Use a linter for learning bash, here is one highly recommended that is available as a plugin/extension is Sublime-Text and VS Code: Shellcheck
In the meantime, work on some basics through example.
# Ask how to use the command `ls`
man ls
In addition, most commands will have an argument -h
or --help
for a full description.
ls --help
echo Hello world
# Exclude tailing newline
echo -n Hello world
# Interpret escape chars
echo -e "Hello\nworld"
# Print an environment variable
echo "Hello $USER"
# Display PID
echo $$
# Print return code (retval) of previous command
echo $?
# Print environment variables - try this on your own time
# env
# Set environment variable
export TEST=123
echo "My test number is $TEST"
# Unset environment variable
unset TEST
echo "Did we unset the value: TEST='$TEST'?"
nano
and vim
less
and more
# Print directory
ls
# Print home directory
ls ~
# Print directory and hidden files
ls -a
# Print directories with glob pattern
ls -a ~/.*
# Print all home directory folders starting with D or A using glob pattern
echo ~/{D,A}*
# Change directory to home
cd ~
# Change directory to home
cd /Users/eddie
# Change directory to previous
cd -
# Print expanded home directory
echo ~
# Make a file
touch my-file.txt
# Remove a file
rm my-file.txt
# Make a directory
mkdir my-dir
# Remove a directory
rm -fr my-dir
# Make directory path
mkdir -p my-dir-parent/my-dir-child
# Remove a directory
rm -fr my-dir-parent
# Make a directory
mkdir my-dir
# Move directory to /tmp
mv my-dir /tmp
cp /tmp/my-dir .
# Print the contents of a file
cat /etc/hosts
# Find a file of folder starting from home directory with name .ssh
find ~ -name .ssh
# Print the size of the application directory from top level
du --human-readable --max-depth=1 /Applications/
Homework, learn about: chmod
and chown
.
- What was the command again? Oh yea, lets use reverse-search!
- I didn't quiet find my command... Lets check
history
. - What environment variables do I have available? I know, Ill check
env
- cannot show you during demo, might be secrets in there
# Request a weather update
curl https://wttr.in
# Display file contents of an http file
curl https://www.google.ca/robots.txt
# Get the http status code
curl --request GET --write-out "%{http_code}" --silent --output /dev/null https://www.google.ca/
# Check if a https port is open
nc --zero --verbose google.ca 443
# Print all lines containing `127.0.0.1` in `/etc/hosts`
grep 127.0.0.1 /etc/hosts
# Print all lines with IPv4 regex pattern
grep -E '\d+\.\d+\.\d+\.\d+' /etc/hosts
# Print all lines containing `localhost` glob pattern
cat /etc/hosts | grep 127.0.0.*
# Append a line to a file owned by root
echo '0.0.0.0 my.dns-override.com' | sudo tee -a /etc/hosts
# Split user part from a url
echo 'edward.corrigall@clearbanc.com' | cut -d@ -f1
# Replace a dot character
echo 'edward.corrigall@clearbanc.com' | cut -d@ -f1 | tr '.' ' '
# Create a file with a multiline string
tee -a hostnames.txt <<EOF
google.com
google.ca
google.ca
yahoo.ca
reddit.com
facebook.com
www.google.com
google.ca
EOF
# Count number of entries
cat hostnames.txt | wc -l
# Sort a file
cat hostnames.txt | sort
# Sort and deduplicate file
cat hostnames.txt | sort -u
# Tally up by unique values, in descending order
cat hostnames.txt | sort | uniq -c | sort -r
# Create a file with a multiline string
tee -a users.csv <<EOF
email,first_name
bob@microsoft.com,Bob
alice@microsoft.com,Alice
EOF
# Display all but the first line of file
cat users.csv | tail -n +2
# Display second column in comma-separated values file
cat users.csv | tail -n +2 | cut -f2 -d,
Write a bash script to parse a CSV file!
Note: This is for demonstration purposes only. I recommend using CSV Kit.
# Create a little script...
echo 'Parsing a csv file, yay!'
# Print csv header
cat users.csv | head -n 1 | tr ',' ' ' | tr '[a-z]' '[A-Z]'
# Loop through records in a comma-separated values file
for row in $(cat users.csv | tail -n +2); do
email="$(echo "$row" | cut -f1 -d,)"
first_name="$(echo "$row" | cut -f2 -d,)"
echo "Processing record for $first_name"
done
JQ is a json parser which is exceptionally handy as well.
Example output:
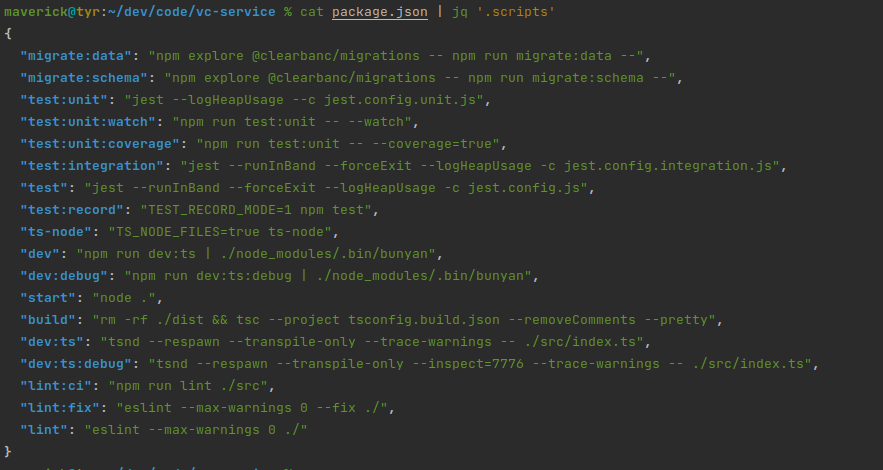