-
-
Save claudioc/94805ded10a1040280a1e9b9528d17fd to your computer and use it in GitHub Desktop.
function getUpdates() { | |
updateCell("B3", "ripple") | |
updateCell("C3", "bitcoin") | |
updateCell("D3", "ethereum") | |
updateCell("E4", "litecoin") | |
updateCell("F4", "iota") | |
} | |
function updateCell(cell, code) { | |
const response = UrlFetchApp.fetch("https://api.coinmarketcap.com/v1/ticker/" + code + "/?convert=EUR") | |
const data = JSON.parse(response)[0] | |
const sheet = SpreadsheetApp.getActive().getActiveSheet() | |
const range = sheet.getRange(cell) | |
const [ p1, p2 ] = cell.split('') | |
const percRange = sheet.getRange(p1 + ++p2) | |
percRange.setValue(data.percent_change_24h + '%') | |
percRange.setBackground(data.percent_change_24h > 0 ? "#c8e6c9" : "#ffcdd2") | |
range.setValue((+data.price_eur).toFixed(2)) | |
} |
Made some more changes. Now it takes the code of the cc directly from the sheet. In this way the user can change the currency in the sheet without editing the script.
function getUpdates() {
updateCell("B")
updateCell("C")
updateCell("D")
updateCell("E")
updateCell("F")
updateCell("G")
updateCell("H")
}
function updateCell(column) {
const sheet = SpreadsheetApp.getActive().getActiveSheet()
const codeRange = sheet.getRange(column + "1")
const response = UrlFetchApp.fetch("https://api.coinmarketcap.com/v1/ticker/" + codeRange.getValue() + "/?convert=EUR")
const data = JSON.parse(response)[0]
const rangePrice = sheet.getRange(column + "3")
rangePrice.setValue((+data.price_eur).toFixed(2))
const percRange = sheet.getRange(column + "4")
percRange.setValue(data.percent_change_24h + '%')
}
My take on this. Simplified and removed hardcoded column letters, specifying the range instead
function getUpdates() {
const sheet = SpreadsheetApp.getActive().getActiveSheet();
// Update the range based on the total number of cryptocurrencies you have in the spreadsheet
const range = sheet.getRange('B1:H1');
const cells = [].concat.apply([], Array(range.getNumColumns())).map(function (x, i) {
return String.fromCharCode(66 + i);
});
cells.forEach(updateCell);
}
function updateCell(column) {
const sheet = SpreadsheetApp.getActive().getActiveSheet();
const codeRange = sheet.getRange(column + '1');
const response = UrlFetchApp.fetch('https://api.coinmarketcap.com/v1/ticker/' + codeRange.getValue() + '/?convert=EUR');
const data = JSON.parse(response)[0];
const priceRange = sheet.getRange(column + '3');
priceRange.setValue((+data.price_eur).toFixed(2));
const percChangeRange = sheet.getRange(column + '4');
const priceChangeRange = sheet.getRange(column + '5');
const bgColour = data.percent_change_24h > 0 ? '#c8e6c9' : '#ffcdd2';
percChangeRange.setValue(data.percent_change_24h + '%');
percChangeRange.setBackground(bgColour);
priceChangeRange.setBackground(bgColour);
}
Instead of colorizing the cells at line 17, I use conditional formatting in the spreadsheet to colorize font colors. I use the background to indicate that the cells are calculated by a script.
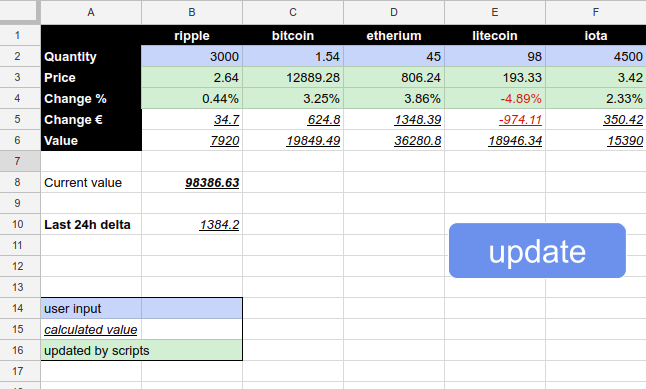