Last active
May 17, 2024 10:34
-
-
Save dgl/ef848e75c03c09b0db63a43173ee5662 to your computer and use it in GitHub Desktop.
A git pager that wraps less and links commits using OSC8
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env perl | |
# ©2023 David Leadbeater <https://dgl.cx/0bsd>; SPDX-License-Identifier: 0BSD | |
# | |
# A git pager that wraps less and links commits, plus makes hiding things via | |
# backspace not work. Your terminal and less will need support for "OSC8": | |
# https://github.com/Alhadis/OSC8-Adoption | |
=pod # Setup; run these commands: | |
wget https://gist.github.com/dgl/ef848e75c03c09b0db63a43173ee5662/raw/git-osc8-pager && | |
cp git-osc8-pager ~/bin && chmod +x ~/bin/git-osc8-pager && | |
git config --global core.pager git-osc8-pager | |
=cut | |
use strict; | |
my $remote = (qx(git status -sb) =~ /\.\.\.([a-zA-Z0-9-]+)/)[0] || "origin"; | |
my $remote_uri = qx{git remote get-url "$remote"}; | |
chomp $remote_uri; | |
# Logic carefully arranged to work for github, sourcehut (sr.ht) and gitlab. | |
$remote_uri =~ s/^git\@//; | |
$remote_uri =~ s/\.git$//; | |
$remote_uri =~ s!^([.\w]+):!https://$1/! unless $remote_uri =~ /^http/; | |
open my $less_fh, "|-", "less" or die $!; | |
while(<STDIN>) { | |
# linkify commits | |
s/^((?:\e\[\d+m)?commit )([a-f0-9]{40,})(.*)/$1 . url("commit", $2) . $3/e; | |
# Issues/PRs (github style, same repo only) | |
s/(^|[ ([])(\#)(\d+)([)., ]|$)/$1 . url("issues", $3, $2) . $4/eg; | |
# For github itself, do cross-repo links | |
if ($remote_uri =~ m{^https://github.com/}) { | |
# https://docs.github.com/en/get-started/writing-on-github/working-with-advanced-formatting/autolinked-references-and-urls | |
s/(^|[ ([])([A-Za-z0-9-]+\/[a-zA-Z0-9-._]+)(\#)(\d+)([)., ]|$)/$1 . repo_url($2, "issues", $4, $3) . $5/eg; | |
s/(^|[ ([])(GH-)(\d+)([)., ]|$)/$1 . url("issues", $3, $2) . $4/eg; | |
} | |
# show backspaces | |
s/\x08/\e[7m^H\e[27m/g; | |
print $less_fh $_ or exit 1; | |
} | |
sub url { | |
my($type, $id, $extra) = @_; | |
return "\e]8;;" . $remote_uri . "/$type/$id\a" . $extra . $id . "\e]8;;\a"; | |
} | |
sub repo_url { | |
my($repo, $type, $id, $extra) = @_; | |
return "\e]8;;https://github.com/" . $repo . "/$type/$id\a" . $repo . $extra . $id . "\e]8;;\a"; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Action shot:
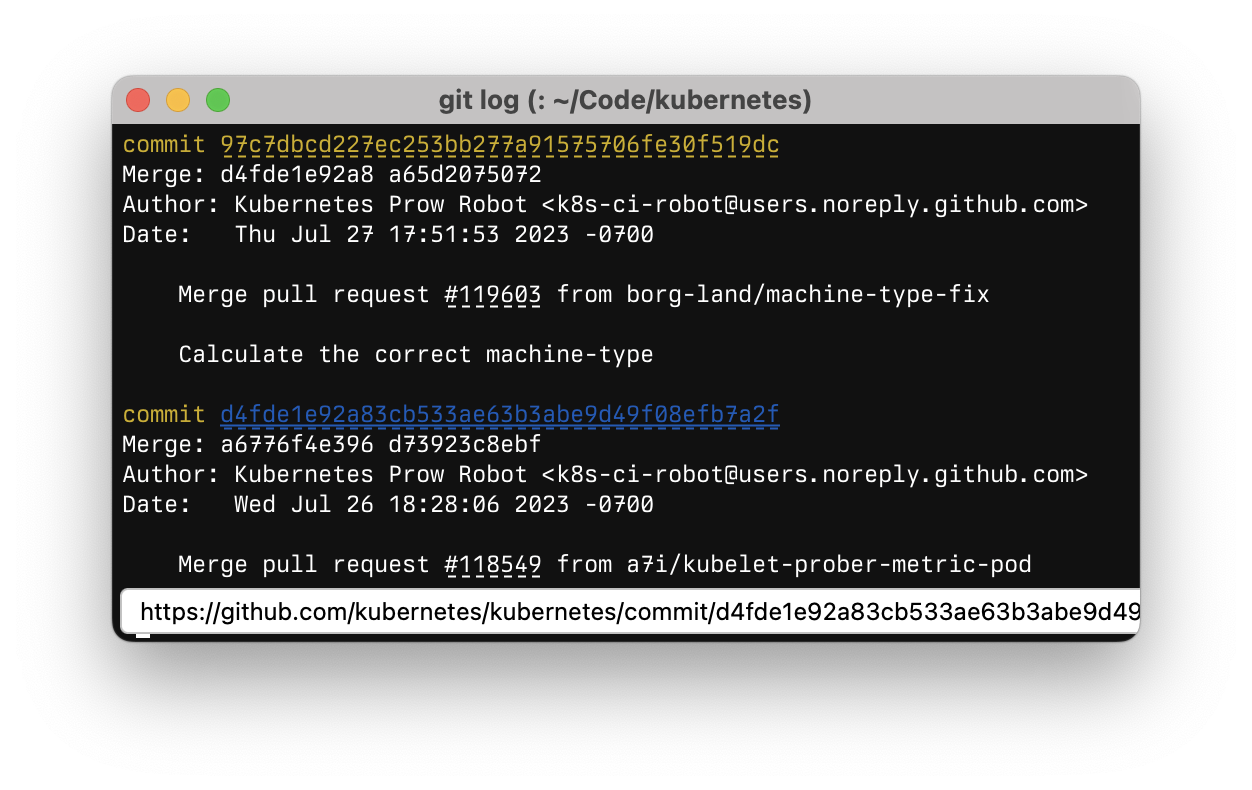