Last active
March 1, 2023 14:08
-
-
Save dcooney/6172abd98d6940e162d0a01a16b7ec34 to your computer and use it in GitHub Desktop.
Add Taxonomy Filters to WordPress Media Library Modal
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
addEventListener('DOMContentLoaded', () => { | |
const taxonomies = window.media_taxonomies || {}; // Pluck the taxonomies from the localized script. | |
const AttachmentsBrowser = wp.media.view.AttachmentsBrowser; | |
const AttachmentFilters = wp.media.view.AttachmentFilters; | |
const mediaFilterClasses = {}; // Filter class storage. | |
if (!taxonomies?.length || !AttachmentsBrowser || !AttachmentFilters) { | |
// Bail if filters or classes don't exist. | |
return; | |
} | |
// Loop each taxonomy filter. | |
taxonomies.forEach(function (tax) { | |
mediaFilterClasses[tax.slug] = wp.media.view.AttachmentFilters.extend({ | |
id: 'media-taxonomy-filters', | |
createFilters() { | |
const filters = {}; | |
const terms = tax?.terms || {}; | |
let index = 0; | |
// Loop over terms in taxonomy to format the 'terms'. | |
for (const term in terms) { | |
filters[index] = { | |
text: terms[term]?.name, | |
props: { | |
[tax.slug]: terms[term]?.slug, | |
}, | |
}; | |
++index; | |
} | |
// Add `All` filter. | |
filters.all = { | |
text: `-- ${tax.label} --`, | |
props: { | |
[tax.slug]: '', | |
}, | |
priority: 10, | |
}; | |
this.filters = filters; // Assign filters to class. | |
}, | |
}); | |
}); | |
// Add filters to media modal. | |
wp.media.view.AttachmentsBrowser = wp.media.view.AttachmentsBrowser.extend({ | |
createToolbar() { | |
// Load the original toolbar. | |
AttachmentsBrowser.prototype.createToolbar.call(this); | |
if (mediaFilterClasses) { | |
for (const key in mediaFilterClasses) { | |
// Loop each taxonomy and toolbar filter. | |
this.toolbar.set( | |
key, | |
new mediaFilterClasses[key]({ | |
controller: this.controller, | |
model: this.collection.props, | |
priority: -75, | |
}).render() | |
); | |
} | |
} | |
}, | |
}); | |
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
/** | |
* Custom media library functionality. | |
*/ | |
/** | |
* Enqueue media library scripts. | |
*/ | |
function enqueue_media_library_scripts() { | |
wp_enqueue_script( | |
'media-taxonomy-filters', | |
get_template_directory_uri() . 'build/admin/media-library.js', | |
[ 'media-editor', 'media-views' ], | |
'1.0', | |
false | |
); | |
// Array of media taxonomy filters. | |
$taxonomies = [ | |
[ | |
'label' => 'Media Type', | |
'slug' => 'media_type', | |
'terms' => get_terms( 'media_type', [ 'hide_empty' => true ] ), | |
], | |
[ | |
'label' => 'Line of Business', | |
'slug' => 'media_line_of_buisness', | |
'terms' => get_terms( 'media_line_of_buisness', [ 'hide_empty' => true ] ), | |
], | |
]; | |
// Load media taxonomy terms into a localized JavaScript variable. | |
wp_localize_script( | |
'media-taxonomy-filters', | |
'media_taxonomies', | |
$taxonomies | |
); | |
// Overrides code styling to accommodate for a third dropdown filter. | |
add_action( | |
'admin_footer', | |
function() { | |
?> | |
<style> | |
.media-modal-content .media-frame select.attachment-filters, | |
body.block-editor-page .media-frame select.attachment-filters:last-of-type { | |
max-width: calc(33% - 12px); | |
} | |
</style> | |
<?php | |
} | |
); | |
} | |
add_action( 'wp_enqueue_media', __NAMESPACE__ . '\enqueue_media_library_scripts' ); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
The above code will result in this:
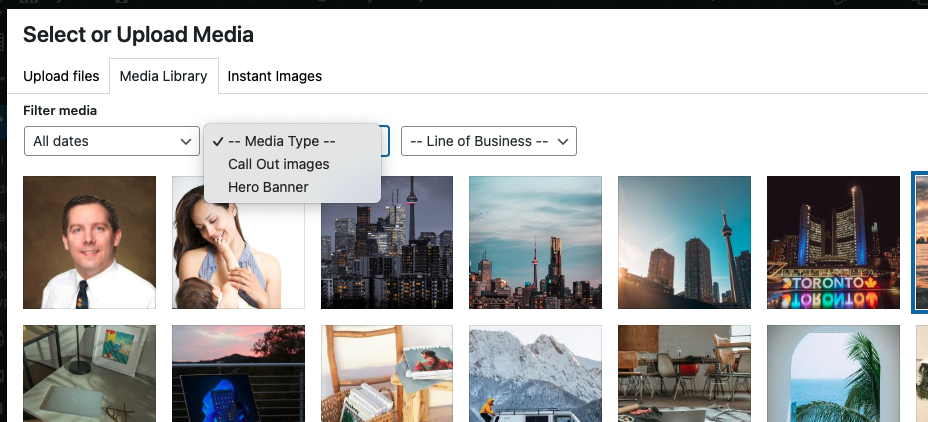