Created
January 11, 2023 21:02
-
-
Save dotcomboom/e831ff7322ddb498064f0d20330cad8c to your computer and use it in GitHub Desktop.
A Teverse calculator app.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
--[[ | |
Really cool calculator app for Teverse in 100 lines or less by dotcomboom/dcb, donut steel | |
]] -- UI objects | |
local frame = teverse.construct("guiFrame", | |
{ -- Parent frame, contains all objects | |
parent = teverse.interface, | |
size = guiCoord(1, 0, 1, 0) | |
}) | |
local topbar = teverse.construct("guiTextBox", | |
{ -- Definitely not ripped from the example | |
parent = frame, | |
size = guiCoord(1, 0, 0, 60), | |
position = guiCoord(0, 0, 0, 0), | |
backgroundColour = colour.rgb(52, 58, 64), | |
text = "teverse calculator", | |
textSize = 36, | |
textFont = "tevurl:fonts/robotoBold.ttf", | |
textColour = colour(1, 1, 1), | |
textAlign = "middle", | |
textShadowSize = 4, | |
textShadowColour = colour(0, 0, 0), | |
dropShadowAlpha = 1, | |
dropShadowBlur = 2, | |
dropShadowColour = colour.rgb(127, 127, 127), | |
dropShadowOffset = vector2(0.5, 1) | |
}) | |
local number1box = teverse.construct("guiTextBox", { | |
parent = frame, | |
size = guiCoord(0.3, 0, 0.0, 40), | |
position = guiCoord(0, 16, 0, 76), | |
text = "0", | |
textSize = 36, | |
textFont = "tevurl:fonts/firaCodeRegular.otf", | |
textEditable = true, | |
dropShadowAlpha = 1, | |
dropShadowBlur = 2, | |
dropShadowColour = colour.rgb(127, 127, 127), | |
dropShadowOffset = vector2(0.5, 1) | |
}) | |
local number2box = teverse.construct("guiTextBox", { | |
parent = frame, | |
size = guiCoord(0.3, 0, 0.0, 40), | |
position = guiCoord(0, 16, 0, 122), | |
text = "0", | |
textSize = 36, | |
textFont = "tevurl:fonts/firaCodeRegular.otf", | |
textEditable = true, | |
dropShadowAlpha = 1, | |
dropShadowBlur = 2, | |
dropShadowColour = colour.rgb(127, 127, 127), | |
dropShadowOffset = vector2(0.5, 1) | |
}) | |
local results = teverse.construct("guiTextBox", { | |
parent = frame, | |
size = guiCoord(0.7, -48, 1, -130), | |
position = guiCoord(0.3, 32, 0, 76), | |
textSize = 36, | |
textFont = "tevurl:fonts/firaCodeRegular.otf", | |
textMultiline = true, | |
textAlign = enums.align.topRight, | |
textWrap = true, | |
dropShadowAlpha = 1, | |
dropShadowBlur = 2, | |
dropShadowColour = colour.rgb(127, 127, 127), | |
dropShadowOffset = vector2(0.5, 1), | |
active = false | |
}) | |
-- Functionality | |
function scale() -- Some delicious, homegrown text-scaling | |
while (results.textDimensions.y < results.absoluteSize.y and | |
results.textSize < 36) do | |
results.textSize = results.textSize + 1 -- Increase font size until text height is larger than the textbox's height | |
end | |
while results.textDimensions.y > results.absoluteSize.y do | |
results.textSize = results.textSize - 1 -- Decrease font size until text height fits in the textbox | |
end | |
end | |
function calc() -- Do the calculations | |
local x = tonumber(number1box.text) | |
local y = tonumber(number2box.text) | |
-- (Note: these will crash the function if the text is alphanumeric) | |
-- (Fortunately, that doesn't crash the app or anything soo) | |
local add = string.format("%s + %s = %s\n", x, y, x + y) | |
local subtract = string.format("%s - %s = %s\n", x, y, x - y) | |
local multiply = string.format("%s * %s = %s\n", x, y, x * y) | |
local divide = string.format("%s / %s = %s\n", x, y, x / y) | |
local modulo = string.format("%s %% %s = %s\n", x, y, x % y) | |
local exponent = string.format("%s ^ %s = %s\n", x, y, x ^ y) | |
-- The above perform the calculations and make them into nicely formatted strings | |
results.text = add .. subtract .. multiply .. divide .. "\n" .. modulo .. | |
exponent | |
-- This just adds them all together; modulo and exponent aren't the basic operators so another newline's added for cosmetic effect | |
scale() | |
-- Run that scaling function so the text all fits | |
end | |
number1box:on("keyUp", calc) -- Calculate function is ran when a key is no longer being pressed | |
number2box:on("keyUp", calc) | |
teverse.input:on("screenResized", scale) -- Scale text to fit when the screen is resized | |
calc() -- Run the first calculation with 0 and 0 |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Screenshot of it in use:
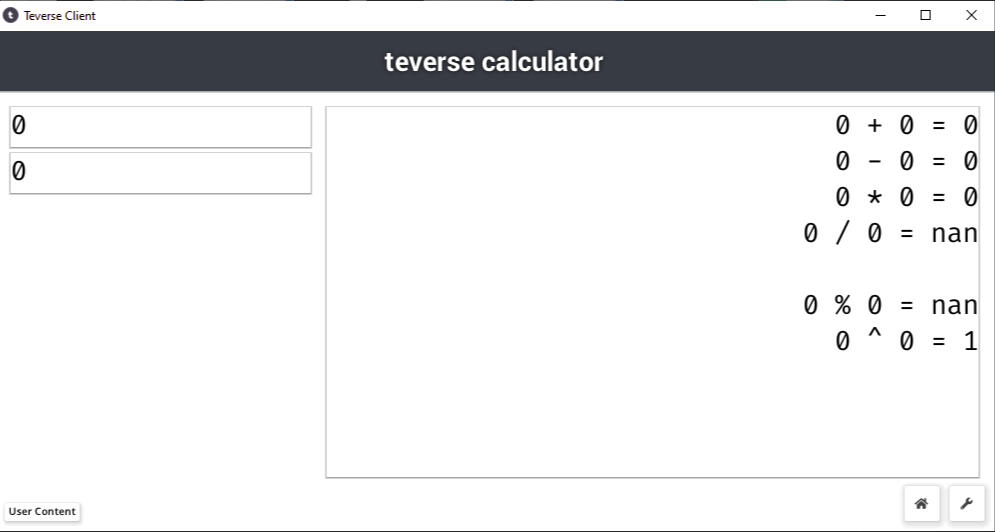
(this was taken in 2020, I don't know when/if Teverse is going online again)