Last active
February 9, 2018 21:47
-
-
Save cesco89/fa436d54470db3cb2e0be656585bbd9d to your computer and use it in GitHub Desktop.
Java Maze Generator based on Maze Generation Algorithm --> https://en.wikipedia.org/wiki/Maze_generation_algorithm
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import java.awt.*; | |
import java.util.ArrayList; | |
public class Cell { | |
public int i; | |
public int j; | |
public int x; | |
public int y; | |
public boolean[] walls = {true, true, true, true}; //top, right, bottom, left | |
public boolean visited = false; | |
public Cell(int _i, int _j){ | |
this.i = _i; | |
this.j = _j; | |
} | |
public void show(int size, Graphics2D g) { | |
this.x = i*size; | |
this.y = j*size; | |
if(walls[0]) { | |
g.drawLine(x, y, x + size, y); | |
} | |
if(walls[1]) { | |
g.drawLine(x + size, y, x + size, y + size); | |
} | |
if(walls[2]) { | |
g.drawLine(x + size, y + size, x, y + size); | |
} | |
if(walls[3]) { | |
g.drawLine(x, y + size, x, y); | |
} | |
if(this.visited) { | |
Rectangle r = new Rectangle(x,y,size,size); | |
//g.drawRect(x,y,size,size); | |
g.setColor(new Color(50,0,250,50)); | |
g.fillRect(x,y,size,size); | |
g.setColor(Color.BLACK); | |
} | |
} | |
public Cell checkNeighbors(ArrayList<Cell> grid, int cols, int rows) { | |
ArrayList<Cell> neighbors = new ArrayList<>(); | |
Cell top = index(i,j-1, cols, rows)!= -1 ? grid.get(index(i,j-1, cols, rows)) : null; | |
Cell right = index(i+1,j,cols,rows) != -1 ? grid.get(index(i+1,j,cols,rows)) : null; | |
Cell bottom = index(i,j+1,cols,rows) != -1 ? grid.get(index(i,j+1,cols,rows)) : null; | |
Cell left = index(i-1,j,cols,rows) != -1 ? grid.get(index(i-1,j,cols,rows)) : null; | |
if(top != null && !top.visited) { | |
neighbors.add(top); | |
} | |
if(right != null && !right.visited) { | |
neighbors.add(right); | |
} | |
if(bottom != null && !bottom.visited) { | |
neighbors.add(bottom); | |
} | |
if(left != null && !left.visited) { | |
neighbors.add(left); | |
} | |
if(neighbors.size() >0 ){ | |
int r = (int)Math.floor(Math.random()*neighbors.size()); | |
return neighbors.get(r); | |
} | |
return null; | |
} | |
private int index(int i, int j, int cols, int rows) { | |
if(i<0 || j<0 || i>cols-1 || j> rows-1){ | |
return -1; | |
} | |
return i+j*cols; | |
} | |
public void highlight(int size, Graphics2D g) { | |
Rectangle r = new Rectangle(x,y,size,size); | |
//g.drawRect(x,y,size,size); | |
g.setColor(Color.GREEN); | |
g.fillRect(x,y,size,size); | |
g.setColor(Color.BLACK); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import javax.swing.*; | |
import java.awt.*; | |
public class Main { | |
public static void main (String... args){ | |
EventQueue.invokeLater(() -> { | |
JFrame frame = new JFrame("Maze Generator Algorithm"); | |
MazePanel mazePanel = new MazePanel(); | |
mazePanel.setPreferredSize(Toolkit.getDefaultToolkit().getScreenSize()); | |
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); | |
frame.add(mazePanel); | |
frame.pack(); | |
frame.setLocationRelativeTo(null); | |
frame.setVisible(true); | |
}); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import javax.swing.*; | |
import java.awt.*; | |
import java.awt.event.ActionEvent; | |
import java.awt.event.ActionListener; | |
import java.util.ArrayList; | |
import java.util.Random; | |
public class MazePanel extends JPanel implements ActionListener{ | |
private static int width = Toolkit.getDefaultToolkit().getScreenSize().width; | |
private static int height = Toolkit.getDefaultToolkit().getScreenSize().height-100; // minus 100 to avoid windows appbar overlapping on screen, i'm lazy AF | |
private int gridDim = 10; //grid aka cell size | |
private int numgenerators = 50; //the number of maze generators | |
private int rows = (int)Math.floor(height/gridDim); | |
private int cols = (int)Math.floor(width/gridDim); | |
private ArrayList<Cell> grid; //RLY!? this is the grid! | |
private ArrayList<ArrayList<Cell>> stack; //stack for every generator | |
private Cell[] currents; //this array saves the current position of every generator while it moves to the next element in the grid | |
private ArrayList<Color> randColors; // 'cause i'm lazy and it's funny | |
Timer timer=new Timer(1, this); //repaint timer | |
//constructor | |
public MazePanel() { | |
grid = new ArrayList<>(); | |
stack = new ArrayList<>(); | |
randColors = new ArrayList<>(); | |
for(int i = 0; i< numgenerators; i++) { | |
stack.add(new ArrayList<>()); | |
} | |
currents = new Cell[numgenerators]; | |
setup(); | |
timer.start(); | |
} | |
//setup all the things! | |
public void setup(){ | |
for(int j = 0; j<rows; j++) { | |
for(int i = 0; i<cols; i++) { | |
grid.add(new Cell(i,j)); | |
} | |
} | |
for(int x = 0; x<numgenerators; x++){ | |
currents[x] = grid.get(pickIndex()); | |
randColors.add(generateCcolor()); | |
} | |
} | |
//here comes the fun stuff | |
void drawGrid(Graphics g) { | |
Graphics2D g2d = (Graphics2D) g; | |
//generate the main grid | |
for(int i = 0; i<grid.size(); i++) { | |
grid.get(i).show(gridDim,g2d); | |
} | |
//Loop through every current element to move it to the next one | |
for(int k = 0; k<currents.length; k++ ) { | |
currents[k].setVisitedColor(randColors.get(k)); //setting the color for every generator's maze | |
currents[k].visited = true; //mark the current cell as visited | |
currents[k].highlight(gridDim, g2d); //highlight the current cell (green) | |
Cell next = currents[k].checkNeighbors(grid, cols, rows); //check if there is a neighbor unvisited | |
if(next != null) { | |
next.visited = true; | |
stack.get(k).add(currents[k]); //add the current to the stack | |
removeWalls(currents[k], next); //remove walls from current and next | |
currents[k] = next; //set the next as the current | |
}else if(stack.get(k).size()>0){ | |
currents[k] = stack.get(k).get(stack.get(k).size()-1); //we dont' have unvisited cell, pop back the last one from the stack and move to it | |
stack.get(k).remove(stack.get(k).size()-1); //delete it, so we can move to the previous visited one | |
} | |
} | |
//this.repaint(); | |
} | |
//necessary stuff | |
public void paint(Graphics g) { | |
super.paint(g); | |
drawGrid(g); | |
} | |
//given current and next cell, check and remove walls from each one | |
private void removeWalls(Cell a, Cell b) { | |
int x = a.i-b.i; | |
if(x == 1) { | |
a.walls[3] = false; | |
b.walls[1] = false; | |
}else if(x == -1) { | |
a.walls[1] = false; | |
b.walls[3] = false; | |
} | |
int y = a.j-b.j; | |
if(y == 1) { | |
a.walls[0] = false; | |
b.walls[2] = false; | |
}else if(y == -1) { | |
a.walls[2] = false; | |
b.walls[0] = false; | |
} | |
} | |
//generate random color (LAZINESS) | |
private Color generateCcolor() { | |
Random rand = new Random(); | |
int r = rand.nextInt(255); | |
int g = rand.nextInt(255); | |
int b = rand.nextInt(255); | |
return new Color(r,g,b, 80); | |
} | |
//Pick a random index as a starting point | |
private int pickIndex() { | |
return new Random().nextInt(grid.size()); | |
} | |
//needed for the timer | |
@Override | |
public void actionPerformed(ActionEvent actionEvent) { | |
if(actionEvent.getSource()==timer){ | |
repaint();// this will call at a given interval | |
} | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Updated gist.
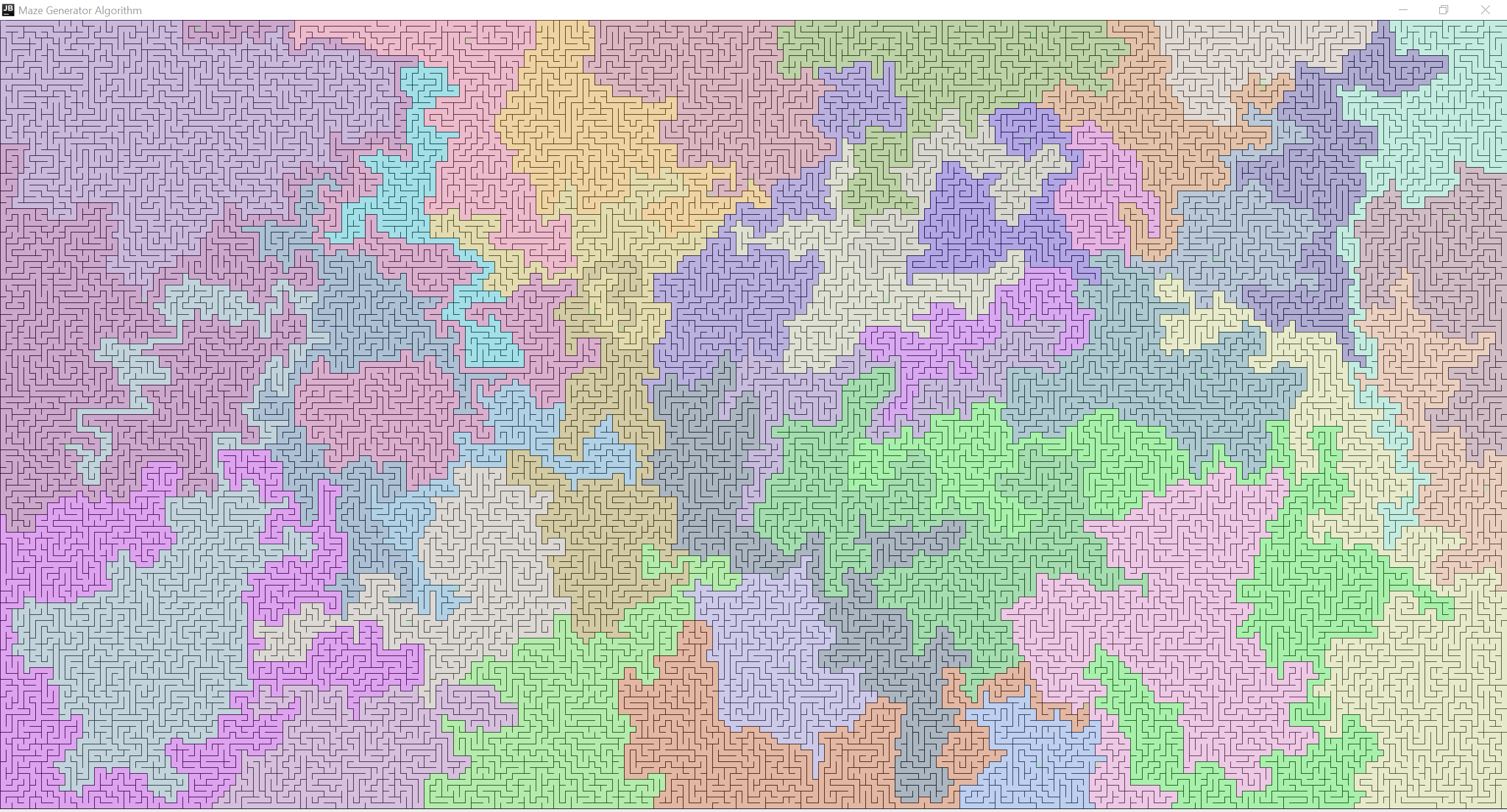
Simply give the number of maze generators you want, the cell size and watch the magic