Created
January 11, 2018 19:42
-
-
Save busticated/1170897ccabeb566951a209d673b9eb1 to your computer and use it in GitHub Desktop.
React Context + GatsbyJS
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// src/components/Foo.js | |
import createClass from 'create-react-class'; | |
import { div, em } from 'react-dom-factories'; | |
import PropTypes from 'prop-types'; | |
export default createClass({ | |
displayName: 'Foo', | |
contextTypes: { | |
count: PropTypes.number | |
}, | |
render(){ | |
const { count } = this.context; | |
return div(null, | |
em(null, `COMPONENT COUNT: ${count}`), | |
); | |
} | |
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// src/layouts/index.js | |
import { createElement } from 'react'; | |
import createClass from 'create-react-class'; | |
import { div, h1 } from 'react-dom-factories'; | |
import PropTypes from 'prop-types'; | |
import Foo from '../components/Foo'; | |
export default createClass({ | |
displayName: 'TestLayout', | |
propTypes: { | |
children: PropTypes.func | |
}, | |
childContextTypes: { | |
count: PropTypes.number | |
}, | |
getChildContext(){ | |
return { | |
count: this.state.count | |
}; | |
}, | |
getInitialState(){ | |
return { count: 0 }; | |
}, | |
componentDidMount(){ | |
(function run(self){ | |
self.setState({ count: self.state.count += 1 }); | |
self._timer = setTimeout(run, 1000, self); | |
}(this)); | |
}, | |
componentWillUnmount(){ | |
if (this._timer){ | |
clearTimeout(this._timer); | |
} | |
}, | |
render(){ | |
const { count } = this.state; | |
const { children } = this.props; | |
return div(null, | |
h1(null, `COUNT: ${count}`), | |
createElement(Foo), | |
children() | |
); | |
} | |
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// src/pages/test.js | |
import createClass from 'create-react-class'; | |
import { div, p } from 'react-dom-factories'; | |
import PropTypes from 'prop-types'; | |
export default createClass({ | |
displayName: 'TestPage', | |
contextTypes: { | |
count: PropTypes.number | |
}, | |
render(){ | |
const { count } = this.context; | |
return div(null, | |
p(null, `PAGE COUNT: ${count}`), | |
); | |
} | |
}); |
This is just an example that context doesn't work in Gatsby, itn't a solution, right? I used a starter that uses context (gatsby-firebase-starter) where the author didn't know context doesn't work in Gatsby. He has another example of the app as a plain React app using React v16.1 where context does work. I thought that if I use the gatsby-react-next that it will allow for use of context, just by including the plugin, but not really...
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
since Gatsby's internal component renderer calls
shouldComponentUpdate()
without checking forcontext
changes, page updates are blocked.screenshots:
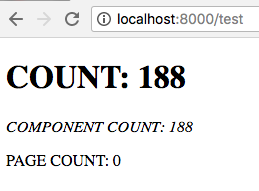
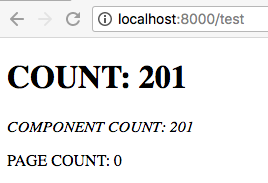