Last active
July 10, 2020 18:14
-
-
Save dosstx/753b74ea7991fdec721fd2de40183cb6 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<section> | |
<img | |
:id="photo.filename" | |
:src="photo.url" | |
/> | |
</section> | |
</template> | |
<script> | |
export default { | |
name: 'PhotoDetail', | |
data() { | |
return { | |
photo: {}, | |
anno: {}, | |
annotations: [] | |
} | |
}, | |
async mounted() { | |
await this.getPhotoDoc() | |
this.anno = this.$initAnnotorious(this.photo.filename) | |
await this.addAnnotations() <--doing it this way does not work | |
// await this.loadAnnotations() <-- this works | |
// listen to annotation events | |
this.onUpdateAnnotate() <--- when updating annotation, I get "update anno doc undefined" in console (see screenshot) | |
}, | |
methods: { | |
findById(id) { | |
const query = this.$fireStore | |
.collection('annotations') | |
.where('id', '==', id) | |
return query.get().then(function (querySnapshot) { | |
console.log('querySnapshot: ', querySnapshot) | |
return querySnapshot.docs[0] | |
}) | |
}, | |
async addAnnotations() { | |
try { | |
const annoSnapshot = await this.$bind( | |
'annotations', | |
this.$fireStore | |
.collection('annotations') | |
.where('target.source', '==', this.photo.url) | |
) | |
annoSnapshot.forEach((doc) => { | |
this.anno.addAnnotation(doc, false) | |
}) | |
} catch (error) { | |
console.log('annotations error: ', error) | |
} | |
}, | |
onUpdateAnnotate() { | |
try { | |
this.anno.on('updateAnnotation', (annotation, previous) => { | |
this.findById(previous.id).then((doc) => { | |
console.log('update anno doc', doc) // <--console says doc is 'undefined' | |
doc.ref.update(annotation) // thus, this doesn't update | |
}) | |
}) | |
} catch (error) { | |
console.log('error updating anno', error) | |
} | |
}, | |
// async loadAnnotations() { <-----------------if I use this method, i have no issues editing docs | |
// try { | |
// const querySnapshot = await this.$fireStore | |
// .collection('annotations') | |
// .where('target.source', '==', this.photo.url) | |
// .get() | |
// const annotations = await querySnapshot.docs.map((doc) => doc.data()) | |
// if (!annotations.length) { | |
// console.log('no anntotations available! Add some!') | |
// } else { | |
// this.anno.setAnnotations(annotations) | |
// } | |
// } catch (error) { | |
// console.log(error) | |
// } | |
// }, | |
async getPhotoDoc() { | |
try { | |
await this.$bind( | |
'photo', | |
this.$fireStore.collection('photos').doc(this.$route.params.id) | |
) | |
} catch (error) { | |
console.log('Error getting document:', error) | |
} | |
} | |
} | |
} | |
</script> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here is screenshot when the above code is ran:
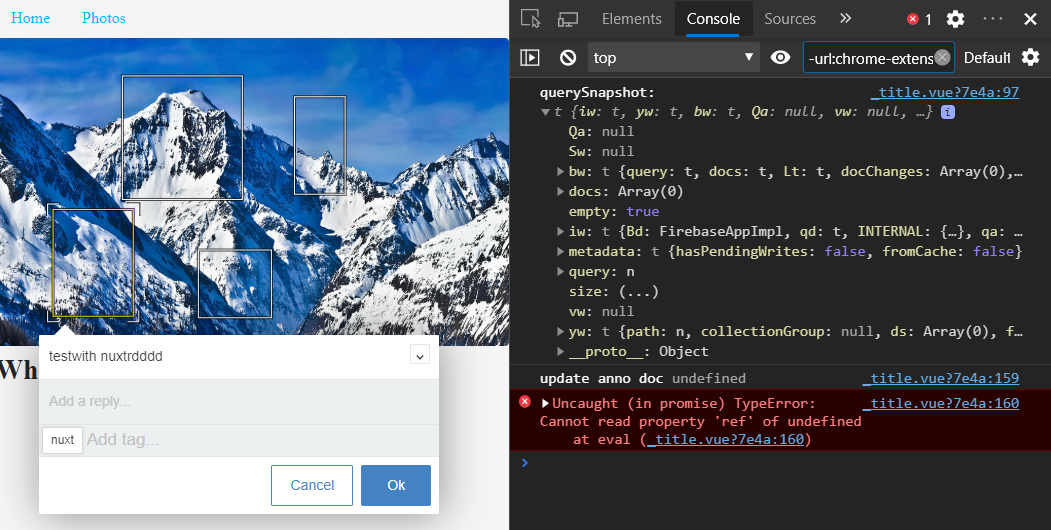