Last active
October 11, 2018 22:19
-
-
Save btastic/cc84c7b9418be66db7fd78a49aebcf62 to your computer and use it in GitHub Desktop.
A simple script to generate a new dotnet project with a default project hierarchy
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
param( | |
[Parameter(Mandatory = $true)] | |
[string]$ProjectName, | |
[Parameter(Mandatory = $true)] | |
[string]$SolutionName, | |
[Parameter(Mandatory = $true)] | |
[string]$DotNetTemplateName | |
) | |
$ErrorActionPreference = "Stop" | |
Function Get-Gitignore { | |
param( | |
[Parameter(Mandatory = $true)] | |
[string[]]$list, | |
[Parameter(Mandatory = $true)] | |
[string] $path | |
) | |
$params = ($list | ForEach-Object { [uri]::EscapeDataString($_) }) -join "," | |
Invoke-WebRequest -Uri "https://www.gitignore.io/api/$params" | Select-Object -ExpandProperty content | Out-File -FilePath $(Join-Path -path $path -ChildPath ".gitignore") -Encoding ascii | |
} | |
Write-Host "Creating project $SolutionName" | |
if(Test-Path $SolutionName) { | |
Write-Error "Project folder does already exists. $SolutionName" | |
exit | |
} | |
# Folder structure | |
mkdir $SolutionName | |
$ProjectDir = Get-Item .\$SolutionName | |
mkdir $ProjectDir\src | |
mkdir $ProjectDir\tests | |
$SourceDir = Get-Item $ProjectDir\src | |
$TestsDir = Get-Item $ProjectDir\tests | |
# Default items | |
New-Item $ProjectDir\README.md | |
New-Item $ProjectDir\LICENSE | |
New-Item $TestsDir\.gitkeep | |
# .gitignore for visual studio | |
Get-Gitignore visualstudio $ProjectDir | |
# dotnet stuff | |
dotnet new $DotNetTemplateName -n $ProjectName -o $SourceDir\$ProjectName | |
dotnet new sln -o $ProjectDir\src -n $SolutionName | |
dotnet sln $SourceDir add $SourceDir\$ProjectName\ | |
dotnet build $SourceDir\$ProjectName | |
# git | |
git init $ProjectDir | |
git -C $ProjectDir\ add . | |
git -C $ProjectDir\ commit -m "Initial project creation" | |
#prompt to open the solution | |
$reply = Read-Host -Prompt "Open solution now? [y/n]" | |
if ( $reply -match "[yY]" ) { | |
Invoke-Item $SourceDir\$SolutionName.sln | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Usage:
.\New-DotNet ProjectName SolutionName DotNetTemplate
ProjectName = your project name
DotNetTemplate = the template name for dotnet scaffolding (https://docs.microsoft.com/de-de/dotnet/core/tools/dotnet-new?tabs=netcore21#arguments)
SolutionName = name of the solution
Example:
.\New-DotNet CoolProject.Api CoolProject webapi
Generates:
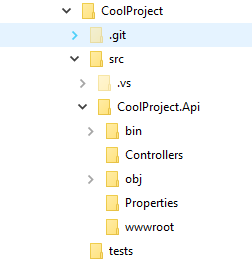