Last active
June 7, 2018 15:57
-
-
Save eliwjones/cdb93e7538043db64af27fad2036c54a to your computer and use it in GitHub Desktop.
Encoding permutations
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package main | |
import ( | |
"github.com/llgcode/draw2d/draw2dimg" | |
"image" | |
"image/color" | |
"math" | |
"math/rand" | |
"time" | |
) | |
func main() { | |
width := 980 | |
height := 980 | |
dest := image.NewRGBA(image.Rect(0, 0, width, height)) | |
gc := draw2dimg.NewGraphicContext(dest) | |
gc.Clear() | |
gc.SetFillColor(color.White) | |
gc.SetStrokeColor(color.Black) | |
gc.SetLineWidth(1) | |
gc.BeginPath() | |
points := circlePoints(float64(width/2), float64(height/2), float64(width/2)-10) | |
l := len(points) | |
for i := 0; i < l; i++ { | |
gc.MoveTo(points[i][0], points[i][1]) | |
gc.LineTo(points[(i+1)%l][0], points[(i+1)%l][1]) | |
} | |
gc.Close() | |
gc.FillStroke() | |
draw2dimg.SaveToPngFile("bong.png", dest) | |
} | |
func circlePoints(centerX float64, centerY float64, radius float64) [][2]float64 { | |
numPoints := 60 | |
angleIncrement := (2 * math.Pi) / float64(numPoints) | |
points := [][2]float64{} | |
var angle float64 = 0 | |
for i := 0; i < numPoints; i++ { | |
x := centerX + radius*math.Cos(angle) | |
y := centerY + radius*math.Sin(angle) | |
points = append(points, [2]float64{x, y}) | |
angle += angleIncrement | |
} | |
prefix := [][2]float64{points[len(points)-1], points[1]} | |
suffix := append(points[2:len(points)-1], points[0]) | |
rand.Seed(time.Now().UnixNano()) | |
rand.Shuffle(len(suffix), func(i, j int) { | |
suffix[i], suffix[j] = suffix[j], suffix[i] | |
}) | |
return append(prefix, suffix...) | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Trying to figure out if I can make a pretty way to encode 256 bit numbers:
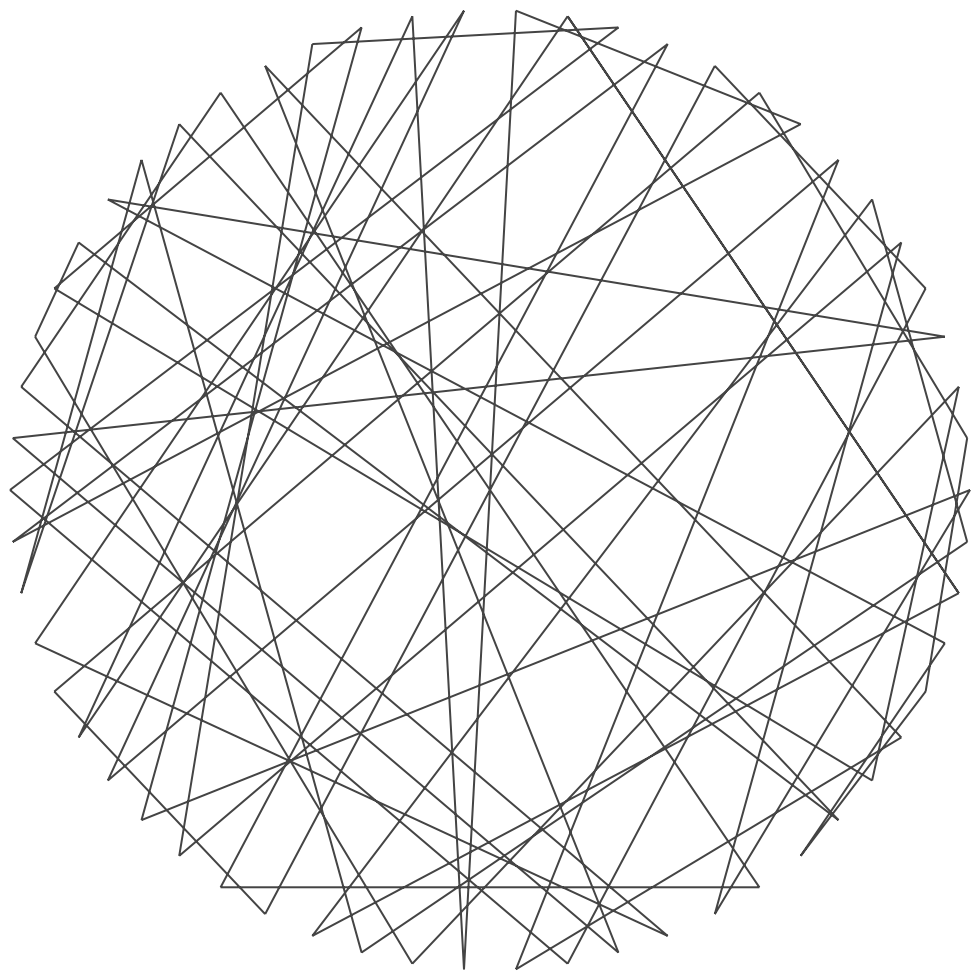