Created
January 21, 2021 09:51
-
-
Save edewit/df87925ebb2c084f0d15052eb7e93ff2 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const KcAdminClient = require('keycloak-admin').default; | |
const kcAdminClient = new KcAdminClient({ | |
baseUrl: 'http://localhost:8180/auth', | |
realmName: 'master', | |
}); | |
(async () => { | |
await kcAdminClient.auth({ | |
username: 'admin', | |
password: 'admin', | |
grantType: 'password', | |
clientId: 'admin-cli' | |
}); | |
// List all flows | |
const flows = await kcAdminClient.authenticationManagement.getFlows(); | |
console.log(flows); | |
/* | |
{ | |
id: 'a3e0e82f-8d48-421b-a246-8ca795dc5cb5', | |
alias: 'Copy of browser', | |
description: 'bla', | |
providerId: 'basic-flow', | |
topLevel: true, | |
builtIn: false, | |
authenticationExecutions: [ [Object], [Object], [Object], [Object] ] | |
}, | |
{ | |
id: 'b9c1e3cf-968c-4f42-8120-759edfbcbda3', | |
alias: 'browser', | |
description: 'browser based authentication', | |
providerId: 'basic-flow', | |
topLevel: true, | |
builtIn: true, | |
authenticationExecutions: [ [Object], [Object], [Object], [Object] ] | |
}, | |
... | |
*/ | |
// copy flow based on existing | |
await kcAdminClient.authenticationManagement.copyFlow({flow: "browser", newName: "Copy of browser"}); | |
// get all executions | |
const executions = await kcAdminClient.authenticationManagement.getExecutions({flow: "browser"}); | |
console.log(executions); | |
/* | |
[ | |
{ | |
id: '5f1ccb9f-832c-4e2b-96e2-ee6309315a46', | |
requirement: 'ALTERNATIVE', | |
displayName: 'Cookie', | |
requirementChoices: [ 'REQUIRED', 'ALTERNATIVE', 'DISABLED' ], | |
configurable: false, | |
providerId: 'auth-cookie', | |
level: 0, | |
index: 0 | |
}, | |
{ | |
id: 'e3bc8a37-12e6-40c7-a15d-7291a5cf0b1e', | |
requirement: 'DISABLED', | |
displayName: 'Kerberos', | |
requirementChoices: [ 'REQUIRED', 'ALTERNATIVE', 'DISABLED' ], | |
configurable: false, | |
providerId: 'auth-spnego', | |
level: 0, | |
index: 1 | |
}, | |
... | |
*/ | |
const addedExecution = await kcAdminClient.authenticationManagement.addExecutionToFlow({flow: "Copy of browser", provider: "direct-grant-validate-otp"}); | |
addedExecution.requirement = "REQUIRED"; | |
await kcAdminClient.authenticationManagement.updateExecution({flow: "Copy of browser"}, addedExecution); | |
await kcAdminClient.authenticationManagement.raisePriorityExecution({id: addedExecution.id}); | |
})(); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
in the admin console it will look like this:
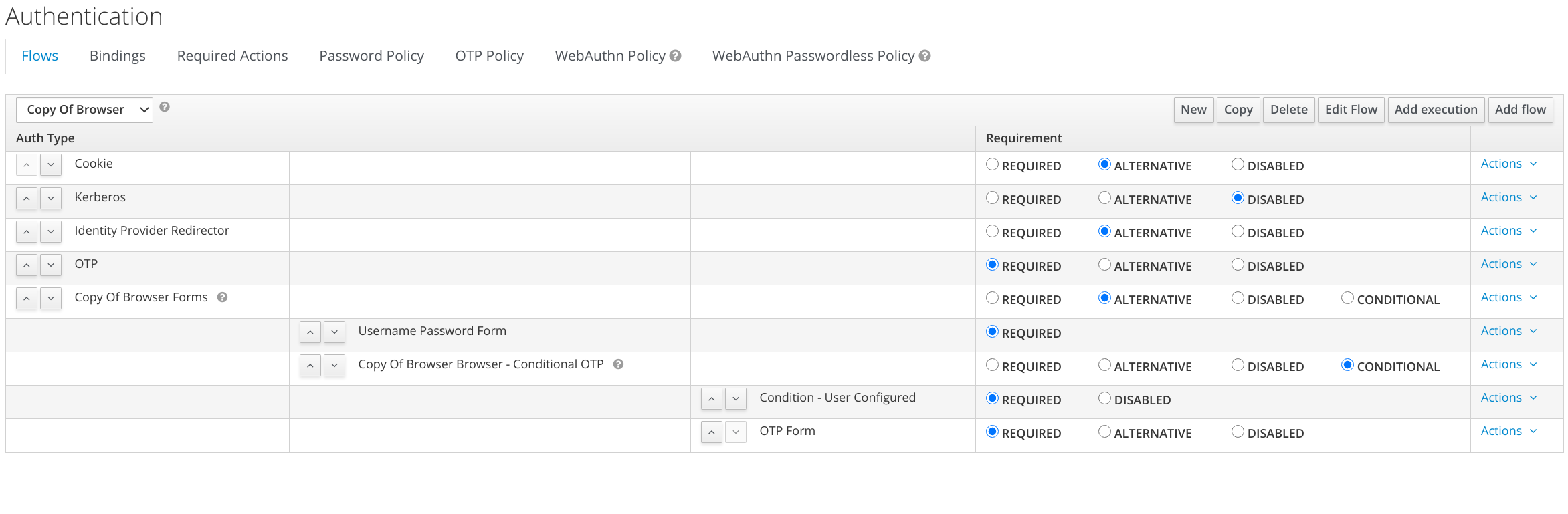