-
-
Save chaodonghu/e4775571f30b2b0f990d0759822ba4c1 to your computer and use it in GitHub Desktop.
// Run GOOGLE CHROME - WORKING AS OF DEC 26 2023 | |
// Please @ me in the comments if this stops working, I will try to get it working again within the month | |
// INSTRUCTIONS | |
// 1. Open Instagram in Chrome | |
// 2. Search for a hashtag (eg. https://www.instagram.com/explore/tags/hikingadventures/) | |
// 3. Click on a photo to reveal the like button as well as arrows for navigating between photos | |
// 3. Open developer tools by right clicking on the page and clicking "INSPECT" | |
// 4. Copy the code below and paste in the developer tools console and press enter to run | |
// 5. Script will not run if tab is navigated away from, minimized of unfocused (It is recommended to open a new chrome window or push tab to the side and let script run in background) | |
// SCRIPT WILL CLICK ON "LIKE" BUTTON AND THEN NAVIGATE TO THE FOLLOWING POST | |
const likeAndClickPosts = (async () => { | |
// Modify these variables to your liking | |
const LIKE_LIMIT = 800; | |
const BREAK_DURATION = 5 * 60 * 1000; // 5 minutes break | |
const TOTAL_DURATION = 10 * 60 * 1000; // 10 minutes duration - Timeout after 10 minutes | |
const delay = (ms) => new Promise((resolve) => setTimeout(resolve, ms)); | |
console.log("Start like posts script..."); | |
let startTime = new Date().getTime(); | |
while (new Date().getTime() - startTime < TOTAL_DURATION) { | |
for (let i = 0; i < LIKE_LIMIT; i++) { | |
// GET LIKE BUTTON | |
const firstLike = document | |
.querySelector('svg[aria-label="Like"]') | |
.closest('[role="button"]'); | |
if (firstLike) { | |
// SCROLL LIKE BUTTON INTO VIEW | |
firstLike.scrollIntoViewIfNeeded(); | |
firstLike.click(); | |
console.log("Clicked like button...\n"); | |
} | |
await delay(100); | |
// CLICK PAGINATION ARROW | |
const nextArrow = document | |
.querySelector('svg[aria-label="Next"]') | |
.closest('[type="button"]'); | |
if (nextArrow) { | |
await nextArrow.click(); | |
} | |
// Increase LIKE_INTERVAL and/or BREAK_DURATION if you are getting rate limited | |
const LIKE_INTERVAL = Math.floor(Math.random() * 10 + 1) * 5000; // Set this to 0 to like as many posts as quickly as possible - not recommended | |
console.log( | |
`Wait ${Math.floor(Math.random() * 10 + 1) * 5000} milliseconds` | |
); | |
await delay(LIKE_INTERVAL); | |
console.log(`Liked #${i + 1} post(s) so far...`); | |
} | |
console.log(`Taking a break for ${BREAK_DURATION / 1000} seconds...`); | |
await delay(BREAK_DURATION); // Take a break to avoid rate limiting | |
startTime = new Date().getTime(); // Reset start time for the next cycle | |
} | |
console.log("Like script complete!"); | |
})(); |
Could it be possible to see if a Button Follow is founded and if not scroll until a Button is founded ?
I get several error with scrolling if there is no Follow button visible
Could it be possible to see if a Button Follow is founded and if not scroll until a Button is founded ?
I get several error with scrolling if there is no Follow button visible
Hey would you be able to post a screenshot of the error? The tab either has to be open while running the script or you can have it in the background but you can’t navigate to another tab or minimize the window while the script is running for it to work.
There should be no scrolling needed since upon clicking “next” the like heart icon should be visible
I need to scroll down all the list to load all the follow buttons and the error don't came anymore
Hi, Is there a mass Like for Twitter?
@WilliamLuppi Probably if you search around on github there will likely be someone who created something similar for twitter. I don't use twitter alot so I probably won't invest time in making a similar script for the twitter interface sorry.
Getting this error not sure why what i am doing wrong .
![]()
Hey @denmoex, I might have to update this script as it could be out of date due to instagram's update. Right now the only script that is working is my follow script. I'll have some time later this month to fix this one
Amazing thanks .. I got u coffe lol
Den
This script should be working again as of Dec 26 2023.
If this helped you, please leave a comment below or support me at:
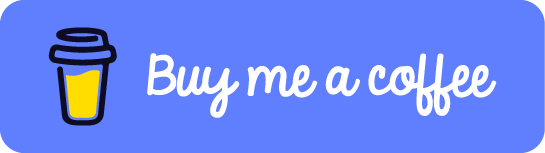