Last active
March 29, 2022 09:47
-
-
Save ervinne13/08f932682f7b06761e14b387df4e35d9 to your computer and use it in GitHub Desktop.
Laravel reset auto increment for tests with databases where you can't do a migrate:refresh
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
namespace <Your namespace here>; | |
use Illuminate\Support\Facades\DB; | |
/** | |
* | |
* @author Ervinne Sodusta <ervinne.sodusta@nuworks.ph> | |
*/ | |
trait ResetsAutoIncrementedState | |
{ | |
static $lastId; | |
static $staticConnection; | |
static $staticTable; | |
static $staticPrimaryKey; | |
public static function saveState() | |
{ | |
if (!static::$staticTable || !static::$staticPrimaryKey) { | |
$model = new static(); | |
static::$staticConnection = $model->getConnectionName(); | |
static::$staticTable = $model->getTable(); | |
static::$staticPrimaryKey = $model->getKeyName(); | |
} | |
static::$lastId = DB::connection(static::$staticConnection)->table(static::$staticTable)->max(static::$staticPrimaryKey) + 1; | |
} | |
public static function resetState() | |
{ | |
if (static::$lastId) { | |
$lastId = static::$lastId; | |
$table = static::$staticTable; | |
$connection = DB::connection(static::$staticConnection); | |
$database = $connection->getConfig('database'); | |
DB::connection(static::$staticConnection)->table(static::$staticTable)->where(static::$staticPrimaryKey, '>=', $lastId)->delete(); | |
DB::connection(static::$staticConnection)->statement("SET SESSION sql_mode='';"); | |
DB::connection(static::$staticConnection)->statement("ALTER TABLE {$database}.{$table} AUTO_INCREMENT = {$lastId}"); | |
DB::connection(static::$staticConnection)->statement("SET SESSION sql_mode='STRICT_TRANS_TABLES';"); | |
} | |
static::$lastId = null; | |
} | |
public function scopeAddedSinceSaveState($query) | |
{ | |
return $query->where($this->primaryKey, '>=', static::$lastId); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Example Usage:
Model:
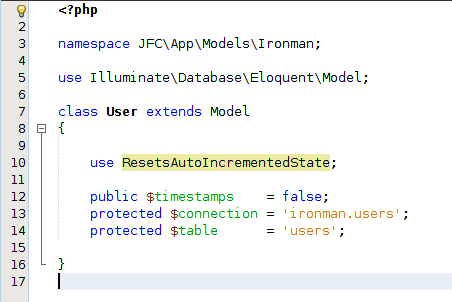
In the Test:
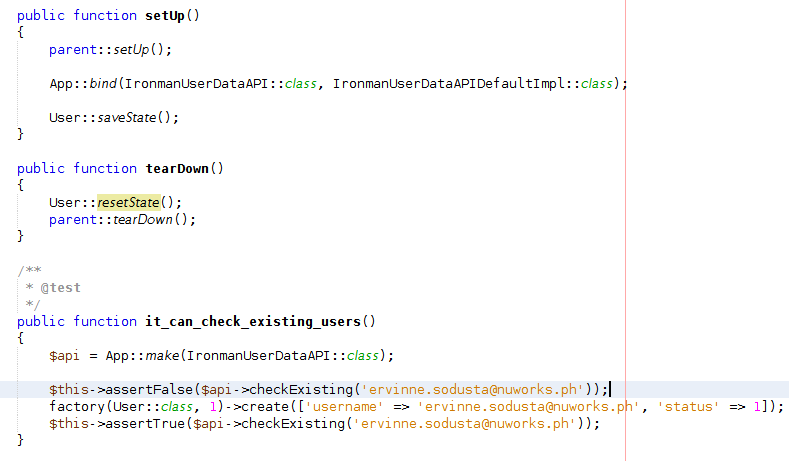