Last active
December 13, 2017 10:02
-
-
Save eliassoares/4717b44bd9b7eb16d9cc7ebfd25ceda4 to your computer and use it in GitHub Desktop.
bitbot.py
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/python | |
# -*- coding: utf-8 -*- | |
#Version: 2.0 | |
import urllib | |
import json | |
#Coloque as informações sobre suas comprar aqui: | |
compras = [ | |
{'moeda': 'BTC', 'moeda_cod': 'BTC', 'exchange': 'foxbit', 'quantidade': 0.666666, 'valor_compra': 66666.00}, | |
{'moeda': 'IOTA', 'moeda_cod': 'tIOTUSD', 'exchange': 'bitfinex', 'quantidade': 666, 'valor_compra': 6.66, 'dolar_dia_compra': 6.66}, | |
] | |
# Coloque aqui as moedas que irão aparecer regularmente no bitbar: | |
moedas = { | |
'BTC': {'valor': 0, '%': 0, 'mensagem': '₿ R$'}, | |
'IOTA': {'valor': 0, '%': 0, 'mensagem': 'IOTA R$'} | |
} | |
apis = { | |
'exchanges' : { | |
'bitfinex': 'https://api.bitfinex.com/v2/ticker/{}', # https://docs.bitfinex.com/v2/reference | |
'foxbit': 'https://api.bitvalor.com/v1/ticker.json', # https://bitvalor.com/api | |
}, | |
'dolar': 'http://api.promasters.net.br/cotacao/v1/valores', # http://api.promasters.net.br/cotacao/#documentacao | |
} | |
exchanges_exterior = { 'bitfinex' } | |
def apiGet(url): | |
res = urllib.urlopen(url).read() | |
try: | |
return json.loads(res.decode()) | |
except: | |
return 0 | |
def getDolar(): | |
try: | |
res = apiGet(apis['dolar']) | |
return res['valores']['USD']['valor'] | |
except: | |
return 0 | |
def getValor(exchange, moeda): | |
valor = 0 | |
if exchange == 'bitfinex': | |
res = apiGet(apis['exchanges']['bitfinex'].format(moeda)) | |
if res: | |
valor = res[6] | |
elif exchange == 'foxbit': | |
res = apiGet(apis['exchanges']['foxbit']) | |
if res: | |
valor = res['ticker_1h']['exchanges']['FOX']['last'] | |
return valor | |
def getRendimentos(): | |
rendimentos = [] | |
for c in compras: | |
valor_atual = getValor(c['exchange'], c['moeda_cod']) | |
valor_compra = c['valor_compra'] | |
if c['exchange'] in exchanges_exterior: | |
dolar = getDolar() | |
# A API do dolar está caindo direto: | |
if not dolar: | |
dolar = c['dolar_dia_compra'] | |
valor_atual *= dolar | |
valor_compra *= c['dolar_dia_compra'] | |
investido = c['quantidade'] * valor_compra | |
if c['exchange'] == 'foxbit': | |
moedas['BTC']['valor'] = valor_atual | |
moedas['BTC']['%'] = ((valor_atual / valor_compra) - 1) * 100 | |
if c['moeda'] == 'IOTA': | |
moedas['IOTA']['valor'] = valor_atual | |
moedas['IOTA']['%'] = ((valor_atual / valor_compra) - 1) * 100 | |
rendimentos.append({ | |
'Moeda': c['moeda'], | |
'R$ Atual': c['quantidade'] * valor_atual, | |
'R$ Investido': investido, | |
'Rendimento %': ((valor_atual / valor_compra) - 1) * 100 | |
}) | |
return rendimentos | |
sumario = {'R$ Atual Total': 0, 'Rendimento %': 0, 'R$ Investido': 0} | |
rendimentos = getRendimentos() | |
for r in rendimentos: | |
sumario['R$ Atual Total'] += r['R$ Atual'] | |
sumario['R$ Investido'] += r['R$ Investido'] | |
sumario['Rendimento %'] = ((sumario['R$ Atual Total'] / sumario['R$ Investido']) - 1) * 100 | |
for m in moedas: | |
print moedas[m]['mensagem'], round(moedas[m]['valor'], 1), '%:', round(moedas[m]['%'], 1) | |
# Bitcoin em Dólar | |
print '₿ U$', round(getValor('bitfinex', 'tBTCUSD'), 2) | |
print("---") | |
for s in sumario: | |
print s + ':',round(sumario[s], 2) | |
print("---") | |
print("Refresh | refresh=true") |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Esse é um script pata o bitbar(https://github.com/matryer/bitbar/releases/tag/v1.9.2) do Mac.
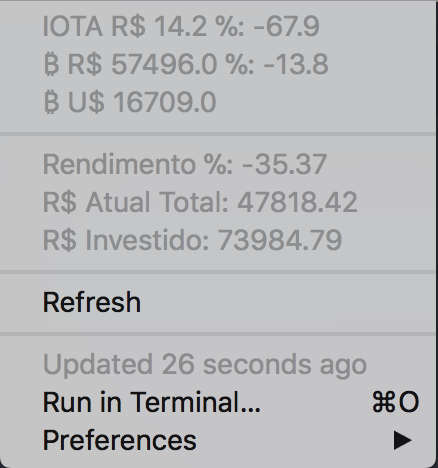
Ele informa a cotação da sua criptomoeda e rendimento. Atualmente está com suporte para as duas exchanges que eu utilizo, mas pode ser facilmente adicionado novas exchanges.
Os valores falsos acima gerarão algo como: