Last active
November 7, 2019 07:48
-
-
Save chriskyfung/a447d2ba9f9e9c129a77e3df2949440a to your computer and use it in GitHub Desktop.
Userscript for f5sys.com Binary plan calculator extension
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// ==UserScript== | |
// @name binary plan calculator extension | |
// @namespace https://chriskyfung.github.io | |
// @version 0.1 | |
// @description Userscript for f5sys.com Binary plan calculator extension | |
// @author Chris KY Fung (https://chriskyfung.github.io) | |
// @match http://www.f5sys.com/calculation/binary+plan+calculator | |
// @grant none | |
// ==/UserScript== | |
function get_total_business(n) { | |
'use strict'; | |
let row = n-13; | |
let x = parseInt(document.querySelector('#form1 > table:nth-child(4) > tbody > tr:nth-child(' + row + ') > td:nth-child(2)').innerHTML); | |
console.log(x); | |
return x; | |
}; | |
function get_binary_payout(n) { | |
'use strict'; | |
let row = n-12; | |
let x = parseInt(document.querySelector('#form1 > table:nth-child(4) > tbody > tr:nth-child(' + row + ') > td:nth-child(2)').innerHTML.replace('-','')); | |
console.log(x); | |
return x; | |
}; | |
function get_total_members(n) { | |
'use strict'; | |
let row = n-14; | |
let x = (document.querySelector('#form1 > table:nth-child(4) > tbody > tr:nth-child(' + row + ') > td:nth-child(2) > strong').innerHTML); | |
console.log(x); | |
return x; | |
}; | |
function payout_ratio() { | |
let n = f.getElementsByTagName('tr').length; | |
console.log(n); | |
let total_members = get_total_members(n); | |
let sales_commission = 12000; | |
let total_sales_commission = sales_commission * total_members; | |
let binary_payout = get_binary_payout(n); | |
let total_payout = binary_payout + total_sales_commission; | |
let revenue = total_sales_commission + get_total_business(n); | |
let payout_ratio = total_payout / revenue * 100; | |
console.log(); | |
p.innerHTML = '<ul><li>Total members = ' + total_members + '</li><li>Sales commission = ' | |
+ sales_commission + '</li><hr><li>Total commission = ' + -total_sales_commission + '<li>Binary payout = ' + -binary_payout + '</li>' | |
+ '</li><li>BP+SC = ' + -total_payout + '</li><hr><li>Revenue = ' + revenue + '</li><li>Payout ratio = ' + payout_ratio + '%</li></ui>'; | |
}; | |
// 1. Create the button | |
var button = document.createElement("button"); | |
button.type = "button"; | |
button.innerHTML = "More..."; | |
button.style.cssFloat = "right"; | |
//button.addEventListener("click", get_total_business); | |
//button.addEventListener("click", get_net_profit); | |
button.addEventListener("click", payout_ratio); | |
var d = document.createElement("div"); | |
d.style.cssFloat = "right"; | |
var p = document.createElement("p"); | |
var f = document.getElementById("form1"); | |
//console.log(f); | |
d.appendChild(p); | |
d.appendChild(button); | |
f.appendChild(d); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Userscript for f5sys.com Binary plan calculator extension
Show a readable summary at the bottom-right of the f5sys.com Binary plan calculator page.
The extra values
Press More... to run the script, after the binary plan output shown.
Demo image
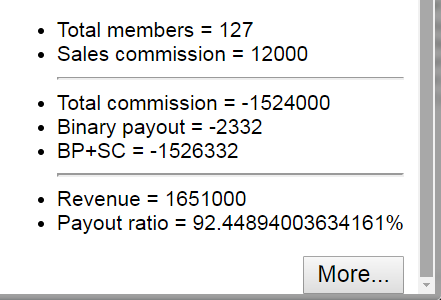