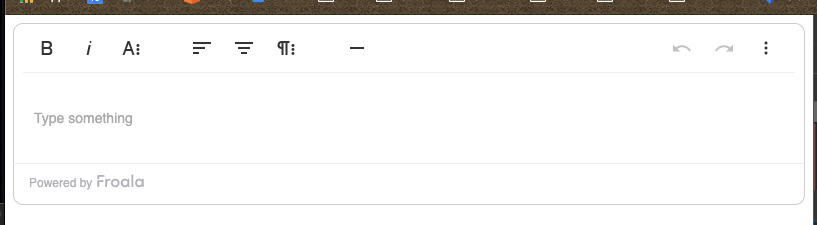