-
-
Save dipakkr/c8dc2561b8078487a6fdfd0fd9f0cc95 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
a = 10; | |
function add(x, y){ | |
const result = x + y; | |
return result; | |
} | |
const name = 'github'; | |
const final = add(10, 20); | |
``` | |
As soon as you run this program, it creates a `Global Execution Content`, the place where the javascript thread will run line by line and run your code. | |
> You can think of `Global execution context` as an environment where the code runs. | |
> There can be multiple `execution context` in a program but a single `Global execution context`. | |
Now let's go back to the above code snippet and see what the JS engine does when we run the code. | |
- JS Engine parses your code line by line & identifies variables & functions created by code (which will be used in the execution phase) | |
- JS Engine setup memory space for variables & functions ( called as **Hoisting**) | |
**Hoisting ** is basically the JS Engine set-asides memory space for variables and functions used inside the code before your code is executed. These variables & functions comprise the Execution Context of any function that is being executed. | |
- A new Execution Context is created whenever function invocation is called. | |
> All variables in JS are initially set to `undefined`. | |
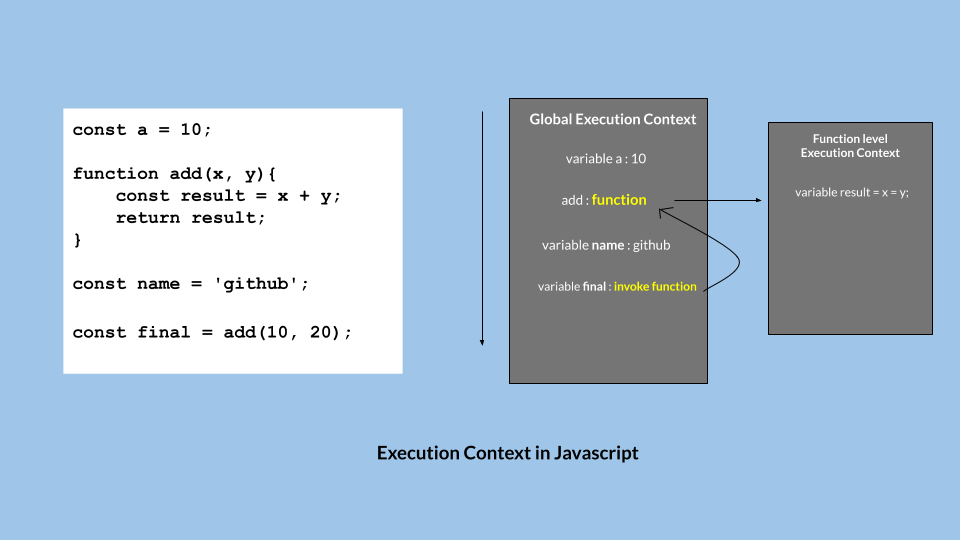 | |
Let's take one more example. | |
``` | |
function y(){ | |
const p = 100; | |
} | |
function x(){ | |
y(); | |
const t = 10; | |
} | |
x(); | |
const r = 20; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment