Created
November 25, 2023 01:45
-
-
Save denisenepraunig/fd8d21eaa1cd0a881ca64630bc8ad021 to your computer and use it in GitHub Desktop.
SwiftUI Metal Shader changing background color based on mouse position
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import SwiftUI | |
struct ContentView: View { | |
@State private var hoverLocation: CGPoint = .zero | |
@State private var isHovering = false | |
@State var toggleText = false | |
var body: some View { | |
ZStack { | |
Rectangle() | |
.foregroundStyle(.black) | |
.layerEffect(ShaderLibrary.circle( | |
.boundingRect, | |
.float2(hoverLocation) | |
), maxSampleOffset: .zero) | |
.blur(radius: 10.0) | |
} | |
.onContinuousHover { phase in | |
switch phase { | |
case .active(let location): | |
hoverLocation = location | |
isHovering = true | |
case .ended: | |
isHovering = false | |
} | |
} | |
.frame(minWidth: 400, idealWidth: 400, maxWidth: .infinity, minHeight: 400, idealHeight: 400, maxHeight: .infinity, alignment: .center) | |
} | |
} | |
#Preview { | |
ContentView() | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here is the is a very small video of it:
background-shader-moving-hover-super-small.mov
And here is a screenshot:
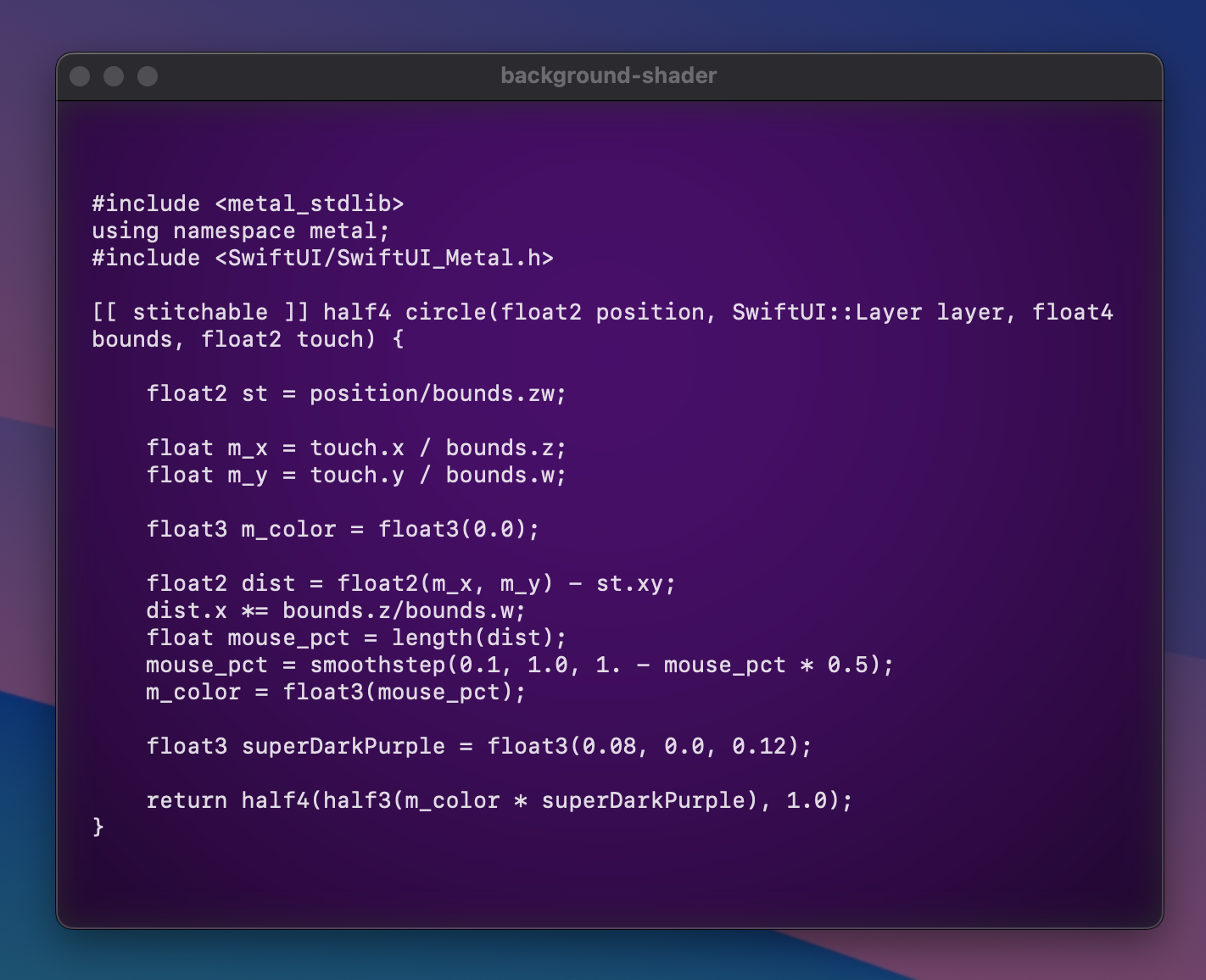