Last active
March 28, 2022 14:38
-
-
Save edmundcwm/2b9487e61a734d00e63daa894ec9be29 to your computer and use it in GitHub Desktop.
Add a checkbox to toggle the "Unfiltered html" capability for Administrators in a multisite.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
/** | |
* Add a checkbox to toggle the "Unfiltered html" capability for Administrators in a multisite. | |
*/ | |
/** | |
* Re-enable unfiltered_html for Administrators only on a Multisite. | |
* | |
* @see https://core.trac.wordpress.org/browser/tags/5.8/src/wp-includes/capabilities.php#L421 | |
*/ | |
add_filter( | |
'map_meta_cap', | |
function( $caps, $cap, $user_id ) { | |
$user = get_userdata( $user_id ); | |
if ( empty( $user->roles ) ) { | |
return $caps; | |
} | |
$can_insert_iframe = get_user_meta( $user_id, 'admin_insert_iframe', true ); | |
if ( 'unfiltered_html' === $cap && in_array( 'administrator', $user->roles, true ) && $can_insert_iframe ) { | |
$caps = array( 'unfiltered_html' ); | |
} | |
return $caps; | |
}, | |
10, | |
3 | |
); | |
/** | |
* Insert a new checkbox in the User Profile page. | |
*/ | |
add_action( | |
'personal_options', | |
function( $profileuser ) { | |
// This option is only available if current logged-in user is a Super Admin | |
// and user being edited is an Administrator. | |
if ( is_super_admin() && in_array( 'administrator', $profileuser->roles, true ) ) { | |
$can_insert_iframe = get_user_meta( $profileuser->ID, 'admin_insert_iframe', true ); | |
?> | |
<tr class="show-admin-bar user-admin-bar-front-wrap"> | |
<th scope="row"><?php esc_html_e( 'Add unfiltered HTML', 'edcwm' ); ?></th> | |
<td> | |
<label for="admin_insert_iframe"> | |
<input name="admin_insert_iframe" type="checkbox" id="admin_insert_iframe" value="1"<?php checked( $can_insert_iframe ); ?> /> | |
<?php esc_html_e( 'Allow user to add restricted tags like iframe and embed into a page/post.', 'edcwm' ); ?> | |
</label><br /> | |
</td> | |
</tr> | |
<?php | |
} | |
} | |
); | |
/** | |
* Save custom data to user meta. | |
* | |
* @param int $user_id User ID. | |
*/ | |
function edcwm_save_custom_user_meta( $user_id ) { | |
if ( ! wp_verify_nonce( $_REQUEST['_wpnonce'], 'update-user_' . $user_id ) ) { | |
// Security check fail. Exit early. | |
return; | |
} | |
$can_insert_iframe = filter_var( isset( $_POST['admin_insert_iframe'] ) ? $_POST['admin_insert_iframe'] : 0, FILTER_SANITIZE_NUMBER_INT ); | |
update_user_meta( $user_id, 'admin_insert_iframe', $can_insert_iframe ); | |
} | |
add_action( 'personal_options_update', 'edcwm_save_custom_user_meta' ); | |
add_action( 'edit_user_profile_update', 'edcwm_save_custom_user_meta' ); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
User profile page
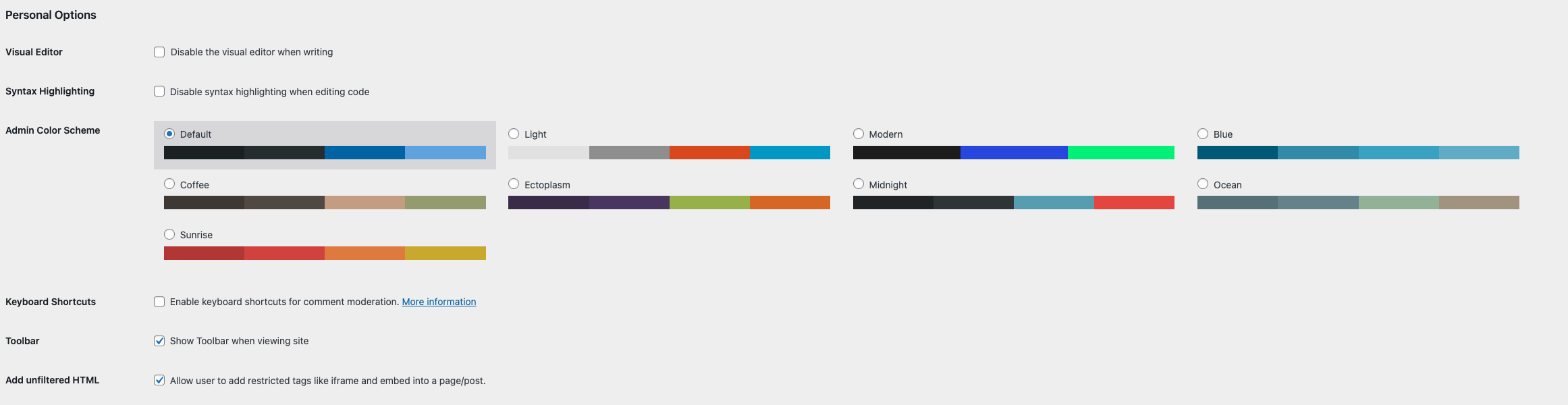