-
-
Save brunomichetti/b7367342a75620d03308d6fca6f75499 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from typing import List | |
from django.contrib.auth.models import AbstractUser | |
from django.contrib.gis.db import models as gis_models | |
from django.core.validators import MinValueValidator | |
from django.db import models | |
from django.db.models import Q, QuerySet | |
from django.utils.translation import gettext_lazy as _ | |
class User(AbstractUser): | |
class ZodiacSigns(models.TextChoices): | |
AQUARIUS = "aquarius", _("Aquarius") | |
PISCES = "pisces", _("Pisces") | |
ARIES = "aries", _("Aries") | |
TAURUS = "taurus", _("Taurus") | |
GEMINI = "gemini", _("Gemini") | |
CANCER = "cancer", _("Cancer") | |
LEO = "leo", _("Leo") | |
VIRGO = "virgo", _("Virgo") | |
LIBRA = "libra", _("Libra") | |
SCORPIO = "scorpio", _("Scorpio") | |
SAGITTARIUS = "sagittarius", _("Sagittarius") | |
CAPRICORN = "capricorn", _("Capricorn") | |
email = models.EmailField(_("Email of user"), unique=True, db_index=True) | |
name = models.CharField(_("Name of user"), max_length=255, blank=True, null=True, default=None) | |
username = models.CharField(_("Username of user"), max_length=50, blank=True, null=True, default=None) | |
date_of_birth = models.DateField(_("Birth date of user"), blank=True, null=True, default=None) | |
zodiac_sign = models.CharField( | |
_("Zodiac sign of user"), | |
max_length=15, | |
choices=ZodiacSigns.choices, | |
blank=True, | |
null=True, | |
default=None, | |
) | |
matched_users = models.ManyToManyField("self") | |
EMAIL_FIELD = "email" | |
USERNAME_FIELD = "email" | |
REQUIRED_FIELDS: List[str] = [] | |
class Target(models.Model): | |
class Topics(models.TextChoices): | |
SPORTS = "sports", _("Sports") | |
MOVIES_SERIES = "movies_series", _("Movies and/or Series") | |
POLITICS = "politics", _("Politics") | |
DATES = "dates", _("Dates") | |
FOOD = "food", _("Food") | |
TRAVELS = "travels", _("Travels") | |
BOOKS = "books", _("Books") | |
user = models.ForeignKey(to=User, related_name="targets", on_delete=models.CASCADE, db_index=True) | |
title = models.CharField(_("Title of the target"), max_length=255) | |
topic = models.CharField(_("Topic of the target"), choices=Topics.choices, max_length=15) | |
radius_in_mts = models.FloatField(_("Radius in meters of the target"), validators=(MinValueValidator(0.0),)) | |
location = gis_models.PointField(_("Location of the target")) | |
created_at = models.DateTimeField(_("Create timestamp of the target"), auto_now_add=True) | |
updated_at = models.DateTimeField(_("Update timestamp of the target"), auto_now=True) | |
@property | |
def matches(self) -> QuerySet["Match"]: | |
return Match.objects.filter(Q(target_1=self) | Q(target_2=self)) | |
class Match(models.Model): | |
target_1 = models.ForeignKey(to=Target, related_name="matches_1", on_delete=models.CASCADE, db_index=True) | |
target_2 = models.ForeignKey(to=Target, related_name="matches_2", on_delete=models.CASCADE, db_index=True) | |
created_at = models.DateTimeField(_("Create timestamp of the match"), auto_now_add=True) | |
class Meta: | |
verbose_name_plural = "matches" | |
unique_together = (("target_1", "target_2"),) |
hey @brunomichetti I think there is a typo in this gist, in the line
matched_users = models.ManyToManyField("self")
has tabs instead of spaces and in the blog post it is visible.
yes you are right
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
hey @brunomichetti I think there is a typo in this gist, in the line
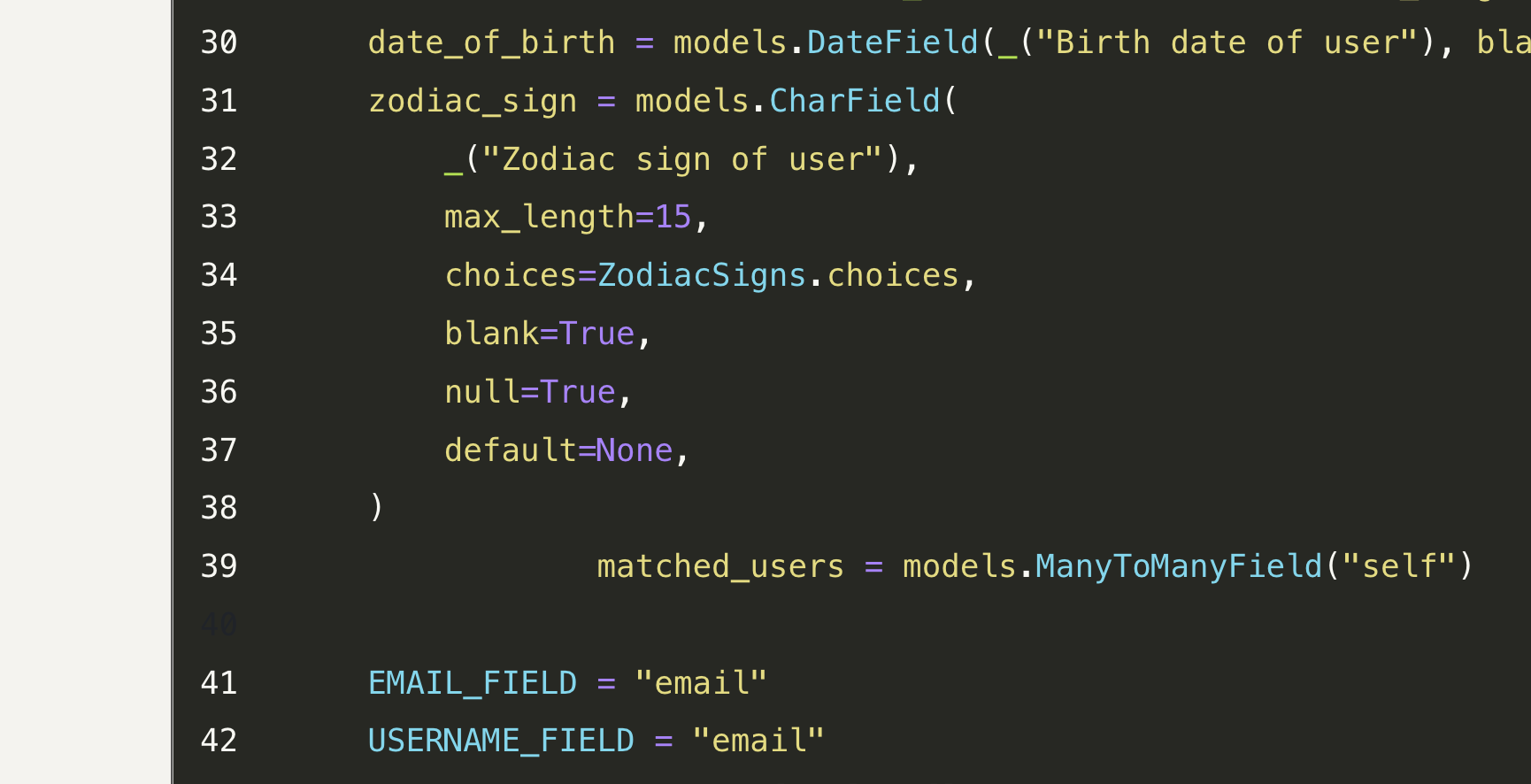
matched_users = models.ManyToManyField("self")
has tabs instead of spaces and in the blog post it is visible.