Created
October 4, 2021 14:11
-
-
Save dmattia/7ac415f36ead6599aa7b39096f4037f8 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// external | |
import * as aws from '@pulumi/aws'; | |
import * as pulumi from '@pulumi/pulumi'; | |
const awsAccountId = aws.getCallerIdentity().then(({ accountId }) => accountId); | |
const accountArn = pulumi.interpolate`arn:aws:iam::${awsAccountId}:root`; | |
const kmsPolicy = accountArn.apply( | |
(arn) => | |
({ | |
Version: '2012-10-17', | |
Id: 'airgap_telemetry_policy', | |
Statement: [ | |
// This is the default KMS policy | |
{ | |
Sid: 'Enable IAM User Permissions', | |
Effect: 'Allow', | |
Principal: { | |
AWS: arn, | |
}, | |
Action: 'kms:*', | |
Resource: '*', | |
}, | |
// Give SNS permissions to use this key | |
{ | |
Sid: 'Enable SNS Permissions', | |
Effect: 'Allow', | |
Principal: { Service: ['sns.amazonaws.com'] }, | |
Action: ['kms:Decrypt', 'kms:GenerateDataKey*'], | |
Resource: '*', | |
}, | |
], | |
} as aws.iam.PolicyDocument), | |
); | |
const kmsKey = new aws.kms.Key(`key`, { | |
policy: kmsPolicy.apply((policy) => JSON.stringify(policy)), | |
}); | |
const snsTopic = new aws.sns.Topic('receiver', { | |
name: 'race-condition', | |
kmsMasterKeyId: kmsKey.id, | |
}); | |
const bucket = new aws.s3.Bucket('bucket', { | |
bucketPrefix: 'race-condition', | |
}); | |
const policy = new aws.iam.Policy(`policy`, { | |
name: 'raceConditionPolicy', | |
path: '/', | |
policy: { | |
Version: '2012-10-17', | |
Statement: [ | |
{ | |
Effect: 'Allow', | |
Action: [ | |
's3:AbortMultipartUpload', | |
's3:GetBucketLocation', | |
's3:GetObject', | |
's3:ListBucket', | |
's3:ListBucketMultipartUploads', | |
's3:PutObject', | |
], | |
Resource: [bucket.arn, pulumi.interpolate`${bucket.arn}/*`], | |
}, | |
], | |
}, | |
}); | |
const role = new aws.iam.Role(`role`, { | |
name: 'raceConditionRole', | |
managedPolicyArns: [policy.arn], | |
assumeRolePolicy: { | |
Version: '2012-10-17', | |
Statement: [ | |
{ | |
Effect: 'Allow', | |
Action: 'sts:AssumeRole', | |
Principal: { | |
Service: 'firehose.amazonaws.com', | |
}, | |
}, | |
], | |
}, | |
}); | |
const firehoseStream = new aws.kinesis.FirehoseDeliveryStream( | |
'raw_data_stream', | |
{ | |
name: 'raceConditionStream', | |
destination: 's3', | |
s3Configuration: { | |
roleArn: role.arn, | |
bucketArn: bucket.arn, | |
}, | |
}, | |
); | |
const snsPolicy = new aws.iam.Policy(`sns_policy`, { | |
name: 'raceConditionPolicySns', | |
path: '/', | |
policy: { | |
Version: '2012-10-17', | |
Statement: [ | |
{ | |
Effect: 'Allow', | |
Action: [ | |
'firehose:DescribeDeliveryStream', | |
'firehose:ListDeliveryStreams', | |
'firehose:ListTagsForDeliveryStream', | |
'firehose:PutRecord', | |
'firehose:PutRecordBatch', | |
], | |
Resource: [firehoseStream.arn], | |
}, | |
], | |
}, | |
}); | |
const snsRole = new aws.iam.Role(`sns_role`, { | |
name: 'raceConditionRoleSns', | |
managedPolicyArns: [snsPolicy.arn], | |
assumeRolePolicy: { | |
Version: '2012-10-17', | |
Statement: [ | |
{ | |
Effect: 'Allow', | |
Action: 'sts:AssumeRole', | |
Principal: { | |
Service: 'sns.amazonaws.com', | |
}, | |
}, | |
], | |
}, | |
}); | |
new aws.sns.TopicSubscription('firehose_sub', { | |
topic: snsTopic.arn, | |
protocol: 'firehose', | |
endpoint: firehoseStream.arn, | |
subscriptionRoleArn: snsRole.arn, | |
}); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Race condition in pulumi/AWS (probably AWS):
Fails with:

Immediately after that failure, it succeeds with:
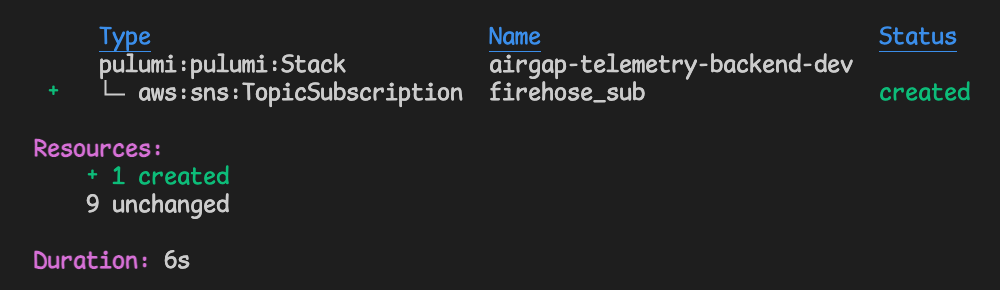