Created
November 25, 2015 14:49
-
-
Save danrigsby/79579d03cffae760f43d to your computer and use it in GitHub Desktop.
React Native Local Storage Wrapper
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React from 'react-native'; | |
var { | |
AsyncStorage | |
} = React; | |
var LocalStorage = { | |
get: function (key) { | |
return AsyncStorage.getItem(key).then(function(value) { | |
return JSON.parse(value); | |
}); | |
}, | |
save: function (key, value) { | |
return AsyncStorage.setItem(key, JSON.stringify(value)); | |
}, | |
update: function (key, value) { | |
return LocalStorage.get(key).then((item) => { | |
// if current value is a string, then overwrite; else merge objects | |
let v = (typeof value === 'string') ? value : Object.assign({}, item, value); | |
return AsyncStorage.setItem(key, JSON.stringify(v)); | |
}); | |
}, | |
delete: function (key) { | |
return AsyncStorage.removeItem(key); | |
} | |
}; | |
export default LocalStorage; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hello
I tried and I stuck on getItem from LocalStorage
This is my code:
LocalStorage.save('text', 'Hello');
console.log(LocalStorage.get('text'));
and this is the result
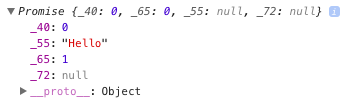
So how to get exactly the String value "Hello"