Last active
October 27, 2020 07:01
-
-
Save danialkalbasi/350d960a6d28016a3331f6a9c7baefa4 to your computer and use it in GitHub Desktop.
Power Translator Demo
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React, { Component } from 'react'; | |
import { PowerTranslator, ProviderTypes, TranslatorConfiguration } from 'react-native-power-translator'; | |
import { View, ScrollView, TouchableOpacity, Text } from 'react-native'; | |
export default class PowerTranslatorDemo extends Component { | |
constructor() { | |
super(); | |
this.state = { languageCode: 'fr' }; | |
} | |
render() { | |
const styles = this.getStyles(); | |
TranslatorConfiguration.setConfig(ProviderTypes.Google, 'AIzaSyB5ip6KC-9KCIjO9Q7Rm47dYJDmOdjLgM0', this.state.languageCode); | |
return ( | |
<ScrollView style={styles.container}> | |
<View style={styles.languageBar}> | |
<TouchableOpacity onPress={() => { this.changeLanguage('en') }}><Text style={styles.p}>English</Text></TouchableOpacity> | |
<TouchableOpacity onPress={() => { this.changeLanguage('fr') }}><Text style={styles.p}>French</Text></TouchableOpacity> | |
<TouchableOpacity onPress={() => { this.changeLanguage('ru') }}><Text style={styles.p}>Russian</Text></TouchableOpacity> | |
<TouchableOpacity onPress={() => { this.changeLanguage('de') }}><Text style={styles.p}>German</Text></TouchableOpacity> | |
</View> | |
<View> | |
<PowerTranslator style={styles.title} text={'A Confucian Revival Began'} /> | |
<PowerTranslator style={styles.subtitle} text={'Author: Confucianism'} /> | |
</View> | |
<View style={styles.section}> | |
<PowerTranslator style={styles.p} text={'Confucianism was particularly strong during the Han Dynasty, whose greatest thinker was Dong Zhongshu, who integrated Confucianism with the thoughts of the Zhongshu School and the theory of the Five Elements. He also was a promoter of the New Text school, which considered Confucius as a divine figure and a spiritual ruler of China, who foresaw and started the evolution of the world towards the Universal Peace.'} /> | |
</View> | |
<View style={styles.section}> | |
<PowerTranslator style={styles.p} text={'Confucianism was particularly strong during the Han Dynasty, whose greatest thinker was Dong Zhongshu, who integrated Confucianism with the thoughts of the Zhongshu School and the theory of the Five Elements. He also was a promoter of the New Text school, which considered Confucius as a divine figure and a spiritual ruler of China, who foresaw and started the evolution of the world towards the Universal Peace.'} /> | |
</View> | |
<View style={styles.section}> | |
<PowerTranslator style={styles.p} text={'Engineering physics or engineering science refers to the study of the combined disciplines of physics'} /> | |
</View> | |
</ScrollView> | |
); | |
} | |
getStyles() { | |
return { | |
container: { | |
padding: 40, | |
backgroundColor: '#FAFAFA', | |
}, | |
section: { | |
marginTop: 15, | |
marginBottom: 15, | |
}, | |
title: { | |
marginTop: 80, | |
marginBottom: 5, | |
fontWeight: 'bold', | |
fontSize: 38, | |
lineHeight: 38 | |
}, | |
subtitle: { | |
color: '#B3B3B3', | |
}, | |
p: { | |
color: '#828280', | |
lineHeight: 24 | |
}, | |
languageBar: { | |
flexDirection: 'row', | |
justifyContent: 'space-between' | |
}, | |
languageBarItem: { | |
color: '#828280', | |
} | |
} | |
} | |
changeLanguage(languageCode) { | |
this.setState({ languageCode: languageCode }); | |
} | |
} |
Only getting warning when sending wrong language code .
TranslatorFactory.createTranslator()
.translate(MainLanguages[currentKey], langCode)
.then(translated => {})
Possible Unhandled Promise Rejection (id: 0):
TypeError: Cannot read property 'translations' of undefined
Why does your Demo show the translated text without the quotations around it but when I use it it has ugly quotes around all the text?
--Possible Unhandled Promise Rejection (id: 0):
--TypeError: Cannot read property 'translations' of undefined..
Getting this issue. anyone able to Fixthis ?
for me am getting unable TypeError: undefined is not an object (evaluating 'translator.translate') any answers plz
@harsha-kotapati This power translator didnt work for me. so check the above link for a solution
danialkalbasi/react-native-power-translator#18
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Pls guide I got this error,
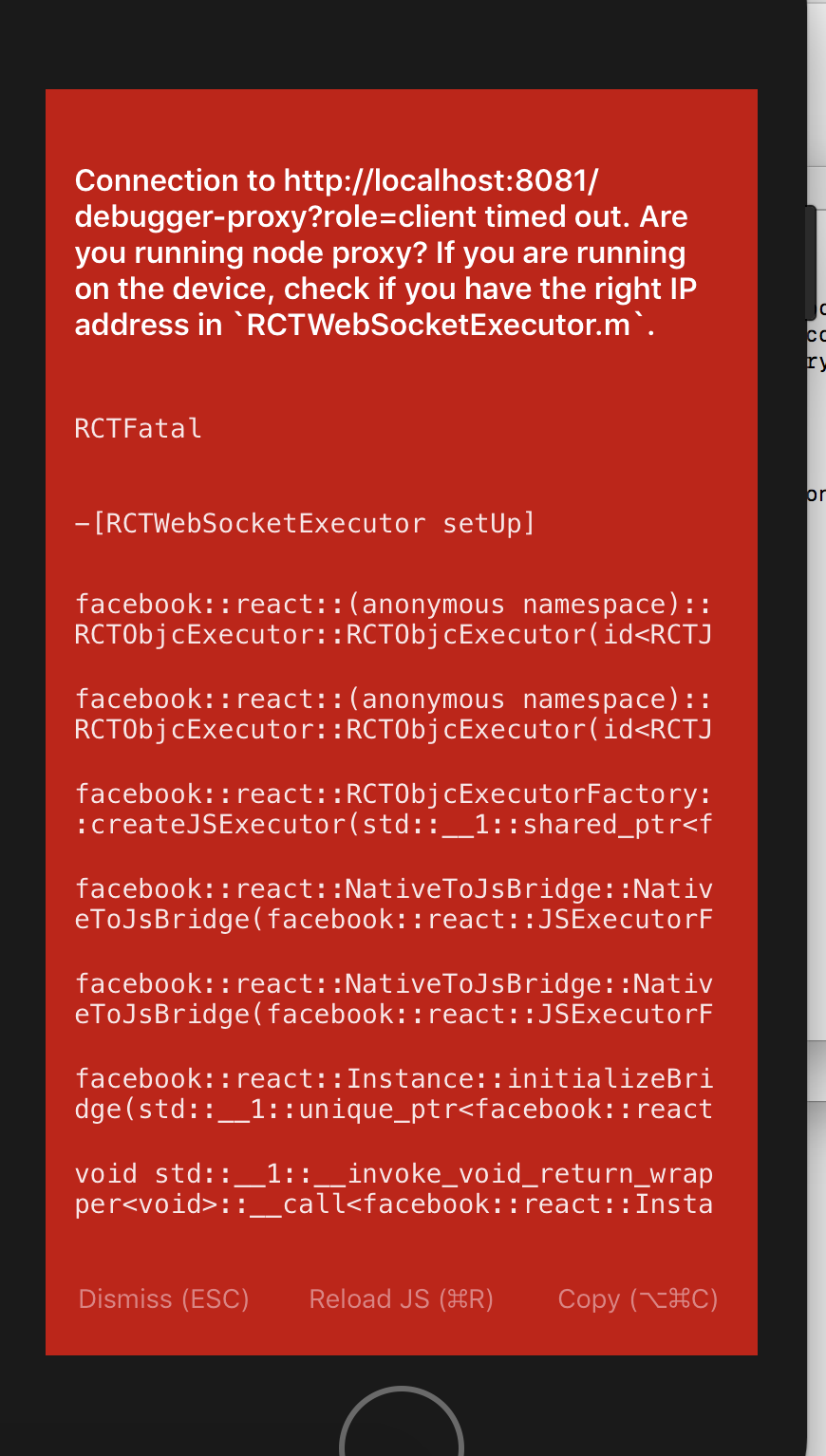
my email id is archana.whatkar@gmail.com