Created
September 12, 2019 21:26
-
-
Save deltastateonline/4194ab2a6f8ead7c6cfca36eb1a54077 to your computer and use it in GitHub Desktop.
Copying Binary File using BufferInputStream and Bufferoutputstream, ensuring that the correct number of bytes are written
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package com.deltastateonline.files; | |
import java.io.BufferedInputStream; | |
import java.io.BufferedOutputStream; | |
import java.io.File; | |
import java.io.FileInputStream; | |
import java.io.FileNotFoundException; | |
import java.io.FileOutputStream; | |
import java.io.IOException; | |
public class App { | |
public App() { | |
// TODO Auto-generated constructor stub | |
} | |
public static void main(String[] args) throws IOException { | |
// TODO Auto-generated method stub | |
String infile = "data\\in\\IMG_2004.JPG"; | |
File aFile = new File(infile); | |
//int BUFFER_SIZE = 8 * 1024; | |
int BUFFER_SIZE = 2 * 1024; | |
long totalLenght = aFile.length(); | |
String outfile = String.format("data\\out.1\\IMG_2004.%s.JPG",BUFFER_SIZE) ; | |
BufferedInputStream inputStream = null ; | |
BufferedOutputStream outStream = null ; | |
System.out.println("Buffer Size : "+BUFFER_SIZE); | |
byte[] buffer = new byte[BUFFER_SIZE]; | |
try { | |
inputStream = new BufferedInputStream(new FileInputStream(infile)); | |
outStream = new BufferedOutputStream(new FileOutputStream(outfile)); | |
int acc = 0; | |
while(inputStream.read(buffer) != -1){ | |
// | |
if((acc+BUFFER_SIZE) < totalLenght){ | |
outStream.write(buffer); | |
}else{ | |
long diff = totalLenght - acc; // howmany bytes are left to be written | |
outStream.write(buffer, 0, (int)diff); // only write the remaining bytes | |
} | |
acc = acc + BUFFER_SIZE; // increase the accumulator | |
} | |
outStream.flush(); | |
} catch (FileNotFoundException e) { | |
// TODO Auto-generated catch block | |
e.printStackTrace(); | |
} catch (IOException e) { | |
// TODO Auto-generated catch block | |
e.printStackTrace(); | |
} finally{ | |
if(inputStream != null){ | |
inputStream.close(); | |
} | |
if(outStream != null){ | |
outStream.close(); | |
} | |
} | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Using the naive solutions found on the internet, copying files result in f having more bytes than they should.
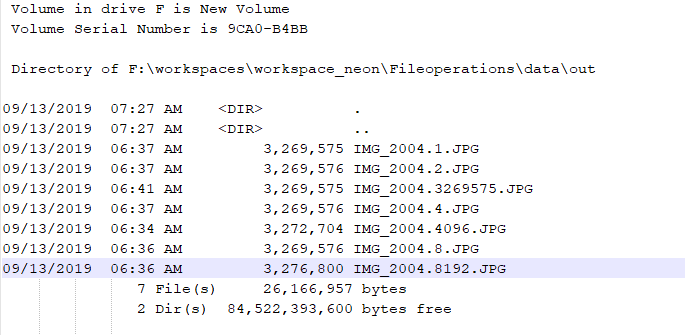
The output below shows a file been copied with different buffer sizes. The file names are IMG_2004.{BUFFER_SIZE}.jpg.
For some image files and text based files, this doesn't matter but it does matter for pdf files.