Created
May 11, 2019 20:21
-
-
Save dannypage/df8885cc2dac5ce79ab5c29d2ac8a5a6 to your computer and use it in GitHub Desktop.
A sample DragonRuby program to display a few learnings; How to include new classes, create buttons to update state, manage the update loop, and easily add new color schemes.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class ColorPalatte | |
attr_accessor :colors | |
def initialize clrs | |
clrs.each_with_index do |c, i| | |
clrs[i] = hex_to_rgb c | |
end | |
@colors = clrs | |
end | |
def get_color n | |
return @colors[n] | |
end | |
def get_colors | |
@colors | |
end | |
def hex_to_rgb hex | |
r = hex[0..1].hex | |
g = hex[2..3].hex | |
b = hex[4..5].hex | |
[r,g,b] | |
end | |
end | |
# As seen on http://clrs.cc/css/colors.css | |
$AQUA = '7FDBFF' | |
$BLUE = '0074D9' | |
$NAVY = '001F3F' | |
$GREEN = '2ECC40' | |
$TEAL = '39CCCC' | |
$OLIVE = '3D9970' | |
$LIME = '01FF70' | |
$MAROON = '85144B' | |
$PURPLE = 'B10DC9' | |
$RED = 'FF4136' | |
$YELLOW = 'FFDC00' | |
$FUCHSIA= 'F012BE' | |
$SILVER = 'DDDDDD' | |
$GRAY = '444444' | |
$BLACK = '111111' | |
$WHITE = 'FFFFFF' | |
$grayscale = [$BLACK, $GRAY, $SILVER, $WHITE] | |
$megaman = [$NAVY, $BLUE, $AQUA, $SILVER] | |
$bright = [$RED, $LIME, $TEAL, $YELLOW] | |
$red_n_blue= [$RED, $MAROON, $BLUE, $NAVY] | |
$reds = [$MAROON, $PURPLE, $RED, $YELLOW, $FUCHSIA] | |
# Other potential palattes on https://lospec.com/palette-list | |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
$dragon.require('app/color_palatte.rb') | |
class PalatteExample | |
attr_accessor :grid, :inputs, :game, :outputs, :dragon | |
def tick | |
defaults | |
render | |
# comment next line to stop the colors cycling every n seconds | |
updates 4 | |
input_mouse | |
end | |
def render | |
# Todo: Center the buttons and colors, especially as more get added. (Maybe even a second row) | |
game.palatte = game.palattes[game.loaded_pallate] | |
game.palatte.get_colors.each_with_index do |c, i| | |
outputs.solids << [grid.w * 0.405 + 60*i, 300, 50, 50, c ] | |
end | |
outputs.labels << [ grid.w * 0.5, 600, "Color scheme loaded: #{game.loaded_pallate}", 0, 1 ] | |
outputs.labels << [ grid.w * 0.5, 550, "First color: #{game.palatte.get_color(0)}", 0, 1] | |
outputs.labels << [ grid.w * 0.5, 500, "Keys: #{game.palattes.keys.map { |e| e.to_s.capitalize }}", 0, 1] | |
game.palattes.keys.each_with_index do |p, i| | |
game.palatte_buttons[i] ||= palatte_button(p, i + 1) | |
outputs.labels << game.palatte_buttons[i].label | |
outputs.borders << game.palatte_buttons[i].border | |
end | |
end | |
def input_mouse | |
return unless inputs.mouse.click | |
button_clicked = outputs.borders.find_all do |b| | |
inputs.mouse.click.point.inside_rect? b | |
end.reject {|b| !game.meta(b)}.first | |
return unless button_clicked | |
label_clicked = game.meta(button_clicked) | |
if game.palattes.keys.include? label_clicked | |
game.loaded_pallate = label_clicked | |
end | |
end | |
def palatte_button name, position | |
game.new_entity(:button) do |b| | |
# Labels (x, y, text, size, align, r, g, b, a) | |
b.label = [grid.w * 0.30 + 105.mult(position), grid.h * 0.6, "#{name.capitalize}", 0, 1, 0, 0, 0] | |
# Borders (x, y, w, h, r, g, b, a) | |
b.border = game.with_meta([grid.w * 0.30 + 105.mult(position) - 50, grid.h * 0.6 - 32, 100, 50], name) | |
end | |
end | |
def updates n | |
if (game.tick_count % (n*60)) == 0 | |
a = game.palattes.keys | |
l = game.loaded_pallate | |
game.loaded_pallate = a[a.find_index(l) + 1] || a[0] | |
end | |
end | |
def defaults | |
# Create the array of colors in app/color_palatte.rb, then include it here | |
game.palattes ||= { | |
grayscale: ColorPalatte.new($grayscale), | |
megaman: ColorPalatte.new($megaman), | |
bright: ColorPalatte.new($bright), | |
red_n_blue: ColorPalatte.new($red_n_blue), | |
reds: ColorPalatte.new($reds) | |
} | |
game.loaded_pallate ||= :megaman | |
game.palatte_buttons ||= [] | |
end | |
end | |
$palatte_example = PalatteExample.new | |
def tick args | |
$palatte_example.grid = args.grid | |
$palatte_example.inputs = args.inputs | |
$palatte_example.game = args.game | |
$palatte_example.outputs = args.outputs | |
$palatte_example.dragon = args.dragon | |
$palatte_example.tick | |
end |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Sample image as of Revision 1 -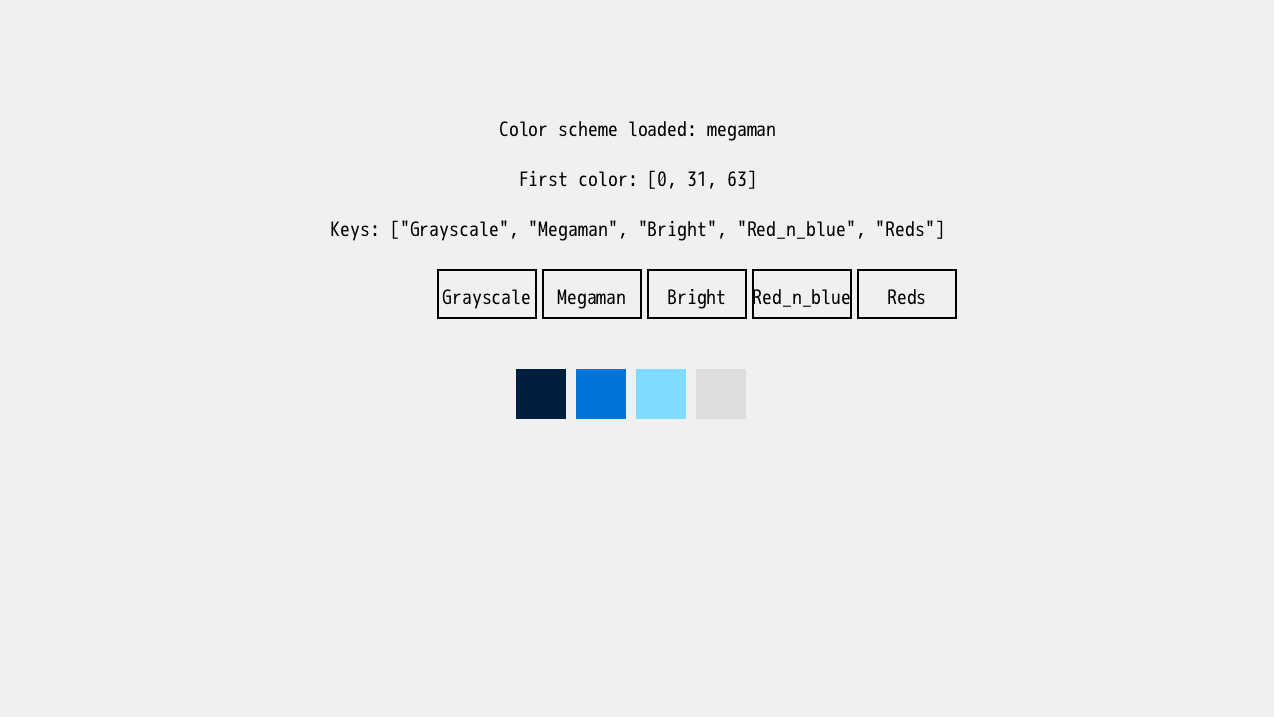