Created
May 30, 2017 04:29
-
-
Save delirious-lettuce/324fdb51f1fdd0cfd76bfd257ea17e05 to your computer and use it in GitHub Desktop.
Response to `https://stackoverflow.com/questions/44252745`
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import sys | |
import urllib.request | |
from PyQt5.QtCore import * | |
from PyQt5.QtWidgets import * | |
class Downloader(QDialog): | |
def __init__(self): | |
QDialog.__init__(self) | |
layout = QVBoxLayout() | |
self.url = QLineEdit() | |
self.save_location = QLineEdit() | |
self.progress = QProgressBar() | |
download = QPushButton("Download") | |
self.progress.setValue(0) | |
self.progress.setAlignment(Qt.AlignHCenter) | |
self.url.setPlaceholderText("URL") | |
self.save_location.setPlaceholderText("Save folder:") | |
layout.addWidget(self.url) | |
layout.addWidget(self.save_location) | |
layout.addWidget(self.progress) | |
layout.addWidget(download) | |
self.setLayout(layout) | |
self.setWindowTitle("Downloader") | |
download.clicked.connect(self.download) | |
def download(self): | |
url = self.url.text() | |
save_location = self.save_location.text() | |
urllib.request.urlretrieve(url, save_location, self.report) | |
try: | |
urllib.request.urlretrieve(url, save_location, self.report) | |
except Exception: | |
QMessageBox.warning(self, "Warning", "The download failed") | |
return | |
QMessageBox.information(self, "Information", "Download is complete") | |
self.progress.setValue(0) | |
self.url.setText("") | |
self.save_location.setText("") | |
def report(self, blocknum, blocksize, totalsize): | |
readsofar = blocknum * blocksize | |
if totalsize > 0: | |
percent = readsofar * 100 / totalsize | |
self.progress.setValue(int(percent)) | |
if __name__ == '__main__': | |
app = QApplication(sys.argv) | |
dl = Downloader() | |
dl.show() | |
app.exec_() | |
sys.exit(app.exec_()) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Didn't work for me...
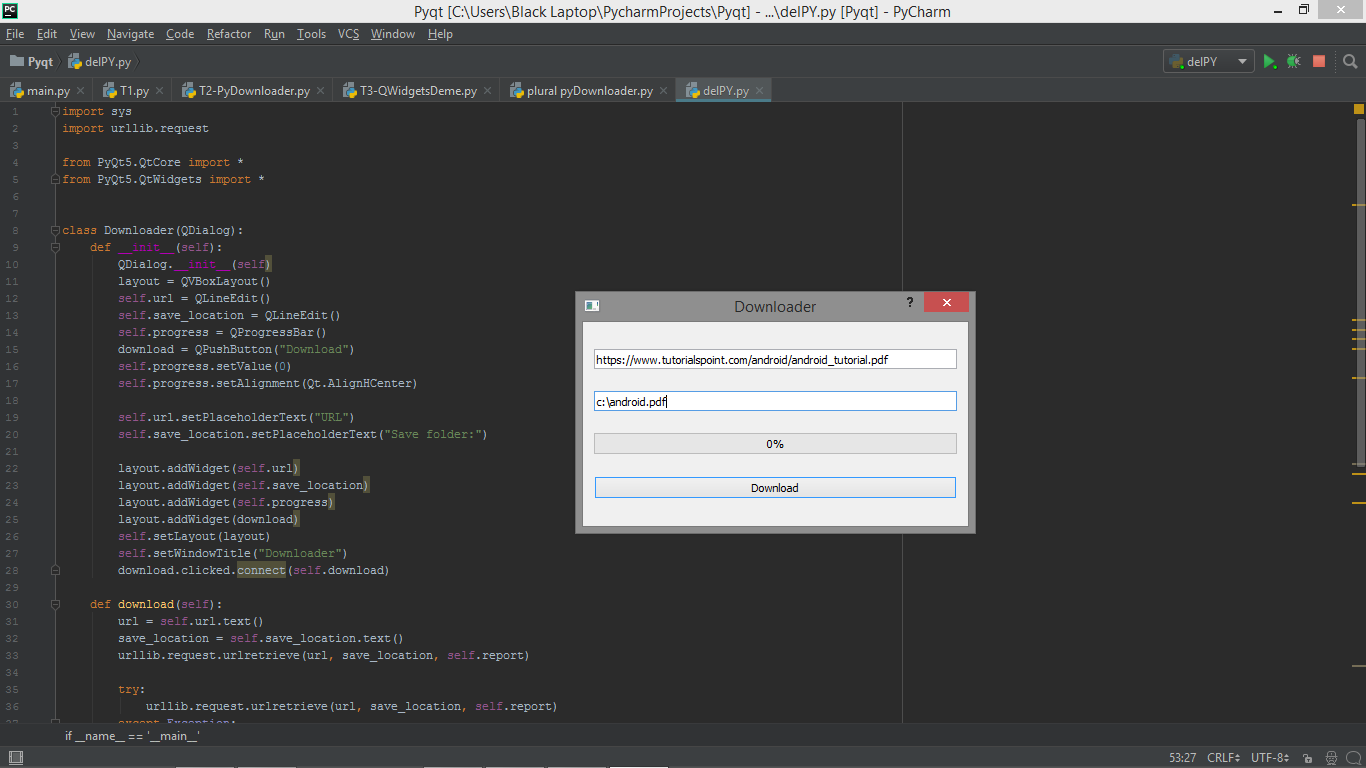
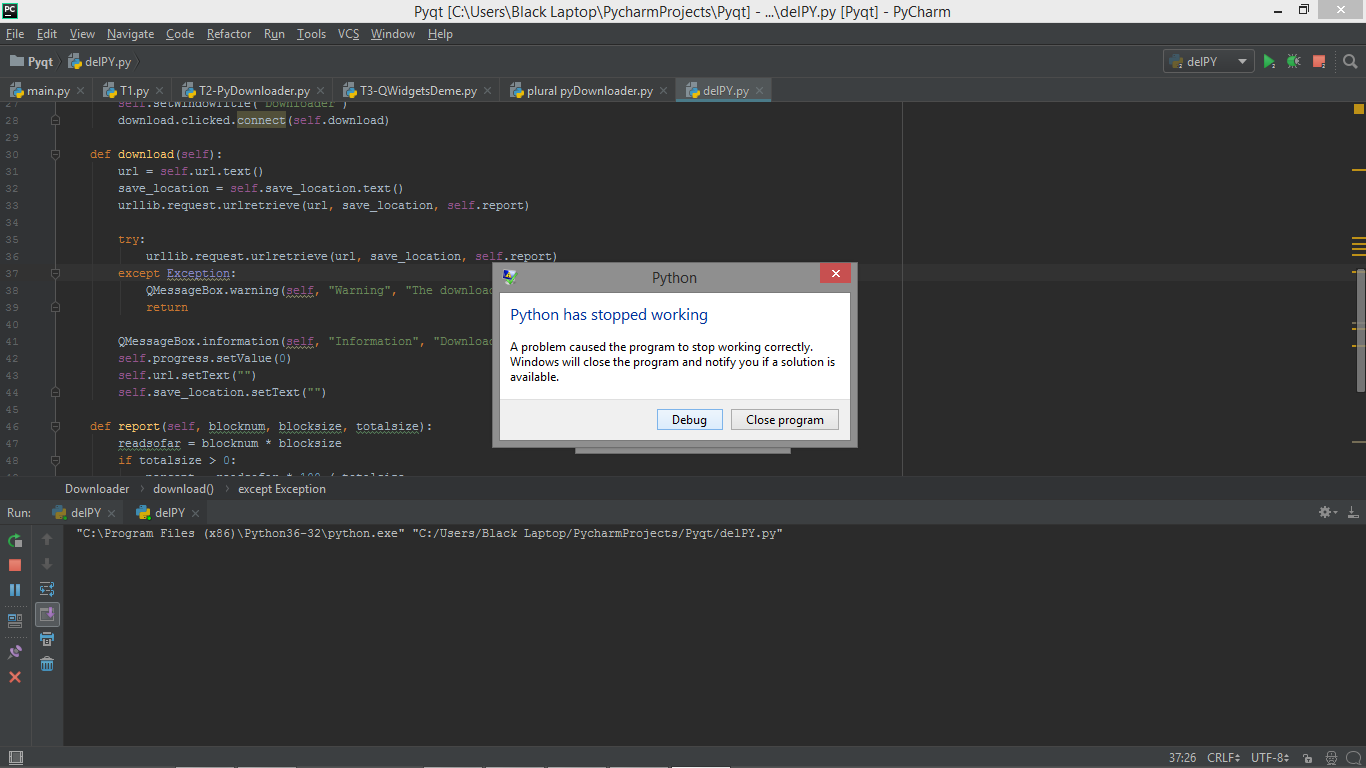