-
-
Save desinas/3f9aea1317eea217028f4575d042a62d to your computer and use it in GitHub Desktop.
const myCustomDiv = document.createElement('div'); | |
function respondToTheClick(evt) { | |
console.log('A paragraph was clicked: ' + evt.target.textContent); | |
} | |
for (let i = 1; i <= 200; i++) { | |
const newElement = document.createElement('p'); | |
newElement.textContent = 'This is paragraph number ' + i; | |
myCustomDiv.appendChild(newElement); | |
} | |
document.body.appendChild(myCustomDiv); | |
myCustomDiv.addEventListener('click', respondToTheClick); |
Now there is only: a single event listener, a single listener function.
Now the browser doesn't have to store in memory two hundred different event listeners and two hundred different listener functions. That's a great for performance`! However, if you test the code above, you'll notice that we've lost access to the individual paragraphs. There's no way for us to target a specific paragraph element.So how do we combine this efficient code with the access to the individual paragraph items that we did before? We use a process called event delegation.
The event object has a .target property. This property references the target of the event. Remember the capturing, at target, and bubbling phases?...these are coming back into play here, too!
Let's say that you click on a paragraph element. Here's roughly the process that happens:
- a paragraph element is clicked
- the event goes through the capturing phase
- it reaches the target
- it switches to the bubbling phase and starts going up the DOM tree
- when it hits the div element, it runs the listener function
- inside the listener function, event.target is the element that was clicked
So event.target gives us direct access to the paragraph element that was clicked. Because we have access to the element directly, we can access its .textContent, modify its styles, update the classes it has - we can do anything we want to it!
🌶️ One of the hot methodologies in the JavaScript world is event delegation, and for good reason. Event delegation allows you to avoid adding event listeners to specific nodes; instead, the event listener is added to one parent. That event listener analyzes bubbled events to find a match on child elements. The base concept is fairly simple but many people don't understand just how event delegation works. Let me explain the how event delegation works and provide pure JavaScript example of basic event delegation.
We're creating a div element, attaching two hundred paragraph elements and attaching an event listener with a respondToTheClick function to each paragraph as we create it. There are a number of ways we could refactor this code. For example, as of right now, we're creating two hundred different respondToTheClick functions (that all actually do the exact same thing!). We could extract this function and just reference the function instead of creating two hundred different functions.
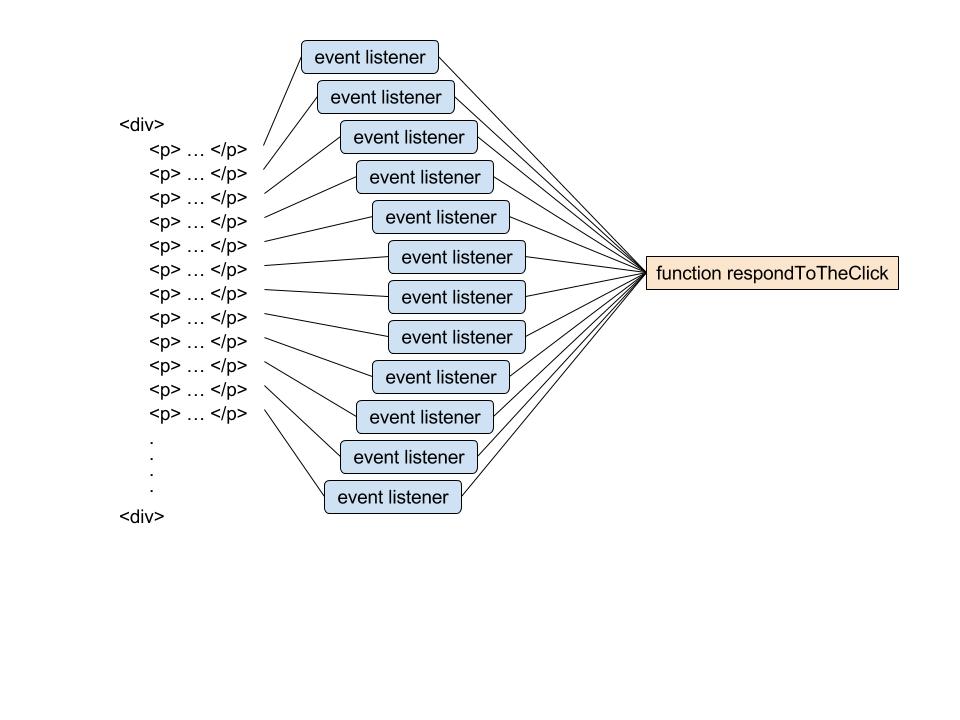